Exercise 11
.docx
keyboard_arrow_up
School
Old Dominion University *
*We aren’t endorsed by this school
Course
150
Subject
Computer Science
Date
Apr 3, 2024
Type
docx
Pages
7
Uploaded by GeneralPigeon2812
Upgrading the Library Management System
This tutorial will guide you through upgrading our Library Management System to utilize a nested dictionary instead of the list.
The primary advantage of using dictionaries in this example is their ability to store more complex, structured data, enabling us to keep track not only of book titles but also of how many copies are available, who has borrowed them, and more.
#Our old Book Repository was a list:
books = []
Now, let's modify the system to use a nested dictionary. This will allow us to store each book's title along with the number of copies available, the number of copies loaned out, and a list of borrowers. Step1:
### Initializing the Book Repository with Nested Dictionaries
We'll transition from a list to a dictionary named `books`. Each key in this dictionary is a book title, and the value is a nested dictionary that tracks the number of copies (`copies`), the number of copies currently
loaned out (`loaned`), and a list of borrowers (`loaned_to`). Your task is to initialize this dictionary with the given book titles and their corresponding information.
Your task is to initialize the `books` dictionary with the provided book entries, following the structure described above.
Define a variable `books` as a dictionary to store the book inventory.
Each key in the `books` dictionary is a string representing a book title.
The value corresponding to each key is another dictionary with the following keys and values:
A key `copies` with an integer value indicating the total number of copies of the book.
A key `loaned` with an integer value starting at `0`, representing the number of copies currently loaned out.
A key `loaned_to` with an empty list value `[]`, which will hold the names of the individuals who have borrowed copies of the book.
Step2:
### Modifying the Functions to Work with Dictionaries. Here is a Summary of the Required Tasks
1. Code the `add_book(title, copies)` function to manage new additions or updates to the inventory.
2. Implement `loan_out(title, borrower_name)` 3. Code the `return_book(title, borrower_name)` to handle the return process.
4. Code the `display_inventory()` to show the current state of all books in the library.
5. Develop `display_loan_history()` to review which users have borrowed books.
6. Code the `search_for_book(query)` to find and display information on specific books.
7. Implement `most_popular_books()` to find which books are favorites among users (Extra Credit).
1. Adding Books:
Our previous `add_book` function simply added a title to the list. We'll modify it to update our dictionary.
1.
Define a function `add_book` that takes two parameters: `title` (a string) and `copies` (an integer)
.
2. Check if the `title` already exists in the `books` dictionary.
- If it does, increment the `copies` value for that book title by the additional number of `copies` provided.
- If it does not, add a new entry to the `books` dictionary with the `title` as the key and a new dictionary as the value. The nested dictionary should include `copies` (set to the number provided), `loaned` (set to 0), and `loaned_to` (set to an empty list).
3. Print a confirmation message with the title and the number of copies added.
2. Loaning Out a book:
The `loan_out` function in the old system, checked the loaned_books_count not to exceed 5 and updated this number when the loan was successful as shown below:
In our new code, since the dictionary stores the number of copies and the names of the individuals who have borrowed copies of the book, The function needs to record the borrower's name and updates the
loan count for the book.
Note: there is no need to have a global variable “loaned_books_count” in this version of the code we will just check and update each book’s loan count without having an upper limit for loaning books (for now).
# Updated loan_out function for nested dictionaries here is the code please add your modification:
*Notice how we need the borrower_name as input for the function to update the list
* The new condition will not only check that the book is in the repository (check if the “title” is in the dictionary), it will also check if there are available copies to loan 3. Return Book:
The `return_book` function will reverse the actions of `loan_out`, updating the loaned count and removing the borrower from the list:
**what will the new condition check?
4. Display Inventory:
A function to display the current state of the inventory, which means display the entries of the books dictionary. It provides the total number of copies and how many are currently loaned out.
1. Define a function `display_inventory` with no parameters.
2. Loop through the `books` dictionary using a `for` loop that retrieves both the key (book title) and the value (book info).
