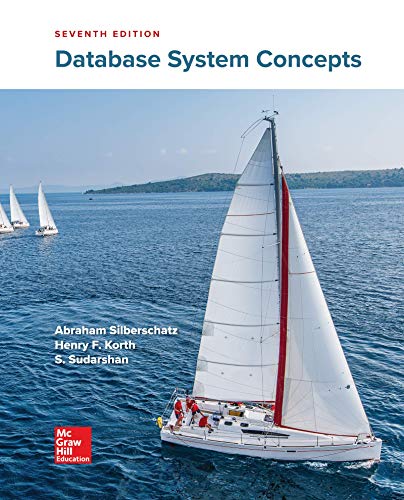
Concept explainers
Please answer the problem in the screenshot. Please use the methods below as a base.
import java.util.*;
class HeapMax {
// we go with arraylist instead of array for size flexibility
private ArrayList<Integer> data;
// DO NOT MODIFY THIS METHOD
public HeapMax() {
data = new ArrayList<Integer>(0);
}
// insert a new element and restore max heap property
public void insert(int element) {
}
// return max
public int getMax() {
// remove this line
return 0;
}
// remove max and restore max heap property
public int removeMax() {
// remove this line
return 0;
}
// heap builder
public void build(int[] arr) {
}
// print out heap as instructed in the handout
public void display() {
}
// you are welcome to add any supporting methods
}
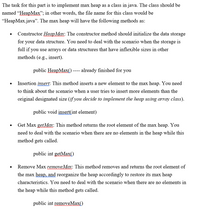
![Display Heap display: This method should print all elements in the max heap in
the order of levels (e.g., root element first, root's two children second, root's
grandchildren third, etc.). Elements on the same level should be separated with
one or two white spaces; elements on different levels should be separated with a
comma.
public void display()
Build a Heap build: This method should take an array as the only parameter and
build a heap based on the given array. Note that this method is NOT supposed to
be called if a heap is non-empty.
public void build(int[] arr)
These methods should NOT be declared as static. You are free to use any extra instances
and helper methods.](https://content.bartleby.com/qna-images/question/1725b614-c61a-470a-b05f-008b5304bcc7/2c7c5757-2b7e-4217-8965-da21ef5d9221/dnwdw74_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Java 1. Implement ArrayUnorderedList<T> class which will extend ArrayList<T> by defining the following additional methods(i) public void addToFront(T element); //Adds the specified element to the front of the list.(ii) public void addToRear(T element); //Adds the specified element to the rear of the list.(iii) public void addAfter(T element, T target); //Adds the specified element after the specified target element.a. Create an object of ArrayUnorderedList<T> class and perform some add, remove, and search operations and finally print the entire Stack.arrow_forwardjava This method gets an Arraylist of Integers and a number(Integer). It returns an Arraylist. It removes any instance of the given number from the Arraylist. Example: romoveInst([1,1,2,3,1,4],1) returns: [2,3,4] romoveInst([3,4,3,3],4) returns: [3,3,3] public static ArrayList<Integer> removeInst(ArrayList<Integer> r,Integer n) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); int n = in.nextInt(); ArrayList<Integer> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextInt()); } System.out.println(removeInst(list, n)); } }arrow_forwardPlease edit this class Authenticator. Remove breaks. Also create user array of size 100 rather than 3 different arrays. Also edit so it can be more OOP import java.util.Scanner; public class AuthenticatorApp { public static void main(String[] args) throws Exception { Scanner input=new Scanner(System.in); Authenticator obj=new Authenticator("user.data"); String Username,Password; boolean done=false; int count=0; while(count<3) { System.out.print("Username? "); Username=input.next(); System.out.print("Password? "); Password=input.next(); obj.authenticate(Username,Password); count++; } System.out.print("Too many failed attempts... please try again later"); input.close(); } } ------------------------------------------------------------------------------------------------------------import java.io.File; import…arrow_forward
