
Analyzable Interface
Modify the CourseGrades class you created in
public interface Analyzable
{
double getAverage();
GradedActivity getHighest();
GradedActivity getLowestf();
}
The getAverage method should return the average of the numeric scores stored in the grades array. The getHighest method should return a reference to the element of the grades array that has the highest numeric score. The getLowest method should return a reference to the element of the grades array that has the lowest numeric score. Demonstrate the new methods in a complete program.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Starting Out with Java: Early Objects (6th Edition)
Additional Engineering Textbook Solutions
Starting Out With Visual Basic (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with Python (4th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- Java program: 1. Write a PaypalAccount class to include both balance and accountID as the instance variables. Make sure each instance of this account will have a unique accountID. In other words, different account object should have different accountID (hint: class variable). 2. Write a Bank class with main method. In the main method, ask the user to input how many accounts (say numOfAccount) to be generated in the bank (assuming less than 1000). Then create an array to hold these numOfAccount of Account objects. For eachAccount object, generate a random balance in the range of 0.0-1000.0. Assume that your campus ID is 141-88-2014; search the array to see if there is an account with accountID as 141 (the first three digits of your campus ID). If there is not an account with accountID as 141, then set the accountID of the last account in the array as 141; transfer all the balance of the first account to the account with accountID of 141. Set the balance of the account with accountID…arrow_forwardURGENtt!! You are asked to write a java class named "Kume". The UML class diagram of the Kume class is as follows. 3 The elements in the set are kept in an ArrayList. The constructor of the class takes an array of int and adds the elements of this array to the ArrayList named al. The add() method adds an integer it takes as a parameter to the get element. The delete() method deletes an Integer object that it takes as a parameter from the get element, returns true if it can be deleted, otherwise false. The print() function prints the elements of the set to the console. The compareTo() method is inherited from the Object class and is suppressed, and compares the get element of the Set object, which it takes as a parameter, with the retrieve element of the current set object in terms of content (the order of the elements is not important). It returns 1 if both sets contain the same elements, 0 otherwise. Below is a test code that creates two objects from the Kume class and performs…arrow_forwardYou are required to design an Movie class which has following attributes String title; String director; Int duration; String release_Date; Provide default & Parameterized constructors. Provide getters & setters for data members. Provide a toString() method to print values. Now create an array of Movie store values in it (take values form user). After that you have to print the following All the movies in the array. Movie’s whose name starts with ‘a’; Movie with longest duration. All the movies having release date before 25-3-2021.arrow_forward
- Part 1: You are required to build an application to assist in the conduct of Assessments. The class Assessment represent the notion of any kind of assessment including Quiz, Assignment and Examination. Please follow the given steps to implement the applications 1. Construct a class Assessment with the following attributes a. Id (int) b. Topic(String) c. MaxMarks(double) d. Date of conduct (doc) (GregorianCalendar) e. attempts ArrayList //an array list that will contain the Attempts objects 2. In order to complete the above class you will have to write a class Attempt with the following attributes. This class represents the attempts that students have made against this assessment. a. studentId(int) b. studentName(String) c. marksObtained(double) 3. Provide constructor in Attempt class with values for all attributes 4. Provide getters and setters for each 5. Provide a toString method that produces the following string Student = --id-- : name Marks Obtained : --marks— 6. Now complete the…arrow_forwardDefine a class called Country. A Country has populationSize, area, capital, currency, and abbreviation (2-3 letters taken from the country name). Define at least two constructors, at least two getter methods, at least two setter methods, and Override the toString() method and the equals() method. The equals() method must return true if the two countries have the same name and same area and false otherwise. 1) Write a main method, define an array of country objects 2) Display countries whose populationSize is greater than one million.arrow_forwardExcercise: Listed next is the skeleton for a class named City. Each city has a name and temperature: public class City { private String cityName; private int temperature; } Flesh out the class with appropriate accessors, constructors, and mutators. Next, modify the class so that it implements the Comparable interface. The order between instances of the City class depends on the temperature. Test your class by creating an array of sample cities and sort them in an ascending order using Arrays.sort Notes: The language used for this question is Java. So far, this is the City class after fleshing it out: public class City implements Comparable<City> {private String cityName;private double temperature; public City() {} public City(String cityName, double temperature) {this.cityName = cityName;this.temperature = temperature;} public String getCityName() {return cityName;} public double getTemperature() {return temperature;} public void setName(String cityName)…arrow_forward
