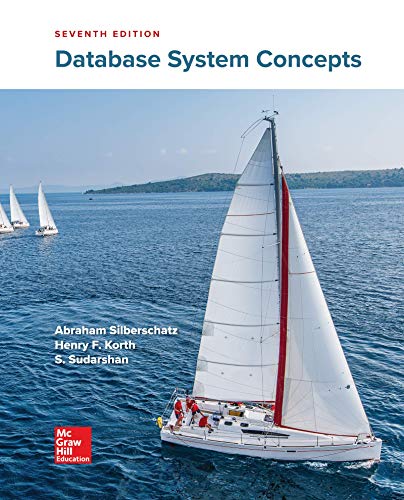
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
-
-
import java.util.Arrays;import java.util.Scanner;
public class Game {private Player[] players;private Board board;private int currentPlayerIndex;private int numOfPlayers;
private Scanner scanner = new Scanner(System.in);// constructorpublic Game(){//create a new board;this.board = new Board();//setup game;this.setupGame();//start game;this.play();}//Game Setuppublic void setupGame(){
System.out.println("How many players would you like to included in this game? (2-4)");numOfPlayers = scanner.nextInt();while (numOfPlayers < 2 || numOfPlayers > 4) {System.out.println("Invalid input. Please enter a number between 2 and 4.");numOfPlayers = scanner.nextInt();}scanner.nextLine();String[] playerNames = new String[numOfPlayers];this.players = new Player[numOfPlayers];
for (int i = 0; i < numOfPlayers; i++) {System.out.println("Enter player " + (i + 1) + " name:");String newPlayerName = scanner.nextLine();//Makes sure players don't have the same namewhile (Arrays.asList(playerNames).contains(newPlayerName)) {System.out.println("Player names cannot be the same. Please enter a different name.");System.out.print("Enter player " + (i + 1) + " name: ");newPlayerName = scanner.nextLine();}playerNames[i] = newPlayerName;// adds player to arrayplayers[i] = new Player(newPlayerName, Integer.toString(i + 1));}
//setup boardthis.board.boardSetUp();//set starting player to a random indexthis.currentPlayerIndex = (int) Math.floor(Math.random() * numOfPlayers);}//Print winnerprivate void printWinner(Player player){System.out.println("------------------------------------------------");System.out.println(" | Player " +player.getPlayerNumber()+" which is "+player.getPlayerName() +" wins | ");System.out.println("------------------------------------------------");}private void playerTurn(Player currentPlayer){System.out.println("\n---------Player " + currentPlayer.getPlayerNumber() +" turn -----\n");// so to be within the bounds of the array.int move = currentPlayer.makeMove() - 1;//to check if the moveif(this.board.columnFull(move)) {System.out.println("Column is full.");}//To make sure our column numbers are always validif(move< 0 || move >= this.board.getColumns()){System.out.println("Invalid column number.");}//update board to add tokenthis.board.addToken(move, currentPlayer.getPlayerNumber());}
}private void play() {while(!this.board.boardFull()){Player currentPlayer = players[currentPlayerIndex];//print boardthis.board.printBoard();//to make a movethis.playerTurn(currentPlayer);//catch blockSystem.out.println("Something went wrong adding a token to the playerTurn function: " + e);;//if a player wins print the current playerif(this.board.checkIfPlayerIsWinner(currentPlayer.getPlayerNumber())) {this.board.printBoard();this.printWinner(currentPlayer);return; // ends game when a player wins;}//alternate current playersetPlayerIndex();}System.out.println("Board is full!");}//to set current player Indexprivate void setPlayerIndex() {this.currentPlayerIndex = (this.currentPlayerIndex + 1) % numOfPlayers;}
}
import java.util.Scanner;
public class Player {//initial variablesprivate String playerName;private String playerNumber;private static Scanner scanner = new Scanner(System.in);//constructor for playerpublic Player(String playerName, String playerNumber) {this.playerName = playerName;this.playerNumber = playerNumber;}
public String getPlayerName() {return this.playerName;}
public String getPlayerNumber() {return this.playerNumber;}public int makeMove() {System.out.println("Enter the coloumn num to put your token");return scanner.nextInt();}public String toString(){return "Player " +playerNumber+ " is " + playerName;}}
-
![public void play()- this method is called to start the game play() method is called to start the game.
