Classes
.pdf
keyboard_arrow_up
School
Toronto Metropolitan University *
*We aren’t endorsed by this school
Course
109
Subject
Computer Science
Date
Dec 6, 2023
Type
Pages
15
Uploaded by ramabarham18
#################
## EXAMPLE: everything
#################
import
import
unittest
unittest
'''
Completing this class rundown should mean that you are pretty well-prepared for the
upcoming exam questions (or at least most of them) on classes.
Generally, concepts you should be familiar with are:
1. Creating an "__init__" method for your class
(Including how to make default variables within your class)
2. Accessing, modifying, and defining variables in the class
3. Creating instance methods within your class
4. How to use helper methods alongside classes
This question will get you to do all of the above, and I'll identify the relevant
sections so you can practice what you need specifically. I'll also be going over
the solution to this during the Review Session on Sunday December 5th at 10 am,
and I'll post the solutions on the Discord too!
'''
'''
Story: The Teletubbies are vanquished, but in the realm of dreams they still haunt
you! Your task is to implement the following classes and helper methods according
to the requirements described in each class/method, so as to conquer your nightmares,
and obliterate all traces of the Teletubbies!
'''
class
class
Dream
Dream
:
'''
Dreams are pretty good. Usually with apple pies and candies and all that good stuff.
Your task is as follows:
Construct the "__init__" method for a Dream class, that takes in 2 arguments (plus self),
self (which you ALWAYS require in your constructors, even if there is nothing else in them!)
a string "title", and
an int "length"
And sets a list dream_elements to start as the empty list: []
[1]
Then, once you have completed your constructor, create an instance method called
change_title(a), which changes the title of your dream instance to the string a.
[2, 3]
Then, create another instance method called add_to_dream(topic), which adds the string
topic to the dream_elements list.
[2, 3]
Finally, create an instance method dreams_to_dust(), which replaces each element of the
dream_elements list with the string "dust".
[2, 3]
'''
def
def
__init__
(
self
, title, length, dream_elements
=
[]):
self
.
title
=
title
self
.
length
=
int
(length)
self
.
dream_elements
=
[]
def
def
change_title
(
self
, a):
self
.
title
=
a
def
def
add_to_dream
(
self
, topic):
# self.topic = topic
self
.
dream_elements
.
append(topic)
def
def
dreams_to_dust
(
self
):
for
for
i
in
in
range
(
len
(
self
.
dream_elements)):
self
.
dream_elements[i]
=
'dust'
class
class
Nightmare
Nightmare
(Dream):
# =============================================================================
# '''
# Nightmares are pretty bad. Usually with Teletubbies and chainsaws and all that bad stuff.
# Your task is as follows:
# Construct the "__init__" method for a Nightmare class, that takes in 0 arguments (except
self of course)!
# However, you should create an instance variable "topic" that defaults to the string
# "Teletubbies", and an instance variable for an attached Dream that the nightmare is
# in, called "attached_dream" (which defaults to None).
# [1]
# Then, create a method attach_to_dream(d), which attached your nightmare instance
# to a dream via setting attached_dream to the argument Dream d.
# [2, 3]
# Then, create a method has_attached_dream() which returns True if your attached_dream
# is not None, and False otherwise.
# [2, 3]
# Finally, create a method WAKE_ME_UP(), which will set the attached_dream's length to 0,
# and call it's dreams_to_dust() method.
# [2, 3]
# '''
# =============================================================================
def
def
__init__
(
self
):
self
.
topic
=
"Teletubbies"
self
.
attached_dream
=
None
def
def
attach_to_dream
(
self
, d):
self
.
attached_dream
=
d
def
def
has_attached_dream
(
self
):
return
return self
.
attached_dream
!=
None
def
def
WAKE_ME_UP
(
self
):
if
if
(
self
.
has_attached_dream()):
self
.
attached_dream
.
length
= 0
self
.
attached_dream
.
dreams_to_dust()
def
def
return_titles
(list_of_dreams):
to_return_list
=
[]
for
for
dream
in
in
list_of_dreams:
to_return_list
.
append(dream
.
title)
return
return
to_return_list
def
def
WAKE_ME_UP_INSIDE
(cant_wake_up):
for
for
i
in
in
cant_wake_up:
i
.
WAKE_ME_UP()
def
def
how_long_are_my_dreams
(list_of_dreams):
summ
= 0
for
for
j
in
in
list_of_dreams:
summ
+=
j
.
length
return
return
summ
def
def
__str__
(
self
):
print
print
(return_titles())
# --------------------------------------------------------------
# The Testing
# --------------------------------------------------------------
class
class
myTests
myTests
(unittest
.