3. For each book, print the title along with the number of `copies` and the number of copies `loaned` from
the book info.
5. Display Loan History:
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
def delete task (..., ...):
I
param: task_collection (list) holds all tasks;
param:
an integer ID indexes each task (stored as a dictionary)
(str) - a string that is supposed to represent
an integer ID that indexes the task in the list
returns:
0 if the collection is empty.
-1 - if the provided parameter is not a string or if it is not a string
that contains a valid integer >=0 representing the task's position on the list.
Otherwise, returns the item (dict) that was removed from the
provided collection.
11 11 11
arrow_forward
As we in general, try to solve the problem first; we start with a brute force, simple, algorithm: design it below
# write your implementation here
def maxProfitBrute(changes):
it returns the indices of (i,j) indicating the day to buy and sell respectively
to have the maximum profit in a list of prices per day in .
Inputs:
- changes: the list holding the changes in prices; the value whose index is k represents
the change between day and day
has at least a single change [two days]
Output:
- i: the index of the change before which we buy
j: the index of the change after which we sell
- maxProfit: the value of the maximum profit
Example:
changes = [1, 2]
- that means the price started with ;
day 1: it became
- day 2: it became
In that case: (i,j) = (0,1) as we should buy at the first day, and sell after the third day
# return the values
return (0,0,0)
# Try vour alaorithm
arrow_forward
please solution Python
language using list
2. Create a program that would save
employee information (Employee ID,
Name, Job Title, and Salary). Then,
print employee names with their
salary and job title. Also, get the
average salary for all employees.
arrow_forward
Topic: Database
On my bookshelf of paper books, I have a dictionary that consists of multiple volumes, each of which is a book. One covers A-E, another F-J, another K-M, and so on. To find a word, I look at the alphabetic ranges to find the right volume. Then I use the ranges at the top of each page to turn to the proper page. Then I find the word on the page. Pick the correct answer.
A. Multi-level Indexes
B. Secondary indexes
C. None the above
arrow_forward
By python
arrow_forward
Implement a function grandparents (person, family) with the following specification.
Input: A string containing a person's name, person, and a family tree database family as specified above.
Output: A list containing only the names of the grandparents of person that are stored in the database, or
an empty list if no such information exists in the database (including if the person themselves are not in
the database).
For example, grandparents should behave as follows:
>>> duck_tree = [['Donald Duck','Quackmore Duck', 'Hortense McDuck'],
['Della Duck', 'Quackmore Duck', 'Hortense McDuck'],
['Hortense McDuck', 'Fergus McDuck', 'Downy ODrake'],
['Scrooge McDuck', 'Fergus McDuck', 'Downy ODrake'],
['Fergus McDuck', 'Dingus McDuck', 'Molly Mallard'],
['Huey Duck', None, 'Della Duck'],
['Dewey Duck', None, 'Della Duck'],
['Louie Duck', None, 'Della Duck']]
>>> sorted (grandparents ('Scrooge McDuck', duck_tree))
['Dingus McDuck', 'Molly Mallard']
>>> sorted (grandparents ('Louie Duck',…
arrow_forward
A task in this lab is a dictionary object that is guaranteed to have key-value pairs that follow certain constraints:
Every task must have a "name" key, with the value being a string of the task's name
Every task must have a "description" field, with the value being a string of the task's description
Every task must have a "priority" field, with the value being a number 1-5, representing the task's priority
1 stands for "Lowest" (priority)
2 stands for "Low" (priority)
3 stands for "Medium" (priority)
4 stands for "High" (priority)
5 stands for "Highest" (priority)
Every task must have a "deadline" field, with the value being a task's deadline represented as a valid date-string in slashed-format (ex. "02/05/2022")
Note: we are using the US format for strings: <MM>/<DD>/<YEAR>. 01/02/2022 represents January 2nd, 2022.
