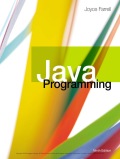
Concept explainers
JAVA Programming Problem 2 - Cycle
[A] Create a class called “Cycle” which has two instance integer variables as properties, “numberOfWheels” and “weight.” Create a constructor with two parameters, using the same variable names in the parameter list. Assign each variable to numberOfWheels” and “weight” respectively. Write a separate application to test the class and display its properties. Note: Do not change the names of the instance variables or the variables listed in the constructor’s parameter list.
[B] Edit your class Cycle by adding a default constructor which will assign the default values of 100 to represent the numberOfWheels, and 1000 to represent the weight, by invoking a call to the other constructor. Modify your application created in [A] to test the class.
Directions
[A] Create a class called Cycle
- Declare integer instance variables numberOfWheels and weight as private.
- Include a toString() method in the Cycle class. No set or get methods are included.
- Create a constructor with two parameters, using the same variable names numberOfWheels and weight in the parameter list. Complete the constructor as necessary.
- Create a separate application to test the class.
- Create an object of the Cycle class.
- Display the properties of the object.
[B] Add a default constructor
- Edit the default constructor such that the default constructor will invoke the existing constructor with the default values of 100 to represent the numberOfWheels, and 1000 to represent the weight. Invoke the constructor using the “this” reference.
- Create the second constructor which will receive the two arguments.
- Create a separate application to test the class.
- Create an object of the Cycle class.
- Display the properties of the object.

Trending nowThis is a popular solution!
Step by stepSolved in 7 steps with 2 images

Programming Problem 3 – CycleFileInput
Revisit the Cycle class in Module 3. Modify your application such that the properties will be read from a text file called “Cycle.txt”.
Directions
Examine your application for the class called Cycle.
- Add an appropriate throws statement in the main method.
- Create a reference to a File class with the appropriate name of a text file (Cycle.txt). Note: Cycle.txt was created in the previous assignment, CycleFileOutput.
- In your code, check that the text file does exist.
- Input the values from the file to memory.
- Close the file.
Programming Problem 1 – CycleThrowTryCatch
Revisit the Cycle class in Module 3. Modify your application such that the properties, numberOfWheels and weightare entered as double values interactively (at the keyboard). Exception handling will be used to determine whether a type mismatch occurs.
Edit your application such that, in addition to [A], the values for numberOfWheels and weight, entered interactively, will throw a new exception “Values cannot be less than or equal to zero” only If the values are less than or equal to zero. Add or use the appropriate try and/or catch blocks.
Directions
Examine your application for the class called Cycle.
- Add Try and Catch blocks appropriately.
- Add the throw statement for the new exception.
- Display an appropriate message if an exception occurs.
- Display the properties of the object.
Programming Problem 3 – CycleFileInput
Revisit the Cycle class in Module 3. Modify your application such that the properties will be read from a text file called “Cycle.txt”.
Directions
Examine your application for the class called Cycle.
- Add an appropriate throws statement in the main method.
- Create a reference to a File class with the appropriate name of a text file (Cycle.txt). Note: Cycle.txt was created in the previous assignment, CycleFileOutput.
- In your code, check that the text file does exist.
- Input the values from the file to memory.
- Close the file.
Programming Problem 1 – CycleThrowTryCatch
Revisit the Cycle class in Module 3. Modify your application such that the properties, numberOfWheels and weightare entered as double values interactively (at the keyboard). Exception handling will be used to determine whether a type mismatch occurs.
Edit your application such that, in addition to [A], the values for numberOfWheels and weight, entered interactively, will throw a new exception “Values cannot be less than or equal to zero” only If the values are less than or equal to zero. Add or use the appropriate try and/or catch blocks.
Directions
Examine your application for the class called Cycle.
- Add Try and Catch blocks appropriately.
- Add the throw statement for the new exception.
- Display an appropriate message if an exception occurs.
- Display the properties of the object.