TestCase):
def
def
test1
(
self
):
# Testing Dream constructor
d
=
Dream(
"my awesome dream"
,
5
)
self
.
assertEqual(d
.
title,
"my awesome dream"
)
self
.
assertEqual(d
.
length,
5
)
self
.
assertEqual(d
.
dream_elements, [])
def
def
test2
(
self
):
# Testing nightmare constructor
n
=
Nightmare()
self
.
assertEqual(n
.
topic,
"Teletubbies"
)
self
.
assertEqual(n
.
attached_dream,
None
)
def
def
test3
(
self
):
# Testing Dream.change_title()
d
=
Dream(
"my awesome dream"
,
5
)
d
.
change_title(
"my great dream"
)
self
.
assertEqual(d
.
title,
"my great dream"
)
def
def
test4
(
self
):
# Testing Dream.add_to_dream()
d
=
Dream(
"my awesome dream"
,
5
)
d
.
change_title(
"my great dream"
)
d
.
add_to_dream(
"rainbows"
)
d
.
add_to_dream(
"butterflies"
)
self
.
assertEqual(d
.
dream_elements, [
"rainbows"
,
"butterflies"
])
def
def
test5
(
self
):
# Testing Dream.dreams_to_dust()
d
=
Dream(
"my ruined dream"
,
5
)
d
.
add_to_dream(
"rainbows"
)
d
.
add_to_dream(
"butterflies"
)
d
.
add_to_dream(
"horsies"
)
d
.
dreams_to_dust()
self
.
assertEqual(d
.
dream_elements, [
"dust"
,
"dust"
,
"dust"
])
def
def
test6
(
self
):
# Testing Nightmare.attach_to_dream()
n
=
Nightmare()
d
=
Dream(
"my awesome dream"
,
5
)
n
.
attach_to_dream(d)
self
.
assertEqual(n
.
attached_dream, d)
def
def
test7
(
self
):
n
=
Nightmare()
d
=
Dream(
"my awesome dream"
,
5
)
self
.
assertEqual(n
.
has_attached_dream(),
False
)
n
.
attach_to_dream(d)
self
.
assertEqual(n
.
has_attached_dream(),
True
)
def
def
test8
(
self
):
# Testing Nightmare.WAKE_ME_UP()
n
=
Nightmare()
d
=
Dream(
"my awesome dream"
,
5
)
d
.
add_to_dream(
"pumpkins"
)
n
.
attach_to_dream(d)
n
.
WAKE_ME_UP()
self
.
assertEqual(d
.
length,
0
)
self
.
assertEqual(d
.
dream_elements, [
"dust"
])
def
def
test9
(
self
):
# Testing return_titles()
d1
=
Dream(
"my awesome dream!"
,
5
)
d2
=
Dream(
"my fantastic dream!!"
,
4
)
d3
=
Dream(
"my superb dream!!!"
,
3
)
self
.
assertEqual(return_titles([d1, d2, d3]), [
"my awesome dream!"
,
"my fantastic dream!!"
,
"my superb dream!!!"
])
def
def
test10
(
self
):
# Testing WAKE_ME_UP_INSIDE()
d1
=
Dream(
"my awesome dream!"
,
5
)
d1
.
add_to_dream(
"sprinkles"
)
d2
=
Dream(
"my fantastic dream!!"
,
4
)
d2
.
add_to_dream(
"cake"
)
d2
.
add_to_dream(
"chocolates"
)
n1
=
Nightmare()
n2
=
Nightmare()
n1
.
attach_to_dream(d1)
n2
.
attach_to_dream(d2)
WAKE_ME_UP_INSIDE([n1, n2])
self
.
assertEqual(d1
.
length,
0
)
self
.
assertEqual(d2
.
length,
0
)
self
.
assertEqual(d1
.
dream_elements, [
"dust"
])
self
.
assertEqual(d2
.
dream_elements, [
"dust"
,
"dust"
])
def
def
test11
(
self
):
# Testing how_long_are_my_dreams()
d1
=
Dream(
"my awesome dream!"
,
5
)
d2
=
Dream(
"my fantastic dream!!"
,
4
)
d3
=
Dream(
"my superb dream!!!"
,
3
)
self
.
assertEqual(how_long_are_my_dreams([d1, d2, d3]),
12
)
if
if
__name__
==
'__main__'
:
unittest
.
main(
exit
=
True
)
#################
## EXAMPLE: inheritance
#################
'''
Here is the implementation of a parent class called
Animal. As you can see, it has the constructor (that __init__)
already implemented, as well as a member method. Implement
the __eq__ method for animal such that Python returns True if
the name and age of both of the animals being compared are identical.
You are to write a child class of Animal called Cat.
Add another member variable to the Cat class called
fur_colour, eye_colour and is_brushed. is_brushed should
be automatically set to False. Add another class function
called brushies. This function will set the is_brushed
member variable to true and print a cat face to the
screen (^._.^)
Finally, finish the __eq__ function for cat, such that it
also compared the other member variables of self to other.
Make two Cat objects in the main and call your member
method for Brushies on one. Then, compare them and print the
result.
'''
class
class
Animal
Animal
():
def
def
__init__
(
self
, name, age):
self
.
name
=
name
self
.
age
=
age
def
def
__eq__
(
self
, other):
return
return
(
self
.
name
==
other
.
name)
and
and
(
self
.
age
==
other
.
age)
def
def
take_for_walk
(
self
):
print
print
(f
"Your {self.name} has gone for a walk!"
)
'''
Put your cat class here.
'''
class
class
Cat
Cat
(Animal):
def
def
__init__
(
self
, name, age, fur_colour, eye_colour, is_brushed):
Animal
.
__init__
(
self
, name, age)
self
.
fc
=
fur_colour
self
.
ec
=
eye_colour
self
.
ib
=
is_brushed
def
def
brushies
(
self
):
if
if self
.
ib
==
True
:
print
print
(
'(^._.^)'
)
def
def
__eq__
(
self
, other):
return
return self
.
ib
==
other
.
ib
if
if
__name__
==
"__main__"
:
c1
=
Cat(
'Theo'
,
2
,
'black'
,
'green'
,
True
)
c2
=
Cat(
'Mooshi'
,
5
,
'white'
,
'black'
,
False
)
c1
.
brushies()
c2
.
brushies()
print
print
(c1
.
