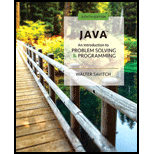
Java: An Introduction To Problem Solving And Programming Plus Mylab Programming With Pearson Etext -- Access Card Package (8th Edition)
8th Edition
ISBN: 9780134710754
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 9, Problem 3PP
Program Plan Intro
Date format converter
Program plan:
“DateFormatConverter.java”:
- Import required packages.
- Define the class “DateFormatConverter”.
- Declare required variables.
- Define an array and assign it with the months.
- Define the “main()” method.
- Do until the user enters other than “Y” or “y”.
- Inside the “try” block,
- Get the date from the user.
- Trim the input.
- Store the month value in a variable.
- Check if that value is less than 0.
-
- Assign “false” to “validInput”.
- Throw an exception.
- Check if month value is less than 1 or greater than 12.
-
- Assign “false” to “validInput”.
- Throw an exception.
- Otherwise,
-
- Get the date value and store it in a variable.
- Call the function “dayCheck()”.
- Catch “MonthException”.
- Print the error message.
- Check if the function “validInput()” returns true.
- Print the month name and its date.
- Get the response from the user whether the user wants to do it again or not.
- Inside the “try” block,
- Do until the user enters other than “Y” or “y”.
- Give function to convert string to integer for month.
- Get the position of “/”.
- Check if that position is equal to 2.
- Return the value.
- Check if that position is equal to 1.
- Return the integer value.
- Otherwise,
- Return -1.
- Give function to convert string to integer for day.
- Get the number of characters.
- Check if that number is equal to 2.
- Return the value.
- Check if that number is equal to 1.
- Return the integer value.
- Otherwise,
- Return -1.
- Function definition to convert ASCII value to integer.
- Switch to the digit.
- Assign the value according to the character digit.
- Switch to the digit.
- Give function definition to check the day.
- Inside the “try” block,
- Switch to the month number.
- If the month number is 1, 3, 5, 7, 8, 10 and 12,
-
- Check if the day is less than 1 or greater than 31.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 31.
- If the month number is 4, 6, 9 and 11,
-
- Check if the day is less than 1 or greater than 30.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 30.
- If the month number is 2,
-
- Check if the day is less than 1 or greater than 29.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 29.
- Switch to the month number.
- Inside the “catch” block for “DayException”, print the error message.
- Inside the “try” block,
“DayException.java”:
- Define the exception class “DayException”.
- Define a default constructor.
- Call the parent class’s method.
- Define two parameterized constructors.
- Call the parent class’s method by passing a message.
- Define a default constructor.
“MonthException.java”:
- Define the exception class “MonthException”.
- Define a default constructor.
- Call the parent class’s method.
- Define two parameterized constructors.
- Call the parent class’s method by passing a message.
- Define a default constructor.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a program that converts a time from 24-hour notation to 12-hour notation. To make the solution easier, a requirement is imposed on the input: It must be in xx:xx format, i.e. it must have two digits, a colon, and then another two digits. Define an exception class called TimeException. If the user enters an illegal time, like 10:65, or even gibberish, like 8&*68, your program should throw and handle a TimeException. Test your program with the file "times.txt" as input and store the result in the file "result.txt"
File times.txt:
00:00
12:00
12:01
11:59
23:59
24:00
10:65
3:23
1145
8&*68
File result.txt:
# 24-hour 12-hour
--------------------------------------------
1 00:00 12:00 AM
2 12:00 12:00 PM
3 12:01 12:01 PM
4 11:59 11:59 AM
5 23:59 11:59 PM
6 24:00 Time Exception
7 10:65…
Write a program that prompts the user to enter a length in feet and then enter a length in inches and outputs the equivalent length in centimeters. If the user enters a negative number or a non-digit number, throw and handle an appropriate exception and prompt the user to enter another set of numbers.
Your error message should read A non positive number is entered and allow the user to try again.
Format your output with setprecision(2) to ensure the proper number of decimals for testing!
Write a class TimeOfDay that uses the exception classes defined in the previous exercise. Give it a method setTimeTo(timeString) that changes the time if timeString corresponds to a valid time of day. If not, it should throw an exception of the appropriate type. [Java]
Chapter 9 Solutions
Java: An Introduction To Problem Solving And Programming Plus Mylab Programming With Pearson Etext -- Access Card Package (8th Edition)
Ch. 9.1 - Prob. 1STQCh. 9.1 - What output would the code in the previous...Ch. 9.1 - Prob. 3STQCh. 9.1 - Prob. 4STQCh. 9.1 - Prob. 5STQCh. 9.1 - Prob. 6STQCh. 9.1 - Prob. 7STQCh. 9.1 - Prob. 8STQCh. 9.1 - In the code given in Self-Test Question 1,...Ch. 9.1 - In the code given in Self-Test Question 1,...
