A)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
B)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
C)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.
D)
Address of (&) operator:
The main purpose of address operator “&” operator in pointers is to return the memory address of variables.
When the address of operator “&” is placed before the pointer variable, it will hold address of the pointer variable.
Example Program:
//include necessary header
#include <iostream>
#include <string>
using namespace std;
//main method
int main()
{
//variable declaration
int a;
//display the address of the variable a
cout << &a;
}
Representing array using pointer notation:
- In general, array will have a continuous allocation of memory bytes, to represent the values present in the array it can be represented using its subscript value.
- So, to represent the values using pointer notation with an indirection operator the name of the array plus the subscript representing the value needs to be specified.
- When we represent only the array name, it will indicate the first element of the array.
- To represent the next element we need to increment the values by one to find the proceeding elements of the array.
- When “&” operator is used before an array it will return the address of the corresponding value.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
STARTING OUT C++,+MATLAB+MYPROGRAMLAB>C
- This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int array and the ratings in another int array. Output these arrays (i.e., output the roster). Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Player 1 -- Jersey number: 84, Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 (2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. The program initially outputs the menu, and outputs the menu after a user chooses an option. The…arrow_forwardComplete the following program should first fill in (by asking the user for input) and then list all the elements in an array: #include <_____>using namespace std;___ main(){int array[8]___for(int x=_; x<_; ___)cin>>______;for(____; x<____; ___)cout<<_____;return __;____arrow_forward(Electrical eng.) Write a program that declares three one-dimensional arrays named volts, current, and resistance. Each array should be declared in main() and be capable of holding 10 double-precision numbers. The numbers to store in current are 10.62, 14.89, 13.21, 16.55, 18.62, 9.47, 6.58, 18.32, 12.15, and 3.98. The numbers to store in resistance are 4, 8.5, 6, 7.35, 9, 15.3, 3, 5.4, 2.9, and 4.8. Your program should pass these three arrays to a function named calc_volts(), which should calculate elements in the volts array as the product of the corresponding elements in the current and resistance arrays (for example ,volts[1]=current[1]resistance[1]). After calc_volts() has passed values to the volts array, the values in the array should be displayed from inside main().arrow_forward
- (Electrical eng.) Write a program that stores the following resistance values in an array named resistance: 16, 27, 39, 56, and 81. Your program should also create two arrays named current and power, each capable of storing five double-precision numbers. Using a for loop nd a cin statement, have your program accept five user-input numbers in the current array when the program is run. Your program should store the product of the values of the squares of the current array and the resistance array in the power array. For example, use power[1]=resistance[1]pow(current[1],2). Your program should then display the following output (fill in the chart):arrow_forward(Electrical eng.) Write a program that specifies three one-dimensional arrays named current, resistance, and volts. Each array should be capable of holding 10 elements. Using a for loop, input values for the current and resistance arrays. The entries in the volts array should be the product of the corresponding values in the current and resistance arrays (sovolts[i]=current[i]resistance[i]). After all the data has been entered, display the following output, with the appropriate value under each column heading: CurrentResistance Voltsarrow_forwardLargest/Smallest Array Values Write a program that lets the user enter 10 values into an array. The program should then display the largest and smallest values stored in the array. Do question 7(above) again this time using 5 functions. void getValues(int [], int); void displayValues(const int[], int); int largest(const int[],int); int smallest(const int[],int); void displayLargestSmallest(int,int);arrow_forward
- Write the following function that tests whether the array has four consecutive numbers with the same value. bool isConsecutiveFour(const int values[], int size) Write a test program that prompts the user to enter a series of integers and displays if the series contains four consecutive numbers with the same value. Your program should first prompt the user to enter the input size—i.e., the number of values in the series. Assume the maximum number of values is 80. Here are sample runs: Enter the number of values: 8 Enter the values: 3 4 5 5 5 5 4 5 The list has consecutive fours Enter the number of values: 9 Enter the values: 3 4 5 5 6 5 5 4 5 The list has no consecutive foursarrow_forwardIn a program you need to store the identification numbers of 10 employees (as i nt s)and their weekly gross pay (as doubl es).A) Define two arrays that may be used in paralle l to store the 10 emp loyeeidentification numbers and 10 weekly gross pay amounts .B) Write a loop that uses these arrays to print each employee's identificationnumber and weekly gross pay.arrow_forward(c++)1. Body Mass Index (BMI) is a measure of health on weight. It can be calculated by taking your weight in kilograms and dividing by the square of your height in meters. Write a program that lets a teacher to calculate the BMI of 20 students. The program should use three one dimensional arrays named studWeight that holds the weight (in kilograms), studHeight that holds the height (in meters) and studBMI that holds the calculated BMI for each student. The program contains the following functions: a) readData: This function receives the studWeight and studHeight arrays from the main function. It reads the weight and height for all the students and stored them in the studWeight and studHeight arrays, respectively. b) calBMI: This function receives one element of the studWeight and studHeight arrays. It calculates the BMI of each student based on her/his weight and height, and then store the BMI in the studBMI array. c) display: This function displays the BMI of the students.arrow_forward
- The strLetters array is declared using the Dim strLetters() As String = {"E", "A","C", "G"} statement. Which of the following will sort the array as follows: G, E, C, A?a. Array.Sort(strLetters) Array.Reverse(strLetters)b. Array.Reverse(strLetters) Array.Sort(strLetters)c. Array.SortDescending(strLetters)d. both a and barrow_forwardWrite a statement that assigns the value 50 to the very last element in the values array int[,] values = new decimal[200, 100];arrow_forward// Reverse.cpp - This program reverses numbers stored in an array.// Input: None// Output: Original contents of array and the reversed contents of the array. #include <iostream>#include <string>using namespace std;void reverseArray(int[]);int main() { int numbers[] = {100, 90, 80, 70, 60}; int x; // Print contents of array // Call reverseArray() function here // Print contents of reversed array return 0;} // End of main() function// Write reverseArray function here.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
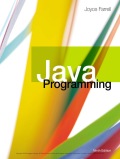
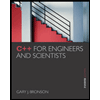
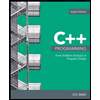
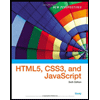
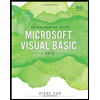