__eq__
(c2))
#################
## EXAMPLE: simple Coordinate class
#################
class
class
Coordinate
Coordinate
(
object
):
""" A coordinate made up of an x and y value """
def
def
__init__
(
self
, x, y):
""" Sets the x and y values """
self
.
x
=
x
self
.
y
=
y
def
def
__str__
(
self
):
""" Returns a string representation of self """
return
return
"<"
+
str
(
self
.
x)
+
","
+
str
(
self
.
y)
+
">"
def
def
distance
(
self
, other):
""" Returns the euclidean distance between two points """
x_diff_sq
=
(
self
.
x
-
other
.
x)
**2
y_diff_sq
=
(
self
.
y
-
other
.
y)
**2
return
return
(x_diff_sq
+
y_diff_sq)
**0.5
c
=
Coordinate(
3
,
4
)
origin
=
Coordinate(
0
,
0
)
print
print
(c
.
x, origin
.
x)
print
print
(c
.
distance(origin))
print
print
(Coordinate
.
distance(c, origin))
print
print
(origin
.
distance(c))
print
print
(c)
#################
## EXAMPLE: simple class to represent fractions
## Try adding more built-in operations like multiply, divide
### Try adding a reduce method to reduce the fraction (use gcd)
#################
class
class
Fraction
Fraction
(
object
):
"""
A number represented as a fraction
"""
def
def
__init__
(
self
, num, denom):
""" num and denom are integers """
assert
assert type
(num)
==
int
and
and
type
(denom)
==
int
,
"ints not used"
self
.
num
=
num
self
.
denom
=
denom
def
def
__str__
(
self
):
""" Retunrs a string representation of self """
return
return str
(
self
.
num)
+
"/"
+
str
(
self
.
denom)
def
def
__add__
(
self
, other):
""" Returns a new fraction representing the addition """
top
=
self
.
num
*
other
.
denom
+
self
.
denom
*
other
.
num
bott
=
self
.
denom
*
other
.
denom
return
return
Fraction(top, bott)
def
def
__sub__
(
self
, other):
""" Returns a new fraction representing the subtraction """
top
=
self
.
num
*
other
.
denom
-
self
.
denom
*
other
.
num
bott
=
self
.
denom
*
other
.
denom
return
return
Fraction(top, bott)
def
def
__float__
(
self
):
""" Returns a float value of the fraction """
return
return self
.
num
/
self
.
denom
def
def
inverse
(
self
):
""" Returns a new fraction representing 1/self """
return
return
Fraction(
self
.
denom,
self
.
num)
a
=
Fraction(
1
,
4
)
b
=
Fraction(
3
,
4
)
c
=
a
+
b
# c is a Fraction object
print
print
(c)
print
print
(
float
(c))
print
print
(Fraction
.
__float__
(c))
print
print
(
float
(b
.
inverse()))
##c = Fraction(3.14, 2.7) # assertion error
##print a*b # error, did not define how to multiply two Fraction objects
##############
## EXAMPLE: a set of integers as class
##############
class
class
intSet
intSet
(
object
):
"""
An intSet is a set of integers
The value is represented by a list of ints, self.vals
Each int in the set occurs in self.vals exactly once
"""
def
def
__init__
(
self
):
""" Create an empty set of integers """
self
.
vals
=
[]
def
def
insert
(
self
, e):
""" Assumes e is an integer and inserts e into self """
if
if
not
not
e
in
in
self
.
vals:
self
.
vals
.
append(e)
def
def
member
(
self
, e):
""" Assumes e is an integer
Returns True if e is in self, and False otherwise """
return
return
e
in
in
self
.
vals
def
def
remove
(
self
, e):
""" Assumes e is an integer and removes e from self
Raises ValueError if e is not in self """
try
try
:
self
.
vals
.
remove(e)
except
except
:
raise
raise
ValueError
ValueError
(
str
(e)
+
' not found'
)
def
def
getMembers
(
self
):
"""Returns a list containing the elements of self.
Nothing can be assumed about the order of the elements."""
return
return self
.
vals[:]
def
def
__str__
(
self
):
""" Returns a string representation of self """
self
.
vals
.
sort()
return
return
'{'
+
','
.
join([
str
(e)
for
for
e
in
in
self
.
vals])
+
'}'
# for e in self.vals:
# result = result + str(e) + ','
# return '{' + result[:-1] + '}' # -1 omits trailing comma
# Instantiation is used to create instances of the class.
# For exampl, the following statement creates a new object of type IntSet.
# s is called an instance of IntSet.
s
=
intSet()
print
print
(s)
s
.
insert(
3
)
s
.
insert(
4
)
s
.
insert(
3
)
print
print
(s)
# Attribute references: use dot notation to access attributes associated with the class.
# For example, s.member refers to the method member associated with the instance s of type
IntSet
s
.
member(
3
)
s
.
member(
5
)
s
.
insert(
6
)
print
print
(s)
#s.remove(5) # leads to an error
print
print
(s)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Instructions
The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly.
An example of the program is shown below:
Enter a radius for a circle >> 7
The radius is 7
The diameter is 14
The area is 153.93791
Task 1: The DebugPen class compiles without error.
This task is dependent on completing task #2.
Task 2: The DebugCircle class methods work without errors.
