lab06_simulation (1)
.html
keyboard_arrow_up
School
Temple University *
*We aren’t endorsed by this school
Course
1013
Subject
Computer Science
Date
Dec 6, 2023
Type
html
Pages
17
Uploaded by samzahroun
Lab 6: Simulation
¶
Elements of Data Science
Welcome to Module 2 and lab 6! This week, we will go over conditionals and iteration,
and introduce the concept of randomness. All of this material is covered in
Chapter 9
and
Chapter 10
of the online
Inferential Thinking
textbook.
First, set up the tests and imports by running the cell below.
In [343]:
name = "Sam"
In [344]:
import numpy as np
from datascience import *
%matplotlib inline
import matplotlib.pyplot as plt
plt.style.use('ggplot')
import os
user = os.getenv('JUPYTERHUB_USER')
from gofer.ok import check
1. Sampling
¶
1.1 Dungeons and Dragons and Sampling
¶
In the game Dungeons & Dragons, each player plays the role of a fantasy character. A
player performs actions by rolling a 20-sided die, adding a "modifier" number to the roll,
and comparing the total to a threshold for success. The modifier depends on her
character's competence in performing the action. For example, suppose Alice's
character, a barbarian warrior named Roga, is trying to knock down a heavy door. She
rolls a 20-sided die, adds a modifier of 11 to the result (because her character is good at
knocking down doors), and
succeeds if the total is greater than 15
.
A Medium posting discusses probability in the context of Dungeons and Dragons
https://towardsdatascience.com/understanding-probability-theory-with-dungeons-and-
dragons-a36bc69aec88
Question 1.1
Write code that simulates that procedure. Compute three values: the
result of Alice's roll (roll_result), the result of her roll plus Roga's modifier
(modified_result), and a boolean value indicating whether the action succeeded
(action_succeeded). Do not fill in any of the results manually; the entire simulation
should happen in code. Hint: A roll of a 20-sided die is a number chosen uniformly from
the array make_array(1, 2, 3, 4, ..., 20). So a roll of a 20-sided die plus 11 is a number
chosen uniformly from that array, plus 11.
In [345]:
possible_rolls = np.arange(1,21,1)
roll_result = np.random.choice(possible_rolls)
modified_result = roll_result + 11
action_succeeded = modified_result > 15
# The next line just prints out your results in a nice way
# once you're done.
You can delete it if you want.
print(f"On a modified roll of {modified_result}, Alice's action {'succeeded' if
action_succeeded else 'failed'}")
On a modified roll of 24, Alice's action succeeded
In [346]:
check('tests/q1.1.py')
Out[346]:
All tests passed!
Question 1.2
Run your cell 7 times to manually estimate the chance that Alice succeeds
at this action. (Don't use math or an extended simulation.). Your answer should be a
fraction.
In [347]:
rough_success_chance = 7/7
In [348]:
check('tests/q1.2.py')
Out[348]:
All tests passed!
Suppose we don't know that Roga has a modifier of 11 for this action. Instead, we
observe the modified roll (that is, the die roll plus the modifier of 11) from each of 7 of
her attempts to knock down doors. We would like to estimate her modifier from these 7
numbers.
Question 1.3
Write a Python function called
simulate_observations
. It should take
two arguments, the modifier and num_oobservations, and it should return an array of
num_observations. Each of the numbers should be the modified roll from one simulation.
Then
, call your function once to compute an array of 7 simulated modified rolls. Name
that array
observations
.
In [349]:
modifier = 11
num_observations = 7
def simulate_observations(modifier, num_observations):
"""Produces an array of 7 simulated modified die rolls"""
possible_rolls = np.arange(1,21)
obs = make_array()
for num in np.arange(num_observations):
obs = np.append(obs, (np.random.choice(possible_rolls) + modifier))
return (obs)
observations = simulate_observations(modifier, num_observations)
observations
Out[349]:
array([ 14.,
20.,
30.,
27.,
31.,
29.,
18.])
In [350]:
check('tests/q1.3.py')
Out[350]:
All tests passed!
