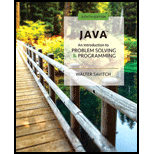
Concept explainers
Explanation of Solution
Creating “TimeOfDay.java”:
- Import required packages.
- Define the “TimeOfDay” class.
- Declare required variables.
- Define the constructor and instantiate the variables.
- Give function definition “setTimeTo ()” to set the time.
- Declare required variables.
- Create an object for the “Scanner” class.
- Split the string using a delimiter “:”.
- Inside the “try” block,
- Get the hour and store it in a variable.
- Catch the exception “Exception”.
- Throw the exception “InvalidFormattingException” with a message.
- Check if the hour value is valid.
- Throw “InvalidHourException” with a message.
- Get the remaining string and cut off the last two characters.
- Check if the string’s length is less than 3.
- Throw “InvalidFormattingException” with a message.
- Get the minutes and store it in a variable.
- Get “am” or “pm” and store it in a variable.
- Inside “try” block,
- Convert the minute string to integer.
- Catch the exception “Exception”.
- Throw “InvalidFormattingException” with a message.
- Check if minute is less than 0 or greater than 59.
- Throw “InvalidMinuteException” with a message.
- Check whether the string is not equal to “am” and “pm”.
- Throw “InvalidFormattingException” with a message.
- Check if the string is equal to “am”.
- Assign “true” to “isAM”.
- Else,
- Assign “false” to “isAM”.
- Function to produce the resultant string.
- Append all the value to a string.
- Define the “main ()” method.
- Create an object for the “Scanner” class.
- Create an object for the class “TimeOfDay”.
- Declare a variable “time”.
- Inside “try” block.
- Get the time from the user.
- Call the function “setTimeTo ()” by passing the time.
- Catch the exception “InvalidFormattingException”.
- Print the message using “getMessage ()” function.
- Catch the exception “InvalidHourException”.
- Print the message using “getMessage ()” function.
- Catch the exception “InvalidMinuteException”.
- Print the message using “getMessage ()” function.
- Print the time.
Creating “InvalidFormattingException.java”:
- Define a class “InvalidFormattingException” that extends “Exception”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Creating “InvalidHourException.java”:
- Define a class “InvalidHourException” that extends “InvalidFormattingException”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Creating “InvlaidMinuteException.java”:
- Define a class “InvalidMinuteException” that extends “InvalidFormattingException”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Program:
TimeOfDay.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class TimeOfDay
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public TimeOfDay()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException, InvalidHourException, InvalidMinuteException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Check the condition
if(hourFound<1 || hourFound>12)
//Throw the exception with a message
throw new InvalidHourException("Hour not in the range of 1 to 12");
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check the condition
if(minuteFound<0 || minuteFound>59)
//Throw the exception with a message
throw new InvalidMinuteException("Minute not in the range of 0 to 59");
//Check condition
if(!amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction To Problem Solving And Programming Plus Mylab Programming With Pearson Etext -- Access Card Package (8th Edition)
- Write a program that calls a method that throws an exception of type ArithmeticExcepton at arandom iteration in a for loop. Catch the exception in the method and pass the iteration countwhen the exception occurred to the calling method by using an object of an exception class youdefinein java codearrow_forwardWrite a method that will take in a time in 24 hour format (1430) and return the time in 12 hour format (2:30pm). This method should have appropriate exception handling. If an incorrect time is sent as an argument (something out of bounds or 23(#4k in other words garbage), then your method should throw a TimeFormatException which you define. In no case should your method throw an unhandled exception! Show how to test the method to be sure.arrow_forwardCreate a class Int that contain an integer variable. Overload the following integer arithmetic operators (+, -, *, /), so that they operate an object of type Int. If the result of any such operation exceeds the normal range of int from-32768 to 32767 or in division operation if the denominator is less than 1, throw an exception including error message and error code encapsulated in exception class object. In relevant catch properly show the error message and error code followed by termination of program in c++.arrow_forward
- What is the difference between using @Test(expected = TypeOfException) and using try/catch when testing if a method or constructor properly returns an exception? A. They do the same thing. B. @Test(expected = ...) is the proper way to unit test in java, and try/catch should only be used when unit testing in Python. C. @Test(expected = ...) will give a passing test as soon as one exception of the proper type is thrown and then stop the test, but using try/catch will allow you to test many examples in the same test and will only stop after running all examples or reaching a fail() or an assert statement that fails. D. We should never use try/catch when unit testing, otherwise we will catch the exception and then won't know if it is actually thrown.arrow_forward*This is a handout from my class that I'm having trouble with I tried YouTube videos and I'm still lost please explain the answers as simply as you can and thank you.* A. Look over the following code fragment, give the output: try { method(); System.out.println("After the method call"); } catch (Exception ex) { System.out.println("Exception in main"); } catch (RuntimeException ex) { System.out.println("RuntimeException in main"); } static void method() throws Exception { System.out.println(1 / 0); } B. Write the code to load an array. Use simple I/O (system.out.print, etc.) to get integer values from the user and load the array (loop?). Your code has the potential to generate 2 exceptions: 1) InputMismatch (research this) and 2) IllegalValueException (assume this exists). If the 1st occurs, change the input to be 99, warn the user and continue. The 2nd exception will occur when the user enters a 0; if this occurs, notify the user that a 0 has been entered, and change the input to…arrow_forwardIn Java when a method throws a checked Exception, what must we always do when calling that method? A. Make sure to declare the method as static. B. Put it inside a try/catch block. C. Set the permission level of the method to private. D. Check our input, and if it will be bad input we must throw an exception before calling the method.arrow_forward
- Solve In Python Provide Screenshots of Input and Ouput For this lab, you will modify your Lab 7 to make several improvements to the getter/settermethods. Instead of having them return True/False, modify each one to throw an exception ifinvalid data is passed.Modify these methods in the following way:• add_hours()o If the number of hours being added is less than 0, throw an exception• add_sales()o If the amount of sales being added is less than 0, throw an exception• set_employee_number()o The employee number must be an integer. If the given input is not an integer,throw an exception• set_office_number()o If the office number given is less than 100 or greater than 500, throw anexception.• set_name()o If the given name is empty, throw an exceptiono Any of the following characters should be removed from the name: ‘_’, ‘.’, ‘-‘ (Underscore, period, and dash)• set_birthdate()o If the given value for the month is less than 1 or greater than 12, throw anexceptiono If the given value for the…arrow_forwardCreate a class Int in oop c++ that contain an integer variable.Overload the following integer arithmetic operators (+, -, *, /), so that they operate an object of type Int. Ifthe result of any such operation exceeds the normal range of int from-32768 to 32767 or in divisionoperation if the denominator is less than 1, throw an exception including error message and error codeencapsulated in exception class object. In relevant catch properly show the error message and error codefollowed by termination of program.arrow_forwardWrite a class called MyException with appropriate attributes and methods toprocess different types of exceptions thrown in C++ and add proper comments in the code.thanksarrow_forward
- Write a JAVA program that reads an array from input file and invokes twodifferent methods Sort and Max ,that sorts the elements of the array and findsthe Max element and writes out the resulted array in to output file . Usetwo interfaces for methods and throw Exception Handling for Out Of Boundindex for the array.arrow_forwardin java Define the class InvalidSideException, which inherits from the Exception class. Also define a Square class, which has one method variable -- an int describing the side length. The constructor of the Square class should take one argument, an int meant to initialize the side length; however, if the argument is not greater than 0, the constructor should throw an InvalidSideError. The Square class should also have a method getArea(), which returns the area of the square.Create a Driver class with a main method to test your classes. Your program should prompt the user to enter a value for the side length, and then create a Square object with that side length. If the side length is valid, the program should print the area of the square. Otherwise, it should catch the InvalidExceptionError, print "Side length must be greater than 0.", and terminate the program. screenshot shows outputarrow_forwardIn Java. Define the class InvalidSideException, which inherits from the Exception class. Also define a Square class, which has one method variable -- an int describing the side length. The constructor of the Square class should take one argument, an int meant to initialize the side length; however, if the argument is not greater than 0, the constructor should throw an InvalidSideError. The Square class should also have a method getArea(), which returns the area of the square.Create a Driver class with a main method to test your classes. Your program should prompt the user to enter a value for the side length, and then create a Square object with that side length. If the side length is valid, the program should print the area of the square. Otherwise, it should catch the InvalidExceptionError, print "Side length must be greater than 0.", and terminate the program. The words for output is highlighted in grey and yellow in the blue box. Class should be named Driver, as shown in blue box…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
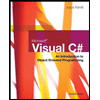