Every task must have a "completed" field, with the value being a Boolean value representing whether a task is completed or not
Here is an…
arrow_forward
Python
arrow_forward
the concept of iterators and how they are better than using a regular loop to process the items in a collection.
arrow_forward
Do this using C++ language using structures and singly linked list.
arrow_forward
FIX THE PROGRAM BELOW.
#Function to get the key value ind dictionary
def get_key(val):
for key, value in result.items():
if val == value:
return key
return "key doesn't exist"
#Function definition to find the tallest height
def tall(list_height):
return max(list_height)
#Function to find the shortest height
def short(list_height):
return min(list_height)
# creating an empty list list_names
list_names = []
# Get the number of baby names as input from user
num1 = int(input("Enter number of baby names : "))
# iterating till the range
for i in range(0, num1):
name = input()
list_names.append(name) # adding the element
print("list of baby names : ",list_names)
# creating an empty list list_height
list_height = []
# iterating till the range
for i in range(0, num1):
height = int(input())
list_height.append(height) # adding the element
print("list of baby heights : ", list_height)
# using…
arrow_forward
JavaScript Practice Question
arrow_forward
A data structure called a deque is closely related to a queue. Deque is an acronym meaning "double-ended queue." With a deque, you may insert, remove, or view from either end of the queue, which distinguishes it from the other two. Utilise arrays to implement a deque
arrow_forward
hi I really need help with this assignment problem
flip_matrix(mat:list)->list
You will be given a single parameter a 2D list (A list with lists within it) this will look like a 2D matrix when printed out, see examples below. Your job is to flip the matrix on its horizontal axis. In other words, flip the matrix horizontally so that the bottom is at top and the top is at the bottom. Return the flipped matrix.
To print the matrix to the console:
print('\n'.join([''.join(['{:4}'.format(item) for item in row]) for row in mat]))
Example:
Matrix:W R I T XH D R L GL K F M VG I S T CW N M N FExpected:W N M N FG I S T CL K F M VH D R L GW R I T X
Matrix:L CS PExpected:S PL C
Matrix:A D JA Q HJ C IExpected:J C IA Q HA D J
arrow_forward
Iterating over a dictionary example: Gradebook statistics.
Write a program that uses the keys(), values(), and/or items() dict methods to find statistics about the student_grades dictionary. Find the following:
Print the name and grade percentage of the student with the highest total of points.
Find the average score of each assignment.
Find and apply a curve to each student's total score, such that the best student has 100% of the total points.
The language is in Python.
# student_grades contains scores (out of 100) for 5 assignmentsstudent_grades = {'Andrew': [56, 79, 90, 22, 50],'Nisreen': [88, 62, 68, 75, 78],'Alan': [95, 88, 92, 85, 85],'Chang': [76, 88, 85, 82, 90],'Tricia': [99, 92, 95, 89, 99]}
So far I have the first part of the question:
for student, scores in student_grades.items(): print(student, sum(scores)/len(scores))
arrow_forward
Please answer the question in image.
Please don't add anything extra
Thank you.
arrow_forward
def get_task(task_collection, ...):
"""
param: task_collection (list) - holds all tasks;
maps an integer ID/index to each task object (a dictionary)
param: ... (str) - a string that is supposed to represent
an integer ID that corresponds to a valid index in the
collection
returns:
0 - if the collection is empty.
-1 - if the provided parameter is not a string or if it is not
a valid integer
Otherwise, convert the provided string to an integer;
returns None if the ID is not a valid index in the list
or returns the existing item (dict) that was requested.
"""
arrow_forward
What are the fundamental similarities and differences between arrays and lists? Please include examples.
arrow_forward
Overview
This program will have you cut and past a dictionary called food_dict from the specifications, the programmatically go through the list to find people who have
not yet listed their favorite foods. It will then ask for their foods, update the dictionary, and finally print it at the end.
Note: I will change the dictionary during grading
Expected Output
Example 1
What is Janelle's favorite food? Steak
What is Thomas's favorite food?
