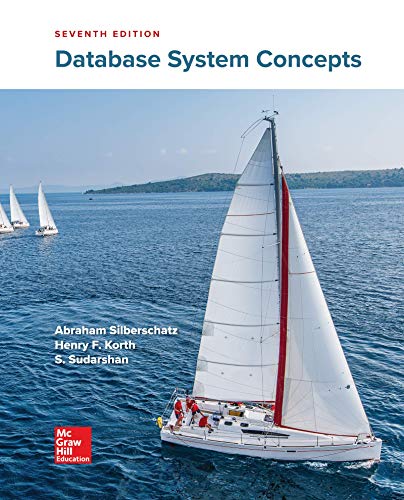
This is Heap class:
package sorting;
import java.util.Arrays;
public class Heap {
private int[] data;
private int heapSize;
public Heap(int[] data,int heapSize) {
this.data = data;
this.heapSize = heapSize;
}
public void setHeapSize(int i) {
heapSize = i;
}
public int getHeapSize() {
return heapSize;
}
//return the parent of ith element in the array.
//should return -1 if the ith element is the root of the heap
public int parent(int i) {
if(i==0)
return -1;
else
return (i-1)/2;
}
//returns the index of the left child of the ith element in the array
//for leaves the index will be greater than the heapSize
public int left(int i) {
return (2*i)+1;
}
//returns the index of the right child of the ith element in the array
//for leaves the index will be greater than the heapSize
public int right(int i) {
return (2*i) + 2;
}
//modifies the data array so that the tree rooted at the loc element
//is a max heap.
//Assumes that the trees rooted at the left and right children of loc
//are max heaps
public void maxHeapify(int loc) {
int l = left(loc);
int r = right(loc);
int largest;
if(l<heapSize && data[l]>data[loc])
largest = l;
else
largest = loc;
if(r<heapSize && data[r]>data[largest])
largest = r;
if(largest != loc) {
swap(loc,largest);
maxHeapify(largest);
}
}
private void swap(int i, int j) {
int temp = data[i];
data[i] = data[j];
data[j] = temp;
}
//converts the data array to an array that represents a max heap
public void buildMaxHeap() {
int n = heapSize;
for(int i=(n/2)-1;i>=0;i--) {
maxHeapify(i);
}
}
}
Please Implement the heapSort using above Heap class:
public static void heapSort(int[] arr){
}
Implement the heapSort
MaxHeap data structure provided for you Heap.java.

Step by stepSolved in 2 steps

- JAVA programming languagearrow_forwardimport java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardJava programming language I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks! I have to write the remove method in the code below. i attached the part where i need to write it. public class ourArrayList<T>{ private Node<T> Head = null; private Node<T> Tail = null; private int size = 0; //default constructor public ourArrayList() { Head = Tail = null; size = 0; } public int size() { return size; } public boolean isEmpty() { return (size == 0); } //implement the method add, that adds to the back of the list public void add(T e) { //HW TODO and TEST //create a node and assign e to the data of that node. Node<T> N = new Node<T>();//N.mData is null and N.next is null as well N.setsData(e); //chain the new node to the list //check if the list is empty, then deal with the special case if(this.isEmpty()) { //head and tail refer to N this.Head = this.Tail = N; size++; //return we are done.…arrow_forward
- Write all the code within the main method in the Test Truck class below. Implement the following functionality. a) Constructs two truck objects: one with any make and model you choose and the second object with gas tank capacity 10. b) If an exception occurs, print the stack trace. c) Prints both truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main ( String [] args ) { heres the truck class information A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas…arrow_forward/** * TODO: file header */ import java.util.*; public class BSTManual { /** * TODO * @author TODO * @since TODO */ // No style for this file. public static ArrayList<String> insertElements() { ArrayList<String> answer_pr1 = new ArrayList<>(11); /* * make sure you add your answers in the following format: * * answer_pr1.add("1"); --> level 1 (root level) of the output BST * answer_pr1.add("2, X"); --> level 2 of the output BST * answer_pr1.add("3, X, X, X"); --> level 3 of the output BST * * the above example is the same as the second pictoral example on your * worksheet */ //TODO: add strings that represent the BST after insertion. return answer_pr1; } public static ArrayList<String> deleteElements() { ArrayList<String> answer_pr2 = new ArrayList<>(11); /* * Please refer to the example in insertElements() if you lose track * of how to properly enter your answers */ //TODO: add strings that represent the…arrow_forwardStarter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forward
- comptuer sciecne helparrow_forwardpackage circularlinkedlist;import java.util.Iterator; public class CircularLinkedList<E> implements Iterable<E> { // Your variablesNode<E> head;Node<E> tail;int size; // BE SURE TO KEEP TRACK OF THE SIZE // implement this constructorpublic CircularLinkedList() {} // I highly recommend using this helper method// Return Node<E> found at the specified index// be sure to handle out of bounds casesprivate Node<E> getNode(int index ) { return null;} // attach a node to the end of the listpublic boolean add(E item) {this.add(size,item);return false; } // Cases to handle// out of bounds// adding to empty list// adding to front// adding to "end"// adding anywhere else// REMEMBER TO INCREMENT THE SIZEpublic void add(int index, E item){ } // remove must handle the following cases// out of bounds// removing the only thing in the list// removing the first thing in the list (need to adjust the last thing in the list to point to the beginning)// removing the last…arrow_forwardJava prgm basedarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
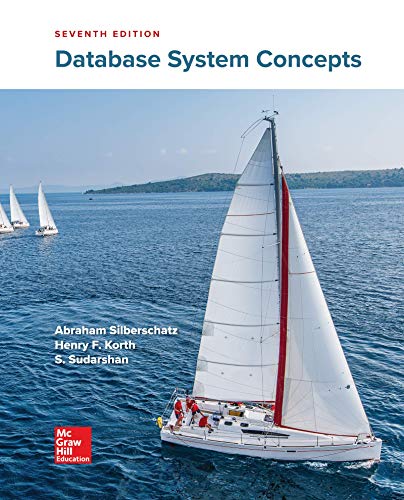
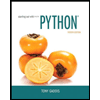
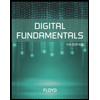
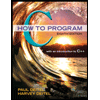
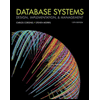
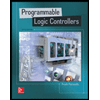