What are name constants and literal values?
A constant is a data item whose value cannot be modified throughout the program execution. When a constant is related to an identifier such as a variable, array, structure, or function, it is called a named constant. In most programming languages, the 'const' keyword is used to declare constants.
The value of a named constant should be assigned while defining the constant. Generally, programmers use uppercase to define named constants. The syntax for defining constants in most programming languages is similar.
Syntax: const type variable= value;
where const is the keyword used to define a constant and type is the data type.
For instance, to define a named constant PI in C language, the following statement will work:
const double PI = 3.14;
Here, the const keyword indicates a constant, double is the data type, PI is the variable, and a constant value (3.14) is assigned.
A literal is a notation used to represent a fixed value (constants) in a program. In other words, literals are the values assigned to constants. Literals can be used in a program directly. For example, they can be written as:
1
3.1
'C'
Ideally, literals are used for variable initialization. For instance, to assign an integer value 5 to a variable, the following statement can be used:
int d = 5;
Variable
A variable is a name assigned to a memory location in computer programming languages. Variables are used to store data, and their values can be altered throughout the program execution.
Variables should be assigned a unique name. They can have uppercase and lowercase alphabets, digits, and underscore. However, the first letter should only be a letter or underscore. Programmers typically use long variable names so that their purpose can be understood by reading the variable name. Below is an example of declaring a variable in C language:
int sum = 14;
Here, int is a data type, the sum is the variable name, and the value 14 is assigned to it.
Types of literals
There are four types of literals.
- Integer literals
- Floating-point literals
- Character literals
- String literals
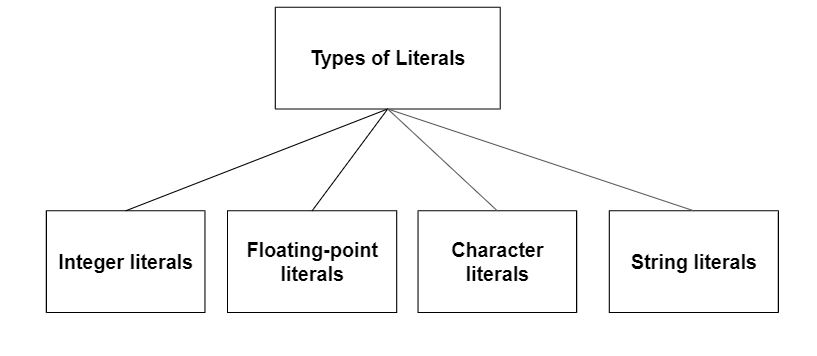
Integer literals
Integer literals are used to define and store integer values. There are mainly three types of integer literals:
Decimal numbers (base 10)
It is a number indicating digits from 0 to 9. Decimals cannot start with 0 or contain a decimal point, commas, or spaces within the number. However, they can be preceded by a minus symbol (-) to indicate negative values.
For example, the values: 56, -1233, 788, 343, and 930 are decimal numbers.
Octal numbers (base 8)
Octal numbers begin with 0, and the rest of the digits can range from 0-7. These numbers cannot contain decimal points, commas, or spaces but can begin with a minus symbol (-).
Examples of Octal numbers include - 0255, 04563, 0245
Hexadecimal numbers (base 16)
Hexadecimal numbers begin with 0x or 0X, followed by digits 0-9 or alphabetic characters a-f or A-F. These numbers cannot contain decimal points, commas, spaces. However, they can begin with the minus sign.
Examples of Hexadecimal integers include:
0x23, 0xAD43, 0Xc123
Floating-point literals
Floating-point literals are used to store real numbers, that is, numbers having an integer part, real part, fractional part, and exponential part. Floating-point literals can be stored in the following two forms:
Decimal form
Float literals represented in decimal form should have a decimal point, exponent part, or both. If the number does not have either of these, the compiler will give an error. The decimal form can be preceded by a minus (-) symbol.
Examples of decimal form float literals include: 1.2, 9.0, 0.1, -4.5, -0.23
Exponential form
Float literals represented in exponential form should include an integer part, fractional part, or both. If either of them is missing, the compiler will produce an error. This form is used to represent a number having a large magnitude. For instance, the number 1260000000000 can be represented as 1.26e12 using the exponential form.
The exponential form has two parts: a mantissa and an exponent part. Mantissa consists of the main digits in a number, and the exponent defines where to place the decimal point. The syntax of exponential form is as follows:
[+/-] <Mantissa> <e/E> [+/-] <Exponent>
Examples of exponential form float literals include:
+5e36, -2e45, +6e-35
Character literals
In computer programming, character literals are used to represent a single character within single quotes. If more than one character is assigned to character literals, the compiler will give a warning stating a multi-character character constant. For example, 'hi', is an invalid character literal.
Character literals should always be enclosed by single quotes. Character literals may contain a single printable character and any non-printable characters. Non-printable characters are represented using escape sequences like ' ' for NULL.
Escape sequences are characters in C used to indicate a special meaning. They are prefixed by a backslash (). For example, (n) indicates newline, b indicates backspace, and t indicates tab.
Examples of character literals include: 'd', 'T', 'n', ‘4’, ‘@’
String literals
A string literal represents multiple characters enclosed in double quotation marks. String literals are always terminated by a null character ' ', which is automatically inserted by the compiler. They can include printable or non-printable characters. To insert double quotes within a string, the escape sequence (") should be added before the quotes.
Furthermore, the plus sign (+) can be used to concatenate two strings.
Examples of string literals include:
"What", "Hey!n", "PREPROCESSOR", "2500", "operand@boolappend.com", "Enumeration constants, "Numeric Values!"
Boolean literals
Apart from the literals mentioned above, some programming languages support one more literal called the boolean literal. The boolean literal represents the boolean data types - true and false. True indicates the statement is true, while false indicates the statement is false. In most cases, true is indicated by the value 1, and false is indicated by using 0.
Context and Applications
The topic name constants and literal value is an important topic covered in various programming languages. Students studying in the following graduate and postgraduate courses study this concept.
- Bachelors in Computer Science
- Bachelors in Information Technology
- Masters in Computer Science
- Masters in Information Technology
Practice Problems
Q1. What is a literal?
- A value assigned to a constant
- Symbolic constants
- Similar to a string
- A data type
Answer: Option a
Explanation: A literal is a value assigned to a constant.
Q2. Character literals are enclosed within-
- Single brackets
- Single quotes
- Double quotes
- Numeric identifier variable
Answer: Option b
Explanation: Character literals are always enclosed in single quotes.
Q3. Which of these is a type of literal?
- User-defined identifier
- String
- Keyword
- Constant
Answer: Option b
Explanation: Integer, Floating-point, Character, and String literals are types of literals.
Q4. Which keyword is used to represent a constant?
- Constant
- struct
- int
- const
Answer: Option d
Explanation: The const keyword is used to indicate a constant.
Q5. Which literal is used to store integer values?
- Character
- String
- Integer
- Decimal
Answer: Option c
Explanation: The integer literal is used to store integer values.
Common Mistakes
Students should be careful while using literals in any programming language. All the literals have a specific use and should be defined according to their rules. If the student makes a mistake in defining a literal, it will generate a compilation error.
Related Concepts
- Preprocessor directives
- Functions
- Control statements
- Arrays
- Structure
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Name Constants and Literal Values Homework Questions from Fellow Students
Browse our recently answered Name Constants and Literal Values homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.