What is Yolanda's favorite food? Soup
Here are the favorite foods:
Jim's favorite food is Tacos
Bob's favorite food is Burgers
Janelle's favorite food is Steak
Lisa's favorite food is Pizza
Thomas's favorite food is Pho
Yolanda's favorite food is Soup
Finn's favorite food is Bread
Specifications
• You should submit a single file called M4A5.py
• It should follow the submission standards outlined here: Submission Standards
• Your program must loop through the dictionary to find empty values.
• You must copy this dictionary definition to the top of your…
arrow_forward
When an author produces an index for his or her book, the first step in this process is to decide which words should go into the index; the second is to produce a list of the pages where each word occurs. Instead of trying to choose words out of our heads, we decided to let the computer produce a list of all the unique words used in the manuscript and their frequency of occurrence. We could then go over the list and choose which words to put into the index.
The main object in this problem is a "word" with associated frequency. The tentative definition of "word" here is a string of alphanumeric characters between markers where markers are white space and all punctuation marks; anything non-alphanumeric stops the reading. If we skip all un-allowed characters before getting the string, we should have exactly what we want. Ignoring words of fewer than three letters will remove from consideration such as "a", "is", "to", "do", and "by" that do not belong in an index.
In this project, you…
arrow_forward
When an author produces an index for his or her book, the first step in this process is to decide which words should go into the index; the second is to produce a list of the pages where each word occurs. Instead of trying to choose words out of our heads, we decided to let the computer produce a list of all the unique words used in the manuscript and their frequency of occurrence. We could then go over the list and choose which words to put into the index.
The main object in this problem is a "word" with associated frequency. The tentative definition of "word" here is a string of alphanumeric characters between markers where markers are white space and all punctuation marks; anything non-alphanumeric stops the reading. If we skip all un-allowed characters before getting the string, we should have exactly what we want. Ignoring words of fewer than three letters will remove from consideration such as "a", "is", "to", "do", and "by" that do not belong in an index.
In this project, you…
arrow_forward
Program - Python
Make a dictionary, the student's name is the key (a string) and the student's test scores is the value (a list). So it should be a dictionary with six key value pairs in it. Here is data to use.
Brian,94,89,92
Rachel,100,90,65
Jon,67.5,95,100
Brit,0,78,80
Greg,65,100,78
Andrea,55.5,67,79
Now print the dictionary data using a loop that starts with
for key, value in.
arrow_forward
Java , Data structures
arrow_forward
Programming Language C Note:No Need for Detailed Explanation. The Answer is Enough For Me.
arrow_forward
Please Explain your work and this should be in "C Language" and this is a data structure subject
Thank you!
arrow_forward
DART PROGRAMMING LANGUAGE Q1
Create database of songs:
a. Choose one type of collection
b. Initialize with data
c. Implement functions:
i. find by index,
ii. find by title
iii. find by artist
d. Remove item
e. Add item
arrow_forward
Simple login system
PYTHON
Create a static dictionary with a number of users and with the following values:
First name
Last name
Email address
Password
Ask the user for:
5. Email address 6. Password
Loop (for()) through the dictionary and if (if()) the user is found print the following:
7. Hello, first name last name you have successfully logged in 8. Notify the user if the password and email address are wrong 9. Additional challenge: if you want the program to keep asking for a username and password when the combination is wrong, you will need a while() loop.
arrow_forward
Suppose a list contains marks earned in the courses CSE110, PHY111, and MAT110 of each student consecutively in a nested list form. Your task is to take a course name as input and sort the list based on the marks obtained in that course to finally print the names of the students in descending order of marks obtained i.e. from the student who earned the highest marks to the student who earned the lowest.
For example, the list may look like
stu_lst = [ ["Alan", 95, 87, 91], ["Turing", 92, 90, 83], ["Elon", 87, 92, 80], ["Musk", 85, 94, 90] ]
where for each nested list, 1st index holds the name of the student, 2nd index is total marks earned in the CSE110 course, 3rd index is PHY111 marks and 4th index is MAT110 marks.