Task 3: The DebugFour1 class compiles without error.
Task 4: The DebugFour1 program accepts user input and displays the correct output.
arrow_forward
PROGRAMMING LANGUAGE: C++
ALSO PUT SCREENSHOTS WITH EVERY TASK.
TASK 1 : A class of ten students took a quiz .The grades (integers are in range 0-100) for this quiz are available to you .Determine class average on quiz .
TASK 2: Write c++ code that print summery of exam result and decide either student should have makeup class or not .If more then 30% of class fails in exam it’s mean they need a makeup class otherwise they don’t need any makeup class. For class strength take input from user
(Hint: take two variables pass and fail)
TASK 3: write a c++ that will determine whether a department-store customer has exceeded the credit limit on the charge account .For each customer , following facts are available :
Account number (an integer)
Balance at beginning of month
Total of all items charged by this customer this month
Total of all credit applied to this customer’s account this month.
Allowed credit limit
You are required to use a while structure to input each of these facts ,…
arrow_forward
Task Performance
A Simple Payroll Program
Objective:
At the end of the activity, the students should be able to:
Create a program that exhibits inheritance and polymorphism.
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
1. Create four (4) Java classes. Name them RunEmployee, Employee, FullTimeEmployee,
PartTimeEmployee. The RunEmployee class shall contain the main method and will be used to
execute the program.
2. Write a simple payroll program that will display employee's information. Refer to the UML Class
Diagram for the names of the variable and method. This should be the sequence of the program
upon execution:
a. Ask the user to input the name of the employee.
а.
b. Prompt the user to select between full time and part time by pressing either F (full time) or P
(part time).
c. If F is pressed, ask the user to type his monthly salary. Then, display his name and monthly
salary.
С.
If P is pressed, ask the user to type his rate (pay) per…
arrow_forward
Python Programming- Invoice Class
Introduction:
Requires creating classes and instantiating objects from created class
Instructions:
Create a class called Invoice that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four pieces of information as data attributes--a part number (a string), a part description (a string), a quantity of the item being purchased (an int.) and a price per item (a Decimal). Your class shold have an __init__ method that intializes the four data ttributes. Provide a property for each data attribute. The quantity and price per item should each be non-negative--use validation in the properties for these data attributes to ensure that they remain valid. Provide a calculate_invoice method that returns the invoice amount (that is, multiplies the quantity by the price per item). Demonstrate class Invoice's capabilities.
Once you have written the class, write a program that creates two Invoice Objects to hold…
arrow_forward
Question 14 papa .use python
Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this line
arrow_forward
Instructions
The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly.
An example of the program is shown below:
Enter name Juan Hello, Juan!
Task 1: The DebugThree3 class compiles without error.
Task 2: The getName() method accepts user input for a name and returns the name value as a String.
Task 3: The displayGreeting() method displays a personalized greeting.
Task 4: The DebugThree3 program accepts user input and displays the correct output.
arrow_forward
Please use Python for this problem:
Make sure code is in correct format so it can run in Python without any errors and has the correct answer. When you are finished, test your solutions using a doctest:
Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that:
The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0.
This applies to the following methods below: __init__, set, up, down
You must write the following methods:
__init__ - constructor. Construct a Volume that is set to a given numeric value, or, if no number given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.)
__repr__ - converts Volume to a str for display, see runs below.
set – sets the volume to…
arrow_forward
Please help with the following: C# .NET
change the main class so that the user is the one that as to put the name in other words. Write a driver class that prompts for the person’s data input, instantiates an object of class HealtProfile and displays the patient’s information from that object by calling the DisplayHealthRecord, method.
MAIN CLASS----------------------
static void Main(string[] args) { // Instance created holding 6 parameters which are field with value HealthProfile heartRate = new HealthProfile("James","Kill",1978, 79.5 ,175.5, 2021 ); heartRate.DisplayPatientRecord();// call the patient record method }
CLASS HeartProfile-------------------
public class HealthProfile { // attibutes which holds the following value private String _FirstName; private String _LastName; private int _BirthYear; private double _Height; private double _Weigth; private int _CurrentYear; public HealthProfile(string firstName, string lastName, int birthYear, double height, double wt, int…
arrow_forward
Objectives:
• More practice designing and writing classes
• Use Java loop structures
• More practice rounding numbers
• Write boolean expression(s)
Background
Companies raise money (to be able to do more) by selling shares of stock. You can buy whole shares of stocks. Stocks prices vary over time. If you buy some shares and then sell them when they are higher, you make money :). For
stocks, the cost basis is the amount that you spent purchasing the shares. It is calculated as the sum of the number of shares purchased multiplied by the purchase price (at the time of the purchase). After selling shares, the profit is
the number of shares sold multiplied the current price, then minus the cost basis.
Look at the following examples for illustrations of calculating the profit.
Example 1 - Acme Corporation
Number of Shares Price per Share
5.50
5.15
100
100
100
6.16
The cost basis is calculated as follows: (100 * 5.50) + (100 * 5.15) + (100 * 6.16) = $1,681.00.
Assume that when you go to sell…
arrow_forward
I need help with this PLEASE NO JAVA NO C++ ONLY PYTHON PLZ
Create a class object with the following attributes and actions:
Class Name
Class number
Classroom
Semester
Level
Subject
Actions:
Store a class list
Print the class list as follows:
Class name Class Number Semester
Level
Subject
Test your object:
Ask the user for all the information and to enter at least 3 classes
test using all the actions of the object
print using the to string action
Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.
arrow_forward
Part 1
E-TechComputer store in Oman sells different electronics products such as Computers, Laptops and accessories.