- Create an application named CarDemo that declares at least two Car objects and demonstrates how they can be incremented using an overloaded ++ operator. Create a Car class that contains a model and a value for miles per gallon. Include two overloaded constructors. One accepts parameters for the model and miles per gallon; the other accepts a model and sets the miles per gallon to 20. Overload a ++ operator that increases the miles per gallon value by 1. The CarDemo application creates at least one Car using each constructor and displays the Car values both before and after incrementation.arrow_forwardAssume that you have created a class named MyClass. The header of the MyClass constructor can be ____________. public MyClass() public MyClass (double d) Either of these can be the constructor header. Neither of these can be the constructor header.arrow_forwardUsing classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType (as defined in Example 10-10, Chapter 10), the class dateType (as designed in this chapters Programming Exercise 2), and the class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information. Use constructors to automatically initialize the member variables. Define the class addressBookType using the previously defined classes. An object of the type addressBookType should be able to process a maximum of 500 entries. The program should perform the following operations: Load the data into the address book from a disk. Sort the address book by last name. Search for a person by last name. Print the address, phone number, and date of birth (if it exists) of a given person. Print the names of the people whose birthdays are in a given month. Print the names of all the people between two last names. Depending on the users request, print the names of all family members, friends, or business associates.arrow_forward
- In previous chapters, you have created programs for the Greenville Idol competition. Now create a Contestant class with the following characteristics: The Contestant class contains public static arrays that hold talent codes and descriptions. Recall that the talent categories are Singing Dancing, Musical instrument, and Other. The class contains an auto-implemented property that holds a contestants name. The class contains fields for a talent code and description. The set accessor for the code assigns a code only if it is valid. Otherwise, it assigns I for Invalid. The talent description is a read-only property that is assigned a value when the code is set. Modify the GreenvilleRevenue program so that it uses the Contestant class and performs the following tasks: The program prompts the user for the number of contestants in this years competition; the number must be between 0 and 30. The program continues to prompt the user until a valid value is entered. The expected revenue is calculated and displayed. The revenue is $25 per contestant. The program prompts the user for names and talent codes for each contestant entered. Along with the prompt for a talent code, display a list of the valid categories. After data entry is complete, the program displays the valid talent categories and then continuously prompts the user for talent codes and displays the names of all contestants in the category. Appropriate messages are displayed if the entered code is not a character or a valid code.arrow_forwardWrite a program named DemoJobs for Harolds Home Services. The program should instantiate several Job objects and demonstrate their methods. The Job class contains four data fields—description (for example, wash windows), time in hours to complete (for example, 3.5), per-hour rate charged (for example, $25.00), and total fee (hourly rate times hours). Include properties to get and set each field except the total fee—that field will be read-only, and its value is calculated each time either the hourly fee or the number of hours is set. Overload the + operator so that two Jobs can be added. The sum of two Jobs is a new Job containing the descriptions of both original Jobs (joined by and), the sum of the time in hours for the original Jobs, and the average of the hourly rate for the original Jobs. Harold has realized that his method for computing the fee for combined jobs is not fair. For example, consider the following: His fee for painting a house is $100 per hour. If a job takes 10 hours, he earns $1000. His fee for dog walking is $10 per hour. If a job takes 1 hour, he earns $10. If he combines the two jobs and works a total of 11 hours, he earns only the average rate of $55 per hour, or $605. Devise an improved, weighted method for calculating Harolds fees for combined Jobs and include it in the overloaded operator+() method. Write a program named DemoJobs2 that demonstrates all the methods in the class work correctly.arrow_forwardA Private variable in a class can be accessed directly by a Public method in the same class. a. True b. Falsearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
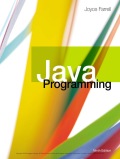
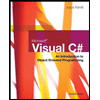
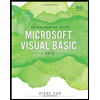
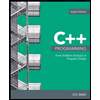
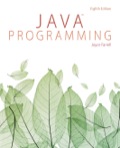