[You cannot use python build-in sort() function, you can call your own sort function or copy your code from previous tasks instead]
===============================================================
Sample Input 1
MAT110
Sample Output 1
['Alan', 'Musk', 'Turing', 'Elon']…
arrow_forward
Suppose a list contains marks earned in the courses CSE110, PHY111, and MAT110 of each student consecutively in a nested list form. Your task is to take a course name as input and sort the list based on the marks obtained in that course to finally print the names of the students in descending order of marks obtained i.e. from the student who earned the highest marks to the student who earned the lowest.
For example, the list may look like
stu_lst = [ ["Alan", 95, 87, 91], ["Turing", 92, 90, 83], ["Elon", 87, 92, 80], ["Musk", 85, 94, 90] ]
where for each nested list, 1st index holds the name of the student, 2nd index is total marks earned in the CSE110 course, 3rd index is PHY111 marks and 4th index is MAT110 marks.
[You cannot use python build-in sort() function, you can call your own sort function or copy your code from previous tasks instead]
===============================================================
Sample Input 1
MAT110
Sample Output 1
['Alan', 'Musk', 'Turing', 'Elon']…
arrow_forward
Question in image.
Please explain how to do the Question with the answer.
Thank you
arrow_forward
Simple Student Management System Build the following app using Python Tkinter library.
Using this app, user can
• add student information
• view all students
• search student(s) by name
• remove student by id
Add
• First name, last name, date of birth cannot be blank while adding a specific student information.
• However major can be blank, which gets set to "Undefined" if left out blank.
• Student Id is automatically generated by the program (ex: 1001, 1002, 1003, 1004 ….)
If the user keeps a mandatory field blank (ex: First name) and presses "Add Student" button, it displays the following message box: first name is required If the user successfully enters all the necessary information and presses the "Add Student" button, it displays the following confirmation message box: student added View All When the user presses the "View All" button, it displays all the student’s information (Id, First name, Last name, Date of birth, and Major) in a nice formatted way. Example below: • Must…
arrow_forward
Question 4
For this question, let's combine ADTS and dictionaries! Let's try to make an ADT that represents a politician. The politician
ADT has a constructor make_politician. There are three selectors, get_name, get_party, and get_age. When
implementing an ADT, you have the freedom to choose how you want to represent it. Some common data structures used to
represent ADTs are lists and dictionaries. The city ADT was implemented using a list. Now, let's use dictionaries to
implement our politician ADT. You must use a dictionary for this question, or else you will not pass the tests.
arrow_forward
Coding language: C++. (Try to avoid linked lists if possible please). Add comments. Thanks.
• Each functionality component must be implemented as a separate function, though the function does not need to be declared and defined separately
• No global variables are allowed
• No separate.hor.hpp are allowed
• You may not use any of the hash tables, or hashing functions provided by the STL or Boost library to implement your hash table
• Appropriate, informative messages must be provided for prompts and outputs
You must implement a hash table using the Double Hashing collision strategy and the mid-square base 10 hashing function with an R of 2. Your collision strategy must be implemented as a separate function, though it may be implemented inside your insert/search/delete functions, and should halt an insert / search/delete functions, and should halt an insert/search/delete after table size number of collisions. Your hash function must be implemented as a separate function. Your hash table…
arrow_forward
*Data Structures and Algorithm
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
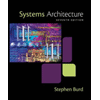
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Related Questions
