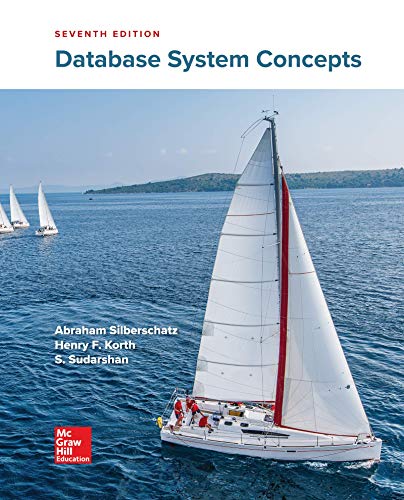
Concept explainers
Implement the RandomizedSet class:
RandomizedSet() Initializes the RandomizedSet object.
bool insert(int val) Inserts an item val into the set if not present. Returns true if the item was not present, false otherwise.
bool remove(int val) Removes an item val from the set if present. Returns true if the item was present, false otherwise.
int getRandom() Returns a random element from the current set of elements (it's guaranteed that at least one element exists when this method is called). Each element must have the same probability of being returned.
You must implement the functions of the class such that each function works in average O(1) time complexity.
Example 1:
Input
["RandomizedSet", "insert", "remove", "insert", "getRandom", "remove", "insert", "getRandom"]
[[], [1], [2], [2], [], [1], [2], []]
Output
[null, true, false, true, 2, true, false, 2]
Explanation
RandomizedSet randomizedSet = new RandomizedSet();
randomizedSet.insert(1); // Inserts 1 to the set. Returns true as 1 was inserted successfully.
randomizedSet.remove(2); // Returns false as 2 does not exist in the set.
randomizedSet.insert(2); // Inserts 2 to the set, returns true. Set now contains [1,2].
randomizedSet.getRandom(); // getRandom() should return either 1 or 2 randomly.
randomizedSet.remove(1); // Removes 1 from the set, returns true. Set now contains [2].
randomizedSet.insert(2); // 2 was already in the set, so return false.
randomizedSet.getRandom(); // Since 2 is the only number in the set, getRandom() will always return 2.
Please solve it in Java Language

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 2 images

- Heapsort has heapified an array to: 99 95 67 42 15 40 26 and is about to start the second for loop. What is the array after the first iteration of the second for loop? Ex: 98, 36, 41arrow_forwardAn element that comprises more than half of the items in an array is referred to as a majority element. Find the dominant element in a list of positive integers. Return -1 if there is no majority element. Execute this in 0(1) space and O(N) time.Input: 1 2 5 9 5 9 5 5 55 outputAn element that comprises more than half of the items in an array is referred to as a majority element. Find the dominant element in a list of positive integers. Return -1 if there is no majority element. Execute this in 0(1) space and O(N) time.Input: 1 2 5 9 5 9 5 5 55 outputarrow_forwardHello all! need some help with this assignment. Need the sample output as listed in the image. Thank you!arrow_forward
- Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10), print: 7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = NUM_VALS - 1. Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element array (int courseGrades[4]). Also note: If the submitted code tries to access an invalid array element, such as courseGrades[9] for a 4-element array, the test may generate strange results. Or the test may crash and report "Program end never reached", in which case the system doesn't print the test case that caused the reported message. 1 #include HNm in 10 m DO G 2 3 int main(void) { 4 5 6 const int NUM_VALS = 4; int courseGrades [NUM_VALS]; int i; 7 8 for (i = 0; i < NUM_VALS; ++i) { scanf("%d", &(courseGrades[i])); 9 } 10 11 12 13 14 15 } /*Your solution…arrow_forwardJava Complete the method with explaining, thank you!arrow_forwardimport random; #importing random module aGrades=[]; #list to store grades of all tests for i in range(0,7):aGrades.append(random.randrange(0,100)); #generating 7 random values for testsexamCount=len(aGrades)-1; #examCount is a variable to store count of all exams except last exam totalPoints=sum(aGrades)-aGrades[len(aGrades)-1]; #totalPoints is a variable to store sum of all scores excluding last exam testAverage=(totalPoints)/examCount; #testAverage is a variable used to store the average of scores except last exam finalAverage= (testAverage*0.6)+(aGrades[len(aGrades)-1]*0.4); #finalAverage is a variable to store weighted average of the tests print(aGrades); #printing list of gradesprint(testAverage); #printing test averageprint(finalAverage); #printing final average How do i rewrite the Grades List programs above using a loop instead of referencing the elements of the lists by index.arrow_forward
- Strings all_colors and updated_value are read from input. Perform the following tasks: Split all_colors into tokens using a comma (',') as the separator and assign color_list with the result. Replace the first element in color_list with updated_value.arrow_forwardCreate a class: “Question 1” with data members: 1D integer array of maximum size: 100, n(int). Create a dynamic constructor which takes input of n and n no. of array elements. Apartfrom taking input this class also displays the maximum and minimum elements from the givenarray elements.arrow_forwardTrue or False: If N represents the number of elements in the collection, then the contains() method of the ArrayCollection class is O(1) in the worst case.arrow_forward
- // Only few methods are mentioned here //Do remaining methods to pass the test methods /*** Removes the specified (num) of the given element from the internal ArrayList of elements. * If num <= 0, don't remove anything. * If num is too large, remove all instances of the given element from the internal ArrayList of elements.* Example:* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 1, 2, 1* - Calling remove(2, 1) would remove the first 2 instances of 1 * - The CustomIntegerArrayList will then contain the integers: 2, 2, 1* - For a defined CustomIntegerArrayList containing the integers: 100, 100, 100 * - Calling remove(4, 100) would remove all instances of 100 * - The CustomIntegerArrayList will then be empty* - For a defined CustomIntegerArrayList containing the integers: 5, 5, 5, 5, 5 * - Calling remove(0, 5) would remove nothing* * @param num number of instances of element to remove * @param element to remove*/ public void remove(int num, int element) {//…arrow_forwardWrite a hangman game that randomly generates a word andprompts the user to guess one letter at a time, as presented in the sample run.Each letter in the word is displayed as an asterisk. When the user makes a correctguess, the actual letter is then displayed. When the user finishes a word, displaythe number of misses and ask the user whether to continue to play with anotherword. Declare an array to store words, as follows:// Add any words you wish in this arrayString[] words = {"write", "that",...}; (Guess) Enter a letter in word ******* > p↵Enter(Guess) Enter a letter in word p****** > r↵Enter(Guess) Enter a letter in word pr**r** > p↵Enterp is already in the word(Guess) Enter a letter in word pr**r** > o↵Enter(Guess) Enter a letter in word pro*r** > g↵Enter(Guess) Enter a letter in word progr** > n↵Entern is not in the word(Guess) Enter a letter in word progr** > m↵Enter(Guess) Enter a letter in word progr*m > a↵EnterThe word is program. You missed 1 timeDo…arrow_forward( aList. insert ( i, item ) ).remove (i) = aListarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
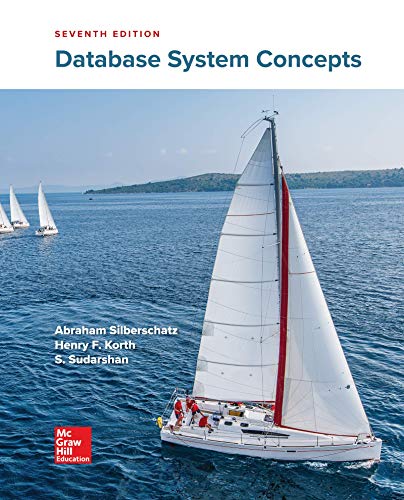
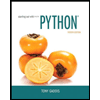
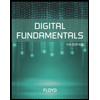
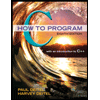
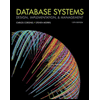
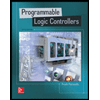