Focus on dictionary methods, use of functions, and good programming style For this assignment, you will create a glossary (dictionary) of technical terms and definitions. It will be set up as a Python dictionary structure. The file glossary_starter.py is a complete starter framework for the assignment. It includes some initial values for the dictionary. It is long because most of the code has already been written for you. Your task is to complete the five individual functions for adding and deleting terms, looking up terms, listing them, and printing out both the terms and definitions. These functions are all short, just a couple of lines, and use basic dictionary methods and techniques. Here is some sample output. Glossary system 1) Add a term 2) List terms 3) Get a definition 4) Delete a term 5) Print out dictionary 6) Quit Enter your choice: 2 argument dictionary hashmap list set 5 terms Glossary system 1) Add a term 2) List terms 3) Get a definition 4) Delete a term 5) Print out dictionary 6) Quit Enter your choice: 1 What term do you want to add? String What is the definition for 'String'? A basic type in Python that stores text. Glossary system 1) Add a term 2) List terms 3) Get a definition 4) Delete a term 5) Print out dictionary 6) Quit Enter your choice: 3 What term do you want to lookup? STRING definition of "STRING" - A basic type in Python that stores text.
Focus on dictionary methods, use of functions, and good programming style
For this assignment, you will create a glossary (dictionary) of technical terms and definitions. It will be
set up as a Python dictionary structure. The file glossary_starter.py is a complete starter framework for
the assignment. It includes some initial values for the dictionary. It is long because most of the code
has already been written for you.
Your task is to complete the five individual functions for adding and deleting terms, looking up terms, listing
them, and printing out both the terms and definitions. These functions are all short, just a couple of lines, and
use basic dictionary methods and techniques.
Here is some sample output.
Glossary system
1) Add a term
2) List terms
3) Get a definition
4) Delete a term
5) Print out dictionary
6) Quit
Enter your choice: 2
argument
dictionary
hashmap
list
set
5 terms
Glossary system
1) Add a term
2) List terms
3) Get a definition
4) Delete a term
5) Print out dictionary
6) Quit
Enter your choice: 1
What term do you want to add? String
What is the definition for 'String'? A basic type in Python that stores text.
Glossary system
1) Add a term
2) List terms
3) Get a definition
4) Delete a term
5) Print out dictionary
6) Quit
Enter your choice: 3
What term do you want to lookup? STRING
definition of "STRING" - A basic type in Python that stores text.

Step by step
Solved in 4 steps with 6 images

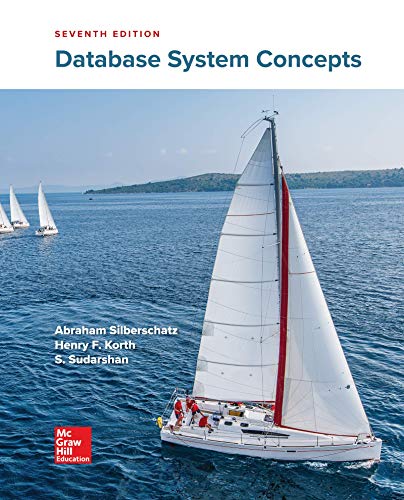
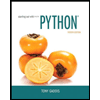
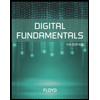
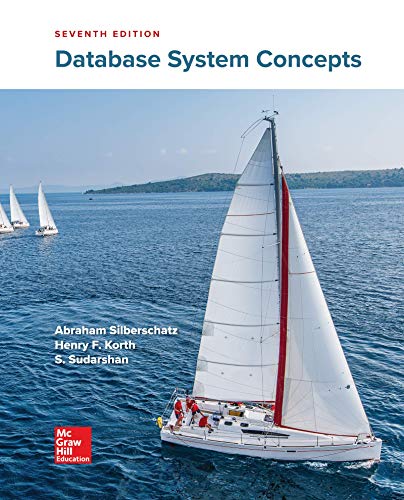
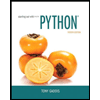
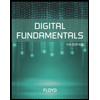
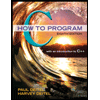
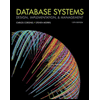
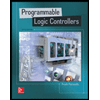