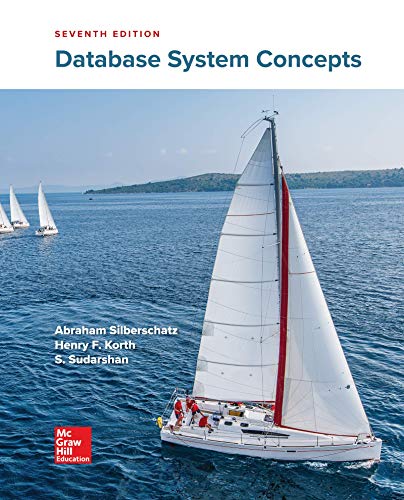
Concept explainers
Personal project Q5.
This question is concerned with the design and analysis of recursive algorithms.
You are given a problem statement as shown below. This problem is concerned with performing calculations on a sequence ? of real numbers. Whilst this could be done using a conventional loop-based approach, your answer must be developed using a recursive
FindAverageAndProduct(a1, ...., an) such that n > 1
Input: A sequence of real values A = (a1, ..., an)
Output:, A 2-tuple (average, product) containing the average (average) of all the values and the product (product) of all the values of the elements in A.
Your recursive algorithm should use a single recursive structure to find the average and product values, and should not use two separate instances of a recursive design. You should not employ any global variables.
(a) Produce a pseudo code design for a recursive algorithm to solve this problem.
(b) Draw a call-stack diagram to show the application of your recursive
algorithm when called using the sequence = (24, 8, −4, 6, −6, 3).
(c) Write down the set of recurrence equations for your recursive algorithm. Remember that one of the equations should correspond to the recursive algorithm base case.
(d) Using the recurrence equations you gave in your answer for part (c), determine the running time complexity of your recursive algorithm.
Personal project Q8.
A piece of code implementing a recursive algorithm has been produced, and a student has analysed the recurrences. They have produced the recurrence equations as shown below:
?(?) = ?(? − 3) + 2(? − 3) + ?1 ?(3) = ?2
So the recursive algorithm features a base case when the size of the problem is ? = 3. The values of ?1 and ?2 are constants. You should assume the initial value of ? (the size of the problem) is divisible by 3.
Determine the running time complexity of this recursive algorithm. To get the full marks, your analysis should be as complete as possible. You can verify your analysis by modelling the recurrence equations in a program like Excel or MATLAB. Your answer must include:
(a) Evidence of at least two cycles of substitutions to establish the running time function ?(?).
(b) A clear statement of the generalisation of that pattern to ? iterations of the recursive step.
(c) A statement of the number of iterations required to solve a problem of size ?.
(d) A statement of the final overall running time complexity that follows from your previous algebra.
You may find it useful to know that the formula for a sum of an arithmetic sequence of numbers of the form (1,2,3, ... . ?) is given by the formula: - attached the photo
∑m=1 m=k m = ?(k+1) /2
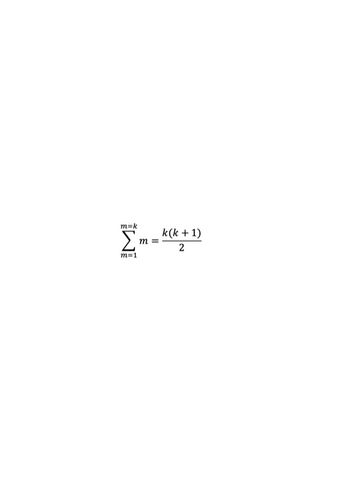

Step by stepSolved in 5 steps with 8 images

- Ex. 01 : Recursion About So far, we have learned that we can perform repetitive tasks using loops. However, another way is by creating methods that call themselves. This programming technique is called recursion and, a method that calls itself within it's own body is called a recursive method. One use of recursion is to perform repetitive tasks instead of using loops, since some problems seem to be solved more naturally with recursion than with loops. To solve a problem using recursion, it is broken down into sub-problems. Each sub-problem is similar to the original problem, but smaller in size. You can apply the same approach to each sub-problem to solve it recursively. All recursive methods use conditional tests to either 1. stop or 2. continue the recursion. Each recursive method has the following characteristics: 1. end/terminating case: One or more end cases to stop the recursion. 