prover
.py
keyboard_arrow_up
School
George Mason University *
*We aren’t endorsed by this school
Course
262
Subject
Computer Science
Date
Feb 20, 2024
Type
py
Pages
2
Uploaded by ConstableFlamingo152
#!python3
import sys
import hashlib
from base64 import b64encode, b64decode
import math
# HINT: why is hash_interna_node included?
from merkle_utils import MerkleProof, hash_internal_node, hash_leaf
merkle_proof_file = "merkle_proof.txt" # File where merkle proof is written.
MAXHEIGHT = 20 # Max height of Merkle tree
NUM_LEAVES = 1000 # Number of leaves in Merkle Tree
def write_merkle_proof(filename, merkle_proof: MerkleProof):
"""Helper function that outputs the merkle proof to a file in a format for it to be read easily by the verifier."""
fp = open(filename, "w")
print("leaf position: {pos:d}".format(pos=merkle_proof.pos), file=fp)
print("leaf value: \"{leaf:s}\"".format(leaf=merkle_proof.leaf.decode('utf-
8')), file=fp)
print("Hash values in proof:", file=fp)
for i in range(len(merkle_proof.hashes)):
print(" {:s}".format(b64encode(merkle_proof.hashes[i]).decode('utf-8')), file=fp)
fp.close()
def gen_leaves_for_merkle_tree():
"""Generates 1000 leaves for the merkle tree"""
# The leaves never change and so they always produce the same # Merkle root (ROOT in verifier.py)
leaves = [b"data item " + str(i).encode() for i in range(NUM_LEAVES)]
print('\nI generated #{} leaves for a Merkle tree.'.format(NUM_LEAVES))
return leaves
def gen_merkle_proof(leaves, pos):
"""Takes as input a list of leaves and a leaf position.
Returns the a the list of hashes that prove the leaf is in the tree at position pos."""
height = math.ceil(math.log(len(leaves),2))
assert height < MAXHEIGHT, "Too many leaves."
# hash all the leaves
state = list(map(hash_leaf, leaves)) # Pad the list of hashed leaves to a power of two
padlen = (2**height)-len(leaves)
state += [b"\x00"] * padlen
# initialize a list that will contain the hashes in the proof
hashes = []
level_pos = pos # local copy of pos
for level in range(height):
new_state = []
####### YOUR CODE GOES HERE ######
####### to hash internal nodes in the tree use the ######
####### function hash_internal_node(left,right) ######
# Returns list of hashes that make up the Merkle Proof
return hashes
### Main program
if __name__ == "__main__":
# Read leaf number from command line
# We generate a Merkle proof for this leaf
pos = 743 # default leaf number
if len(sys.argv) > 1:
pos = int(sys.argv[1])
assert 0 <= pos < NUM_LEAVES, "Invalid leaf number"
leaves = gen_leaves_for_merkle_tree()
# Generate the merkle proof
hashes = gen_merkle_proof(leaves, pos)
merkle_proof = MerkleProof(leaves[pos], pos, hashes)
# Write merkle proof to a file for the verifier to access
write_merkle_proof(merkle_proof_file, merkle_proof)
print('I generated a Merkle proof for leaf #{} in file {}\
n'.format(pos,merkle_proof_file))
sys.exit(0)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Its a java data structures class. Lab dire is also given on second page. Thank you
arrow_forward
class Solution:
def searchRange(self, nums, target):
def binary_search(nums, target, left, right):
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
return mid
elif nums[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
def find_leftmost(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] >= target:
right = mid - 1
else:
left = mid + 1
return left
def find_rightmost(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] <= target:
left = mid + 1…
arrow_forward
JavaScript Practice Question
arrow_forward
code used:
python
class Node: def __init__(self, value): self.data_val = value self.next_node = None
def insert_after(self, node): tmp_node = self.next_node self.next_node = node node.next_node = tmp_node
def get_next(self): return self.next_node
def print_data(self): print(self.data_val, end=", ")
