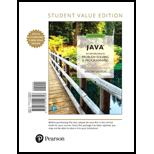
Explanation of Solution
Exception classes:
The two classes that derives “InvalidFormattingException” are “InvalidHourException” and “InvalidMinuteException”.
InvalidHourException.java:
//Create a class that extends Exception
public class InvalidHourException extends InvalidFormattingException
{
//Define a parameterized constructor
public InvalidHourException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidHourException” extends “InvalidFormattingException” and this exception occurs when the hour entered by the user in invalid.
InvalidMinuteException.java:
//Create a class that extends Exception
public class InvalidMinuteException extends InvalidFormattingException
{
//Define a parameterized constructor
public InvalidMinuteException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidMinuteException” extends “InvalidFormattingException” and this exception occurs when the minute entered by the user in invalid.
Complete program:
Main.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class Main
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public TimeOfDay()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException, InvalidHourException, InvalidMinuteException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Check the condition
if(hourFound<1 || hourFound>12)
//Throw the exception with a message
throw new InvalidHourException("Hour not in the range of 1 to 12");
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check the condition
if(minuteFound<0 || minuteFound>59)
//Throw the exception with a message
throw new InvalidMinuteException("Minute not in the range of 0 to 59");
//Check condition
if(!amString.equals("am") && !amString.equals("pm"))
//Throw the exception with a message
throw new InvalidFormattingException("Did not have am/pm");
//Assign the value
hour = hourFound;
//Assign the value
minute = minuteFound;
//Check if the string is am
if(amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java Format: Unbound (saleable)
- Write a program that converts a time from 24-hour notation to 12-hour notation. To make the solution easier, a requirement is imposed on the input: It must be in xx:xx format, i.e. it must have two digits, a colon, and then another two digits. Define an exception class called TimeException. If the user enters an illegal time, like 10:65, or even gibberish, like 8&*68, your program should throw and handle a TimeException. Test your program with the file "times.txt" as input and store the result in the file "result.txt" File times.txt: 00:00 12:00 12:01 11:59 23:59 24:00 10:65 3:23 1145 8&*68 File result.txt: # 24-hour 12-hour -------------------------------------------- 1 00:00 12:00 AM 2 12:00 12:00 PM 3 12:01 12:01 PM 4 11:59 11:59 AM 5 23:59 11:59 PM 6 24:00 Time Exception 7 10:65…arrow_forwardIn Java when a method throws a checked Exception, what must we always do when calling that method? A. Make sure to declare the method as static. B. Put it inside a try/catch block. C. Set the permission level of the method to private. D. Check our input, and if it will be bad input we must throw an exception before calling the method.arrow_forwardThe following code is causing an exception during the runtime. class test{ public static void main(String[] args) { Object o = "Hello"; Object s = new Random(); m(o); m(s); } public static void m(Object o) { String s = (String)o; System.out.println(s.length()); }} Exception can be prevented by adding an if statement and type casting in the method m Exception can be handled by adding try-catch in the main method answers A or B both works Non of the answersarrow_forward
- Write a JAVA PROGRAM to create a user defined exception class. In this, a constructor of InvalidGradeException accepts a string as an argument. This string is passed to constructor of parent class Exception using the super () method. Also the constructor of Exception class can be called without using a parameter and calling super () method is not mandatory. WRITE JAVA PROGRAM only, and give screenshot of sample runarrow_forwardGiven the following code, the output is __. try { Integer number = new Integer("1"); System.out.println("An Integer instance."); } catch (Exception e) { System.out.println("An Exception."); } Group of answer choices A- An Exception. B- 1 C- Error: Exception D- An Integer instance. E- None of the optionsarrow_forwardWrite a class called MyException with appropriate attributes and methods toprocess different types of exceptions thrown in C++ and add proper comments in the code.thanksarrow_forward
- What is exception propagation? Give an example of a class that contains at least two methods, in which one method calls another. Ensure that the subordinate method will call a predefined Java method that can throw a checked exception. The subordinate method should not catch the exception. Explain how exception propagation will occur in your example.arrow_forwardWrite a program that calls a method that throws an exception of type ArithmeticExcepton at arandom iteration in a for loop. Catch the exception in the method and pass the iteration countwhen the exception occurred to the calling method by using an object of an exception class youdefinein java codearrow_forwardWhat is the difference between using @Test(expected = TypeOfException) and using try/catch when testing if a method or constructor properly returns an exception? A. They do the same thing. B. @Test(expected = ...) is the proper way to unit test in java, and try/catch should only be used when unit testing in Python. C. @Test(expected = ...) will give a passing test as soon as one exception of the proper type is thrown and then stop the test, but using try/catch will allow you to test many examples in the same test and will only stop after running all examples or reaching a fail() or an assert statement that fails. D. We should never use try/catch when unit testing, otherwise we will catch the exception and then won't know if it is actually thrown.arrow_forward
- Hello. Please explain the ‘f()’ portion of the Java code below. If it is a function, where is it defined? What does it do in this context? Thanks, JZ // exceptions/InheritingExceptions.java // Creating your own exceptions class SimpleException extends Exception {} public class InheritingExceptions { public void f() throws SimpleException { System.out.println( "Throw SimpleException from f()"); throw new SimpleException(); } public static void main(String[] args) { InheritingExceptions sed = new InheritingExceptions(); try { sed.f(); } catch(SimpleException e) { System.out.println("Caught it!"); } } } /* Output: Throw SimpleException from f() Caught it! */arrow_forwardWrite an exception class that is appropriate for indicating that a time enteredby a user is not valid. The time will be in the format hour:minute followed by“am” or “pm.”arrow_forwardWrite a method that will take in a time in 24 hour format (1430) and return the time in 12 hour format (2:30pm). This method should have appropriate exception handling. If an incorrect time is sent as an argument (something out of bounds or 23(#4k in other words garbage), then your method should throw a TimeFormatException which you define. In no case should your method throw an unhandled exception! Show how to test the method to be sure.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
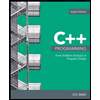