Question 1.4
Draw a histogram to display the
probability distribution
of the modified
rolls we might see. Check with a neighbor or a CA to make sure you have the right
histogram. Carry this out again using 100 rolls.
In [351]:
num_observations = 100
def roll_sim(mod, num_observtions):
"""Produces the probability distribution of the seven observations from the preveous
code cell."""
possible_rolls = np.arange(1,21)
modrolls = np.random.choice(possible_rolls, num_observations) + mod
return modrolls
In [352]:
# We suggest using these bins.
roll_bins = np.arange(1, modifier+2+20, 1)
roll_bins
plt.hist(roll_sim(11,100))
Out[352]:
(array([ 12.,
14.,
10.,
9.,
9.,
12.,
6.,
7.,
13.,
8.]),
array([ 12. ,
13.9,
15.8,
17.7,
19.6,
21.5,
23.4,
25.3,
27.2,
29.1,
31. ]),
<BarContainer object of 10 artists>)
Estimate the modifier
¶
Now let's imagine we don't know the modifier and try to estimate it from observations.
One straightforward (but clearly suboptimal) way to do that is to find the smallest total
roll, since the smallest roll on a 20-sided die is 1, which is roughly 0. Use a random
number for
modifier
to start and keep this value through the next questions. We will also
generate 100 rolls based on the below unknown modifier.
Question 1.5
Using that method, estimate modifier from observations. Name your
estimate min_estimate.
In [353]:
modifier = np.random.randint(1,20) # Generates a random integer modifier from 1 to 20
inclusive
observations = simulate_observations(modifier, num_observations)
min_roll = min(observations)
min_estimate = min_roll -1
min_estimate
Out[353]:
1.0
In [354]:
check('tests/q1.5.py')
Out[354]:
All tests passed!
Estimate the modifier based on the mean of observations.
¶
Question 1.6
Figure out a good estimate based on that quantity. Then, write a function
named mean_based_estimator that computes your estimate. It should take an array of
modified rolls (like the array observations) as its argument and return an estimate (single
number)of the modifier based on those numbers contianed in the array.
In [355]:
def mean_based_estimator(obs):
"""Estimate the roll modifier based on observed modified rolls in the array nums."""
return int(round(np.mean(obs)- 11))
# Here is an example call to your function.
It computes an estimate
# of the modifier from our
observations.
mean_based_estimate = mean_based_estimator(observations)
print(mean_based_estimate)
1
In [356]:
check('tests/q1.6.py')
Out[356]:
All tests passed!
Question 1.7
Construct a histogram and compare to above estimates, are they
consistent? What is your best estimate of the random modiifer based on the above,
without examining the value?
In [357]:
plt.hist(observations, bins = roll_bins) # Use to plot histogram of an array of 100
modified rolls
estimated_modifier = mean_based_estimator(observations)
In [358]:
check('tests/q1.7.py')
Out[358]:
All tests passed!
2. Sampling and GC content of DNA sequence
¶
DNA within a cell contains codes or sequences for the ultimate synthesis of proteins. In
DNA is made up of four types of nucleotides, guanine (G), cytosine (C), adenine (A), and
thymine (T) connected in an ordered sequence. These nucleotides on a single strand pair
with complimentary nucleotides on a second strand, G pairs with C and A with T. Regions
of DNA code for RNA which ultimately directs protein synthesis and these segments are
known as genes and these segments often have higher GC content. Here we will sample
10 nuclotide segments of a DNA sequence and determine the GC content of these DNA
segments. See
DNA sequnce basics
and
GC Content details
.