# TODO: Write recursive print_list() function here.
if __name__ == "__main__": size = int(input()) value = int(input()) head_node = Node(value) # Make head node as the first node last_node = head_node # Insert the second and the rest of the nodes for n in range(1, size): value = int(input()) new_node = Node(value) last_node.insert_after(new_node) last_node = new_node print_list(head_node)
arrow_forward
bool add(int anInt)// Pre: contains(anInt) ? size() <= MAX_SIZE : size() < MAX_SIZE// Post: If contains(anInt) returns false, anInt has been// added to the invoking IntSet as a new element and// true is returned, otherwise the invoking IntSet is// unchanged and false is returned.// bool remove(int anInt)// Pre: (none)// Post: If contains(anInt) returns true, anInt has been// removed from the invoking IntSet and true is// returned, otherwise the invoking IntSet is unchanged// and false is returned.
bool add(int anInt); bool remove(int anInt);
bool IntSet::add(int anInt){ if(used <= data[MAX_SIZE]) // check if the array is not full { return contains(used); //if not full then return to data } return false;}
bool IntSet::remove(int anInt){ cout << "remove() is not implemented yet..." << endl; return false; // dummy value returned}
arrow_forward
Write searchR(self, root, data) that will search the data in a BST using recursion.
python language
please avoid chatgpt please humble request
arrow_forward
javascript only: debugg the following code to pass specs.
arrow_forward
In java
Create a hash table using an array with elements 324,221,563,679,234,569,890,5678,654
then perform the following operations.
1) Insert 227
2) Delete 679 and Insert 9
3) Insert 67
4) Display the hash table content
Note: This hash table uses technique linear probing when encounters a collision.
arrow_forward
Generics enable you to specify the exact type that will be stored in a collection and give you the benefits of compile-time type checking—the compiler issues error messages if you use inappropriate types in your collections. Once you specify the type stored in a generic collection, any reference you retrieve from the collection will have that type.
Instructions
Write a program that inserts 25 random integers from 0 to 100 in order into Java’s LinkedList object (you must use Java’s LinkedList class to get any credit for this assignment).
The program must:
sort the elements,
then calculate the sum of the elements, and
calculate the floating-point average of the elements.
For this assignments make sure that your screen shot(s) show your program running and that your runtime display shows your random list, sorted list, sum of the elements, and average of the elements. You only get credit for what you demonstrate. Below is a sample screen shot. Your output doesn’t have to look…
arrow_forward
Don't copy from anywhere... please fast... typed answer
Assignment: Linked List of Students
You have been tasked with implementing a program in Java that uses a linked list to store and manage a list of students in a class. Each student should have a name and a grade.
Your program should include the following classes:
Student: Represents a student in the class. Each student should have a name and a grade.
Node: Represents a node in the linked list. Each node should store a reference to a student and a reference to the next node in the list.
LinkedList: Represents the linked list itself. Each linked list should have a reference to the first node in the list.
Your task is to implement these classes using a linked list and demonstrate their functionality by creating a console-based interface for users to interact with the system.
Your program should allow users to:
Add a new student to the class at the end of the list.
View information about a student, including their name and grade.…
arrow_forward
Buffer overlow :computer security
In a C program, we print the address of relevant variables and arrays and get the following:
0xbfffe7b8 i
0xbfffe7bc length
0xbfffe7c0 hash_ptr
0xbfffe7ce targetuid
0xbfffe7d5 userid
0xbfffe817 pw
0xbfffe858 t
0xbfffe899 hashhex
0xbfffe8da target
0xbfffe91b hash
0xbfffe95c buffer
The program executes the following instruction:
strcpy(pw, buffer);
You want to exploit the buffer overflow vulnerability by putting in buffer a string aaaaaaaaaaaaa ... 0c0beacef8877bbf2416eb00f2b5dc96354e26dd1df5517320459b1236860f8c
with the goal of putting the hash 0c0beacef8877bbf2416eb00f2b5dc96354e26dd1df5517320459b1236860f8c variable target.
If you count offset as 0 for the first a, 1 for the second a, and so on, what should be the offset of 0, the first character of the hash? In other words, how many a's should you put before the first zero character?
