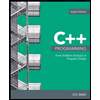
Given integer inputs howMany and maxNum, generate an array of howMany unique random integers from 0 to maxNum (exclusive).
The structure of the program is:
- main() calls uniqueRandomInts() with arguments howMany and maxNum.
- uniqueRandomInts() returns an array of howMany unique random integers.
- The required output is already provided in main() and printNums().
Complete uniqueRandomInts(), which generates random integers until howMany unique integers have been collected in array nums.
Hint: If a generated number is new, add the number to the array nums and the set alreadySeen. If the number has been seen before, increment the static variable retries and generate another random integer.
Note: For testing purposes, a random number generator object is created with a fixed seed value (29) in uniqueRandomInts(). Refer to the textbook section on Random numbers to learn more about pseudo-random numbers.
Ex: When the input is:
5 8
the output is
5 0 1 7 3 [3 retries]
LabProgram.java

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters to 0, and then generate a large number of pseudorandom integers between 0 and 9. Each time a 0 occurs, increment the variable you have designated as the zero counter; when a 1 occurs, increment the counter variable that’s keeping count of the 1s that occur; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of the time they occurred.arrow_forward(Numerical) Given a one-dimensional array of integer numbers, write and test a function that displays the array elements in reverse order.arrow_forward(Practice) Write array declarations, including initializers, for the following: a. A list of 10 integer voltages: 89, 75, 82, 93, 78, 95, 81, 88, 77, and 82 b. A list of five double-precision slopes: 11.62, 13.98, 18.45, 12.68, and 14.76 c. A list of 100 double-precision distances; the first six distances are 6.29, 6.95, 7.25, 7.35, 7.40, and 7.42 d. A list of 64 double-precision temperatures; the first 10 temperatures are 78.2, 69.6, 68.5, 83.9, 55.4, 67.0, 49.8, 58.3, 62.5, and 71.6 e. A list of 15 character codes; the first seven codes are f, j, m, q, t, w, and zarrow_forward
- (Electrical eng.) a. An engineer has constructed a two-dimensional array of real numbers with three rows and five columns. This array currently contains test voltages of an amplifier. Write a C++ program that interactively inputs 15 array values, and then determines the total number of voltages in these ranges: less than 60, greater than or equal to 60 and less than 70, greater than or equal to 70 and less than 80, greater than or equal to 80 and less than 90, and greater than or equal to 90. b. Entering 15 voltages each time the program written for Exercise 7a runs is cumbersome. What method could be used for initializing the array during the testing phase? c. How might the program you wrote for Exercise 7a be modified to include the case of no voltage being present? That is, what voltage could be used to indicate an invalid voltage, and how would your program have to be modified to exclude counting such a voltage?arrow_forward(Program) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters, such as zerocount, onecount, twocount, and so forth, to 0. Then generate a large number of pseudorandom integers between 0 and 9. Each time 0 occurs, increment zerocount; when 1 occurs, increment onecount; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of time they occurred.arrow_forward(Practice) Write array declarations for the following: a. A list of 100 double-precision voltages b. A list of 50 double-precision temperatures c. A list of 30 characters, each representing a code d. A list of 100 integer years e. A list of 32 double-precision velocities f. A list of 1000 double-precision distances g. A list of 6 integer code numbersarrow_forward
- (Practice) Write specification statements for the following: a. An array of integers with 6 rows and 10 columns b. An array of integers with 2 rows and 5 columns c. An array of characters with 7 rows and 12 columns d. An array of characters with 15 rows and 7 columns e. An array of double-precision numbers with 10 rows and 25 columns f. An array of double-precision numbers with 16 rows and 8 columnsarrow_forwardMark the following statements as true or false. A double type is an example of a simple data type. (1) A one-dimensional array is an example of a structured data type. (1) The size of an array is determined at compile time. (1,6) Given the declaration: int list[10]; the statement: list[5] - list[3] * list[2]; updates the content of the fifth component of the array list. (2) If an array index goes out of bounds, the program always terminates in an error. (3) The only aggregate operations allowable on int arrays are the increment and decrement operations. (5) Arrays can be passed as parameters to a function either by value or by reference. (6) A function can return a value of type array. (6) In C++, some aggregate operations are allowed for strings. (11,12,13) The declaration: char name [16] = "John K. Miller"; declares name to be an array of 15 characters because the string "John K. Miller" has only 14 characters. (11) The declaration: char str = "Sunny Day"; declares str to be a string of an unspecified length. (11) As parameters, two-dimensional arrays are passed either by value or by reference. (15,16)arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
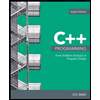
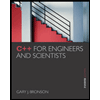
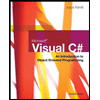
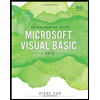
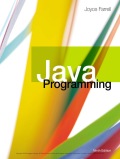