Our goal is to sample portions (10 nucelotides) of the sequence and determine
the relative content of guanine (G) and cytosine (C) to adenine (A) and thymine (T)
In [359]:
# DNA sequence we will examine, a string
seq = "CGTAACAAGGTTTCCGTAGGTGAACCTGCGGAAGGATCATTGATGAGACCGTGGAATAAACGATCGAGTG \
AATCCGGAGGACCGGTGTACTCAGCTCACCGGGGGCATTGCTCCCGTGGTGACCCTGATTTGTTGTTGGG \
CCGCCTCGGGAGCGTCCATGGCGGGTTTGAACCTCTAGCCCGGCGCAGTTTGGGCGCCAAGCCATATGAA \
AGCATCACCGGCGAATGGCATTGTCTTCCCCAAAACCCGGAGCGGCGGCGTGCTGTCGCGTGCCCAATGA \
ATTTTGATGACTCTCGCAAACGGGAATCTTGGCTCTTTGCATCGGATGGAAGGACGCAGCGAAATGCGAT \
AAGTGGTGTGAATTGCAAGATCCCGTGAACCATCGAGTCTTTTGAACGCAAGTTGCGCCCGAGGCCATCA \
GGCTAAGGGCACGCCTGCTTGGGCGTCGCGCTTCGTCTCTCTCCTGCCAATGCTTGCCCGGCATACAGCC \
AGGCCGGCGTGGTGCGGATGTGAAAGATTGGCCCCTTGTGCCTAGGTGCGGCGGGTCCAAGAGCTGGTGT \
TTTGATGGCCCGGAACCCGGCAAGAGGTGGACGGATGCTGGCAGCAGCTGCCGTGCGAATCCCCCATGTT \
GTCGTGCTTGTCGGACAGGCAGGAGAACCCTTCCGAACCCCAATGGAGGGCGGTTGACCGCCATTCGGAT \
GTGACCCCAGGTCAGGCGGGGGCACCCGCTGAGTTTACGC" # LCBO-Prolactin precursor-Bovine
seq
Out[359]:
'CGTAACAAGGTTTCCGTAGGTGAACCTGCGGAAGGATCATTGATGAGACCGTGGAATAAACGATCGAGTG
AATCCGGAGGACCGGTGTACTCAGCTCACCGGGGGCATTGCTCCCGTGGTGACCCTGATTTGTTGTTGGG
CCGCCTCGGGAGCGTCCATGGCGGGTTTGAACCTCTAGCCCGGCGCAGTTTGGGCGCCAAGCCATATGAA
AGCATCACCGGCGAATGGCATTGTCTTCCCCAAAACCCGGAGCGGCGGCGTGCTGTCGCGTGCCCAATGA
ATTTTGATGACTCTCGCAAACGGGAATCTTGGCTCTTTGCATCGGATGGAAGGACGCAGCGAAATGCGAT
AAGTGGTGTGAATTGCAAGATCCCGTGAACCATCGAGTCTTTTGAACGCAAGTTGCGCCCGAGGCCATCA
GGCTAAGGGCACGCCTGCTTGGGCGTCGCGCTTCGTCTCTCTCCTGCCAATGCTTGCCCGGCATACAGCC
AGGCCGGCGTGGTGCGGATGTGAAAGATTGGCCCCTTGTGCCTAGGTGCGGCGGGTCCAAGAGCTGGTGT
TTTGATGGCCCGGAACCCGGCAAGAGGTGGACGGATGCTGGCAGCAGCTGCCGTGCGAATCCCCCATGTT
GTCGTGCTTGTCGGACAGGCAGGAGAACCCTTCCGAACCCCAATGGAGGGCGGTTGACCGCCATTCGGAT
GTGACCCCAGGTCAGGCGGGGGCACCCGCTGAGTTTACGC'
Question 2.1A
Run the first two code cells below to see how substrings are extracted
and how a character can be counted within a substring. Use the same strategy to
determine GC content as fraction of the total in the first 10 nucleotides in the larger
sequence above,
seq
In [360]:
# Example A
samplesize = 4
# Use this short sequence in this example
seq0 = 'GTGAAAGATT'
# How to get a substring
seq0[0:samplesize]
Out[360]:
'GTGA'
In [361]:
# Example B
# How to count the number of times 'A' appears in sequence
seq0[0:samplesize].count('A')
Out[361]:
1
In [362]:
GCcount = seq[0:10].count('G') + seq[0:10].count('C')
GCfraction = (GCcount)/(seq[0:10].count('A') + seq[0:10].count('T') + GCcount)
GCfraction
Out[362]:
0.5
Lists
¶
Below we assemble a list and append an additional entry, 0.7. A useful strategy in
creating your function
In [363]:
gc = []
gc.append(0.8)
gc.append(0.7)
gc
Out[363]:
[0.8, 0.7]
Fill a list with 30 random G, C, T, A nucleotides
¶
use iteration and
np.random.choice
In [364]:
my_sim_seq = []
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Solve using integer programming
arrow_forward
Write this program in Java using a custom method.