(You can give the answer in decimal or in hexadecimal, but please specify.)
arrow_forward
Python
Why am I getting an error?
class HashTableProb: def __init__(self, size=10): # Initialize the hashtable with the given size and an empty array to hold the key-value pairs. self.__size = size # size of the hashtable self.__hashtable = [None for _ in range(size)] self.__itemcount = 0 # Keeps track of the number of items in the current hashtable
def __contains__(self, key): return self.__searchkey(key)
def __next_prime(self, x): def is_prime(x): return all(x % i for i in range(2, x))
return min([a for a in range(2*x+1, x*x) if is_prime(a)])
def __hash(self, key): # Hash function to be used for mapping a key to the indices in the hashtable. bucket = key % self.__size return bucket
def __resize(self): # Resizes the hashtable to the next prime number after current table size. Also, rehashes all the current items. n = self.__next_prime(self.__size*2) new = [None…
arrow_forward
In python please!
arrow_forward
Code to start with:
public void addStudents(String[] ids){ }
arrow_forward
import hashlib
def hash_function(password, salt, al): if al == 'md5': #md5 hash = hashlib.md5(salt.encode() + password.encode()) hash.update(password.encode('utf-8')) return hash.hexdigest() + ':' + salt elif (al == 'sha1'): #sha1 hash = hashlib.sha1() hash.update(password.encode('utf-8')) return hash.hexdigest() + ':' + salt elif al == 'sha224': #sha224 hash = hashlib.sha224() hash.update(password.encode('utf-8')) return hash.hexdigest() + ':' + salt elif al == 'sha256': #sha256 hash = hashlib.sha256() hash.update(password.encode('utf-8')) return hash.hexdigest() + ':' + salt elif al == 'blake2b': #blake2b512 hash = hashlib.blake2b() hash.update(password.encode('utf-8')) return hash.hexdigest() + ':' + salt else: print("Error: No Algorithm!")
if __name__ == "__main__": # TODO: create a list called hash_list that contains # the five hashing algorithsm as strings # md5, sha1, sha224, sha256, blake2b hash_list =…
arrow_forward
In java, the Arrays class contains a static binarySearch() method that returns …… if element is found.
false
true
(-k–1) where k is the position before which the element should be inserted
The index of the element
arrow_forward
import numpy as np%matplotlib inlinefrom matplotlib import pyplot as pltimport mathfrom math import exp
np.random.seed(9999)def is_good_peak(mu, min_dist=0.8): if mu is None: return False smu = np.sort(mu) if smu[0] < 0.5: return False if smu[-1] > 2.5: return False for p, n in zip(smu, smu[1:]): #print(abs(p-n)) if abs(p-n) < min_dist: return False return True
maxx = 3ndata = 500nset = 10l = []answers = []for iset in range(1, nset): npeak = np.random.randint(2,4) xs = np.linspace(0,maxx,ndata) ys = np.zeros(ndata) mu = None while not is_good_peak(mu): mu = np.random.random(npeak)*maxx for ipeak in range(npeak): m = mu[ipeak] sigma = np.random.random()*0.3 + 0.2 height = np.random.random()*0.5 + 1 ys += height*np.exp(-(xs-m)**2/sigma**2) ys += np.random.randn(ndata)*0.07 l.append(ys) answers.append(mu)
p6_ys = lp6_xs =…
arrow_forward
Make sure the program run smooth and perfectly, make sure no error encounter. compatible for python software.
Complete the sample code template to implement a student list as a binary search tree (BST).Specification1. Each Studentrecord should have the following fields: =studentNumber =lastname =firstName =course =gpa2. Run the program from an appropriate menu allowing for the following operations:* Adding a Student record
* Deleting a Student record
* Listing students by: • All students • A given course • GPA above a certain value • GPA below a certain value
class Record:def __init__(self, studentNumber = None, lastname = None, firstName = None, course = None, gpa =None,):self.studentNumber = studentNumberself.lastname = lastnameself.firstName = firstNameself.course = courseself.gpa = gpadef __str__(self):record = "\n\nStudent Number : {}\n".format(self.studentNumber)record += "Lastname : {}\n".format(self.lastname)record += "FirstName : {}\n".format(self.firstName)record…
arrow_forward
API documentation link ------> https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/ArrayList.html
PREVIOUS CODE -
import java.util.ArrayList;public class BasicArrayList { // main method
public static void main(String[] args) {
// create an arraylist of type Integer
ArrayList<Integer> basic = new ArrayList<Integer>();
// using loop add elements
for(int i=0;i<4;i++){
basic.add(i+1);
}
// using loop set elements
for(int i=0;i<basic.size();i++){
basic.set(i,(basic.get(i)*5));
}
// using loop print elements
for(int i=0;i<basic.size();i++){
System.out.println(basic.get(i));
}
}}
Q1)
a)Consider how to remove elements from the ArrayList. Give two different lines of code that would both remove 5 from the ArrayList.
