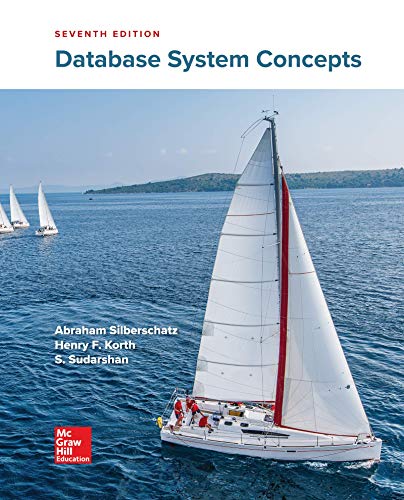
(my question and explanation is in one of the images)
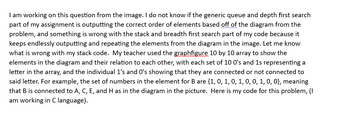
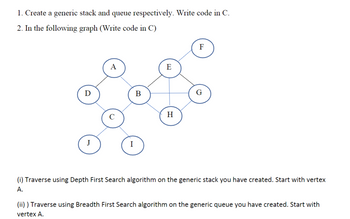

The scope of the question is to provide a complete explanation and implementation of generic stack and queue data structures in the C programming language. The question asks for code examples, explanations, and a step-by-step breakdown of how to create these data structures using linked lists for flexibility. It also covers demonstrating the usage of these data structures by pushing and popping elements in the stack and enqueuing and dequeuing elements in the queue, including different data types.
The question is specifically focused on the following aspects:
- Implementing a generic stack data structure in C.
2. Implementing a generic queue data structure in C.
3. Providing code examples for creating and using these data structures.
4. Offering a step-by-step explanation of the code.
Generic Stack:
- A stack is a data structure that follows the last-in-first-out (LIFO) principle, meaning the most recently added element is the first to be removed.
- A generic stack allows elements of any data type to be pushed onto the stack and popped off the stack without the need to specify the data type in advance.
- Generic stacks are useful for a wide range of applications, such as managing function call states, parsing expressions, and reversing sequences of data.
Generic Queue:
- A queue is a data structure that follows the first-in-first-out (FIFO) principle, meaning the first element added is the first to be removed.
- A generic queue allows elements of any data type to be enqueued (added to the back of the queue) and dequeued (removed from the front of the queue) without requiring prior knowledge of the data type.
- Generic queues are commonly used in scenarios like task scheduling, order processing, and managing data flows where the order of processing is critical.
Both generic stack and queue data structures are highly versatile and enable programmers to work with various data types in a consistent and reusable way, simplifying code and making it adaptable to different use cases. This flexibility is particularly valuable in programming languages that support generics or templates, as it allows developers to create highly adaptable and reusable code.
Note:
"Since you have posted a multiple questions , we will provide the solution only to the first question as per our Q&A guidelines. Please repost the remaining questions separately."
Step by stepSolved in 6 steps

- Data Structure & Algorithm: Describe and show by fully java coded example how a hash table works. You should test this with various array sizes and run it several times and record the execution times. At least four array sizes are recommended. 10, 15, 20, 25. It is recommended you write the classes and then demonstrate/test them using another classarrow_forwardUse stack concepts Q #2 Ô https://www.loc-cs.org/~chu/DataStructu Essay Questions (20% each)- continue [0] [1] [2] [3] myList "Bashful" "Awful" Jumpy "Happy The above "myList" is an ArrayList in Java program. 2. If I want to remove "Awful" from myList, which of the following actions should I take first? a. Move "Happy" to the previous element first. b. Move "Jumpy" to the previous element first. Describe the reason of your choice. Next Pagearrow_forward#include <iostream>#include <list>#include <string>#include <cstdlib>using namespace std;int main(){int myints[] = {};list<int> l, l1 (myints, myints + sizeof(myints) / sizeof(int));list<int>::iterator it;int choice, item;while (1){cout<<"\n---------------------"<<endl;cout<<"***List Implementation***"<<endl;cout<<"\n---------------------"<<endl;cout<<"1.Insert Element at the Front"<<endl;cout<<"2.Insert Element at the End"<<endl;cout<<"3.Front Element of List"<<endl;cout<<"4.Last Element of the List"<<endl;cout<<"5.Size of the List"<<endl;cout<<"6.Display Forward List"<<endl;cout<<"7.Exit"<<endl;cout<<"Enter your Choice: ";cin>>choice;switch(choice){case 1:cout<<"Enter value to be inserted at the front: ";cin>>item;l.push_front(item);break;case 2:cout<<"Enter value to be inserted at the end:…arrow_forward
- #include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forward#include #include using namespace std; void PrintSize(vector numsList) { cout intList (3); PrintSize(intList); cin >> currval; while (currval >= 0) { } Type the program's output intList.push_back(currval); cin >> currVal; PrintSize(intList); intList.clear(); PrintSize(intList); return 0; 1 se mice with camScanner Check Next Input 1234-1 Outputarrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
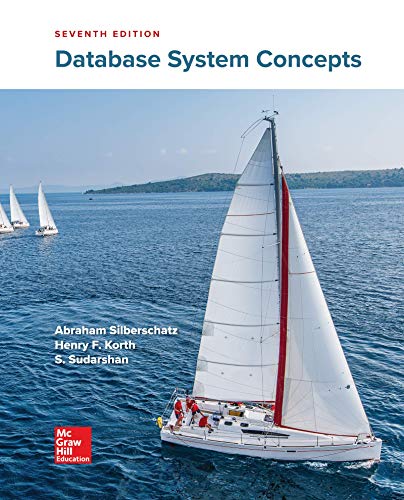
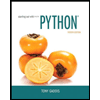
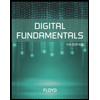
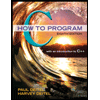
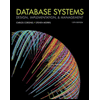
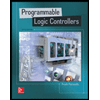