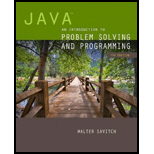
Concept explainers
Explanation of Solution
Exception class:
The exception class to find whether the time entered is valid or not. The time should be in the format “hour:minute” followed by “am” or “pm”.
InvalidFormattingException.java:
//Create a class that extends Exception
public class InvalidFormattingException extends Exception
{
//Define a parameterized constructor
public InvalidFormattingException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidFormattingException” occurs when the time entered by the user in invalid.
Complete program:
Main.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class Main
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public Main()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check condition
if(!amString.equals("am") && !amString.equals("pm"))
//Throw the exception with a message
throw new InvalidFormattingException("Did not have am/pm");
//Assign the value
hour = hourFound;
//Assign the value
minute = minuteFound;
//Check if the string is am
if(amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming plus MyProgrammingLab with Pearson eText -- Access Card Package (7th Edition)
- Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info. **This is the output i am having trouble with. on line 20 The print string shows the print(ve, 'could not calculate heart rate info.') And i know that the extra space i see at the begining of that second line of output is due to the comma but I cannot figure out how to make the same line print but with out the space from the comma after…arrow_forwardWrite a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info. **This is the output i am having trouble with. on line 20 The print string shows the print(ve, 'could not calculate heart rate info.') And i know that the extra space i see at the begining of that second line of output is due to the comma but I cannot figure out how to make the same line print but with out the space from the comma after…arrow_forwardA thrown exception may contain more information about what caused the exception. True or Falsearrow_forward
- Derive exception classes from the class you wrote in the previous exercise. Each new class should indicate a specific kind of error. For example, InvalidHourException could be used to indicate that the value entered for an hour was not an integer in the range of 1 to 12. (Also the previous answer was not preferd from the teacher cause its not simple and use def which we arent taken it)arrow_forwardJava : Write the code segments in main( ) and in methodA( ) for the following scenario: main( ) passes methodA an int variable called a. methodA generates an exception, but does not deal with it, if a is not between 1 and 100. Hint: main will try and catch the exception and methodA throws exception.arrow_forwardWhich of the following statements is false? A software developer can write his own exceptions in Java. A try statement is associated with one and only one catch statement.. A programmer written Exception implements the Exception interface. None of the above statements are falsearrow_forward
- Please do this in Java! 3. RetailItem Exceptions Programming Challenge 4 of Chapter 6 required you to write a RetailItem class that holds data pertaining to a retail item. Write an exception class that can be instantiated and thrown when a negative number is given for the price. Write another exception class that can be instantiated and thrown when a negative number is given for the units on hand. Demonstrate the exception classes in a program. /** *Description: This program will displays a string without any user interaction *Class: Fall - COSC 1437.81002 *Assignment1: Hello World *Date: 08/15/2011 *@author Zoltan Szabo *@version 0.0.0 */ For each method, you will also be required to create docstring as follows: /** * @param String as args * @return Termination code as int, 0 for normal, anything else is error condition * @throws Nothing is implemented */ Flowcharts/UML and Pseudo code All assignment questions must show design flowchart/UML and/or pseudo code unless otherwise…arrow_forwardI need to know how to do this in python: Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.arrow_forwardGiven the following code, the output is __. try { Integer number = new Integer("1"); System.out.println("An Integer instance."); } catch (Exception e) { System.out.println("An Exception."); } Group of answer choices A- An Exception. B- 1 C- Error: Exception D- An Integer instance. E- None of the optionsarrow_forward
- Modify the GreenvilleRevenue program created in Chapter 10, Case Study 1 so that it performs the following tasks: The program prompts the user for the number of contestants in this year’s competition; the number must be between 0 and 30. Use exception-handling techniques to ensure a valid value and display the error message:Number must be between 0 and 30 The program prompts the user for talent codes. Use exception-handling techniques to ensure a valid code and update the displayed message to the following message:x is not a valid talent code. Assigned as Invalid.where x was the invalid code entered into the console. After data entry is complete, the program prompts the user for codes so the user can view lists of appropriate contestants. Use exception-handling techniques for the code verification and display the following message: Enter a talent type or Z to quit >> x x is not a valid code and for valid codes: Enter a talent type or Z to quit >> S Contestants with talent…arrow_forwardModify the GreenvilleRevenue program created in Chapter 10, Case Study 1 so that it performs the following tasks: The program prompts the user for the number of contestants in this year’s competition; the number must be between 0 and 30. Use exception-handling techniques to ensure a valid value and display the error message:Number must be between 0 and 30 The program prompts the user for talent codes. Use exception-handling techniques to ensure a valid code and update the displayed message to the following message:x is not a valid talent code. Assigned as Invalid.where x was the invalid code entered into the console. After data entry is complete, the program prompts the user for codes so the user can view lists of appropriate contestants. Use exception-handling techniques for the code verification and display the following message: Enter a talent type or Z to quit >> x x is not a valid code and for valid codes: Enter a talent type or Z to quit >> S Contestants with talent…arrow_forwardWrite a program that includes the header using the pre-processor directive. Then create a class named MyException that inherits all properties from the exception class. In that class create a function what() which basically returns an error message string (“C++ Exception”). Finally inside the main function create a try{}…catch() block and inside the try block create an object of MyException class and use the throw keyword to explicitly throw an exception using this object.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
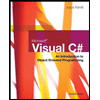
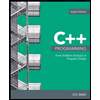
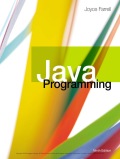
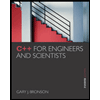