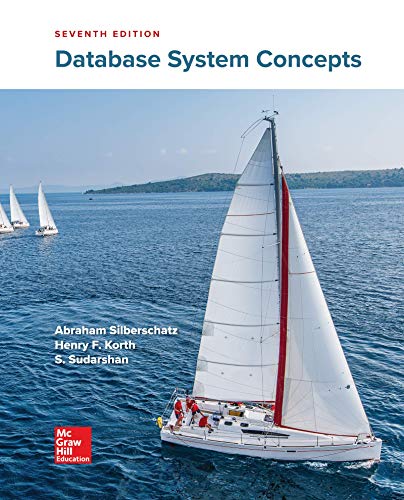
Concept explainers
Finish this code:
import java.util.Scanner;
import java.util.Stack;
/**
Class for reversing the order of a sentence.
*/
public class SentenceReverser
{
/**
Reverses the given sentence.
@param sentence Sentence to be reversed.
@return Reversed sentence.
*/
public static String reverse(String sentence)
{
String reversed = "";
Scanner scanner = new Scanner(sentence);
Stack<String> stack = new Stack<String>();
// put your work below
// ...
return reversed;
}
public static void main(String[] args)
{
String sentence = "Mary had a little lamb. Its fleece was white as snow.";
String reversed = SentenceReverser.reverse(sentence);
System.out.println("Given: " + sentence);
System.out.println("Expected: Lamb little a had mary. Snow as white was fleece its.");
System.out.println("Actual: " + reversed);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- USING THE FOLLOWING METHOD SWAP CODE: import java.util.*; class ListIndexOutOfBoundsException extends IndexOutOfBoundsException { public ListIndexOutOfBoundsException(String s) { super(s); } } class ListUtil { public static int maxValue(List<Integer> aList) { if(aList == null || aList.size() == 0) { throw new IllegalArgumentException("List cannot be null or empty"); } int max = aList.get(0); for(int i = 1; i < aList.size(); i++) { if(aList.get(i) > max) { max = aList.get(i); } } return max; } public static void swap(List<Integer> aList, int i, int j) throws ListIndexOutOfBoundsException { if(aList == null) { throw new IllegalArgumentException("List cannot be null"); } if(i < 0 || i >= aList.size() || j < 0 || j >= aList.size()) { throw new ListIndexOutOfBoundsException("Index out…arrow_forwardRPN.java import java.util.Scanner; /** * Reverse Polish Notation calculator. It evaluates * a string with expressions in RPN format and prints * the results. Exampel of a stack use. */public class RPN{ /** * Given a string, return an integer version of * the string. Check if the string contains only * numbers, if so, then does the conversion. If * it does not, it reurns 0. * @param t string with numeric token * @return int version of the numeric token in t */ public int getValue(String t) { if (t.matches("[0-9]+")) { return Integer.parseInt(t); } else { return 0; } } /** * Evaluates a single token in an RPN expression. If it is * a number, it pushes the token to the stack. If it is an * operator, then it pulls 2 numbers from the stack, performs * the operation and pushes back into the stack the result. * @param token to be evaluated * @param stack holding values for…arrow_forwardimport java.util.*; public class Main{ public static void main(String[] args) { Main m = new Main(); m.go(); } private void go() { List<Stadium> parks = new ArrayList<Stadium>(); parks.add(new Stadium("PNC Park", "Pittsburgh", 38362, true)); parks.add(new Stadium("Dodgers Stadium", "Los Angeles", 56000, true)); parks.add(new Stadium("Citizens Bank Park", "Philadelphia", 43035, false)); parks.add(new Stadium("Coors Field", "Denver", 50398, true)); parks.add(new Stadium("Yankee Stadium", "New York", 54251, false)); parks.add(new Stadium("AT&T Park", "San Francisco", 41915, true)); parks.add(new Stadium("Citi Field", "New York", 41922, false)); parks.add(new Stadium("Angels Stadium", "Los Angeles", 45050, true)); Collections.sort(parks, Stadium.ByKidZoneCityName.getInstance()); for (Stadium s : parks) System.out.println(s); }}…arrow_forward
- JAVA PROGRAM MODIFY THIS PROGRAM SO IT READS THE TEXT FILES IN HYPERGRADE. I HAVE PROVIDED THE INPUTS AND THE FAILED TEST CASE AS A SCREENSHOT. HERE IS THE WORKING CODE TO MODIFY: import java.io.*;import java.util.*;public class NameSearcher { private static List<String> loadFileToList(String filename) throws FileNotFoundException { List<String> namesList = new ArrayList<>(); File file = new File(filename); if (!file.exists()) { throw new FileNotFoundException(filename); } try (Scanner scanner = new Scanner(file)) { while (scanner.hasNextLine()) { String line = scanner.nextLine().trim(); String[] names = line.split("\\s+"); for (String name : names) { namesList.add(name.toLowerCase()); } } } return namesList; } private static Integer searchNameInList(String name, List<String> namesList) {…arrow_forwardWrite all the code within the main method in the Test Truck class below. Implement the following functionality. a) Constructs two truck objects: one with any make and model you choose and the second object with gas tank capacity 10. b) If an exception occurs, print the stack trace. c) Prints both truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main ( String [] args ) { heres the truck class information A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
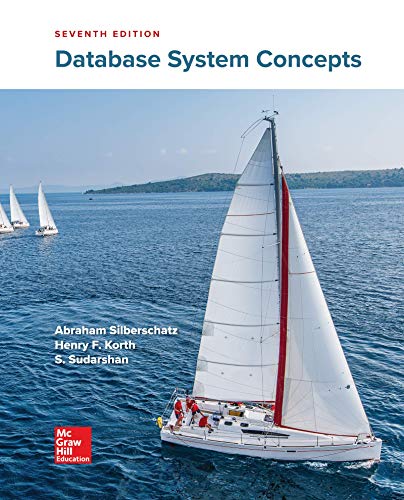
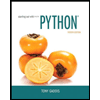
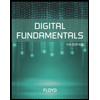
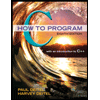
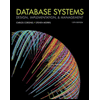
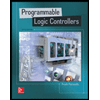