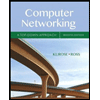
Execute program maxHeap.java and heapApplication.java(Attached Screenshot):
- Insert the nodes listed in
95
77
43
66
64
25
44
11
10
47
- Display the heap array and compare it with the array in
95
77
43
66
64
25
44
11
10
47
Max Heap
import java.util.*;
public class maxHeap
{
public int heapSize, capacity;
public int[] Arr;
public maxHeap(int cap)
{
heapSize = 0;
capacity = cap;
Arr = new int[cap];
}
public void heapify(int parent)
{
int Lson, Rson, largest, temp;
Lson = 2 * parent + 1;
Rson = 2 * parent + 2;
/* find the largest among A[parent], A[lson] and A[rson] */
if ( Lson <= heapSize - 1 && Arr[Lson] > Arr[parent] )
{
largest = Lson;
}
else
{
largest = parent;
}
if (Rson <= heapSize - 1 && Arr[Rson] > Arr[largest] )
{
largest = Rson;
}
if (largest != parent)
{
temp = Arr[parent];
Arr[parent] = Arr[largest];
Arr[largest] = temp;
heapify(largest);
}
}
public void buildHeap()
{
for (int i = (heapSize - 2) / 2; i >= 0; i--)
{
heapify(i);
}
}
public void displayHeap()
{
System.out.println("heapArray: "); // array format
for(int m=0; m<heapSize; m++)
if(Arr[m] != 0)
System.out.print( Arr[m] + " ");
else
System.out.print( "-- ");
System.out.println();
// heap format
int nBlanks = 32;
int itemsPerRow = 1;
int column = 0;
int j = 0; // current item
String dots = "...............................";
System.out.println(dots+dots); // dotted top line
while(heapSize > 0) // for each heap item
{
if(column == 0) // first item in row?
for(int k=0; k<nBlanks; k++) // preceding blanks
System.out.print(' ');
// display item
System.out.print(Arr[j]);
if(++j == heapSize) // done?
break;
if(++column==itemsPerRow) // end of row?
{
nBlanks /= 2; // half the blanks
itemsPerRow *= 2; // twice the items
column = 0; // start over on
System.out.println(); // new row
}
else // next item on row
for(int k=0; k<nBlanks*2-2; k++)
System.out.print(' '); // interim blanks
} // end for
System.out.println("\n"+dots+dots); // dotted bottom line
} // end displayHeap()
public void printHeap()
{
for (int i = 0; i <= heapSize - 1; i++)
{
System.out.println(Arr[i]);
}
}
public int extractMax()
{
if (heapSize == 0)
{
System.out.println("Heap is empty");
return -9999999;
}
else
{
int max = Arr[0];
Arr[0] = Arr[heapSize - 1];
heapSize = heapSize - 1;
heapify(0);
return max;
}
}
public void heapInsert(int item)
{
if(heapSize == capacity){
System.out.println("The heap is full!");
return;
}
int parent;
heapSize = heapSize + 1;
int i = heapSize - 1;
parent = (i - 1) / 2;
while (i > 0 && Arr[parent] < item)
{
Arr[i] = Arr[parent];
i = parent;
parent = (i - 1) / 2;
}
Arr[i] = item;
}
public void heapSort()
{
int temp;
// buildHeap();
int keepSize = heapSize;
for (int i = heapSize - 1; i >= 1; i--)
{
temp = Arr[heapSize-1];
Arr[heapSize - 1] = Arr[0];
Arr[0] = temp;
heapSize = heapSize - 1;
heapify(0);
}
heapSize = keepSize;
printHeap();
}
}
![import java.util.;
public class heapApplication
public static vold main(String args[])
Random rnd new Random();
System.out.println("Enter the capacity of the heap: ");
int size = Integer.parseInt(new Scanner(System.in).nextLine());
maxHeap H = new maxHeap(size); // create a new heap H
for(int i = 0; i<15; i++){
int x = rnd.nextInt(1001);
H. heapInsert(x);
System.out.printf("%d ", x);
H.displayleap();
System.out.println("Enter 1 for extractMax, 2 for heapInsert, 3 Heap Sort, 4 Exit");
int s = Integer.parseInt(new Scanner(System.in).nextLine());
while (s == 1 || s == 2 || s==3 || s==4)
if (s 1)
int max = H.extractMax();
if (max != -9999999)
System.out.println("The maximum element is:");
System.out.println(max);
System.out.println("The elements in the array after extracting the max:");
H.displayleap();
};
};
if (s = 2)
System.out.println("Insert a new item to the heap:");
int newItem = Integer.parseInt (new Scanner(System.in).nextLine());
H. heapInsert(newItem);
System.out.println("The elements in the array after inserting the new item: ");
H.displayleap();
};
if (s == 3)
int ans;
System.out.println("Warning: After heap sort, current heap will not be restored and the application will end!");
System.out.println("Continue with sorting? 1 for yes, 2 for no: \n");
ans = Integer.parseInt(new Scanner(System.in).nextLine());
if(ans1){
H. heapSort();
System.out.println("Goodbye!");
return;
};
if (s == 4)
{
System.out.printin("Goodbye!");
return;
};
System.out.println("Enter 1 for extractMax, 2 for heapInsert, 3 Heap Sort, 4 Exit");
s = Integer.parseInt(new Scanner(System.in).nextLine());](https://content.bartleby.com/qna-images/question/3ded9074-643d-40d8-825c-f0dff7af7ebf/f2475972-3af4-4ffd-800b-352814c897ee/p82cwnb_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- java dont use others answers please follow thw istructions on the photo Thank you!!!arrow_forwardPP 12.4 The array implementation in this chapter keeps the top variablepointing to the next array position above the actual top of thestack. Rewrite the array implementation such that stack[top] isthe actual top of the stack. PP 12.5 There is a data structure called a drop-out stack that behaves likea stack in every respect except that if the stack size is n, whenthe n + 1 element is pushed, the first element is lost. Implement adrop-out stack using an array. (Hint: A circular array implementation would make sense.)arrow_forwardPython: Written without libraries method find_ansestors , that takes in an index i and returns all ancestors of node located at index i. What is the time complexity of your function? class ArrayBinaryTree: def __init__(self): self._heap = [] def find_ancestors(self, i: int): if(i==0): return;arrow_forward
- Draw a memory map for the code you see on the next page, until the execution reaches the point indicated by the comment /* HERE */.In your diagram:• You must have a stack, heap, and static memory sections • Identify each frame as illustrated by the previous examples.• Draw your variables as they are encountered during program execution. Code: public class Passenger {private String name;private int ticketCost;private StringBuffer luggage;private static final int LUGGAGE_COST = 20;public Passenger(String name, int ticketCost) {this.name = name;this.ticketCost = ticketCost;luggage = new StringBuffer();}public Passenger addLuggage(String desc) {ticketCost += LUGGAGE_COST;luggage.append(desc);return this;}public int getTicketCost() {return ticketCost;}public Passenger reduceCost(int by) {ticketCost -= by;/* HERE */return this;}public String toString() {return "Passenger [name=" + name + ", ticketCost=" + ticketCost + ", luggage=" + luggage + "]";}}public class Driver {public static void…arrow_forwardAnswer in 5 mins else downvotearrow_forwardexplain this codes : class Heap{ int [] heapArray; int maxSize, heapSize; public Heap(int max) { maxSize=max; heapSize=0; heapArray = new int[maxSize]; } public boolean insert(int ele) { if((heapSize+1) == maxSize) return false; heapArray[++heapSize]=ele; int pos = heapSize; while(pos != 1 && ele < heapArray[pos/2]) { heapArray[pos] = heapArray[pos/2]; pos = pos/2; } heapArray[pos] = ele; return true; } public void displayHeap() { System.out.println("The contents of heap are: "); for(int i = 1;i <= heapSize;i++) System.out.print(heapArray[i]+" "); }}public class HeapTest { public static void main(String args[]){ Heap h=new Heap(7); h.insert(7); h.insert(8);h.insert(2);h.insert(4);h.insert(12);h.insert(5);h.displayHeap();}}arrow_forward
- Project Overview: This project is for testing the use and understanding of stacks. In this assignment, you will be writing a program that reads in a stream of text and tests for mismatched delimiters. First, you will create a stack class that stores, in each node, a character (char), a line number (int) and a character count (int). This can either be based on a dynamic stack or a static stack from the book, modified according to these requirements. I suggest using a stack of structs that have the char, line number and character count as members, but you can do this separately if you wish.Second, your program should start reading in lines of text from the user. This reading in of lines of text (using getline) should only end when it receives the line “DONE”.While the text is being read, you will use this stack to test for matching “blocks”. That is, the text coming in will have the delimiters to bracket information into blocks, the braces {}, parentheses (), and brackets [ ]. A string…arrow_forwardUSING C LANGUAGE IN NETBEANS : Write a program menu that creates and manages Fibonacci heaps. The program must implement the following operations: creation, insertion, find min, extract min, decrease key, and deletion. **NOTE: The program should present a menu where user may choose from implemented options.arrow_forwardSimple JAVA linkedlist code implementation please help and complete any part you can - Without using the java collections interface (ie do not import java.util.List,LinkedList, Stack, Queue...)- Create an implementation of LinkedList interface- For the implementation create a tester to verify the implementation of thatdata structure performs as expected Build Bus Route – Linked List- Your task is to:o Implement the LinkedList interface (fill out the implementation shell)o Put your implementation through its paces by exercising each of themethods in the test harnesso Create a client (a class with a main) ‘BusClient’ which builds a busroute by performing the following operations on your linked list:o§ Create (insert) 4 stations§ List the stations§ Check if a station is in the list (print result)• Check for a station that exists, and onethat doesn’t§ Remove a station§ List the stations§ Add a station before another station§ List the stations§ Add a station after another station§ Print the…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
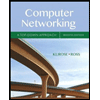
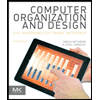
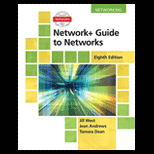
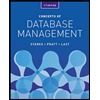
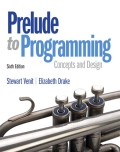
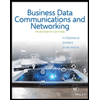