The store Management requires an automated software to keep track of items in stock and prices. You as a software designer and developer are asked to develop a system for the store.
The first task you should carry out is to design and code a class related to stocked Items. You can name this class as Item_in_Stock.
The class should consist of the following:
• A data member Item_code to store an item code e.g, C15, a data member to store item name, a data member to store item description, a data member to store quantity of items in stock and a data member item_price to store price of an item. You must use the appropriate data types and access specifier for each data member.
• Class should have a constructor that initialises class objects with specified item_code, quantity and price of items.
• Getter and setters methods for all the data members including getItemCat()…
arrow_forward
# Question 10 - To help keep your program files uncluttered, you can store your classes in modules #and _________ the classes you need into your main program.
arrow_forward
#Python IDLE:
#Below is my Pizza class ,how would I write the function described in the attached image,based on this class?
# The Pizza class should have two attributes(data items):
class Pizza:
# The Pizza class should have the following methods/operators):
# __init__ -
# constructs a Pizza of a given size (defaults to ‘M’)
# and with a given set of toppings (defaults to empty set).
def __init__(self, size='M', toppings=set()):
self.size = size
self.toppings = toppings
# setSize – set pizza size to one of ‘S’,’M’or ‘L’
def setSize(self, size):
self.size = size
# getSize – returns size
def getSize(self):
return self.size
# addTopping – adds a topping to the pizza, no duplicates, i.e., adding ‘pepperoni’ twice only adds it once
def addTopping(self, topping):
self.toppings.add(topping)
# removeTopping – removes a topping from the pizza
def removeTopping(self, topping):…
arrow_forward
nyoni.trinos87@gmail.com
Question 1
Using C# create a class called shapes, the class is supposed to have attributes length, width and height. There should be methods for calculating the area and the perimeter of a shape. Create classes circle, rectangle and square to inherit from class shapes. The derived classes should override methods in the parent class for them to calculate their own areas and perimeters.
arrow_forward
#python codeplease find the attached image
arrow_forward
True/False Questions. Circle the correct option.
1.T / F-A class protects the code inside it via inheritance.
2.T / F -PASCAL is used for scientific purposes and work is to be done in batches
3.T / F -In high level programming language Pascal, each program statement ends with the single quotation marks
4.T/ F-In unstructured programming language BASIC, program can be divided into the different procedures
5.T / F-'object program' is also called machine code
6.T / F -Type of program which can be run on different types of computer by making little changes into it is classified as fixed program
7.T / F -OOP paradigm was introduced in Ada with version Ada95.
8.T / F -A collateral command specifies that exactly twocommands may be executed in any order
9.T / F -PYTHON adopts reference semantics. This is especially significant for mutable values, which can be selectively updated
10.T / F-#Character = 256 (ISO LATIN character set)
arrow_forward
Use Python Programming Language
Write code that defines a class named Animal:
Add an attribute for the animal name.
Add an eat() method for Animal that prints ``Munch munch.''
A make_noise() method for Animal that prints ``Grrr says [animal name].''
Add a constructor for the Animal class that prints ``An animal has been born.''
A class named Cat:
Make Animal the parent.
A make_noise() method for Cat that prints ``Meow says [animal name].''
A constructor for Cat that prints ``A cat has been born.''
Modify the constructor so it calls the parent constructor as well.
A class named Dog:
Make Animal the parent.
A make_noise() method for Dog that prints ``Bark says [animal name].''
A constructor for Dog that prints ``A dog has been born.''
Modify the constructor so it calls the parent constructor as well.
Create a test program that will:
Code that creates a cat, two dogs, and an animal.
Sets the name for each animal.
Code that calls eat() and make_noise() for each animal. (Don't…
arrow_forward
9 public class PictureViewer
10{
public static void main(String[] args)
11
12
// Step 2: Fix the syntax errors and submit it
to Codecheck to pass the test.
// Note: Do not add or remove any statements
13
//
14
15
//
Do not change the semantics of each statement
Do not add or remove any variables
Do not add or remove any objects
Magic numbers are allowed
16
17
18
//
19
//
20
// Create an object of Picture by loading an image
Picture pic = Picture("starry_night.png");
21
22
23
// Display the width and height
System.out.println("Width : " + pic.getWidth());
System.out.println("Height:
24
25
+ pic.getHeight());
%3D
26
27
// Increase the width by 50 on both sides and
// increase the height by 40 on both sides.
// Must use dw and dh when calling method grow().
int dw, dh = 40;
pic.grow(dw, dh);
28
29
30
31
32
33
// Display the width and height
System.out.println("Width :
System.out.println("Height:
34
+ pic.getWidth());
+ pic.getHeight());
35
36
37
// Draw the picture
draw();
38
39
40
//…
arrow_forward
c++
You are an IT Manager for a small company and must create a program to track your company’s personal computer inventory.
Create a class that can be used for a Personal Computer. The class should have attributes for the:
Manufacturer (e.g. Dell, Gateway, etc.),Form Factor (laptop/desktop),Serial NumberProcessor ( I3, I5, I7, AMD Ryzen 3, AMD Ryzen 5, etc.),RAM (4, 6, 8, 16, 32, or 64GB),Storage Type (UFS, SDD, HDD) andStorage Size (128GB, 256GB, 512GB, 1TB, 2TB).The constructor must accept the manufacturer, form factor, serial number, processor, RAM, storage type/size.