Ch. 9.1 - Prob. 11STQCh. 9.1 - Prob. 12STQCh. 9.1 - Prob. 13STQCh. 9.1 - Prob. 14STQCh. 9.2 - Prob. 15STQCh. 9.2 - Prob. 16STQCh. 9.2 - Prob. 17STQCh. 9.2 - Prob. 18STQCh. 9.2 - Prob. 19STQCh. 9.2 - Prob. 20STQCh. 9.2 - Suppose that, in Self-Test Question 19, we change...Ch. 9.2 - Prob. 22STQCh. 9.2 - Prob. 23STQCh. 9.3 - Prob. 24STQCh. 9.3 - Prob. 25STQCh. 9.3 - Prob. 26STQCh. 9.3 - Prob. 27STQCh. 9.3 - Prob. 28STQCh. 9.3 - Repeat Self-Test Question 27, but change the value...Ch. 9.3 - Prob. 30STQCh. 9.3 - Prob. 31STQCh. 9.3 - Prob. 32STQCh. 9.3 - Consider the following program: a. What output...Ch. 9.3 - Write an accessor method called getPrecision that...Ch. 9.3 - Prob. 35STQCh. 9.4 - Prob. 36STQCh. 9.4 - Prob. 37STQCh. 9.4 - Prob. 38STQCh. 9 - Write a program that allows students to schedule...Ch. 9 - Prob. 2ECh. 9 - Prob. 3ECh. 9 - Prob. 4ECh. 9 - Prob. 5ECh. 9 - Write code that reads a string from the keyboard...Ch. 9 - Create a class Rational that represents a rational...Ch. 9 - Prob. 9ECh. 9 - Suppose that you are going to create an object...Ch. 9 - Revise the class RoomCounter described in the...Ch. 9 - Prob. 12ECh. 9 - Write a class LapTimer that can be used to time...Ch. 9 - Prob. 1PCh. 9 - Prob. 2PCh. 9 - Prob. 3PCh. 9 - Write a program that uses the class calculator in...Ch. 9 - Prob. 3PPCh. 9 - Prob. 7PPCh. 9 - Suppose that you are in change of customer service...Ch. 9 - Write an application that implements a trip-time...
Knowledge Booster
Similar questions
- Write a method that will take in a time in 24 hour format (1430) and return the time in 12 hour format (2:30pm). This method should have appropriate exception handling. If an incorrect time is sent as an argument (something out of bounds or 23(#4k in other words garbage), then your method should throw a TimeFormatException which you define. In no case should your method throw an unhandled exception! Show how to test the method to be sure.arrow_forwardWrite a program that prompts the user to enter a person’s date of birthin numeric form such as 8-27-1980. The program then outputs thedate of birth in the form: August 27, 1980. Your program must containat least two exception classes: invalidDay and invalidMonth.If the user enters an invalid value for day, then the program shouldthrow and catch an invalidDay object. Follow similar conventions forthe invalid values of month and year. (Note that your program musthandle a leap year.)arrow_forwardSolve In Python Provide Screenshots of Input and Ouput For this lab, you will modify your Lab 7 to make several improvements to the getter/settermethods. Instead of having them return True/False, modify each one to throw an exception ifinvalid data is passed.Modify these methods in the following way:• add_hours()o If the number of hours being added is less than 0, throw an exception• add_sales()o If the amount of sales being added is less than 0, throw an exception• set_employee_number()o The employee number must be an integer. If the given input is not an integer,throw an exception• set_office_number()o If the office number given is less than 100 or greater than 500, throw anexception.• set_name()o If the given name is empty, throw an exceptiono Any of the following characters should be removed from the name: ‘_’, ‘.’, ‘-‘ (Underscore, period, and dash)• set_birthdate()o If the given value for the month is less than 1 or greater than 12, throw anexceptiono If the given value for the…arrow_forward
- Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError as a variable and output "Zero Division Exception: " followed by the exception message from the variable. Use another except block to catch any ValueError caused by invalid input as a variable and output "Input Exception: " followed by the exception message from the variable. Note: ZeroDivisionError is raised when a division by zero happens. ValueError is raised when a user enters a value of different data type than what is defined in the program. Do not include code to raise any exception in the program. (in Python)arrow_forwardWrite a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError as a variable and output "Zero Division Exception: " followed by the exception message from the variable. Use another except block to catch any ValueError caused by invalid input as a variable and output "Input Exception: " followed by the exception message from the variable. Note: ZeroDivisionError is raised when a division by zero happens. ValueError is raised when a user enters a value of different data type than what is defined in the program. Do not include code to raise any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of…arrow_forwardWhat is the difference between using @Test(expected = TypeOfException) and using try/catch when testing if a method or constructor properly returns an exception? A. They do the same thing. B. @Test(expected = ...) is the proper way to unit test in java, and try/catch should only be used when unit testing in Python. C. @Test(expected = ...) will give a passing test as soon as one exception of the proper type is thrown and then stop the test, but using try/catch will allow you to test many examples in the same test and will only stop after running all examples or reaching a fail() or an assert statement that fails. D. We should never use try/catch when unit testing, otherwise we will catch the exception and then won't know if it is actually thrown.arrow_forward
