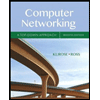
Complete the PoundDog code by adding a constructor having a constructor initializer list that initializes age with 1, id with -1, and name with "NoName". Notice that MyString's default constructor does not get called.
Note: If you instead create a traditional default constructor as below, MyString's default constructor will be called, which prints output and thus causes this activity's test to fail. Try it!
// A wrong solution to this activity... PoundDog::PoundDog() { age = 1; id = -1; name.SetString("NoName"); } |
Here is the code:
#include <iostream>
#include <string>
using namespace std;
class MyString {
public:
MyString();
MyString(string s);
string GetString() const { return str; };
void SetString(string s) { str = s; };
private:
string str;
};
MyString::MyString() {
cout << "MyString default constructor called" << endl;
str = "";
}
MyString::MyString(string s): str(s) {
}
class PoundDog {
public:
PoundDog();
void Print() const;
private:
int age;
int id;
MyString name;
};
/*PRINT YOUR CODE HERE*/
void PoundDog::Print() const {
cout << "age: " << age << endl;
cout << "id: " << id << endl;
cout << "name: " << name.GetString() << endl;
}
int main() {
PoundDog currDog;
currDog.Print();
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 5 images

- This is for python class, so please answer in python 9.12 LAB: Product class In main.py define the Product class that will manage product inventory. Product class has three attributes: a product code, the product's price, and the number count of product in inventory. Implement the following methods: A constructor with 3 parameters that sets all 3 attributes to the value in the 3 parameters set_code(self, code) - set the product code (i.e. SKU234) to parameter code get_code(self) - return the product code set_price(self, price) - set the price to parameter price get_price(self) - return the price set_count(self, count) - set the number of items in inventory to parameter count get_count(self) - return the count add_inventory(self, amt) - increase inventory by parameter amt sell_inventory(self, amt) - decrease inventory by parameter amtarrow_forwardHelp, I am making a elevator simulation using polymorphism. I am not sure to do now. Any help would be appreciated. My code is at the bottom, that's all I could think off. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. public abstract class Passenger { public static int passangerCounter = 0; private String passengerID; protected…arrow_forwardCan each class be done please I am not sure how to do each one. In java Make a program with classes named Vehicle, LandVehicle, AirVehicle, Car, Truck, Glider, Balloon, VehicleFactoryMain, MyFileManager. The Vehicle Inheritance looks like this: Note: Vehicle should also have a range. Make sure to override the toString() method for returning a String that includes all the information about an instance of a vehicle. VehicleFactoryMain will use these classes to make a random number and assortment of vehicle types. For example: Car - red, 526Km,1524Kg, 1988Kg, 1001Kg, gas, 4-wheels, 2-doors, fastback. Balloon - orange, 234Km,320Kg, 423Kg, 256Kg, gas, 950m, hot air. For the various ranges, altitudes, and weights set a max and min value and randomly choose between those. For values that have types (hot-air, hydrogen, helium or gas, electric, diesel, kerosene, methane,none) make a static array in the class and randomly choose one of the values. Have the factory select a random number…arrow_forward
- For this assignment you will be building on the Fraction class you began last week. All the requirements from that class are still in force. You'll be making five major changes to the class. Delete your set() function. Add two constructors, a default constructor (a constructor that takes no parameters) and a parameterized constructor (a constructor that takes parameters). The default constructor assigns the value 0 to the Fraction. In the parameterized constructor, the first parameter will represent the initial numerator of the Fraction, and the second parameter will represent the initial denominator of the Fraction.Since Fractions cannot have denominators of 0, the default constructor should assign 0 to the numerator and 1 to the denominator. Also, the parameterized constructor should check to make sure that the second parameter is not a 0 by using the statement "assert(denominatorParameter != 0);". To use the assert() function you'll also need to #include <cassert>. (Note, I…arrow_forwardIf you fly in economy class, then you can be promoted to businessclass, especially if you have a gold airline card for privateflights. If you do not have a gold card, you can "reset" fromflight if the plane is full or you are late for check-in. All thesethe conditions are shown in the diagram below. Note that all statements(operators) are numbered. You run 3 tests:Test 1 - Gold card holder upgraded to business classTest 2 - Passenger without gold card remains in economy classTest 3 - Passenger who was "thrown" off the flightWhat is the statement coverage of these three tests?A. 60%B. 70%C. 80%D. 90%arrow_forwardWould you mind help me write racer class, sensor class, and timestamp class? (Note: The sensor class can have functions to determine if two sensors are adjacent and calculate the speed between them, while the time stamp class can have functions to calculate the time elapsed between two time stamps.) The class of racer should include : 1. Private data: racer number (int), an unknown number of sensor data. Sensor data includes sensor number (int that starts at 0 for start time; the last sensor is finish line), mile marker for the sensor double) and time stamp for each sensor (when racer passes the sensor) with hour, minute, second and millisecond. (You may want instance variables that holds racer's total race time, total race distance, total race speed.) 2. Default constructor 3. Copy constructor: make sure the code copies any vectors contained in the class 4. Set and get functions for private dat. 5. "get" function that computes and returns the racer's total time to complete…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
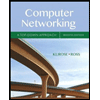
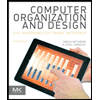
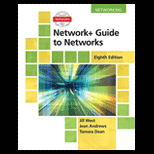
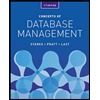
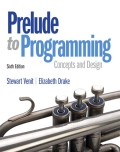
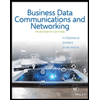