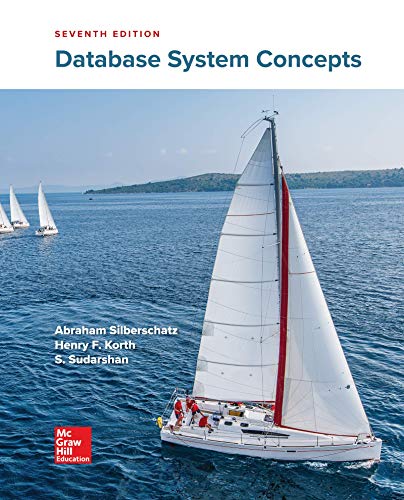
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
thumb_up100%
PLEASE USE C++
HERE IS THE CODE I HAVE SO FAR :
#include <iostream>
using namespace std;
int Fibonacci(int n) {
/* Type your code here. */
}
int main() {
int startNum;
cin >> startNum;
cout << "Fibonacci(" << startNum << ") is " << Fibonacci(startNum) << endl;
return 0;
}
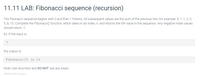
Transcribed Image Text:11.11 LAB: Fibonacci sequence (recursion)
The Fibonacci sequence begins with O and then 1 follows. All subsequent values are the sum of the previous two, for example: 0, 1, 1, 2, 3,
5, 8, 13. Complete the Fibonacci() function, which takes in an index, n, and returns the nth value in the sequence. Any negative index values
should return -1.
Ex: If the input is:
7
the output is:
Fibonacci (7) is 13
Note: Use recursion and DO NOT use any loops.
385056.2528316.qx3zqy7
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- #include #include using namespace std; //function prototypesint countLetters(char*); int countDigits(char*); int countWhiteSpace(char*); int main() { int numLetters, numDigits, numWhiteSpace; char inputString[51]; cout <<"Enter a string of no more than 50 characters: " << endl << endl; cin.getline(inputString,51); numLetters = countLetters(inputString); numDigits = countDigits(inputString); numWhiteSpace = countWhiteSpace(inputString); cout << "The number of letters in the entered string is " << numLetters << endl; cout << "The number of digits in the entered string is " << numDigits << endl; cout << "The number of white spaces in the entered string is " << numWhiteSpace << endl; return 0; } int countLetters(char *strPtr) { int occurs = 0; while(*strPtr != '\0') // loop is executed as long as// the pointer strPtr does not point // to the null character which// marks the end of the string // isalpha determines…arrow_forwardLook at the following C++ code and comment each line about how it works with pointer. int i = 33; double d = 12.88; int * iPtr = &i; double * dPtr = &d; // iPtr = &d; // dPtr = &i; // iPtr = i; // int j = 99; iPtr = &j; //arrow_forward//I need help debugging the C code below the image is what I was following for directions while doing the code// #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for (int i = 0; i < 9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct…arrow_forward
- Question 1 is already done need help with the others though This is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to…arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forwardCreate a flowchart for this program in c++, #include <iostream>#include <vector> // for vectors#include <algorithm>#include <cmath> // math for function like pow ,sin, log#include <numeric>using std::vector;using namespace std;int main(){ vector <float> x, y;//vector x for x and y for y float x_tmp = -2.5; // initial value of x float my_function(float x); while (x_tmp <= 2.5) // the last value of x { x.push_back(x_tmp); y.push_back(my_function(x_tmp)); // calculate function's value for given x x_tmp += 1;// add step } cout << "my name's khaled , my variant is 21 ," << " my function is y = 0.05 * x^3 + 6sin(3x) + 4 " << endl; cout << "x\t"; for (auto x_tmp1 : x) cout << '\t' << x_tmp1;//printing x values with tops cout << endl; cout << "y\t"; for (auto y_tmp1 : y) cout << '\t' << y_tmp1;//printing y values with tops…arrow_forward
- #include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> T func(T a) { cout<<a; return a; } template<class U> void func(U a) { cout<<a; } int main(int argc, char const *argv[]) { int a = 5; int b = func(a); return 0; } Give output for this code.arrow_forwardC++ 6.34 #include <iostream>#include <cstdlib>#include <ctime>using namespace std; void guessGame(); // function prototypebool isCorrect( int, int ); // function prototype int main(){srand( time( 0 ) ); // seed random number generatorguessGame();} // end main // guessGame generates numbers between 1 and 1000 and checks user's guessvoid guessGame(){int answer; // randomly generated numberint guess; // user's guesschar response; // 'y' or 'n' response to continue game // loop until user types 'n' to quit gamedo {// generate random number between 1 and 1000// 1 is shift, 1000 is scaling factoranswer = 1 + rand() % 1000; // prompt for guesscout << "I have a number between 1 and 1000.\n" << "Can you guess my number?\n" << "Please type your first guess." << endl << "? ";cin >> guess; // loop until correct numberwhile ( !isCorrect( guess, answer ) ) cin >> guess; // prompt for another gamecout << "\nExcellent! You guessed the…arrow_forwardQuestion 1 is already done need help with the others though This is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to…arrow_forward
- Please answer in C++arrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
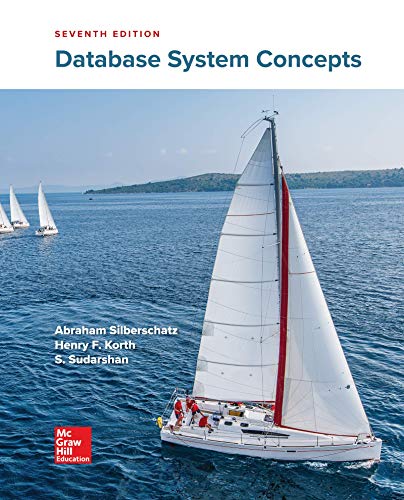
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
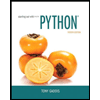
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
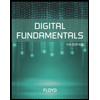
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
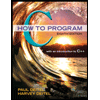
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
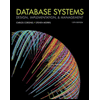
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
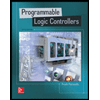
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education