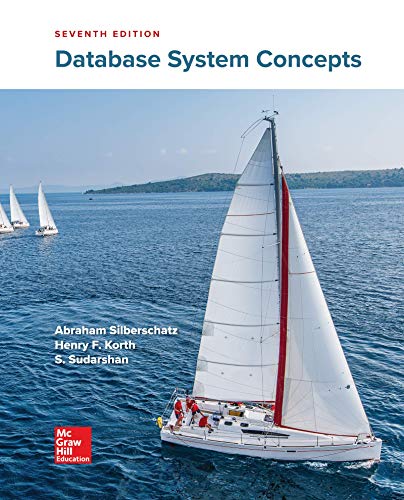
Concept explainers
Given the sequence, S2 = 1, 2, 4, 5, 7, 8, 10, 11, 13, 14, …
Write a RECURSIVE method called “sequence2” that takes a single int parameter (n) and returns the int value of the nth element of the sequence S2. You will need to determine any base cases and a recursive case that describes the listed sequence.
Use the following code to test your answers to questions 3 and 4
the output should print the two sequences given (S & S2):
public class TestSequences {
public static void main(String[] args) {
for(int i = 0; i < 10; i++) {
System.out.print(sequence(i) + " "); // 2, 4, 6, 12, 22, 40, 74, 136, 250, 460
}
System.out.println();
for(int i = 0; i < 10; i++) {
System.out.print(sequence2(i) + " "); // 1, 2, 4, 5, 7, 8, 10, 11, 13, 14
}
}
// *** Your method for sequence here ***
// *** Your method for sequences2 here ***
} // end of TestSequences class

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Make a code using Recursion The countSubstring function will take two strings as parameters and will return an integer that is the count of how many times the substring (the second parameter) appears in the first string without overlapping with itself. This method will be case insensitive. For example: countSubstring(“catwoman loves cats”, “cat”) would return 2 countSubstring(“aaa nice”, “aa”) would return 1 because “aa” only appears once without overlapping itself. public static int countSubstring(String s, String x) { if (s.length() == 0 || x.length() == 0) return 1; if (s.length() == 1 || x.length() == 1){ if (s.substring(0,1).equals(x.substring(0,1))){ s.replaceFirst((x), " "); return 1 + countSubstring(s.substring(1), x); } else { return 0 + countSubstring(s.substring(1), x); } } return countSubstring(s.substring(0,1), x) + countSubstring(s.substring(1), x); } public class Main { public static void main(String[] args) {…arrow_forwardWrite and test a Java/Python recursive method for finding the minimum element in an array, A, of n elements. What the running time? Hint: an array of size 1 would be the stop condition. The implementation is similar to linearSum method in lecture 5 examples. You can use the Math.min method for finding the minimum of two numbers.arrow_forwardImplement the logarithmBase22 method which, given a long integer number, returns the result of Logz2(number), rounded down to the nearest floor integer. Design and implement this as a recursive method. Read the test cases in the JUnit tester file for more detailed specification.arrow_forward
- The 4th problem mimics the situation where eagles flying in the sky can be spotted and counted.FindEagles: a recursive function that examines and counts the number of objects (eagles) in aphotograph. The data is in a two-dimensional grid of cells, each of which may be empty (value 0) orfilled (value 1 to 9). Maximum grid size is 50 x 50. The filled cells that are connected form an object(eagle). Two cells are connected if they are vertically, horizontally, or diagonally adjacent. Thefollowing figure shows 3 x 4 grids with 3 eagles. 0 0 1 21 0 0 01 0 3 1 FindEagle function takes as parameters the 2-D array and the x-y coordinates of a cell that is a part ofan eagle (non-zero value) and erases (change to 0) the image of an eagle. The function FindEagleshould return an integer value that counts how many cells has been counted as part of an eagle and havebeen erased. The following sample data has two pictures, the first one is 3 x 4, and the second one is 5 x 5 grids. Notethat your program…arrow_forwardT/F 11. A recursion will still be replaced by an iteration and the other way around.arrow_forwardThe following recursive method get Number Equal searches the array x of 'n integers for occurrences of the integer val. It returns the number of integers in x that are equal to val. For example, if x contains the 9 integers 1, 2, 4, 4, 5, 6, 7, 8, and 9, then getNumberEqual(x, 9, 4) returns the value 2 because 4 occurs twice in x. public static int getNumberEqual(int x[], int n, int val) { if (n< 0) ( return 0; } else { if (x[n-1) == val) { return getNumberEqual(x, n-1, val) +1; } else { return getNumber Equal(x, n-1, val); } // end if ) // end if } // end get Number Equal Demonstrate that this method is recursive by listing the criteria of a recursive solution and stating how the method meets each criterion.arrow_forward
- The Fibonacci algorithm is a famous mathematical function that allows us to create a sequence of numbers by adding together the two previous values. For example, we have the sequence:1, 1, 2, 3, 5, 8, 13, 21…Write your own recursive code to calculate the nth term in the sequence. You should accept a positive integer as an input, and output the nth term of the sequence.Once you have created your code, add comments describing how the code works, and the complexity of any code you have created.arrow_forwardThe factorial of a positive integer n —which we denote as n!—is the product of n and the factorial of n 1. The factorial of 0 is 1. Write two different recursive methods in Java that each return the factorial of n. Analyze your algorithm in Big-Oh notation and provide the appropriate analysis, ensuring that your program has a test class.arrow_forwardI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…arrow_forward
- Write a program called Recursive_fibonacci.java that implements a recursive function for computing the nth term of a Fibonacci Sequence. In the main method of your program accept the value of n from the user as a command-line argument and then call your function named Fibonacci with this value. The output should look exactly like what is pictured below.arrow_forwardsolve q5 only pleasearrow_forwardProvide JAVA source code with proper comments for attached assginment.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
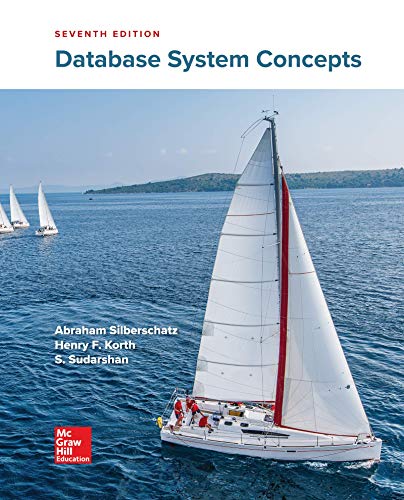
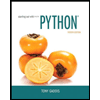
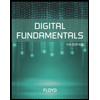
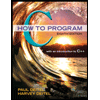
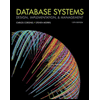
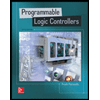