Create accessor methods that allow these attributes to be retrieved individually.
Create mutator methods that allow the RAM and the storage drive (type and size) to be changed.
Incorporate exception handling to reject invalid values in the constructor and mutator methods.
Create a toString() method formulate a string containing the manufacturer, form factor, serial number, processor, RAM, and storage type/size.…
arrow_forward
java
arrow_forward
1. Instructions: The program you will analyze is shown below. The program listing contains a
class definition followed by a main program. Your task is to find and write the missing code
lines to make the program work correctly. Use the output statements from the print()
statements to reveal this mystery :-) Please write the missing code lines clearly in the empty
boxes.
###### start of Rectangle class
class Rectangle:
def
init
(self,
length, width):
self.length
length
self.width
= width
def GetLength (self):
return self.length
def GetWidth(self):
return self.width
def GetArea (self):
return
self.length * self.width
def SetScale(self, scale):
self.length
self.length *
scale
self.width
self.width * scale
#### start of main program
rec
Rectangle (3,5)
%3D
print ('Rectangle Area: ', rectangleArea)
for
ES
in
range (2,5) :
width =
rec.GetWidth()
arrow_forward
Java question
OverviewIn this assignment, you will create a class Publisher (publisher) and two test classes that create a number of different objects / instances of Publisher. The purpose of the task is to get acquainted with the concepts of object, class, method, constructor and overlay.TaskYou must write a class that represents a publisher (which will then be used in the next assignment). You rename the class to Publisher and are consequently saved in a source file called Publisher.java. The class must contain information about the publisher's name and telephone number.The operations we should be able to perform on objects of the class are to set and retrieve names and set and retrieve telephone numbers. It should also be possible to print all information about a publisher on the screen. In addition to this, the class must also contain a static instance variable for counting the number of created publishers, as well as a method that returns the number of created publishers.You…
arrow_forward
Please Answer this Question quickly..
arrow_forward
Chat Application
Write a GUI Java program to implement a client/server chat application using Java sockets
and threads.
1000
...
Server
Client
Client
Connected to: localhost
Now Connected to localhost
Send
Send
Project instructions
1) The total grade for the project is 05 marks.
2) Each student must implement the project individually without any participation with
other students.
3) Your program has to be split into several appropriate functions and classes.
4) The style and readability of your program will also determine your grade, in addition
to the correctness of the program.
Write your Code here
Paste your Output Screen here
Server
arrow_forward
ISP-JAVA
Create a new program.The program must include instructions for the user. Be in the mindset that the program you develop will be used by anyone. Your program must include (but not limited to) the following:• Comments• Javadoc comments for method(s) and class(s)• Input(s) and output(s)• Decision structures• Loops• Arrays• Methods• Classes & ObjectsBe as creative as possible!
arrow_forward
Py.
arrow_forward
Answer in Swift language please: Urgent
Class design and implementation. create a command line in which you should design and implement a math operation class for fraction calculation. The class name is MOpt, which should include numerator and denominator and support the functions: add, subtract, multiply, and divide.
You should also implement the operation reduce, where all the operations performed (+, -, *, /) must be reduced to the simplest form before being returned.
Your class should perform the following math operations: 1/4 + 3/4 = 4/4 = 1
1/4 – 3/4 = -1/2 (not -2/4 using operation reduce)
3/4 – 1/4 = 1/2 (not 2/4)
1/2 * 2/5 = 1/5 (not 2/10)
1/4 / 1/2 = 1/2 (not 2/4)
Your code should look like this:
let m1 = MOpt(1, 4)
let m2 = MOpt(3, 4)
let m3 = MOpt(1, 2)
let m4 = MOpt(2, 5)
let m5 = m1.add(m2)
let m6 = m1.subtract(m2)
let m7 = m2.subtract(m1)
let m8 = m3.multiply(m4)
let m9 = m1.divide(m3)
The toString() method should be create to print out the fraction number, for example,…
arrow_forward
2-Define a class named Wall . The class should have two private double variables,one to store the length of the Wall and another to store the height . Write Input andoutput function .Add accessor and mutator functions to read and set both variables.Add another function that returns the area of the Wall as double Write programthat tests all your functions for at least three different Wall objects.
arrow_forward
Create a flowchart and modify the code.
INSTRUCTION:
Create a new class called CalculatorWithMod. This class should be a sub class of the base class Calculator. This class should also have an additional method for calculating the modulo. The modulo method should only be seen at the sub class, and not the base class! Include exception handling for instances when dividing by 0 or calculating the modulo with 0. You would need to use throw, try, and catch. The modulo (or "modulus" or "mod") is the remainder after dividing one number by another.Example: 20 mod 3 equals 2Because 20/3 = 6 with a remainder of 2
CODE TO COPY:
#include <iostream>
using namespace std;
class Calculator{public:Calculator(){printf("Welcome to my Calculator\n");
}
int addition(int a, int b);int subtraction(int a, int b);int multiplication(int a, int b);float division(int a, int b);};
int Calculator::addition(int a, int b){return (a+b);}
int Calculator::subtraction(int a, int b){return (a-b);}
int…
arrow_forward
Please use Python for this problem:
Please make sure your code is in the correct format and please post a screenshot of the code from Python. When you are finished, test your solutions using a doctest:
Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that:
The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0.
This applies to the following methods below: __init__, set, up, down
You must write the following methods:
__init__ - constructor. Construct a Volume that is set to a given numeric value, or, if no number given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.)
__repr__ - converts Volume to a str for display, see runs below.
set – sets the volume to…
arrow_forward
Program - Python
Make a class names Foo
Add a class variable to foo called x and initialize it to 5
Add an instance variable to foo called y and initialize it to 100
Make an instance of the class, call it f
Using f, print out the value of x
Print out the value of y
arrow_forward
* CENGAGE MINDTAP
Programming Exercise 4-1
Instructions
FormLetterWriter.java
+
1
Modify the class named FormLetterWriter that includes two
overloaded methods named displaySalutation().The first
method takes one String parameter that represents a customer's
last name, and it displays the salutation Dear Mr. or Ms. followed by
the last name. The second method accepts two String
parameters that represent a first and last name, and it displays the
greeting Dear followed by the first name, a space, and the last
name. After each salutation, display the rest of a short business
letter using the displayLetter method: Thank you for your
recent order.
An example of the program is shown below:
Dear Mr. or Ms. Kelly,
Thank you for your recent order.
Dear Christy Johnson,
Thank you for your recent order.
arrow_forward
Java Question
OverviewThe task in this part is also to create a class and a number of objects / instances of this class. To be able to do this, we also need a test class.The purpose of the task is to, in addition to getting acquainted with the concepts of object, class, constructor and overlay, learn to use other classes in a class. You should also know how we use the Integer class to convert strings to integers.TaskYou must create a class that represents a CD. The class should be named CD and saved in a source file called CD.java. The class must contain data about the CD's title, artist, length (in number of seconds) and which publisher the disc is published on.Methods must be available for setting and retrieving data for all instance variables, as well as for printing all information about the disk. The setPublisher method should be superimposed so that a variant takes an object of the type Publisher as an argument. The second variant should take two strings as arguments, which…
arrow_forward
Java program
Overview
In this task, you will try to create a class called Course and a test class that creates objects of the Course class. The purpose is to teach you to write classes, create objects of classes and call public methods on the created object.
Task
Write a class that represents a course given here at San University. The class must contain information about the course course code, name, number of credits and a description.
This should be stored as instance variables in the class. Use English names for all variables and methods. All instance variables must use the String class as the data type. The instance variables may only be accessible within the own class.
It must be possible to set new values for all instance variables in the class. This must be implemented with public set methods for all instance variables. There should also be a method that prints all information about the course on the screen in a "nice" and structured way.
You must also write a "test class"…
arrow_forward
python program for each question
1- Write a Shopping Cart class to implement a shopping cart that you often find on websites where you could purchase some goods. Think about what things could you store in a cart and also what operations you could perform on the cart. To simplify matters, you could consider the website to be an electronics e-store that has goods like flat-panel TVs, boomboxes, iPods, camcorders and so on.
2- Design a class for an airline ticket. Some of the field of an airline ticket are start, destination, date of travel, class, price of ticket, number of people traveling, and name of the primary person. Think about the methods appropriate for the class. Add one method call reserve, which will take details of passengers, start date, starting point, destination and other related attributes and returns flight details.
3- Create a class named Player with a default name as "player", a default level as 0, and a default class type of "fighter". Feel free to add any…
arrow_forward
PLEASE CODE IN PYTHON
PLEASE USE CLASSES
In the Game of 21, a player is dealt two cards from a deck of playing cards and then optionally givena third card. The player closest to 21 points without going over is the winner. Use object-orienteddevelopment to create a Game of 21 application that allows the user to play the Game of 21 against thecomputer. The Game of 21 application and its objects should:
• Deal a card from a deck of playing cards by generating a random number between 1and 13. A 1 corresponds to an Ace, numbers 2 through 10 correspond to those cards,and 11 through 13 correspond to Jack, Queen, and King. The Jack, Queen, and Kinghave a value of 10 in the Game of 21. An Ace can have a value of either 1 or 11.
• Allow the player to stay with two cards or be given a third card.
• Announce the winner.
• Play rounds until the player says to stop.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
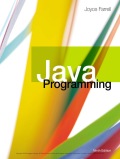
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Related Questions
- Instructions The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. An example of the program is shown below: Enter a radius for a circle >> 7 The radius is 7 The diameter is 14 The area is 153.93791 Task 1: The DebugPen class compiles without error. This task is dependent on completing task #2. Task 2: The DebugCircle class methods work without errors. Task 3: The DebugFour1 class compiles without error. Task 4: The DebugFour1 program accepts user input and displays the correct output.arrow_forwardPROGRAMMING LANGUAGE: C++ ALSO PUT SCREENSHOTS WITH EVERY TASK. TASK 1 : A class of ten students took a quiz .The grades (integers are in range 0-100) for this quiz are available to you .Determine class average on quiz . TASK 2: Write c++ code that print summery of exam result and decide either student should have makeup class or not .If more then 30% of class fails in exam it’s mean they need a makeup class otherwise they don’t need any makeup class. For class strength take input from user (Hint: take two variables pass and fail) TASK 3: write a c++ that will determine whether a department-store customer has exceeded the credit limit on the charge account .For each customer , following facts are available : Account number (an integer) Balance at beginning of month Total of all items charged by this customer this month Total of all credit applied to this customer’s account this month. Allowed credit limit You are required to use a while structure to input each of these facts ,…arrow_forwardTask Performance A Simple Payroll Program Objective: At the end of the activity, the students should be able to: Create a program that exhibits inheritance and polymorphism. Software Requirements: Latest version of NetBeans IDE Java Development Kit (JDK) 8 Procedure: 1. Create four (4) Java classes. Name them RunEmployee, Employee, FullTimeEmployee, PartTimeEmployee. The RunEmployee class shall contain the main method and will be used to execute the program. 2. Write a simple payroll program that will display employee's information. Refer to the UML Class Diagram for the names of the variable and method. This should be the sequence of the program upon execution: a. Ask the user to input the name of the employee. а. b. Prompt the user to select between full time and part time by pressing either F (full time) or P (part time). c. If F is pressed, ask the user to type his monthly salary. Then, display his name and monthly salary. С. If P is pressed, ask the user to type his rate (pay) per…arrow_forward
- Python Programming- Invoice Class Introduction: Requires creating classes and instantiating objects from created class Instructions: Create a class called Invoice that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four pieces of information as data attributes--a part number (a string), a part description (a string), a quantity of the item being purchased (an int.) and a price per item (a Decimal). Your class shold have an __init__ method that intializes the four data ttributes. Provide a property for each data attribute. The quantity and price per item should each be non-negative--use validation in the properties for these data attributes to ensure that they remain valid. Provide a calculate_invoice method that returns the invoice amount (that is, multiplies the quantity by the price per item). Demonstrate class Invoice's capabilities. Once you have written the class, write a program that creates two Invoice Objects to hold…arrow_forwardQuestion 14 papa .use python Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forwardInstructions The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. An example of the program is shown below: Enter name Juan Hello, Juan! Task 1: The DebugThree3 class compiles without error. Task 2: The getName() method accepts user input for a name and returns the name value as a String. Task 3: The displayGreeting() method displays a personalized greeting. Task 4: The DebugThree3 program accepts user input and displays the correct output.arrow_forward
- Please use Python for this problem: Make sure code is in correct format so it can run in Python without any errors and has the correct answer. When you are finished, test your solutions using a doctest: Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that: The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0. This applies to the following methods below: __init__, set, up, down You must write the following methods: __init__ - constructor. Construct a Volume that is set to a given numeric value, or, if no number given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.) __repr__ - converts Volume to a str for display, see runs below. set – sets the volume to…arrow_forwardPlease help with the following: C# .NET change the main class so that the user is the one that as to put the name in other words. Write a driver class that prompts for the person’s data input, instantiates an object of class HealtProfile and displays the patient’s information from that object by calling the DisplayHealthRecord, method. MAIN CLASS---------------------- static void Main(string[] args) { // Instance created holding 6 parameters which are field with value HealthProfile heartRate = new HealthProfile("James","Kill",1978, 79.5 ,175.5, 2021 ); heartRate.DisplayPatientRecord();// call the patient record method } CLASS HeartProfile------------------- public class HealthProfile { // attibutes which holds the following value private String _FirstName; private String _LastName; private int _BirthYear; private double _Height; private double _Weigth; private int _CurrentYear; public HealthProfile(string firstName, string lastName, int birthYear, double height, double wt, int…arrow_forwardObjectives: • More practice designing and writing classes • Use Java loop structures • More practice rounding numbers • Write boolean expression(s) Background Companies raise money (to be able to do more) by selling shares of stock. You can buy whole shares of stocks. Stocks prices vary over time. If you buy some shares and then sell them when they are higher, you make money :). For stocks, the cost basis is the amount that you spent purchasing the shares. It is calculated as the sum of the number of shares purchased multiplied by the purchase price (at the time of the purchase). After selling shares, the profit is the number of shares sold multiplied the current price, then minus the cost basis. Look at the following examples for illustrations of calculating the profit. Example 1 - Acme Corporation Number of Shares Price per Share 5.50 5.15 100 100 100 6.16 The cost basis is calculated as follows: (100 * 5.50) + (100 * 5.15) + (100 * 6.16) = $1,681.00. Assume that when you go to sell…arrow_forward
- I need help with this PLEASE NO JAVA NO C++ ONLY PYTHON PLZ Create a class object with the following attributes and actions: Class Name Class number Classroom Semester Level Subject Actions: Store a class list Print the class list as follows: Class name Class Number Semester Level Subject Test your object: Ask the user for all the information and to enter at least 3 classes test using all the actions of the object print using the to string action Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.arrow_forwardPart 1 E-TechComputer store in Oman sells different electronics products such as Computers, Laptops and accessories. The store Management requires an automated software to keep track of items in stock and prices. You as a software designer and developer are asked to develop a system for the store. The first task you should carry out is to design and code a class related to stocked Items. You can name this class as Item_in_Stock. The class should consist of the following: • A data member Item_code to store an item code e.g, C15, a data member to store item name, a data member to store item description, a data member to store quantity of items in stock and a data member item_price to store price of an item. You must use the appropriate data types and access specifier for each data member. • Class should have a constructor that initialises class objects with specified item_code, quantity and price of items. • Getter and setters methods for all the data members including getItemCat()…arrow_forward# Question 10 - To help keep your program files uncluttered, you can store your classes in modules #and _________ the classes you need into your main program.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
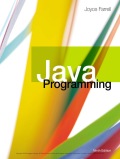
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT