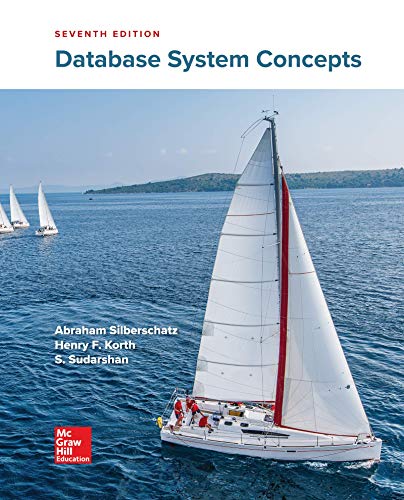
Create a python program for the following details: Create an abstract class Shape Under Shape create abstract methods name, sides, and color. Create subclasses of Shape with the name Square, Pentagon, and Triangle. Under each subclass, implement the methods name, sides, and color with the following details. Square: print "Square", "I have 4 sides", "My color is yellow" Pentagon: print "Pentagon", "I have 5 sides", "My color is blue" Triangle: print "Triangle", "I have 3 sides", "My color is red" Create an instance of each subclasses. Ask the user for an input. (Assume the user will input 3, 4, or 5 only) if the user input 3 - print the details of an object of subclass Triangle if the user input 4 - print the details of an object of subclass Square if the user input 5 - print the details of an object of subclass Pentagon SAMPLE INPUT 1. num SAMPLE OUTPUT NEEDED: Enter a number: 3 Triangle I have 3 sides My color is red

Step by stepSolved in 3 steps with 1 images

- It has just one Main class which tests abstract class Animal and its 3 subclasses: Dog, Cat, and Fish. It also tests the Talkers: Dog, Cat, and Radio. So your job is to write all 6 of these simple classes (they should be less than one page each) : Talker.java - the interface Talker, which has just one void method called speak() Animal.java - the abstract class Animal, which stores an animal's name. (No abstract methods). It should contain 2 methods: a constructor with 1 argument (the name) a method getName() which returns the name. Dog.java - the class Dog, which extends Animal and implements Talker. It should contain 3 methods: a constructor with no arguments, giving the dog a default name of "Fido" a constructor with 1 argument (the name) a speak() method that prints "Woof" on the screen. Use @Override Cat.java - the class Cat, which extends Animal and implements Talker. It should contain just 2 methods: a constructor with 1 argument (the name) (no default name like dogs…arrow_forwardwhat are the positives and negatives of the two designs attached. The Java code next to each design shows an example of how the constructors are implemented in the subclasses. Constructor code for the other two other subclasses is same as the Bipedal constructor in each design (except the number of legs value). designs attachedarrow_forwardPython:Please read the following. The Game class The Game class brings together an instance of the GameBoard class, a list of Player objects who are playing the game, and an integer variable “n” indicating how many tokens must be aligned to win (as in the name “Connect N”). The game class has a constructor and two additional methods. The most complicated of these, play, is provided for you and should not be modified. However, you must implement the constructor and the add_player method. __init__(self,n,height,width) The Game constructor needs to initialize three instance variables. The first, self.n, simply holds the integer value “n” provided as a parameter. The second, self.board, should be a new instance of the GameBoard class (described below), built using the provided height and width as dimensions. The third, self.players, should be an initially empty list. add_player(self,name,symbol) The add_player method is provided a name string and a symbol string. These two values should be…arrow_forward
- Using Java - Creating an Abstract Class Shape II Create an abstract class "Shape" with the following specifications: an abstract method "area()" of return type double an abstract method "perimeter()" of return type double. Put your code in a Java source file named "Shape.java." I. Create a class "Rectangle" that extends the Shape class with the following specifications: Attributes: width, length Constructor: Implement a parameterized constructor needed initialize the data. toString: Implement a "toString" method that prints out the area and perimeter. Implement methods to compute the area and perimeter. II. Create a class "Circle" that extends the Shape class with the following specifications: Attributes: radius Constructor: Implement a parameterized constructor to initialize the data. toString: Implement a "toString" method that prints out the area and perimeter. Implement methods to compute the area and perimeter. III. Create a class "Triangle" that extends the Shape class…arrow_forwardDefine and implement a class named animal that has the following constructor and attributes: animal(string n, int v) ; // creates an animal with name n and body volume v. string name ; int animalID ; int volume ; // animals are allocated a unique ID on creation // the animal's name // the animal's unique ID // the volume of the animal's body Following the principles of encapsulation and information hiding, set the proper access modifiers for the attributes and provide methods to access the object's data. You need to set the name and volume of an animal, as well as get the animal's name, animallD and volume. There must not be a set function for animallD. The get and set function names should be the attribute name prefixed with "get_" or "set_" respectively. For example, the get and set methods for volume would be named get_volume and set_volume respectively. Declare the get_name function as pure virtual. When selecting between the three types of access modifiers for each of the…arrow_forward1. Define a Class: Circle Circle - radius: double + Circle() +Circle(double r) +setradius(double r): void +getradius() : double +calculateArea(): double +calculateCircumference: double 2. Use a Circle instance (object) in a main() method Program.arrow_forward
- Create a Point class to hold x and y values for a point. Create methods show(), add() and subtract() to display the Point x and y values, and add and subtract point coordinates. Create another class Shape, which will form the basis of a set of shapes. The Shape class will contain default functions to calculate area and circumference of the shape, and provide the coordinates (Points) of a rectangle that encloses the shape (a bounding box). These will be overloaded by the derived classes; therefore, the default methods for Shape will only print a simple message to standard output. Create a display() function for Shape, which will display the name of the class and all stored information about the class (including area, circumference and bounding box). Build the hierarchy by creating the Shape classes Circle, Rectangle and Triangle. Search the Internet for the rules governing these shapes, if necessary. For these three Shape classes, create default constructors, as well as constructors…arrow_forwardUSING JAVA: Part A) Implement a superclass Appointment and subclasses Onetime, Daily, and Monthly. An Appointment has a description (for example, “See the dentist”) and a date. Write a method, occuresOn(int year, int month, int day) The check whether the appointment occurs on that date. For example, for appointment, you must check whether the day of the month matches. This fill an array of Appointment objects with a mixture of appointments. Have the user enter a date and print out all appointments that occur on that date. Part B) Improve the appointment book by giving the user the option to add new appointments. The user must specify the type of the appointment, the description, and the date.arrow_forwardWRITE A PYTHON PROGRAM (RECTANCLE.PY) THAT HAS A CLASS NAMED RECTANGLE. RECTANGLE CLASS HAS TWO ATTRIBUTES, A LENGTH AND WIDTH, (BOTH INT). YOUR CLASS SHOULD HAVE THE FOLLOWING METHODS: (1) _INIT._(SELF, LENCTH, WIDTH): THIS METHOD CALLED WHEN AN OBJECT IS CREATED FROM THE CLASS AND IT ALLOWS THE CLASS TO INITIALIZE THE ATTRIBUTES OF A CLASS. (2) RECTANGLE.AREA(SELF): THIS METHOD WILL COMPUTE THE AREA OF A RECTANGLE, THEN RETURN IT. TO FIND THE AREA OF A RECTANGLE, MULTIPLY THE LENGTH BY THE WIDTH. IN THE MAIN, YOU DO THE FOLLOWING: A. WHEN YOU START THE PROGRAM, PRINT "RECTANGLE CALCULATIONS USING CLASS" B. PROMPT THE USER FOR LENGTH AND WIDTH. C. CREATE ONE OBJECT OF THE RECTANGLE CLASS, REC1. D. PRINT THE AREA OF THE RECTANGLE BY CALLING RECTANGLE.AREA() HERE IS AN EXAMPLE RUN OF THE PROGRAM: RECTANGLE CALCULATIONS USING CLASS INPUT THE LENGTH: 5 INPUT THE WIDTH: 6 THE AREA OF THE RECTANGLE IS 30arrow_forward
- Java: According to this diagram, what are the methods that the StagBeetle class can use? (Select all that apply) Group of answer choices: A: hornSize B: fly() C: emitPheromone() D: eat()arrow_forwardJAVA. THIS IS A PROGRAMMING ASSIGNMENT NOT A WRITING ASSIGNMENT IF YOU ANSWERED BEFORE DO NOT ANSWER AGAIN PLEASE DO NOT PASTE A PAPER Design a class to store the following information about computer scientists⦁ Name⦁ Research area(s)⦁ Major contribution A scientist may have more than one area of research.⦁ Implement the usual setters and getters methods ⦁ Implement a method that takes a research area as an input and return thenames of scientists associated with that area ⦁ Implement a method that takes a word as an input and return the names ofscientists whose contribution mention that word Next, perform desktop research to find at least five prominent black computerscientists, with their research area(s) and major contribution, and store this data intoobjects of the class you designed. You can just hardcode this data. No user input needed.Define JUnit tests to check your research area and contribution search methods.arrow_forwardJava- Suppose that Vehicle is a class and Car is a new class that extends Vehicle. Write a description of which kind of assignments are permitted between Car and Vehicle variables.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
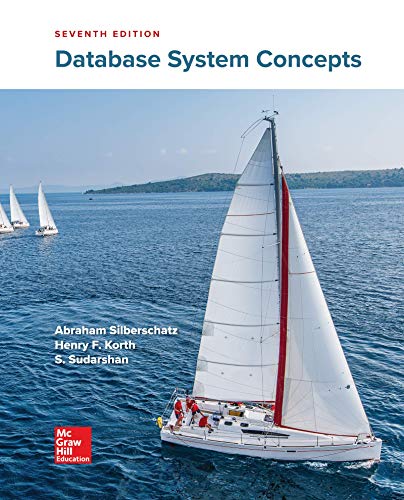
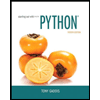
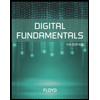
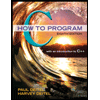
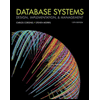
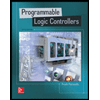