// Add to this partially built code. // fill in code where there is a TODO #include<iostream> using namespace std; int main() { cout.setf(ios::fixed); cout.setf(ios::showpoint); // show decimals even if not needed cout.precision(2); // two places to the right of the decimal // TODO: enter the missing types below int time; float ticketPrice; destination; //'C'=Chicago, 'P'=Portland, 'M'=Miami typeOfDay; //'D'=weekDay 'E'=weekEnd cout << "Welcome to Airlines!" << endl; cout << "What is your destination? ([C]hicago, [M]iami, [P]ortland) "; cin >> destination; cout << "What time will you travel? (Enter time between 0-2359) "; cin >> time; // TODO: set isDayTime to true if time 5AM or later, but before 7PM cout << "What type of day are you traveling? (week[E]nd or week[D]ay) "; cin >> typeOfDay; // TODO: set isWeekend to true if typeOfDay is 'E', otherwise false // Depending upon the destination, and whether it is weekend, day/night // set the appropriate price // I recommend using a switch // I am providing much of the Input and Output dialog to simplify this program cout << "Each ticket will cost: $" << ticketPrice << endl; int numTickets; cout << "How many tickets do you want? "; cin >> numTickets; // TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it cout << "You owe: $" << totalCost << endl; // TODO: declare a variable that will hold the user's payment // prompt the user for "Amount paid? " // read in the user's Payment // TODO: calculate the change (a float) // TODO: If the user's payment is too little, then print this message: cout << "That is too little! No tickets ordered."; // otherwise print these 2 lines: cout << "You will get in change: $" << change << endl; cout << "Your tickets have been ordered!"; return 0; }
// Add to this partially built code.
// fill in code where there is a TODO
#include<iostream>
using namespace std;
int main()
{
cout.setf(ios::fixed);
cout.setf(ios::showpoint); // show decimals even if not needed
cout.precision(2); // two places to the right of the decimal
// TODO: enter the missing types below
int time;
float ticketPrice;
destination; //'C'=Chicago, 'P'=Portland, 'M'=Miami
typeOfDay; //'D'=weekDay 'E'=weekEnd
cout << "Welcome to Airlines!" << endl;
cout << "What is your destination? ([C]hicago, [M]iami, [P]ortland) ";
cin >> destination;
cout << "What time will you travel? (Enter time between 0-2359) ";
cin >> time;
// TODO: set isDayTime to true if time 5AM or later, but before 7PM
cout << "What type of day are you traveling? (week[E]nd or week[D]ay) ";
cin >> typeOfDay;
// TODO: set isWeekend to true if typeOfDay is 'E', otherwise false
// Depending upon the destination, and whether it is weekend, day/night
// set the appropriate price
// I recommend using a switch
// I am providing much of the Input and Output dialog to simplify this program
cout << "Each ticket will cost: $" << ticketPrice << endl;
int numTickets;
cout << "How many tickets do you want? ";
cin >> numTickets;
// TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it
cout << "You owe: $" << totalCost << endl;
// TODO: declare a variable that will hold the user's payment
// prompt the user for "Amount paid? "
// read in the user's Payment
// TODO: calculate the change (a float)
// TODO: If the user's payment is too little, then print this message:
cout << "That is too little! No tickets ordered.";
// otherwise print these 2 lines:
cout << "You will get in change: $" << change << endl;
cout << "Your tickets have been ordered!";
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

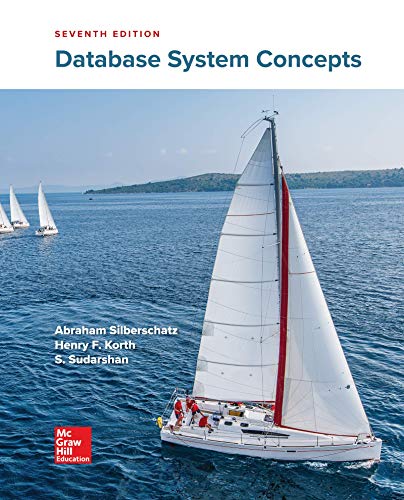
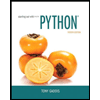
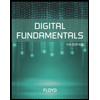
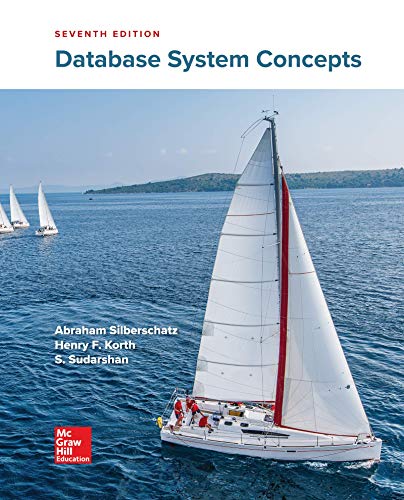
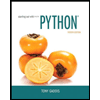
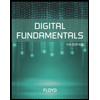
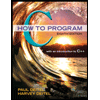
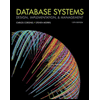
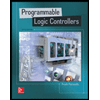