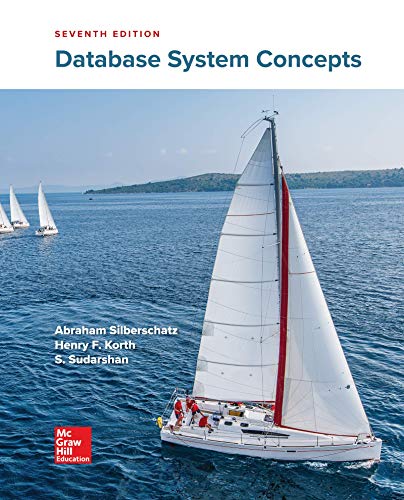
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Implement a Java method clone(ArrayList<String>) to make a deep clone of the ArrayList object.
class ListClone { public ArrayList<String> clone(ArrayList<String> list) { } }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- javaarrow_forward44 // create a loop that reads in the next int from the scanner to the intList 45 46 47 // call the ReverseArray function 48 49 50 System.out.print("Your output is: "); 51 // print the output from ReverseArray 52 ww 53 54 55 }arrow_forwardPlease answer the problem in the screenshot. Please use the methods below as a base. The language is in Java. import java.util.*; class HeapMax { // we go with arraylist instead of array for size flexibility private ArrayList<Integer> data; // DO NOT MODIFY THIS METHOD public HeapMax() { data = new ArrayList<Integer>(0); } // insert a new element and restore max heap property public void insert(int element) { } // return max public int getMax() { // remove this line return 0; } // remove max and restore max heap property public int removeMax() { // remove this line return 0; } // heap builder public void build(int[] arr) { } // print out heap as instructed in the handout public void display() { } // you are welcome to add any supporting methods }arrow_forward
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardUsing Java, modify the doubly linked list class presented below so it works with generic types. Add the following methods drawn from the java.util.List interface:• void clear(): remove all elements from the list.• E get(int index): return the element at position index in the list.• E set(int index, E element): replace the element at the specified position with the specified element and return the previous element. Test your generic linked list class by processing a list of numbers of type double. ====================================/**The DLinkedList class implements a doubly Linked list. */class DLinkedList {/**The Node class stores a list element and a reference to the next node.*/private class Node{String value; // Value of a list elementNode next; // Next node in the listNode prev; // Previous element in the list/**Constructor. @param val The element to be stored in the node.@param n The reference to the successor node.@param p The reference to the predecessor node.*/ Node(String…arrow_forwardimport java.util.ArrayList;import java.util.Random; class Main { public static void main(String[] args) { Random rand = new Random(); ArrayList<Integer> a = new ArrayList<Integer>(); for(int i = 0; i < 100; i++) { a.add(rand.nextInt(100)); } print(a); System.out.println("----"); sort(a); print(a); // simplified Quicksort public static void sort(ArrayList<Integer> a) { if (a.size() <= 1) return; // Pick a Pivot int m = a.size() / 2; int pivot = a.get(m); } ArrayList<Integer> smaller = new Arraylist<Integer>(); ArrayList<Integer> same = new ArrayList<Integer>(); ArrayList<Integer> larger = new ArrayList<Integer>(); for (Integer x : a) { if (x < pivot) { smaller.add(x); } else if(x > pivot) { larger.add(x); } else { same.add(x); } } sort(smaller); sort(larger); a.clear(); a.addAll(smaller);…arrow_forward
- JAVAarrow_forwardIn java Write a method public static ArrayList<Integer> merge(ArrayList<Integer> a, ArrayList<Integer> b) that merges one array list with another. Ex: a = 1 2 3 4 5, b = 6 7 8 9 10 then merge returns 1 6 2 7 3 8 4 9 5 10arrow_forwardimport java.util.*; public class Main{ public static void main(String[] args) { Main m = new Main(); m.go(); } private void go() { List<Stadium> parks = new ArrayList<Stadium>(); parks.add(new Stadium("PNC Park", "Pittsburgh", 38362, true)); parks.add(new Stadium("Dodgers Stadium", "Los Angeles", 56000, true)); parks.add(new Stadium("Citizens Bank Park", "Philadelphia", 43035, false)); parks.add(new Stadium("Coors Field", "Denver", 50398, true)); parks.add(new Stadium("Yankee Stadium", "New York", 54251, false)); parks.add(new Stadium("AT&T Park", "San Francisco", 41915, true)); parks.add(new Stadium("Citi Field", "New York", 41922, false)); parks.add(new Stadium("Angels Stadium", "Los Angeles", 45050, true)); Collections.sort(parks, Stadium.ByKidZoneCityName.getInstance()); for (Stadium s : parks) System.out.println(s); }}…arrow_forward
- Fix the following method printEvenIndex so that it will print out the Integers at even indices of the passed-in ArrayList list. import java.util.*; public class Test1 { public static void printEvenIndex(ArrayList<Integer> list) { for (int i) { if (i % 2 == 1) { System.out.print(list.get(i) + ", "); } } } public static void main(String[] args) { //instantiate ArrayList and fill with Integers ArrayList<Integer> values = new ArrayList<Integer>(); int[] nums = {1, 5, 7, 9, -2, 3, 2}; for (int i = 0; i < nums.length; i ++) { values.add(nums[i]); } System.out.println("Expected Result:\t 1, 7, -2, 2,"); System.out.print("Your Result:\t\t "); printEvenIndex(values); } }arrow_forwardJavaarrow_forwardCode debug JAVA import java.util.ArrayList;public class ArrayList {public static ArrayList<Integer> reverse(ArrayList<Integer> list) {for (int i = 0; i < list.size(); i++) {System.out.println(list);}public static ArrayList<Integer> getReverse(ArrayList<Integer> list){for (int index = 0; index < list.size() / 2; index++) {int temp = list.get(index);list.set(index, list.get(list.size() - index - 1));//swaplist.set(list.size() - index - 1, temp); //swap}return list;}}public static void main(String[] args) {ArrayList<Integer> list = new ArrayList<>();list.add(1);list.add(2);list.add(3);System.out.println("Original elements : " + list);System.out.println("Reversed elements : " + getReverse(list));}}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
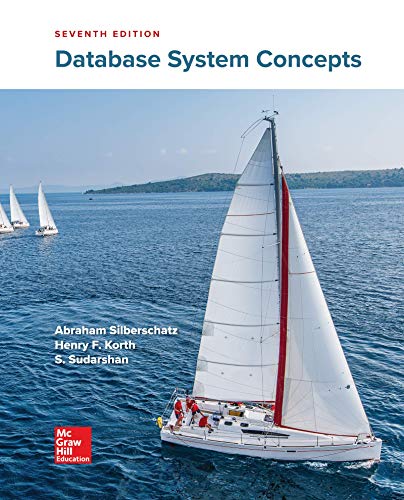
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
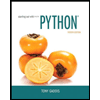
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
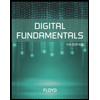
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
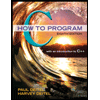
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
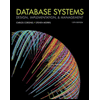
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
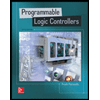
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education