Implementation details
You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists.
Use an array of strings to store the 4 strings listed in the description.
Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below:
determine the fruits to display (step 3 below) and print them
determine if there are 3 or 4 of the same image
display the results
update the customer balance as necessary
prompt to play or quit
continue loop if customer wants to play and there's money for another game.
Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an…
arrow_forward
I already have the code for the assignment below, but the code has an error in the driver class. Please help me fix it.
The assignment:
Make a recursive method for factoring an integer n. First, find a factor f, then recursively factor n / f. This assignment needs a resource class and a driver class. The resource class and the driver class need to be in two separate files. The resource class will contain all of the methods and the driver class only needs to call the methods. The driver class needs to have only 5 lines of code
The code of the resource class:
import java.util.ArrayList;import java.util.List;
public class U10E03R{
// Recursive function to // print factors of a number public static void factors(int n, int i) { // Checking if the number is less than N if (i <= n) { if (n % i == 0) { System.out.print(i + " "); } // Calling the function recursively // for the next number factors(n, i +…
arrow_forward
do in java
arrow_forward
Personal project Q5.
This question is concerned with the design and analysis of recursive algorithms.
You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence ? of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops.
FindAverageAndProduct(a1, ...., an) such that n > 1
Input: A sequence of real values A = (a1, ..., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A.
Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables.
(a) Produce a pseudo code design for a recursive algorithm to solve this problem.
(b) Draw a call-stack…
arrow_forward
The for construct is a kind of loop that iteratively processes a sequence of items. So long as there are things to process, it will continue to operate. In your opinion, how true is this statement?
arrow_forward
2
UP FAMNIT, DSA 2021/22 - First midterm 211203
Page 2 of 4
UP FAMNIT, DSA 2021/22 - First midterm 211203
3
1. task: Introduction and basics. And again, Peter Puzzle has found a piece of
paper with the following code:
int FooBar (A, i) {
n=
len(A);
if (i == n-1) return A;
for (j= (i + 1); j < n; j++) {
}
}
if (A[i] < A[j]) { temp= A[i]; A[i]= A[j]; A[j]= temp; }
return FooBar (A, i+1)
QUESTIONS:
A) (i.) What is the loop invariant for the for loop based on which you can
show what the function actually returns? (ii.) Show that it remains invariant
throughout the execution of the function.
B) (i.) What does the array A returned by the function FooBar (A, i) contain?
Justify your answer. (ii.) What does the array A returned by the function
FooBar (A, 0) contain? Justify your answer.
HINT: Use induction.
C) (i.) What is the time complexity of the function FooBar if we count the
number of comparisons over the elements of the array A? Justify your answer.
HINT: You will earn more points, if…
arrow_forward
Create, compile, and run a recursive program. Choose one of the labs listed below and program it. More details for the
lab can be found in the first PowerPoint for Chapter 9. Make sure your code is recursive. You can think of recursive
code this way: If you cut and paste a recursive method into another class, it should still run without problems. In other
words, you cannot have any instance variables (class-level variables) that the method depends on.
Choice 1 - boolean isPalindrome (String str) that returns true if the input string is a palindrome.