• initializes a boolean variable nowinner to true.
• calls the setupGame () method to get the board and player ready for the game.
• initializes an integer variable currentPlayerIndex to 0.
• enters a while loop that continues as long as nowinner is true.
• Inside the while loop, it checks if the board is full using the boardFull() method of the board object.
• If the board is full, it prints a message saying "Board is now full. Game Ends." and returns.
• If the board is not full, it assigns the current player to the players array at the currentPlayerIndex position.
• It then prints a message saying "It is player [player number]'s turn. [player name]"
• It then calls the playerTurn (currentPlayer) method, passing the current player as an argument.
• It then checks if the current player is the winner using the checkIfPlayer Is Thewinner (currentPlayer.getPlayer Number()) method of the
board object.
• If the current player is the winner, it calls the printwinner (currentPlayer) method, passing the current player as an argument.
• It then sets the nowinner variable to false, ending the while loop.
• If the current player is not the winner, it increments the currentPlayer Index variable by 1, and takes the modulus of that value by the
length of the players array. This ensures that the index stays within the range](https://content.bartleby.com/qna-images/question/a3cab9c1-3149-4232-8d34-9f213defe2a3/2b4a085c-62f5-49bb-b5ea-917236fe7b0c/tlll9r6_thumbnail.jpeg)
Transcribed Image Text:public void play()- this method is called to start the game play() method is called to start the game.
• initializes a boolean variable nowinner to true.
• calls the setupGame () method to get the board and player ready for the game.
• initializes an integer variable currentPlayerIndex to 0.
• enters a while loop that continues as long as nowinner is true.
• Inside the while loop, it checks if the board is full using the boardFull() method of the board object.
• If the board is full, it prints a message saying "Board is now full. Game Ends." and returns.
• If the board is not full, it assigns the current player to the players array at the currentPlayerIndex position.
• It then prints a message saying "It is player [player number]'s turn. [player name]"
• It then calls the playerTurn (currentPlayer) method, passing the current player as an argument.
• It then checks if the current player is the winner using the checkIfPlayer Is Thewinner (currentPlayer.getPlayer Number()) method of the
board object.
• If the current player is the winner, it calls the printwinner (currentPlayer) method, passing the current player as an argument.
• It then sets the nowinner variable to false, ending the while loop.
• If the current player is not the winner, it increments the currentPlayer Index variable by 1, and takes the modulus of that value by the
length of the players array. This ensures that the index stays within the range
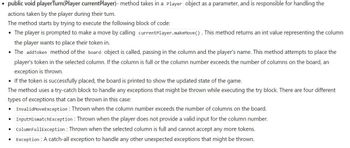
Transcribed Image Text:public void playerTurn (Player currentPlayer)- method takes in a Player object as a parameter, and is responsible for handling the
actions taken by the player during their turn.
The method starts by trying to execute the following block of code:
• The player is prompted to make a move by calling currentPlayer.makeMove (). This method returns an int value representing the column
the player wants to place their token in.
• The addToken method of the board object is called, passing in the column and the player's name. This method attempts to place the
player's token in the selected column. If the column is full or the column number exceeds the number of columns on the board, an
exception is thrown.
• If the token is successfully placed, the board is printed to show the updated state of the game.
The method uses a try-catch block to handle any exceptions that might be thrown while executing the try block. There are four different
types of exceptions that can be thrown in this case:
InvalidMoveException: Thrown when the column number exceeds the number of columns on the board.
Input MismatchException: Thrown when the player does not provide a valid input for the column number.
Column FullException: Thrown when the selected column is full and cannot accept any more tokens.
Exception: A catch-all exception to handle any other unexpected exceptions that might be thrown.
●
●
●
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java Programming Please do not change anything in Student class or Course class class John_Smith extends Student{ public John_Smith() { setFirstName("John"); setLastName("Smith"); setEmail("jsmith@jaguar.tamu.edu"); setGender("Male"); setPhoneNumber("(200)00-0000"); setJNumber("J0101459"); } class MyCourse extends Course { public MyCourse { class Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String…arrow_forwardWrite a class named IceCreamCup that implements the Customizable interface. The class should have the following private instance variables. Do NOT give any method implementations for this question, but only declare the instance variables. Do NOT initialize the instance variables -- except nCups below. A String variable name describing the ice cream name • A List of Flavor s added to the cup. Name it flist Additionally, define the following static variable: • An int nCups describing the total number of ice cream cups instantiated. Initialize this variablearrow_forwardpublic class Artwork { // TODO: Declare private fields - title, yearCreated // TODO: Declare private field artist of type Artist // TODO: Define default constructor // TODO: Define get methods: getTitle(), getYearCreated() // TODO: Define second constructor to initialize // private fields (title, yearCreated, artist) // TODO: Define printInfo() method // Call the printInfo() method in Artist.java to print an artist's information }} public class ArtworkLabel { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userTitle, userArtistName; int yearCreated, userBirthYear, userDeathYear; userArtistName = scnr.nextLine(); userBirthYear = scnr.nextInt(); scnr.nextLine(); userDeathYear = scnr.nextInt(); scnr.nextLine(); userTitle = scnr.nextLine(); yearCreated =…arrow_forward
- public class VisitorPackage{// Declare data fields: an int named travelerID, // a String named destination, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of destination to N/A, and choose an // appropriate value for the other data fields,// Your code here... // Implement the overloaded constructor that passes new// values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method applyDiscount.//// This method applies a discount off of the total cost// of the tour. The way the discount is applied depends// on the kind of visitor.//// Child discount: the unit discount is subtracted ONCE// from the total cost of the tour.// Senior discount: the unit discount is subtracted TWICE// from the total cost of the tour.// Parameters:// - a char to indicate the kind of visitor// ('c' for child, 's' for senior)// - a double that indicates the amount of the…arrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardThis is a subclass and doesn't has a main method yet. class Vector{Object items[];int length;int size; void Grow(){// Duplicate sizesize = size * 2; // Allocate new itemsObject new_items[] = new Object[size]; // Copy old itemsfor (int i = 0; i < length; i++)new_items[i] = items[i]; // Discard old itemsitems = new_items; // MessageSystem.out.println("Growing capacity to " + size + " elements");} public Vector(){size = 2;items = new Object[2];} public void Print(){// MessageSystem.out.println("Content:"); // Traversefor (int i = 0; i < length; i++)System.out.println(items[i]);} public void Insert(int index, Object item){// Check indexif (index < 0 || index > length){System.out.println("Invalid index");return;} // Grow if necessaryif (length == size)Grow(); // Shiftfor (int i = length - 1; i >= index; i--)items[i + 1] = items[i]; // Insertitems[index] = item; // One more itemlength++; // MessageSystem.out.println("Inserted " + item);} public void Add(Object…arrow_forward
