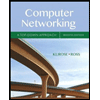
My homework was to design and implement a simple social network program in Java. I should use an adjacency matrix data structure in my implementation.
Write a social network program in Java. The default information for this network is stored in two files: index.txt and friend.txt. The file index.txt stores the names of the people in the network – you may assume that we only store the given names and these names are unique; and friend.txt stores who knows whom. The program must read these two files. The following section describes the format of these two files.
The friend.txt takes the following format. The first line is the number of pairs of friends. Each subsequent line has two integer numbers. The first two numbers are the indices of the names. The following is an example of friend.txt:
5
0 3
1 3
0 1
2 4
1 5
The index.txt stores the names of the people in the network. The first line is the number of people in the file; for example:
6
0 Gromit
1 Gwendolyn
2 Le-Spiderman
3 Wallace
4 Batman
5 Superman
The second line “Gromit” is the label for vertex 0, the third line "Gwendolyn" is for vertex 1. For this system, we will only record the given names (without spaces). By looking at the content of these two files, we know that Gromit is a friend of Wallace and Wallace is a friend of Gromit. We also know that Gwendolyn knows Wallace and Wallace knows Gwendolyn, and so on. Unknown to Wallace and Gromit, Gwendolyn is a friend of Superman!
To build the social network, the program reads both files. It uses friend.txt to build the vertices and edges of the social network, and use index.txt to label the vertices. If it fails to read the files (such as file not found), then it must also print appropriate error messages using System.err.
The program must also check that the number of relations or indices read is the same as the number of relations or indices specified at the start of each file. The two files above are kept short to simplify the explanation and can be used when you first start developing the program. Eventually, the program will be tested with larger datasets of about 20 friends and 30 pairs of friends.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Java programming 1. Write the code that will read the inventory information from a file, populate the object, and populate a linked list with all the inventory objects. Then find a particular item in the linked list based on user input and display the information for that item. Inventory inventory = new Inventory (); Write the line of code to find an inventory object in the linked list using the property for InventoryIDarrow_forwardIn C++ language. Please look at the instructions and help with program. This is for intermediate not advanced. so help me understand pleasearrow_forwardjava program For this question, the server contains the id, name and cgpa of some Students in a file. The client will request the server for specific data, which the server will provide to the client. Server: The server contains the data of some Students. For each student, the server contains id, name and cgpa data in a file. Here's an example of the file: data.txt101 Saif 3.52201 Hasan 3.81.... Create a similar file in you server side. The file should have at least 8 students. The client will request the server to provide the details (id, name, cgpa) of the Nth highest cgpa student in the file. If N's value is 1, the server will return the details of the student with the highest cgpa. if N's value is 3, the server will return the details of the student with the third highest cgpa. If N's value is incorrect, the server returns to the client: "Invalid request". Client: The client sends the server the value of N, which is an integer number. The client takes input the value of N…arrow_forward
- write in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forwardC++ Complete the program that will allow the user to enter data on 10 Halloween costumes. The program will create Costume objects and then store the Costume objects in a Hash Table. The Costume ID will be the key and a pointer to the Costume object will be the value. You are given Lab7.cpp, HashEntry.h, and Costume.h and you should not have to make any changes to these files at all. You will need to write HashTable.h, which implements the HashTable class. This should NOT be a template class. Your HashTable class should resolve collisions by linear probing and should use the HashEntry class for the linkedlist nodes. Because we’re using probing, there is no removal function. This is okay for this application, since we want to keep a record of costumes that are no longer being kept in stock. ---GIVEN--- Lab.cpp #include <iostream> #include "HashTable.h" #include "Costume.h" using namespace std; int main() { int size; int key; Costume *newCostume; string name; float price;…arrow_forwardWrite a c++ program that reads in input commands related to a queue with no more than ten elements and performs the specified operations. Note that you should create three files, one for the main, one for Queue.h, and one for Queue.cpp. Those commands are: 'E', which will be followed by a number. You should enqueue that number 'D', which will dequeue the value from the front of the queue and print it, followed by a new line 'K'. which will peek at the value at the front of the queue and print it, followed by a new line 'Q', which will quit the program You can assume that all the input is valid.arrow_forward
