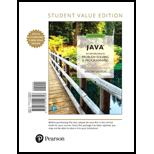
Explanation of Solution
Creating a class “RationalAssert.java”:
- Import required package.
- Define the class “RationalAssert”.
- Declare the private variables “the_numerator”, and “the_denominator”.
- Define parameterized constructor.
- Assign “numerator” to “the_numerator”.
- Assign “denominator” to “the_denominator”.
- Create an assertion with the condition “assert the_denominator != 0”.
- Give mutator methods to set numerator and denominator.
- Give accessor methods to get the value of numerator and denominator.
- Give function definition for “value ()”.
- Return the value produced by “the_numerator / the_denominator”.
- Given function “toString ()”.
- Return the string “the_numerator / the_denominator”.
- Define the “main ()” method.
- Create an object for “Scanner” class.
- Declare the variables “n”, and “d”.
- Get the numerator and denominator from the user.
- Call the constructor by passing “n”, and “d”.
- Call the function “value”.
- Call the function to get the numerator and denominator.
- Print new line.
Program:
Rational.java:
import java.util.Scanner;
//Define a class
public class RationalAssert
{
//Declare required variables
private int the_numerator;
private int the_denominator;
//Parameterized constructor
public RationalAssert(int numerator, int denominator)
{
//Assign the value of numerator
the_numerator = numerator;
//Assign the value of denominator
the_denominator = denominator;
//Declare an assertion
assert the_denominator != 0;
}
//Function to set the value of numerator
public void setNumerator(int numerator)
{
//Set the value
the_numerator = numerator;
}
//Function to set the denominator
public void setDenominator(int denominator)
{
//Declare an assertion
assert the_denominator != 0;
//Assign the value
the_denominator = denominator;
}
�...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java Format: Unbound (saleable)
- What precisely does it mean when an operator or function is overloaded? What are its beneficial attributes? How can the user be prevented from committing mistakes via the use of programmed exception handling strategies?arrow_forwardPLZ help with the following: IN JAVA Given the following code (where the function FunTest() and the main method are inside one class in the same file where classes X, Y and Z are), predict what is the output?arrow_forwardWhat exactly does it imply when someone overloads an operator or a function? What are the positive aspects of it? How can the user be safeguarded from making errors via the usage of various exception handling strategies that may be programmed?arrow_forward
- Complete the following Java program by typing in the missing statements in the space provided. This program inputs a value/s from user, and displays the result of some operation. If the operation generated an exception / error, it is handled by the user defined exception class. The main method in the application class is declared to throw a user-defined exception which will take care of the exception. import // for input from keyboard public class MyExceptionApp { public static void main( ) throws MyDivByZeroException { Scanner kb = System.out.println("Enter two numbers for division : "); double n1 = kb.nextDouble(); double n2 = kb.nextDouble(); try{ if( n2 == 0 ) throw else System.out.println("Answer = " + n1/n2); } catch ( e){ System.out.println("Exception caught : " + e); }…arrow_forwardWrite a JAVA PROGRAM to create a user defined exception class. In this, a constructor of InvalidGradeException accepts a string as an argument. This string is passed to constructor of parent class Exception using the super () method. Also the constructor of Exception class can be called without using a parameter and calling super () method is not mandatory. WRITE JAVA PROGRAM only, and give screenshot of sample runarrow_forwardLet's revisit chapter 5 example, but this time, no method can throw any exception out of the method and if an exception was detected then you will have to throw your own custom exception to let the user know what happened. public class Chapter11Demo{ public static void main(java.lang.String[] args) {method1();}private static void method1(){ method2();}private static void method2(){ method3();}private static void method3(){ method4();}private static void method4(){ method5();}private static void method5(){ method6();}private static void method6(){ java.io.File in=new java.io.File("somefile.txt"); java.io.File out=new java.io.File("somefile2.txt"); java.util.Scanner inFile=new java.util.Scanner(in); java.io.FileWriter outFile=new java.io.FileWriter(out); }}arrow_forward
- Write a class called MyException with appropriate attributes and methods toprocess different types of exceptions thrown in C++ and add proper comments in the code.thanksarrow_forwardWrite a java program using OOP concept (including data members with their suitable modifier, two constructers (default, and with parameters, and setter and getter methods) to print a student card including university name (Univesiti Tenaga Nasional) student name (string) and student id (string) that will be given by the user. Your Program should have at least two classes Main class to display the student card and StudentCard class to create objects. Your program should also apply exception to ensure valid input, if input is invalid ask the user again to insert until valid input is givenarrow_forwardWrite the code example in java to handle the “Exception” when the user istrying to give input this age as a float value from console whereas your codetakes input as integer.arrow_forward
- What is exception propagation? Give an example of a class that contains at least two methods, in which one method calls another. Ensure that the subordinate method will call a predefined Java method that can throw a checked exception. The subordinate method should not catch the exception. Explain how exception propagation will occur in your example.arrow_forwardJava give an example with exception handled and not ha [arrow_forwardIf a programming language does not provide exception-handling facilities, it is common to have subprograms indicate an error either through return value or through an error out parameter. What are some disadvantages of this approach comapared to exception-handling facilities provided by programming languages such as C++ and Java?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
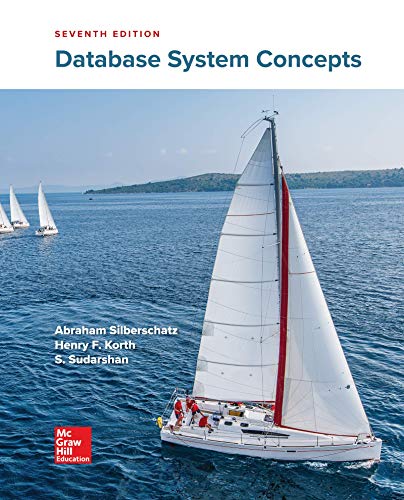
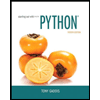
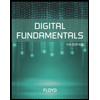
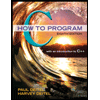
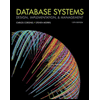
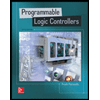