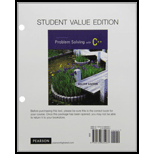
Pointer in C++:
A pointer is a variable whose value will be another variable’s address. Generally, a pointer variable is declared as follows:
type *var-name;
Here, type is the pointer’s base type and var-name is the pointer variable name. The asterisk is used to designate a variable as a pointer.
Given Program:
//Include libraries
#include <iostream>
//Use namespace
using namespace std;
//Define main method
int main()
{
// Declare array
int a[10];
// Declare variable
int *p = a;
// Declare variable
int i;
//Loop executes until the value of i exceeds 10
for (i = 0; i < 10; i++)
//Assign value i to a[i]
a[i] = i;
//Loop executes until the value of i exceeds 10
for (i = 0; i < 10; i++)
//Display value
cout << p[i] << " ";
cout << endl;
//Pause console window
system("pause");
//Return 0 value
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Problem Solving With C++ (Looseleaf) - With Access
- Consider the following function: int secret(int m, int n){int temp = n;for (int i = 1; i < abs(m); i++)temp = temp + n;if (m < 0)temp = -temp;return temp;} a. What is the output of the following C++ statements? i. cout << secret(18, 4) << endl; ii. cout << secret(-10, 20) << endl;b. What does the function secret do?arrow_forwardPLEASE ANSWER THE QUESTION IN C! 1) Instead of reading several lines of text, just hard-code the following text in the programa. myString= “The quick Brown ? Fox ? jumps over the Lazy Dog and the !##! LAZY DOG is still sleeping”.b. The program should work with any value assigned to myString.c. Use a very small set of text (like “Ab#C#d” ) to test/debug your program first. Use lots of tracing statements to help you to trace the value of array index and elements.2) The program should use of your own function that converts the text to lower case (do not use the built-in tolower function ) and then perform the analysis in the main function. Ignore any numbers, symbols or special characters, etc in the text if any.Note : It’s easier to create a new string for the result instead of modifying the original string.3) Instead of the three methods outlined in the original question, complete only part a and part b. Assume maximum word length is 10 for part b.4) Turn in one program that contains…arrow_forwardAnswer the given question with a proper explanation and step-by-step solution. In Java, please. Consider the following problem. Input: an array of integers A[] Output: rearranges the array to have the following property: Suppose the first element in the original array A[], A[0] = x. In the rearranged array, suppose that x is at index i, that is A[i] = x. Then, we want the rearranged array to be such that A[j] <= x for all j < i and A[j] > x for all j > i. The rearranging should place all the values less than (or equal to) x to the "left" of x and all values larger than x to the right of x. Here's an example. Suppose the array has the elements in this initial order: 4 3 9 2 7 6 5 A[0] = 4. After rearranging elements, we get: 3 2 4 5 9 7 6 That is, A[0] = 4, is positioned in the resulting array so that all elements less than 4 (that is, 2, 3) are to its left (in no particular order), and all elements larger than 4 are to its right (again, in no particular order).arrow_forward
- 7. Write a program that will create a dynamically created 1D array of length n (given by the user) and store first n odd numbers in it. Try printing the size of the array using size of() function. Are you satisfied with the result of size of() function? If no, explain why? need the solve in c++arrow_forward09. Python Write the in_order() function, which has a list of integers as a parameter, and returns True if the integers are sorted (in order from low to high) or False otherwise. The program outputs "In order" if the list is sorted, or "Not in order" if the list is not sorted. Ex: If the list passed to the in_order() function is [5, 6, 7, 8, 3], then the function returns False and the program outputs: Not in order Ex: If the list passed to the in_order() function is [5, 6, 7, 8, 10], then the function returns True and the program outputs: In order Note: Use a for loop. DO NOT use sorted() or sort()..arrow_forwardCreate a program that accepts an integer N, and pass it to the function generatePattern. generatePattern() function which has the following description: Return type - void Parameter - integer n This function prints a right triangular pattern of letter 'T' based on the value of n. The top of the triangle starts with 1 and increments by one down on the next line until the integer n. For each row of in printing the right triangle, print "T" for n times. In the main function, call the generatePattern() function. Input 1. One line containing an integer Output Enter N: 4 T TT TTT TTTTarrow_forward
- John Here Need correct java code for the following questionarrow_forward1.The following is the C code that you need to implement for this lab: uint8_ t f(uint8_tn) return(n<2)?(n):(f(n-1)+f(n-2)); The main function can be assumed as follows: int main() uint8_ t x; x=f(???); return 0; Obviously, " ? ? ? " is representing a value used to test the algorithm! Is "f"recursive? a.No answer text provided. b.Yes c.no d.depends on whether it is in for main 2.First, make the code in the previous question an actual C program so that it can be compiled and it runs. Play with it so that you feel comfortable with the logic of the code. Then implement the code in TTPASM. Note that you need to preserve the actual C code structure, this means you cannot it into a non-recursive subroutine. Furthermoreall conventions discussed in class regarding subroutines must be followed. The idea is that I should be able to substitute f with my own code, and main should work. Or, I can substitute main with my own, and f should work. Attach the source code of your assembly…arrow_forward1. Implement function postal() that takes 6 inputs: a first name (as a string) a last name (as a string) an address (as a string) a city (as a string) a state (as a string) a zip code (as an integer)and then prints a mailing address in 3 lines as shown below.>>> postal('Ljubomir', 'Perkovic', '243 S Wabash Ave','Chicago', 'IL', 60304)Ljubomir Perkovic243 S Wabash AveChicago, IL 60304arrow_forward
- 12. Consider the recursive function int gcd( int a, int b) int gcd( int a, int b){ if (b > a) return gcd(b,a); if ( b == 0 ) return a; return gcd( b, a% b); } How many invocation (calls) of the gcd() function will be made by the call gcd(72, 30)?arrow_forwardConsider the following function: int secret(int m, int n) { int temp = n; for (int i = 1; i < abs(m); i++) temp = temp + n; if (m < 0) temp = -temp; return temp; } What is the output of the following C++ statements? i.cout << secret(5, 4) << endl; ii.cout << secret(-3, 20) << endl;arrow_forwardDescribe what problem occurs in the following code. What modifications should be made to it to eliminate the problem? int[] sums = {6, 12, 3, 32, 12, 10, 9, 6}; for (int index = 1; index <= sums.length; index += 1) { System.out.println(sums[index]); }arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
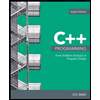