- Write a program in C++ that prints a sorted phone list from a database of names and phone numbers. The data is contained in two files named "phoneNames.txt" and "phoneNums.txt". The files may contain up to 2000 names and phone numbers. The files have one name or one phone number per line. To save paper, only print the first 50 lines of the output. Note: The first phone number in the phone number file corresponds to the first name in the name file. The second phone number in the phone number file corresponds to the second name in the name file. etc. You will find the test files in the Asn Five.ziparrow_forwardUse the started code provided with QUEUE Container Adapter methods and provide the implementation of a requested functionality outlined below. This program has to be in c++, and have to use the already started code below. Scenario: A local restaurant has hired you to develop an application that will manage customer orders. Each order will be put in the queue and will be called on a first come first served bases. Develop the menu driven application with the following menu items: Add order Next order Previous order Delete order Order Size View order list View current order Order management will be resolved by utilization of an STL-queue container’s functionalities and use of the following Queue container adapter functions: enQueue: Adds the order in the queue DeQueue: Deletes the order from the queue Peek: Returns the order that is top in the queue without removing it IsEmpty: checks do we have any orders in the queue Size: returns the number of orders that are in the queue…arrow_forwardcreate a class Person to represent a person and another one to represent the database. A dictionary should be a data member of the database class, with Person objects as search keys for this dictionary. Write a program that tests and demonstrates your database.arrow_forward
- You are going to write a program (In Python) called BankApp to simulate a banking application.The information needed for this project are stored in a text file. Those are:usernames, passwords, and balances.Your program should read username, passwords, and balances for each customer, andstore them into three lists.userName (string), passWord(string), balances(float)The txt file with information is provided as UserInformtion.txtExample: This will demonstrate if file only contains information of 3 customers. Youcould add more users into the file.userName passWord Balance========================Mike sorat1237# 350Jane para432@4 400Steve asora8731% 500 When a user runs your program, it should ask for the username and passwordfirst. Check if the user matches a customer in the bank with the informationprovided. Remember username and password should be case sensitive.After asking for the user name, and password display a menu with the…arrow_forwardWrite a python program that reads the contents of a text file. The program should create a dictionary in which the keys are the individual words found in the file and the values are the number of times each word appears. For example, if the word "the" appears 128 times, the dictionary would contain an element with 'the' as the key and 128 as the value. The program should either display the frequency of each word or create a second file containing a list of each word and it's frequency. This program has really been giving me trouble. Any help is great appreciated. Thanks so much!arrow_forwardBackground Often, data are stored in a very compact but not human-friendly way. Think of how dishes in a menu can be stored in a restaurant database somewhere in the cloud. One way a dish object can be stored is this: { "name": "Margherita", "calories": 800, "price": 18.90, "is_vegetarian": "yes", "spicy_level": 2 } Obviously, this format is not user-readable, so the restaurant's frontend team produced many lines of code to render and display this content in a way that's more understandable to us, the restaurant users. We have a mini-version of the same task coming up. Given a list of similar complex objects (represented by Python dictionaries), display them in a user-friendly way that we'll define in these instructions. What is a "dish"? In this project, one dish item is a dictionary object that is guaranteed to have the following keys: "name": a string that stores the dish's name. "calories": an integer representing the calorie intake for one serving of the dish. "price": a float to…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
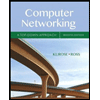
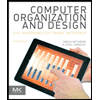
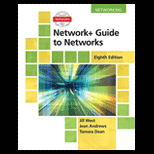
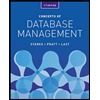
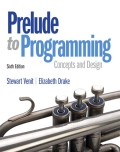
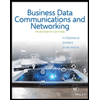