Choice 2 - boolean find (string fullStr, string subStr) that tests whether the string subStr is a substring of fullStr
Choice 3- int numDigits (int num) to determine the number of digits in the number num
Choice 4 - double intPower (double x, int n) to compute x^n, where n is a positive integer and x is a double precision
variable
Choice 5-void printSubstr (String str) that prints all substrings of the input string.
arrow_forward
Problem 1:
Create a Java class RecursiveMethods.java and create the following methods
inside:
ALL THE METHODS NEED TO BE COMPLETED RECURSIVLY. NO LOOPS ALLOWED.
oddEvenMatch Rec: the method takes an integer array as a parameter
and returns a boolean. The method returns true if every odd index
contains an odd integer AND every even index contains an even integer(0 is
even). Otherwise it returns false.
sumNRec: The method takes an integer array A and returns the sum of all
integers in the parameter array.
nDownToOne: Takes an integer n and prints out the numbers from n down
to 1, each number on its own line.
inputAndPrintReverse: Inputs integers from the user until the user
enters 0, then prints the integers in reverse order. For this method, you
may NOT use an array or any type of array structure, in other words, you
may not use any structure to store the user input.
After completing the methods, use the main method to test them. You can hard
code the tests.
arrow_forward
Assignment 5B: Keeping Score. Now that we know about arrays, we can keep track of when
events occur during our program. To prove this, let's create a game of Rock-Paper-Scissors.
For those not familiar with this game, the basic premise is that each player says "Rock-Paper-
Scissors!" and then makes their hand into the shape of one of the three objects. Winners are
determined based on the following rules:
Rock beats Scissors
Scissors beats Paper
Paper beats Rock
At the start of the program, it will ask the player how many rounds of Rock-Paper-
Scissors they want to play. After this, the game will loop for that many number of times.
Each loop, it will ask the player what item they want to use – Rock, Paper, or Scissors.
The computer will randomly generate its own item, and a winner will be determined.
The game will then save the result as an element of an array, and the next round will
begin.
Once all the rounds have been played, the program will say "Game Over" and display a
list of who…
arrow_forward
Personal project Q5.
This question is concerned with the design and analysis of recursive algorithms.
You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence A of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops.
FindAverageAndProduct(a1, ...., an) such that n > 1
Input: A sequence of real values A = (a1, ...., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A.
Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables.
(a) Produce a pseudo code design for a recursive algorithm to solve this problem.
(b) Draw a call-stack…
arrow_forward
I need the code from start to end with no errors and the explanation for the code
ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ##########
RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…
arrow_forward
Use java and correctly indent code.
arrow_forward
Knapsack Problem
This exercise is due Tuesday, May 14. It has to be turned in on time in order to get participation credit. I'll come into class and go over the solution right away. Then I will collect your work on the exercise. Be ready to turn it in at that time.
Fill out the table for the knapsack problem, where the objects, weights, and values are as given, and the overall weight limit is 10.
Next, circle the entries in the table that are used when backtracking to find objects to use in the solution.
Then list the object numbers that can be used for an optimal solution.
Also list the weights and values of those objects.
Verify that the values of your solution objects add up to the optimal number in the last row and column in the table.
Verify that the sum of the weights of your solution the objects is not more than the overall weight limit of 10.
Weight Capacity ----->
obj
# wt val | 0 1 2 3 4 5 6 7 8 9 10…
arrow_forward
Please follow directions correctly.
After completing the methods, use the main method to test them.
arrow_forward
Multiple Frequencies. In the last assignment, we calculated the frequency ofa coin flip. This required us to have two separate variables, which we used to record the numberof heads and tails. Now that we know about arrays, we can track the frequency of all numbers ina randomly generated sequence.For this program, you will ask the user to provide a range of values (from 1 to that number,inclusive) and how long of a number sequence you want to generate using that number range.You will then generate and save the sequence in an array. After that, you will count the numberof times each number occurs in the sequence, and print the frequency of each number.Hints: You can use multiple arrays for this assignment. One array should hold thenumber sequence, and another could keep track of the frequencies of each number.Sample Output #1:What’s the highest number you want to generate?: 5How long of a number sequence do you want to generate?: 10Okay, we’ll generate 10 number(s) ranging from 1 to 5!1,…
arrow_forward
need help in writing full code for the Quick select probabilistic version. which divides the given array by 0.20, 0.50 by random pivot
arrow_forward
Use the right loop for the right assignment, using all the follow- ing loops: for, while without hasNext(), while with hasNext() and do-while.
So I cannot use array.
it has to be done in java.
arrow_forward
READY CAREFULLY
Java add comment to code, please write your test-cases on how you would
test your solution assumptions and hence your code.