b)What do you think the contents of the ArrayList will look like after the 5 has been removed? (Sketch or list)
c)Now update the BasicArrayList program to remove the 5 (index 1) from the ArrayList. Add this step before the last loop that…
arrow_forward
main.py
Load default template.
1 def all_permutations (permList, namelist):
2
# TODO: Implement method to create and output all permutations of the List of names.
3
4 if
name
== "
main ":
namelist = input ().split('')
permlist = []
all_permutations(permList, namelist)
5
6
7
arrow_forward
Fix an error and show it please?
arrow_forward
Data Structures/Algorithms in Java
arrow_forward
Create an interface called Stack with methods Push (), Pop () and property Length.Create a class that implements this interface. Use an array to implement stack datastructure.
language C#
arrow_forward
Java Objects and Linked Data: Select all of the following statements that are true.
The Java Class Library's class ArrayList implements the LinkedList interface.
In Java, when one reference variable is assigned to another reference variable,
both references then refer to the same object.
In Java, the "equals"-method that any object inherits can be overwritten to
compare objects field by field.
arrow_forward
INSTRUCTION: make this a flowchart
import java.util.HashSet;import java.util.Scanner;import java.util.Set;
public class elements { public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Creating two sets Set<Integer> set1 = new HashSet<Integer>();
Set<Integer> set2 = new HashSet<Integer>();
System.out.println("Enter the element of set A [max-5 elements]: "); for (int i = 0; i < 5; i++) { int num = sc.nextInt(); set1.add(num); } System.out.println("Enter the element of set B [max-5 elements]: "); for (int i = 0; i < 5; i++) { int num1 = sc.nextInt(); set2.add(num1); } // creating a menu of opeartions System.out.println("a. Identify elements of set"); System.out.println("b. Check Union"); System.out.println("c. Check Difference"); System.out.println("d. Check Intersection"); System.out.println("e. Check Subset"); System.out.println("f. If set A and B are…
arrow_forward
// MergeSortTest.java// Sorting an array with merge sort.import java.security.SecureRandom;import java.util.Arrays;
public class MergeSortTest { // calls recursive sortArray method to begin merge sorting public static void mergeSort(int[] data) { sortArray(data, 0, data.length - 1); // sort entire array }
// splits array, sorts subarrays and merges subarrays into sorted array private static void sortArray(int[] data, int low, int high) { // test base case; size of array equals 1 if ((high - low) >= 1) { // if not base case int middle1 = (low + high) / 2; // calculate middle of array int middle2 = middle1 + 1; // calculate next element over
// split array in half; sort each half (recursive calls) sortArray(data, low, middle1); // first half of array sortArray(data, middle2, high); // second half of array
// merge two sorted arrays after split calls…
arrow_forward
import java.util.HashSet;
import java.util.Set;
// Define a class named LinearSearchSet
public class LinearSearchSet {
// Define a method named linearSearch that takes in a Set and an integer target
// as parameters
public static boolean linearSearch(Set<Integer> set, int target) {
// Iterate over all elements in the Set
for () {
// Check if the current value is equal to the target
if () {
// If so, return true
}
}
// If the target was not found, return false
}
// Define the main method
public static void main(String[] args) {
// Create a HashSet of integers and populate integer values
Set<Integer> numbers = new HashSet<>();
// Define the target to search for
numbers.add(3);
numbers.add(6);
numbers.add(2);
numbers.add(9);
numbers.add(11);
// Call the linearSearch method with the set…
arrow_forward
The fastest data-processing construction we've seen in the class is
Choose one:
Almost Universal hash
block cipher
stream cipher
cryptogrpahic hash
arrow_forward
Step1: Write Stack Java Interface
Step 2: Create an ArrayStack class that implements the interface methods
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
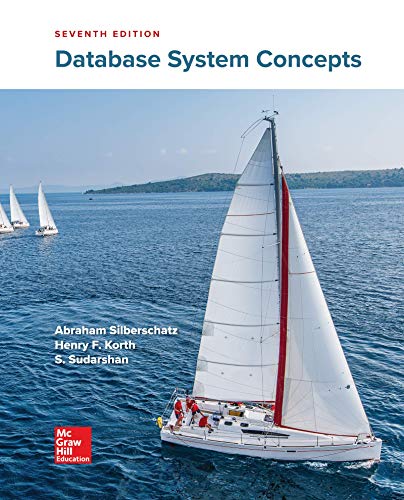
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
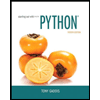
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
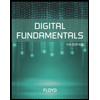
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
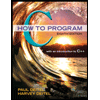
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
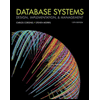
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
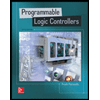
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Its a java data structures class. Lab dire is also given on second page. Thank youarrow_forwardclass Solution: def searchRange(self, nums, target): def binary_search(nums, target, left, right): while left <= right: mid = (left + right) // 2 if nums[mid] == target: return mid elif nums[mid] < target: left = mid + 1 else: right = mid - 1 return -1 def find_leftmost(nums, target): left, right = 0, len(nums) - 1 while left <= right: mid = (left + right) // 2 if nums[mid] >= target: right = mid - 1 else: left = mid + 1 return left def find_rightmost(nums, target): left, right = 0, len(nums) - 1 while left <= right: mid = (left + right) // 2 if nums[mid] <= target: left = mid + 1…arrow_forwardJavaScript Practice Questionarrow_forward
- code used: python class Node: def __init__(self, value): self.data_val = value self.next_node = None def insert_after(self, node): tmp_node = self.next_node self.next_node = node node.next_node = tmp_node def get_next(self): return self.next_node def print_data(self): print(self.data_val, end=", ") # TODO: Write recursive print_list() function here. if __name__ == "__main__": size = int(input()) value = int(input()) head_node = Node(value) # Make head node as the first node last_node = head_node # Insert the second and the rest of the nodes for n in range(1, size): value = int(input()) new_node = Node(value) last_node.insert_after(new_node) last_node = new_node print_list(head_node)arrow_forwardbool add(int anInt)// Pre: contains(anInt) ? size() <= MAX_SIZE : size() < MAX_SIZE// Post: If contains(anInt) returns false, anInt has been// added to the invoking IntSet as a new element and// true is returned, otherwise the invoking IntSet is// unchanged and false is returned.// bool remove(int anInt)// Pre: (none)// Post: If contains(anInt) returns true, anInt has been// removed from the invoking IntSet and true is// returned, otherwise the invoking IntSet is unchanged// and false is returned. bool add(int anInt); bool remove(int anInt); bool IntSet::add(int anInt){ if(used <= data[MAX_SIZE]) // check if the array is not full { return contains(used); //if not full then return to data } return false;} bool IntSet::remove(int anInt){ cout << "remove() is not implemented yet..." << endl; return false; // dummy value returned}arrow_forwardWrite searchR(self, root, data) that will search the data in a BST using recursion. python language please avoid chatgpt please humble requestarrow_forward
- javascript only: debugg the following code to pass specs.arrow_forwardIn java Create a hash table using an array with elements 324,221,563,679,234,569,890,5678,654 then perform the following operations. 1) Insert 227 2) Delete 679 and Insert 9 3) Insert 67 4) Display the hash table content Note: This hash table uses technique linear probing when encounters a collision.arrow_forwardGenerics enable you to specify the exact type that will be stored in a collection and give you the benefits of compile-time type checking—the compiler issues error messages if you use inappropriate types in your collections. Once you specify the type stored in a generic collection, any reference you retrieve from the collection will have that type. Instructions Write a program that inserts 25 random integers from 0 to 100 in order into Java’s LinkedList object (you must use Java’s LinkedList class to get any credit for this assignment). The program must: sort the elements, then calculate the sum of the elements, and calculate the floating-point average of the elements. For this assignments make sure that your screen shot(s) show your program running and that your runtime display shows your random list, sorted list, sum of the elements, and average of the elements. You only get credit for what you demonstrate. Below is a sample screen shot. Your output doesn’t have to look…arrow_forward
- Don't copy from anywhere... please fast... typed answer Assignment: Linked List of Students You have been tasked with implementing a program in Java that uses a linked list to store and manage a list of students in a class. Each student should have a name and a grade. Your program should include the following classes: Student: Represents a student in the class. Each student should have a name and a grade. Node: Represents a node in the linked list. Each node should store a reference to a student and a reference to the next node in the list. LinkedList: Represents the linked list itself. Each linked list should have a reference to the first node in the list. Your task is to implement these classes using a linked list and demonstrate their functionality by creating a console-based interface for users to interact with the system. Your program should allow users to: Add a new student to the class at the end of the list. View information about a student, including their name and grade.…arrow_forwardBuffer overlow :computer security In a C program, we print the address of relevant variables and arrays and get the following: 0xbfffe7b8 i 0xbfffe7bc length 0xbfffe7c0 hash_ptr 0xbfffe7ce targetuid 0xbfffe7d5 userid 0xbfffe817 pw 0xbfffe858 t 0xbfffe899 hashhex 0xbfffe8da target 0xbfffe91b hash 0xbfffe95c buffer The program executes the following instruction: strcpy(pw, buffer); You want to exploit the buffer overflow vulnerability by putting in buffer a string aaaaaaaaaaaaa ... 0c0beacef8877bbf2416eb00f2b5dc96354e26dd1df5517320459b1236860f8c with the goal of putting the hash 0c0beacef8877bbf2416eb00f2b5dc96354e26dd1df5517320459b1236860f8c variable target. If you count offset as 0 for the first a, 1 for the second a, and so on, what should be the offset of 0, the first character of the hash? In other words, how many a's should you put before the first zero character? (You can give the answer in decimal or in hexadecimal, but please specify.)arrow_forwardPython Why am I getting an error? class HashTableProb: def __init__(self, size=10): # Initialize the hashtable with the given size and an empty array to hold the key-value pairs. self.__size = size # size of the hashtable self.__hashtable = [None for _ in range(size)] self.__itemcount = 0 # Keeps track of the number of items in the current hashtable def __contains__(self, key): return self.__searchkey(key) def __next_prime(self, x): def is_prime(x): return all(x % i for i in range(2, x)) return min([a for a in range(2*x+1, x*x) if is_prime(a)]) def __hash(self, key): # Hash function to be used for mapping a key to the indices in the hashtable. bucket = key % self.__size return bucket def __resize(self): # Resizes the hashtable to the next prime number after current table size. Also, rehashes all the current items. n = self.__next_prime(self.__size*2) new = [None…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
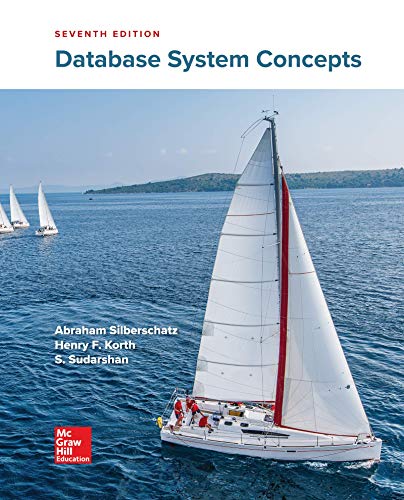
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
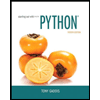
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
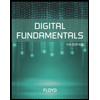
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
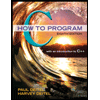
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
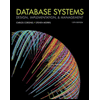
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
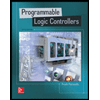
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education