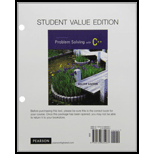
Pointer in C++:
A pointer is a variable whose value will be another variable’s address. Generally, a pointer variable is declared as follows:
type *var-name;
Here, type is the pointer’s base type and var-name is the pointer variable name. The asterisk is used to designate a variable as a pointer.
Given Program:
//Include libraries
#include <iostream>
//Use namespace
using namespace std;
//Define main method
int main()
{
// Declare pointer variables
int *p1, *p2;
//Assign value
p1 = new int;
//Assign value
p2 = new int;
//Assign value
*p1 = 10;
//Assign value
*p2 = 20;
//Display value
cout << *p1 << " " << *p2 << endl;
//Assign value
*p1 = *p2;
//Display value
cout << *p1 << " " << *p2 << endl;
//Assign value
*p1 = 30;
//Display value
cout << *p1 << " " << *p2 << endl;
//Pause console window
system("pause");
//Return 0 value
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Problem Solving With C++ (Looseleaf) - With Access
- In Java: Develop a void function that takes two integers and prints whether the first number is divisible by the second. For example, with arguments 51 and 17, the function should print “51 is divisible by 17”. With arguments 17 and 8, the function should print “17 is not divisible by 8”. Call the function twice with different arguments to show each possible result. What happens when the second argument is greater than the first?arrow_forwardwhat will be the output? #include int main () {int i = 3; i++; print ("%d", i); i--; print ("%d", i); return 0;} a. 34, b. 33, c. 44, d. 43arrow_forwardWhat is the the output of the following code? int **p;p = new int* [5];for (int i = 0; i < 5; i++)p[i] = new int[3];for (int i = 1; i < 5; i++) for (int j = 0; j < 3; j++)p[i][j] = 2 * i + j;for (int i = 1; i < 5; i++){for (int j = 0; j < 3; j++)cout << p[i][j] << " ";cout << endl;}arrow_forward
- Consider the definition of the class integerManipulation . What is the output of the following C++ code? integerManipulation number(3510862895423079232);number.classifyDigits();cout << number.getNum() << ": The number of digits---- "<< endl;cout << " Even: " << number.getEvensCount() << endl<< " Zeros: " << number.getZerosCount() << endl<< " Odd: " << number.getOddsCount() << endl;arrow_forwardint const MULTIPLIER = 5; is a valid way to declare a constant integer variable. Group of answer choices True False ------- Given the following code segment, what is output to the screen? int num1 = 6; int num2 = 4 * num1++; cout << "num1=" << num1 << " num2=" << num2; Group of answer choices num1=7 num2=24 num1=6 num2=24 num1=6 num2=28 Nothing, because the code does not compile.arrow_forwardWhat is the output of the following code? int *intArrayPtr;int *temp;intArrayPtr = new int[5];*intArrayPtr = 7;temp = intArrayPtr;for (int i = 1; i < 5; i++){intArrayPtr++;*intArrayPtr = *(intArrayPtr - 1) 1 2 * i;}intArrayPtr = temp;for (int i = 0; i < 5; i++){cout << *intArrayPtr << " ";intArrayPtr++;}cout << endl;arrow_forward
- #include <iostream> using namespace std; void additionProblem(int topNumber, int bottomNumber) { int userAnswer; cout << "\n\n\n " << topNumber << " + " << bottomNumber << " = "; cin >> userAnswer; cin.ignore(1000, 10); int theAnswer = topNumber + bottomNumber; if (theAnswer == userAnswer) cout << " Correct!" << endl; else cout << " Very good, but a better answer is " << theAnswer << endl; } // additionProblem int main() { additionProblem(8, 2); additionProblem(4, 8); additionProblem(3, 7); additionProblem(4, 10); additionProblem(11, 2); } // main The above code needs to be modified according to the image I've attatched.arrow_forwardGive solution in C ++ Language with secreenshoot of source code. Part 01In this task, you need to do the following:• Write a function named displayMessage() that takes user name as input in character array and then shows greetings• Now take the name input in main() and pass the name as an argument to displayMessage() function• Change the displayMessage() method such that it returns the number of characters after displaying the greetings part 02Write a function power that takes two parameters a and b. And it returns the power as ab.arrow_forwardDetermine the output for the following code. Box in your final output result. public class Beta extends Baap { public int h = 44; public int getH( ) { System.out.println("Beta " + h); return h; } public static void main(String[ ] args) { Baap b = new Beta(); System.out.println(b.h + " " + b.getH( )); Beta bb = (Beta) b; System.out.println(bb.h + " " + bb.getH( )); } } public class Baap { public int h = 4; public int getH( ) { System.out.println("Baap " + h); return h; } }arrow_forward
- int j; for (int i = 0; i < 5; i++) {j = 2*j*i;} What is j?arrow_forwardConsider the following code. What will be printed out by the code?arrow_forwardReplace (????) with relevant code for the program to function. Details about the programs are provided below. (Jav has been used). Show the output. a)This program prints four lines: kimarr() 4 [9, 0, 0, 14] 2 [9, 0, 0, 14] kimarr() 8 [9, 9, 14, 14] 4 [9, 9, 14, 14] */ package finalexamtakehome2; import java.util.*; // for Arrays class /** * * @author sweetkim */ public class Finalexamtakehome2 { // public static void kimarr(int x, int[] a) { x = x * 2; if (x > 6) { a[2] = 4; a[1] = 9; } else { a[0] = 9; a[3] = 1; } System.out.println("kimarr() " + x + " " + Arrays.toString(????)); } // public static void main(String[] args) { int x = 1; int[] a = new int[4]; x = x * ????; kimarr(x, a); System.out.println(x + " " + Arrays.toString(????)); x = x * 2; kimarr(x, a); System.out.println(x + " " +…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
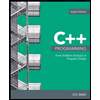