- Subclass toString should call include super.toString(); re-submit these codes. public class Vehicle { private String registrationNumber; private String ownerName; private double price; private int yearManufactured; Vehicle [] myVehicles = new Vehicle[100]; public Vehicle() { registrationNumber=""; ownerName=""; price=0.0; yearManufactured=0; } public Vehicle(String registrationNumber, String ownerName, double price, int yearManufactured) { this.registrationNumber=registrationNumber; this.ownerName=ownerName; this.price=price; this.yearManufactured=yearManufactured; } public String getRegistrationNumber() { return registrationNumber; } public String getOwnerName() { return ownerName; } public double getPrice() { return price; } public int getYearManufactured() { return yearManufactured; }…arrow_forwardUML for the following Code: public class ProductOrder { private int numBook = 0; private int numCD = 0; private int numDVD = 0; public ProductOrder(int numBook, int numCD, int numDVD){ this.numBook = numBook; this.numCD = numCD; this.numDVD = numDVD; } public int getBook(){ return numBook; } public void setBook(int numBook){ this.numBook = numBook; } public int getCD(){ return numCD; } public void setCD(int numCD){ this.numCD = numCD; } public int getDVD(){ return numDVD; } public void setDVD(int numDVD){ this.numDVD = numDVD; } public int getProductTotal(){ return getBook() + getCD() + getDVD(); } }arrow_forwardQuestion 6 Consider: class Bike { } private String color; public Bike() { } public Bike(String newColor) { color = newColor; } public Bike (Bike bike) { color = } bike.color; public String getColor() { } } return color; public void setColor(String newColor) { color = newColor; Bike bike1= new Bike(); = Bike bike2 new Bike("blue"); Bike bike3 = new Bike(bike2); bike2.setColor("green"); Bike bike4 = new Bike(bike2); Which of the following are correct? bike4.getColor() returns null bike4.getColor() return "blue" bike3.getColor() return "green" bike2.getColor() returns "blue" bike4.getColor() return "green" bike1.getColor() returns bike3.getColor() return null bike3.getColor() returns "blue" bike2.getColor() returns "green" bike1.getColor() returns nullarrow_forward
- class smart { public: void print() const; void set(int, int); int sum(); smart(); smart(int, int); private: int x; int y; int secret(); }; class superSmart: public smart { public: void print() const; void set(int, int, int); int manipulate(); superSmart(); superSmart(int, int, int); private: int z; }; Now answer the following questions: a. Which private members, if any, of smart are public members of superSmart? b. Which members, functions, and/or data of the class smart are directly accessible in class superSmart?arrow_forward// This class discounts prices by 10% public class DebugFour4 { public static void main(String args[]) { final double DISCOUNT_RATE = 0.90; int price = 100; double price2 = 100.00; tenPercentOff(price DISCOUNT_RATE); tenPercentOff(price2 DISCOUNT_RATE); } public static void tenPercentOff(int p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println("Ten percent off " + p); System.out.println(" New price is " + newPrice); } public static void tenPercentOff(double p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println"Ten percent off " + p); System.out.println(" New price is " + newprice); } }arrow_forwardJava script. class Chameleon { static colorChange(newColor) { this.newColor = newColor; return this.newColor; } constructor({ newColor = 'green' } = {}) { this.newColor = newColor; } } const freddie = new Chameleon({ newColor: 'purple' }); console.log(freddie.colorChange('orange'));.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
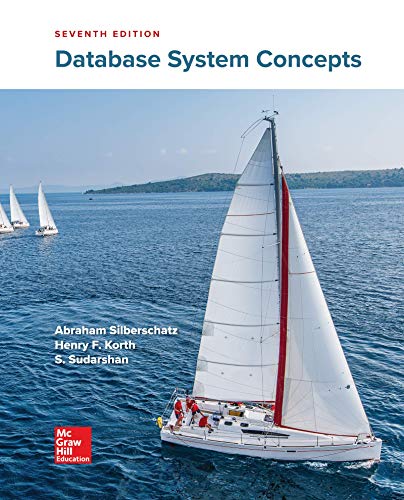
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
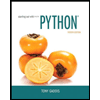
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
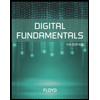
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
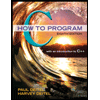
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
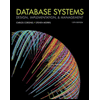
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
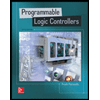
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education