- Design a new Account class as follows:■■ Add a new data field name of the String type to store the name of thecustomer.■■ Add a new constructor that constructs an account with the specified name, id,and balance.■■ Add a new data field named transactions whose type is ArrayList thatstores the transaction for the accounts. Each transaction is an instance of theTransaction class, which is defined as shown in Figure ■■ Modify the withdraw and deposit methods to add a transaction to thetransactions array list. Write a test program that creates an Account with annual interest rate 1.5%,balance 1000, id 1122, and name George. Deposit $30, $40, and $50 to theaccount and withdraw $5, $4, and $2 from the account. Print an account summarythat shows the account holder name, interest rate, balance, and all transactions.arrow_forwardIn Assignment 4, you created a Card class that represents a standard playing card. Use this to design and implement a class called DeckOfCards that stores 52 objects of the Card class using an array. Include methods to shuffle the deck, deal a card, return the number of cards left in the deck, and a toString to show the contents of the deck. The shuffle methods should assume a full deck. Document your design with a UML Class diagram. Create a separate driver class that first outputs the populated deck to prove it is complete, shuffles the deck, and then deals each card from a shuffled deck, displaying each card as it is dealt along with the number of cards left in the deck. Hint: The constructor for DeckOfCards should have nested for loops for the face values (1 to 13) within the suit values (1 to 4) calling the two parameter constructor. The shuffle method does not have to simulate how a deck is physically shuffled; you can achieve the same effect by repeatedly swapping pairs of…arrow_forwardIn Assignment 4, you created a Card class that represents a standard playing card. Use this to design and implement a class called DeckOfCards that stores 52 objects of the Card class using an array. Include methods to shuffle the deck, deal a card, return the number of cards left in the deck, and a toString to show the contents of the deck. The shuffle methods should assume a full deck. Document your design with a UML Class diagram. Create a separate driver class that first outputs the populated deck to prove it is complete, shuffles the deck, and then deals each card from a shuffled deck, displaying each card as it is dealt along with the number of cards left in the deck. Hint: The constructor for DeckOfCards should have nested for loops for the face values (1 to 13) within the suit values (1 to 4) calling the two parameter constructor. The shuffle method does not have to simulate how a deck is physically shuffled; you can achieve the same effect by repeatedly swapping pairs of…arrow_forward
- ShoppingCart.java - Class definition ShoppingCartManager.java - Contains main() method Build the ShoppingCart class with the following specifications. Private fields String customerName - Initialized in default constructor to "none" String currentDate - Initialized in default constructor to "January 1, 2016" ArrayList cartItems Default constructor Parameterized constructor which takes the customer name and date as parameters Public member methods getCustomerName() accessor getDate() accessor addItem() Adds an item to cartItems array. Has a parameter of type ItemToPurchase. Does not return anything. removeItem() Removes item from cartItems array. Has a string (an item's name) parameter. Does not return anything. If item name cannot be found, output a message: Item not found in cart. Nothing removed. modifyItem() Modifies an item's description, price, and/or quantity. Has a parameter of type ItemToPurchase. Does not return anything. If item can be found (by name) in cart,…arrow_forwardDefine a class called Book. The Book class should store attributes such as the title, ISBN, author, edition, publisher, and year of publication. These attributes must be private. Provide public get/set methods in this class to access these attributes. Test your Book class by creating several books and displaying the attributes. Define a class called Bookshelf, which contains only a main() method. The Bookshelf class must create a dozen (12) Book objects with distinct attributes, and it must store them in an ArrayList of Books. The Bookshelf class must then list all the attributes of all books in the ArrayList in the order they were entered into the ArrayList. Create a sort function for Bookshelf, which will sort books in the ArrayList in ascending order by name, and then by year of publication.arrow_forwardYou are required to design an item class which has following attributes String name; Int stock; Int price; String expiry_Date; Provide default & Parameterized constructors. Provide getters & setters for data members. Provide a toString() method to print values. Now create an array of items store values in it (take values form user). After that you have to print the following All the items in the array. items whose name starts with ‘a’; All sold out items (whose stock is zero). All the items having expire date before 2-11-2020. Solve this using Simple java, arrays in java and string methods if required.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
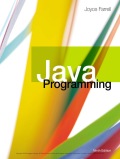
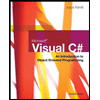