- Assume you are considering writing a method and are deciding what should happen when given input that is outside of perfect input. Which of the following is not a way to handle this? Group of answer choices 1. Use better JUnit testing 2. Throw an exception 3. Try to reasonably auto-correct 4. Prevent the errorarrow_forwardWrite a JAVA program to calculate percentage of a student for 3 different subjects. Create an exception if the marks entered greater than 100.arrow_forwardC++Write a program SEGMENT that does the following: Asks the user for his/her birthday using 2 integer values, i.e., int month, int year. Using a try/catch block with two catch blocks, check for the following exceptions: Invalid month (i.e., a month less than or equal to 0 or greater than 12). Throw a string exception to be caught by a catch block accepting a string. Output the message thrown by the exception indicating the month is an invalid month. Year less than 1900 or greater than 2020. Throw an integer exception to be caught by a catch block accepting an integer. In the catch block, if the integer thrown is less than 1900, output the message “You are very old”. If the integer exception is greater than 2020, output the message “You have not been born yet!” If neither exception occurs, simply output (cout) a message with the user’s birth month and year, for example, “You were born in “ << month << “ and “ << year. Declare any variables needed.…arrow_forward
- *This is a handout from my class that I'm having trouble with I tried YouTube videos and I'm still lost please explain the answers as simply as you can and thank you.* A. Look over the following code fragment, give the output: try { method(); System.out.println("After the method call"); } catch (Exception ex) { System.out.println("Exception in main"); } catch (RuntimeException ex) { System.out.println("RuntimeException in main"); } static void method() throws Exception { System.out.println(1 / 0); } B. Write the code to load an array. Use simple I/O (system.out.print, etc.) to get integer values from the user and load the array (loop?). Your code has the potential to generate 2 exceptions: 1) InputMismatch (research this) and 2) IllegalValueException (assume this exists). If the 1st occurs, change the input to be 99, warn the user and continue. The 2nd exception will occur when the user enters a 0; if this occurs, notify the user that a 0 has been entered, and change the input to…arrow_forwardOnly new java code can be added after the code given: The while loop makes multiple attempts to read a nonnegative integer from input into userAge. Use multiple exception handlers to: Catch an InputMismatchException, output "Unexpected input: The UserAge program quits", and assign retry with false. Catch an Exception and output the message of the Exception. End each output with a newline. Ex: If the input is 44, then the output is: Valid input: User's age is 44 Ex: If the input is L, then the output is: Unexpected input: The UserAge program quits Ex: If the input is -65 44, then the output is: User's age must be nonnegative Valid input: User's age is 44 import java.util.Scanner;import java.util.InputMismatchException; public class UserAge { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int userAge; boolean retry = true; while (retry) { try { userAge = scnr.nextInt(); if (userAge < 0) {…arrow_forwardin java Write a program that reads integers userNum and divNum as input, and output the quotient (userNum divided by divNum). Use a try block to perform the statements. Use a catch block to catch any ArithmeticException and output an exception message with the getMessage() method. Use another catch block to catch any InputMismatchException and output an exception message with the toString() method. Note: ArithmeticException is thrown when a division by zero happens. InputMismatchException is thrown when a user enters a value of different data type than what is defined in the program. Do not include code to throw any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Arithmetic Exception: / by zero Ex: If the input of the program is: 15.5 5 the output of the program is: Input Mismatch Exception: java.util.InputMismatchException LabProgram.java…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
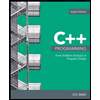
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning