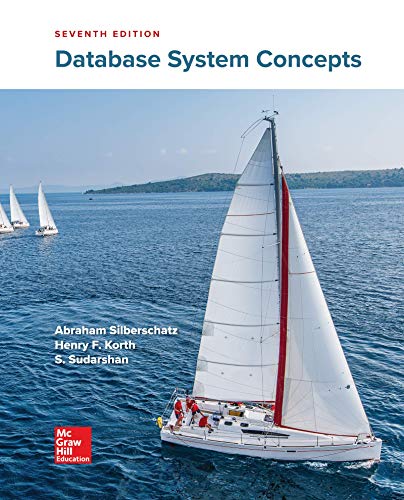
Concept explainers
Implement in C
9.9.1: LAB: Thesaurus
Given a set of text files containing synonyms for different words, complete the main program to output the synonyms for a specific word. Each text file contains synonyms for the word specified in the file’s name, and the synonyms within the file are grouped according to the synonyms' first letters, separated by an '*'. The program reads a word and a letter from the user and opens the text file associated with the input word. The program then stores the contents of the text file into a two-dimensional array of char* predefined in the program. Finally the program searches the array and outputs all the synonyms that begin with the input letter, one synonym per line, or a message if no synonyms that begin with the input letter are found.
Hints: Use the malloc() function to allocate memory for each of the synonyms stored in the array. A string always ends with a null character ('\0'). Use ASCII values to map the row index of the array to the first letter of a word when storing the synonyms into the array. Ex: Index 0 to an 'a', index 25 to a 'z'. Assume all letters are in lowercase.
Ex: If the input of the program is:
educate c
the program opens the file educate.txt, which contains:
brainwash brief * civilize coach cultivate * develop discipline drill * edify enlighten exercise explain * foster * improve indoctrinate inform instruct * mature * nurture * rear * school * train tutor *
then the program outputs:
civilize coach cultivate
Ex: If the input of the program is:
educate a
then the program outputs:
No synonyms for educate begin with a.
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(void) {
int NUM_CHARACTERS = 26; // Maximum number of letters
int MAX_SYNONYMS = 10; // Maximum number of synonyms per starting letter
int MAX_WORD_SIZE = 30; // Maximum length of the input word
int n;
char* synonyms[NUM_CHARACTERS][MAX_SYNONYMS]; // Declare 2D array of string pointers for all synonyms
// Initialize the first column of the 2D array
for (n = 0; n < NUM_CHARACTERS; n++) {
synonyms[n][0] = NULL;
}
/* Type your code here. */
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 2 images

- Using C++ Assume proper includes have been executed, but not using directive or declaration. Write a definition of an iterator for a vector named vec of int values. Write a for loop that will display the contents vec on the screen, separated by spaces. Use iterators for the loop control.arrow_forwardpython: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter, Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "Luna Lovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger, Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "James Potter, Gryffindor"])['Hermione Granger, Gryffindor',…arrow_forwardHello, can someone help me with this assignment? I am trying to do it on Python language: Develop a program that first reads in the name of an input file, followed by two strings representing the lower and upper bounds of a search range. The file should be read using the file.readlines() method. The input file contains a list of alphabetical, ten-letter strings, each on a separate line. Your program should output all strings from the list that are within that range (inclusive of the bounds). Ex: If the input is: input1.txt ammoniated millennium and the contents of input1.txt are: aspiration classified federation…arrow_forward
- Python please: Given the input file input1.csv write a program that first reads in the name of the input file and then reads the file using the csv.reader() method. The file contains a list of words separated by commas. Your program should output the words in a sorted list. The contents of the input1.csv are: hello,cat,man,hey,dog,boy,Hello,man,cat,woman,dog,Cat,hey,boy Example: If the input is input1.csv the output is: ['Cat', 'Hello', 'boy', 'boy', 'cat', 'cat', 'dog', 'dog', 'hello', 'hey', 'hey', 'man', 'man', 'woman'] Note: There is a newline at the end of the output.arrow_forwardpython: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter,Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "LunaLovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger,Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "JamesPotter, Gryffindor"])['Hermione Granger, Gryffindor', 'James…arrow_forwardPlease help. This is Python I feel so lostarrow_forward
- In c++ and without the use of vectors, please. Thanks very much! A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3…arrow_forwardWrite a Python program that calculates the average 5 quiz scores from a file with student ID and scores. Your program must have 2 functions; the main and calculateAvg- The main function reads data from an input file, which has the format shown below. Each line in the file starts with the Student ID followed by 5 values representing the scores attained by the Student in 5 quizzes. For each Student, the calculateAvg function receives a list containing the 5 values and returns another list containing all the values in the original list plus the average score of the quizzes for each student. This information will be written in an output file as shown below. Also, you must include the class average at the end. Which includes all the students in the list. Note: You program must be general and works for any number of Students. All reading and writing must be done in the main function. Assume each student has 5 quiz scores Input text file name must be grades.txt Output text file name must be…arrow_forwardIn c++ and please without the use of vectors. Thanks very much! A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use linear search. Modify the algorithm to output the count of how many comparisons were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Joe 123-5432 Linda 983-4123…arrow_forward
- plaese code in python You are tasked with creating a square grid of values in which each value only appears once in each column and once in each row (think like a Sudoku). Write a function called grid that inputs a list and outputs a square grid of values of that list in which each value only appears once in each column and once in each row. Specifically, one in which each row is the one above it shifted by one item. Your answer should be in the form of a list of lists, where each sub-list is a row of the square. For example: grid([1, 2, 3, 4]) -> [[1, 2, 3, 4], [4, 1, 2, 3], [3, 4, 1, 2], [2, 3, 4, 1]]arrow_forwardWrite a C++ Program using classes, functions (recursive and otherwise), arrays and other C++ commands to sort a file using Selection Sort. The user will enter the filename. The file should be sorted by the following fields: first by Last Name, then by Mother’s Maiden Name, then by First Name and finally by Second Name (or Initial). The sorted file should be written to file and the first 10 results displayed on screen. Use the following format for both the screen output and the file created: ----------------------------------------------------------------| Last Name | Mother’s Maiden Name | First Name | Second Name | ----------------------------------------------------------------| Saotome | Tendo | Ranma | P | |---------------------------------------------------------------| Son | Kakarot | Goku | A |----------------------------------------------------------------| Yeager | Kaiser | Eren…arrow_forwardUsing c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
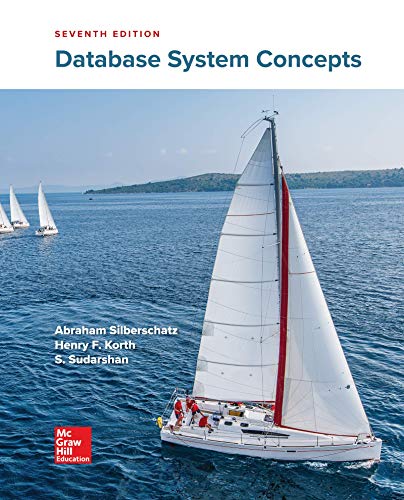
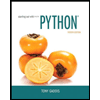
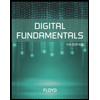
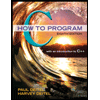
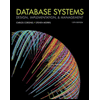
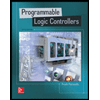