2. recursive case: reduces the problem in to smaller sub-problems, until it reaches (becomes) the end…arrow_forwardNeed help with this python recursive basic pathfinding question. I have the layout of the code but I need help filling in the parts that say "pass" and I need the output of the code to match the sample run below. Our goal is to find a path (not the best path, but just any path) from A to Z. You see that the green path is not the shortest but it does let us navigate from start to finish. (The picture below) First, we need to know A and Z, our starting and ending points. We'll pass these into our function. I'm going to use a dictionary to represent this graph. Each node (vertex, circle) will have a name, in this case "A" and "Z" were the names of the nodes, but in the generated maps I'm going to use "Node 1", "Node 2", "Node 3", etc. Here is an example web_map web_map = { 'Node 1': ['Node 3', 'Node 2'], 'Node 2': ['Node 1', 'Node 4'], 'Node 3': ['Node 1'], 'Node 4': ['Node 2'] } Node 1 is connected to 2 and 3 for instance, and then also note that Node 3 is connected back to…arrow_forwardC++arrow_forward
- Please help me make a a t square fractal using recursion. if you can please also make a sierpenski triangle ( and comment it). You do not have to comment the first task.arrow_forwardPython Using recursion only No loops Note that in a correct solution the isdigit method or in operator will never be applied to the entire string s. The function must return and not print the resulting string. def getDigits(s):arrow_forwardWrite and test a Java/Python recursive method for finding the minimum element in an array, A, of n elements. What the running time? Hint: an array of size 1 would be the stop condition. The implementation is similar to linearSum method in lecture 5 examples. You can use the Math.min method for finding the minimum of two numbers.arrow_forward
- T/F 3. Iteratively traversing a labyrinth is much faster than recursively traversing one.arrow_forwardT/F 11. A recursion will still be replaced by an iteration and the other way around.arrow_forwardJava source code writing - a recursive algorithm. Please use non-recursive and recursive ways to compute the nth Harmonic number, defined as H. Turn in your java source code file with three methods, including one main() method.arrow_forward
- discrete matharrow_forwardThe Tower of Hanoi is a puzzle where n disks of different sizes arestacked in ascending order on one rod and there are two other rods with nodisks on them. The objective is to move all disks from the first rod to thethird, such that:- only one disk is moved at a time- a larger disk can never be placed on top of a smaller oneWrite a recursive function that outputs the sequence of steps needed tosolve the puzzle with n disks.Write a test program in C++ that allows the user to input number of disks andthen uses your function to output the steps needed to solve the puzzle.Hint: If you could move up n−1 of the disks from the first post to thethird post using the second post as a spare, the last disk could be moved fromthe first post to the second post. Then by using the same technique you canmove the n−1 disks from the third post to the second post, using the firstdisk as a spare. There! You have the puzzle solved. You only have to decidewhat the nonrecursive case is, what the recursive…arrow_forwarddef height(words, word): The length of a word is easy enough to define by tallying up its characters. Taking the road less traveled, we define the height of the given word with a recursive rule for the height of the given word to follow from the heights of two words whose concatenation it is. First, any character string that is not one of the actual words automatically has zero height. Second, an actual word that cannot be broken into a concatenation of two nonempty actual words has the height of one. Otherwise, the height of an actual word equals one plus the larger of the heights of the two actual words whose combined concatenation it can be expressed as. To make these heights unambiguous for words that can be split into two non-empty subwords in multiple ways, this splitting is done the best way that produces the tallest final height. Since the list of words is known to be sorted, you can use binary search (available as the function bisect_left in the bisect module) to quickly…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
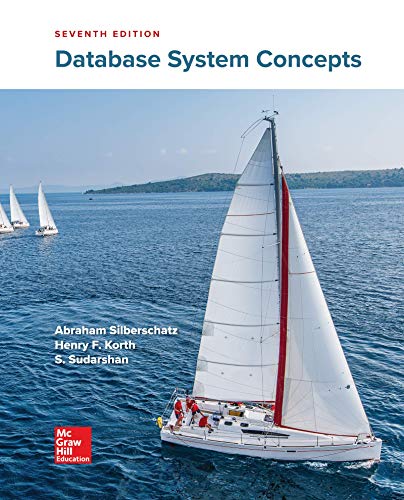
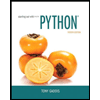
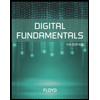
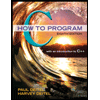
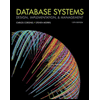
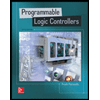