Example of cases to be tested for are like : What if the array input which
is expected does not exist - that is , input is a null. How should your code
handle such a situation ? Maybe output some message like ”Null input
case, so no output”? What if the length of the array is one ?....so on and
so forth.
Please Remember : Although, written as comments - You will address
your test cases in the form of code and not prose
In this assignment you are required to do a programs which takes as input two sorted arrays and returns a new array containing the elements found in both the sorted arrays. It is alright if the input arrays have duplicates, but the returned array should be duplicate free!
Below is how the arrays are represented
ARRAY1[] = [1, 5, 6, 6, 9, 9, 9, 11, 11, 21]
Here length of ARRAY1 is m.
ARRAY2[] = [6, 6, 9, 11, 21, 21, 21]
Here…
arrow_forward
ANSWER MUST BE IN PYTHON3. METHOD HEADER BELOW.
#!/bin/python3
import math
import os
import random
import re
import sys
import numpy as np
import pandas as pd
from sklearn.linear_model import LinearRegression
def read_dataframe():
# Return a pandas dataframe from stdin
return pd.read_csv(sys.stdin)
def read_matrix():
# Return column names and data as a matrix
df = read_dataframe()
return list(df.columns), df.values
def read_list():
# Return column names and data as a list of lists
# Each element in the list corresponds to columns in the data
col_names, val = read_matrix()
return col_names, val.T.tolist()
#
# Complete the 'main' function below.
#
# The function is expected to return a STRING.
#
def main():
# Write your code here
if __name__ == '__main__':
fptr = open(os.environ['OUTPUT_PATH'], 'w')
result = main()
fptr.write(result + '\n')
fptr.close()
arrow_forward
Please write Python code.
Movie Recommendations via Item-Item Collaborative Filtering. You are provided with real data (Movie-Lens dataset) of user ratings for different movies. There is a readme file that describes the data format. In this project, you will implement the item-item collaborative filtering algorithm that we discussed in the class. The high-level steps are as follows:
a) Construct the profile of each item (i.e., movie). At the minimum, you should use the ratings given by each user for a given item (i.e., movie). Optionally, you can use other information (e.g., genre information for each movie and tag information given by the user for each movie) creatively. If you use this additional information, you should explain your methodology in the submitted report.
b) Compute similarity score for all item-item (i.e., movie-movie) pairs. You will employ the centered cosine similarity metric that we discussed in class.
c) Compute the neighborhood set N for each item (i.e. movie).…
arrow_forward
Java:
Write a recursive method that finds and returns the sum of the elements of an int array. Also, write a program to test your method.
arrow_forward
In java, please read the text file as array. Then, replace the null with 0 and calculate the sum of the new students in ABC University.Example of text file: (university,year,total_students,new_students,new_staff,ABC, 2000, 3457, , ,ABC, 2001, 3675,218, ,EFG, 2000, 2389,602, ,EFG, 2001, 2785,396, 65,)
arrow_forward
What is the probability that in a classroom of x people, at least 2 will be born on the same day of the year (ignore leap year)? Use a Monte Carlo Simulation and a frequency table to write a program that calculates this probability, where the number of people (x) in the simulated class is given by the user.
PLEASE USE the outline of the code GIVEN to answer this question:
import mathimport random
# create and initialize frequency table:ft = []k = 0while(k < 365) : ft.append(0) k = k+1
# Allow the user to determine class size:print("Please type in how many people are in the class: ")x= int(input())
success = 0
# Simulate:c = 0while(c < 10000) : # Step 1: re-initialize birthday frequency table (it must be re-initialized for each play-through (why?): k = 0 while(k < 365) : ft[k] = 0 k = k+1 # Step 2: randomly get x birthdays and update frequency table: k = 0 while(k < x): # your code goes here ##########################
k = k+1 # Step 3:…
arrow_forward
READY CAREFULLY
Java add COMMENT TO CODE, please TEST-CASE on how you would
test your solution assumptions and hence your code.
Example of cases to be tested for are like : What if the array input which
is expected does not exist - that is , input is a null. How should your code
handle such a situation ? Maybe output some message like "Null input
case, so no output"? What if the length of the array is one ?....so on and
so forth.
In this assignment you are required programs which takes as input two sorted arrays and returns a new array containing the elements found in both the sorted arrays. It is alright if the input arrays have duplicates, but the returned array should be duplicate free!
Below is how the arrays are represented
ARRAY1[] = [1, 5, 6, 6, 9, 9, 9, 11, 11, 21]
Here length of ARRAY1 is m.
ARRAY2[] = [6, 6, 9, 11, 21, 21, 21]
Here length of ARRAY2 is n.
Array to be returned would be:
ARRAY[] = [6, 9, 11, 21]
ANSWER THE QUESTION BELOW.
Implement the function…
arrow_forward
Use Java.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
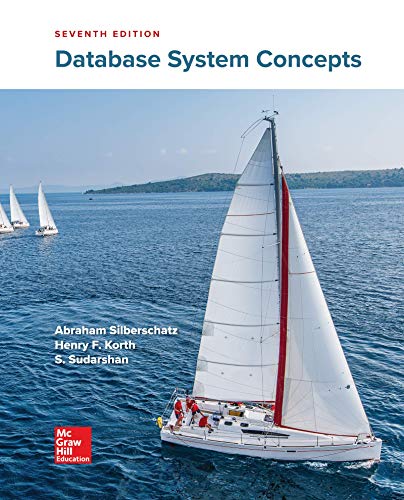
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
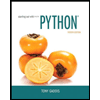
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
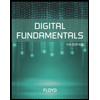
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
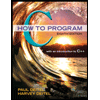
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
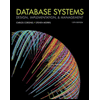
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
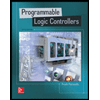
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Solve using integer programmingarrow_forwardWrite this program in Java using a custom method. Implementation details You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists. Use an array of strings to store the 4 strings listed in the description. Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below: determine the fruits to display (step 3 below) and print them determine if there are 3 or 4 of the same image display the results update the customer balance as necessary prompt to play or quit continue loop if customer wants to play and there's money for another game. Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an…arrow_forwardI already have the code for the assignment below, but the code has an error in the driver class. Please help me fix it. The assignment: Make a recursive method for factoring an integer n. First, find a factor f, then recursively factor n / f. This assignment needs a resource class and a driver class. The resource class and the driver class need to be in two separate files. The resource class will contain all of the methods and the driver class only needs to call the methods. The driver class needs to have only 5 lines of code The code of the resource class: import java.util.ArrayList;import java.util.List; public class U10E03R{ // Recursive function to // print factors of a number public static void factors(int n, int i) { // Checking if the number is less than N if (i <= n) { if (n % i == 0) { System.out.print(i + " "); } // Calling the function recursively // for the next number factors(n, i +…arrow_forward
- do in javaarrow_forwardPersonal project Q5. This question is concerned with the design and analysis of recursive algorithms. You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence ? of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops. FindAverageAndProduct(a1, ...., an) such that n > 1 Input: A sequence of real values A = (a1, ..., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A. Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables. (a) Produce a pseudo code design for a recursive algorithm to solve this problem. (b) Draw a call-stack…arrow_forwardThe for construct is a kind of loop that iteratively processes a sequence of items. So long as there are things to process, it will continue to operate. In your opinion, how true is this statement?arrow_forward
- 2 UP FAMNIT, DSA 2021/22 - First midterm 211203 Page 2 of 4 UP FAMNIT, DSA 2021/22 - First midterm 211203 3 1. task: Introduction and basics. And again, Peter Puzzle has found a piece of paper with the following code: int FooBar (A, i) { n= len(A); if (i == n-1) return A; for (j= (i + 1); j < n; j++) { } } if (A[i] < A[j]) { temp= A[i]; A[i]= A[j]; A[j]= temp; } return FooBar (A, i+1) QUESTIONS: A) (i.) What is the loop invariant for the for loop based on which you can show what the function actually returns? (ii.) Show that it remains invariant throughout the execution of the function. B) (i.) What does the array A returned by the function FooBar (A, i) contain? Justify your answer. (ii.) What does the array A returned by the function FooBar (A, 0) contain? Justify your answer. HINT: Use induction. C) (i.) What is the time complexity of the function FooBar if we count the number of comparisons over the elements of the array A? Justify your answer. HINT: You will earn more points, if…arrow_forwardCreate, compile, and run a recursive program. Choose one of the labs listed below and program it. More details for the lab can be found in the first PowerPoint for Chapter 9. Make sure your code is recursive. You can think of recursive code this way: If you cut and paste a recursive method into another class, it should still run without problems. In other words, you cannot have any instance variables (class-level variables) that the method depends on. Choice 1 - boolean isPalindrome (String str) that returns true if the input string is a palindrome. Choice 2 - boolean find (string fullStr, string subStr) that tests whether the string subStr is a substring of fullStr Choice 3- int numDigits (int num) to determine the number of digits in the number num Choice 4 - double intPower (double x, int n) to compute x^n, where n is a positive integer and x is a double precision variable Choice 5-void printSubstr (String str) that prints all substrings of the input string.arrow_forwardProblem 1: Create a Java class RecursiveMethods.java and create the following methods inside: ALL THE METHODS NEED TO BE COMPLETED RECURSIVLY. NO LOOPS ALLOWED. oddEvenMatch Rec: the method takes an integer array as a parameter and returns a boolean. The method returns true if every odd index contains an odd integer AND every even index contains an even integer(0 is even). Otherwise it returns false. sumNRec: The method takes an integer array A and returns the sum of all integers in the parameter array. nDownToOne: Takes an integer n and prints out the numbers from n down to 1, each number on its own line. inputAndPrintReverse: Inputs integers from the user until the user enters 0, then prints the integers in reverse order. For this method, you may NOT use an array or any type of array structure, in other words, you may not use any structure to store the user input. After completing the methods, use the main method to test them. You can hard code the tests.arrow_forward
- Assignment 5B: Keeping Score. Now that we know about arrays, we can keep track of when events occur during our program. To prove this, let's create a game of Rock-Paper-Scissors. For those not familiar with this game, the basic premise is that each player says "Rock-Paper- Scissors!" and then makes their hand into the shape of one of the three objects. Winners are determined based on the following rules: Rock beats Scissors Scissors beats Paper Paper beats Rock At the start of the program, it will ask the player how many rounds of Rock-Paper- Scissors they want to play. After this, the game will loop for that many number of times. Each loop, it will ask the player what item they want to use – Rock, Paper, or Scissors. The computer will randomly generate its own item, and a winner will be determined. The game will then save the result as an element of an array, and the next round will begin. Once all the rounds have been played, the program will say "Game Over" and display a list of who…arrow_forwardPersonal project Q5. This question is concerned with the design and analysis of recursive algorithms. You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence A of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive algorithm. No marks will be given if your answer uses loops. FindAverageAndProduct(a1, ...., an) such that n > 1 Input: A sequence of real values A = (a1, ...., an) Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A. Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables. (a) Produce a pseudo code design for a recursive algorithm to solve this problem. (b) Draw a call-stack…arrow_forwardI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
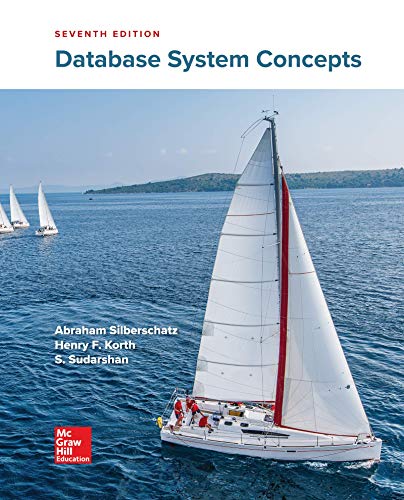
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
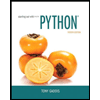
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
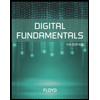
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
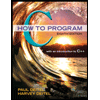
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
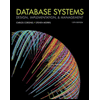
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
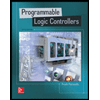
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education