- JAVA programming question i will attach images of the ourQueue code and the main I have to do the following: *create an ourQueue object, add the following sequence: *2,-3,4,-6,7,8,-9,-11 *create and ourStack object *write a program/ one loop that would result into the stack containing all of the negative number at the bottom and the positive numbers at the top. *outStack at the end should contain the following *[-3,-6,-9,-11,2,4,7,8]arrow_forwardQuestion 7: Write a method for the ArrayBoundedStack class that creates and returns a stack copy of this. (Hint: use topIndex and elements) Use following method signature: public ArrayBoundedStack<T> copy()arrow_forward44 // create a loop that reads in the next int from the scanner to the intList 45 46 47 // call the ReverseArray function 48 49 50 System.out.print("Your output is: "); 51 // print the output from ReverseArray 52 ww 53 54 55 }arrow_forward
- package edu.umsl.iterator;import java.util.ArrayList;import java.util.Arrays;import java.util.Collection;import java.util.Iterator;public class Main {public static void main(String[] args) {String[] cities = {"New York", "Atlanta", "Dallas", "Madison"};Collection<String> stringCollection = new ArrayList<>(Arrays.asList(cities));Iterator<String> iterator = stringCollection.iterator();while (iterator.hasNext()) {System.out.println(/* Fill in here */);}}} Rewrite the while loop to print out the collection using an iterator. Group of answer choices iterator.toString() iterator.getClass(java.lang.String) iterator.remove() iterator.next()arrow_forwardPlease answer the problem in the screenshot. Please use the methods below as a base. The language is in Java. import java.util.*; class HeapMax { // we go with arraylist instead of array for size flexibility private ArrayList<Integer> data; // DO NOT MODIFY THIS METHOD public HeapMax() { data = new ArrayList<Integer>(0); } // insert a new element and restore max heap property public void insert(int element) { } // return max public int getMax() { // remove this line return 0; } // remove max and restore max heap property public int removeMax() { // remove this line return 0; } // heap builder public void build(int[] arr) { } // print out heap as instructed in the handout public void display() { } // you are welcome to add any supporting methods }arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forward
- The following code prints out all permutations of the string generate. Insert the missing statement. public class PermutationGeneratorTester { public static void main(String[] args) { PermutationGenerator generator = new PermutationGenerator("generate"); ArrayList<String> permutations = generator.getPermutations(); for (String s : permutations) { ____________________ } } }arrow_forwardstarter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardJava programming language I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks! I have to write the remove method in the code below. i attached the part where i need to write it. public class ourArrayList<T>{ private Node<T> Head = null; private Node<T> Tail = null; private int size = 0; //default constructor public ourArrayList() { Head = Tail = null; size = 0; } public int size() { return size; } public boolean isEmpty() { return (size == 0); } //implement the method add, that adds to the back of the list public void add(T e) { //HW TODO and TEST //create a node and assign e to the data of that node. Node<T> N = new Node<T>();//N.mData is null and N.next is null as well N.setsData(e); //chain the new node to the list //check if the list is empty, then deal with the special case if(this.isEmpty()) { //head and tail refer to N this.Head = this.Tail = N; size++; //return we are done.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
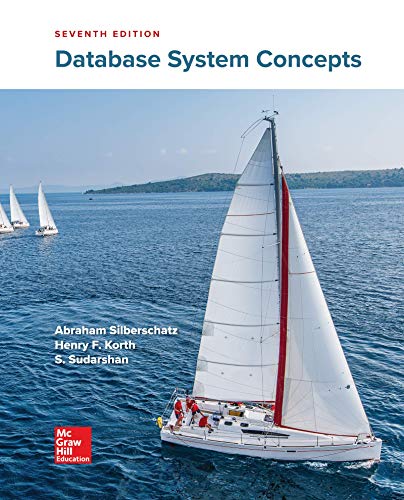
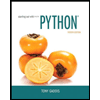
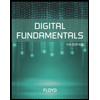
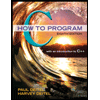
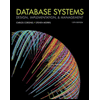
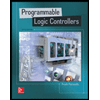