- def delete task (..., ...): I param: task_collection (list) holds all tasks; param: an integer ID indexes each task (stored as a dictionary) (str) - a string that is supposed to represent an integer ID that indexes the task in the list returns: 0 if the collection is empty. -1 - if the provided parameter is not a string or if it is not a string that contains a valid integer >=0 representing the task's position on the list. Otherwise, returns the item (dict) that was removed from the provided collection. 11 11 11arrow_forwardAs we in general, try to solve the problem first; we start with a brute force, simple, algorithm: design it below # write your implementation here def maxProfitBrute(changes): it returns the indices of (i,j) indicating the day to buy and sell respectively to have the maximum profit in a list of prices per day in . Inputs: - changes: the list holding the changes in prices; the value whose index is k represents the change between day and day has at least a single change [two days] Output: - i: the index of the change before which we buy j: the index of the change after which we sell - maxProfit: the value of the maximum profit Example: changes = [1, 2] - that means the price started with ; day 1: it became - day 2: it became In that case: (i,j) = (0,1) as we should buy at the first day, and sell after the third day # return the values return (0,0,0) # Try vour alaorithmarrow_forwardplease solution Python language using list 2. Create a program that would save employee information (Employee ID, Name, Job Title, and Salary). Then, print employee names with their salary and job title. Also, get the average salary for all employees.arrow_forward
- Topic: Database On my bookshelf of paper books, I have a dictionary that consists of multiple volumes, each of which is a book. One covers A-E, another F-J, another K-M, and so on. To find a word, I look at the alphabetic ranges to find the right volume. Then I use the ranges at the top of each page to turn to the proper page. Then I find the word on the page. Pick the correct answer. A. Multi-level Indexes B. Secondary indexes C. None the abovearrow_forwardBy pythonarrow_forwardImplement a function grandparents (person, family) with the following specification. Input: A string containing a person's name, person, and a family tree database family as specified above. Output: A list containing only the names of the grandparents of person that are stored in the database, or an empty list if no such information exists in the database (including if the person themselves are not in the database). For example, grandparents should behave as follows: >>> duck_tree = [['Donald Duck','Quackmore Duck', 'Hortense McDuck'], ['Della Duck', 'Quackmore Duck', 'Hortense McDuck'], ['Hortense McDuck', 'Fergus McDuck', 'Downy ODrake'], ['Scrooge McDuck', 'Fergus McDuck', 'Downy ODrake'], ['Fergus McDuck', 'Dingus McDuck', 'Molly Mallard'], ['Huey Duck', None, 'Della Duck'], ['Dewey Duck', None, 'Della Duck'], ['Louie Duck', None, 'Della Duck']] >>> sorted (grandparents ('Scrooge McDuck', duck_tree)) ['Dingus McDuck', 'Molly Mallard'] >>> sorted (grandparents ('Louie Duck',…arrow_forward
- A task in this lab is a dictionary object that is guaranteed to have key-value pairs that follow certain constraints: Every task must have a "name" key, with the value being a string of the task's name Every task must have a "description" field, with the value being a string of the task's description Every task must have a "priority" field, with the value being a number 1-5, representing the task's priority 1 stands for "Lowest" (priority) 2 stands for "Low" (priority) 3 stands for "Medium" (priority) 4 stands for "High" (priority) 5 stands for "Highest" (priority) Every task must have a "deadline" field, with the value being a task's deadline represented as a valid date-string in slashed-format (ex. "02/05/2022") Note: we are using the US format for strings: <MM>/<DD>/<YEAR>. 01/02/2022 represents January 2nd, 2022. Every task must have a "completed" field, with the value being a Boolean value representing whether a task is completed or not Here is an…arrow_forwardPythonarrow_forwardthe concept of iterators and how they are better than using a regular loop to process the items in a collection.arrow_forward
- Do this using C++ language using structures and singly linked list.arrow_forwardFIX THE PROGRAM BELOW. #Function to get the key value ind dictionary def get_key(val): for key, value in result.items(): if val == value: return key return "key doesn't exist" #Function definition to find the tallest height def tall(list_height): return max(list_height) #Function to find the shortest height def short(list_height): return min(list_height) # creating an empty list list_names list_names = [] # Get the number of baby names as input from user num1 = int(input("Enter number of baby names : ")) # iterating till the range for i in range(0, num1): name = input() list_names.append(name) # adding the element print("list of baby names : ",list_names) # creating an empty list list_height list_height = [] # iterating till the range for i in range(0, num1): height = int(input()) list_height.append(height) # adding the element print("list of baby heights : ", list_height) # using…arrow_forwardJavaScript Practice Questionarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
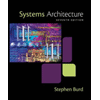
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning