Implementing your own version of a stack. You will be using the List provided below (List.java) to create this stack. Use the StackAssignment.zip (as shown below). Please look at javadoc comments in StackInterface.java to understand what each method should be doing; DO NOT modify StackInterface.java. You have been provided with the class header for the stack in Stack.java, you WILL be modifying Stack.java to implement your stack. I have also included StackTester.java to help test your stack; DO NOT modify StackTester.java. You will need to create a new exception class "EmptyCollectionException" that extends "RuntimeException". Please read the javadoc comment for the pop() method in StackInterface.java for details. Testing: Use the provided StackTester.java to test your stack. Do NOT modify StackTester.java. When running StackTester.java, your should see the following output: List.java public class List { private T[] items = (T[]) new Object[10]; private int size = 0; public void add(T a) { if (items.length == this.size) { this.resize(); } this.items[size] = a; this.size++; } public void add(int index, T a) { if (index < this.size && index >= 0) { if (items.length == this.size) { this.resize(); } for (int i = this.size; i > index; i--) { this.items[i] = this.items[i - 1]; } this.items[index] = a; this.size++; } } public void remove(int index) { if (index < size && index >= 0) { for (int i = index; i < this.size - 1; i++) { this.items[i] = this.items[i + 1]; } size--; } } public void remove(T a) { for (int i = 0; i < this.size; i++) { if (this.items[i] == a) { this.remove(i); break; } } } public void set(int index, T a) { if (index < size && index >= 0) { this.items[index] = a; } } public T get(int index) { if (index < this.size && index >= 0) { return this.items[index]; } return null; } private void resize() { T[] newItems = (T[]) new Object[this.items.length * 2]; for (int i = 0; i < this.items.length; i++) { newItems[i] = this.items[i]; } this.items = newItems; } public int size() { return this.size; } } StackInterface.java (DO NOT MODIFY) public interface StackInterface> { /** * Adds an element to the top of the stack. * @param element the element to add to the stack */ void push(T element); /** * Removes and returns an element from the top of the stack. * If the stack is empty, throw EmptyCollectionException * @return the element from top of the stack. */ T pop() throws RuntimeException; /** * Returns an element from the top of the stack or null if the stack is empty. * @return the element from top of the stack. */ T peek(); /** * Returns true if the stack is empty else false. * @return true if the stack is empty. */ boolean isEmpty(); /** * Returns the number of elements in the stack. * @return size of the stack */ int size(); } StackTester.java (DO NOT MODIFY) public class StackTester { public static void main(String[] args) { Stack stack = new Stack<>(); stack.push(10); stack.push(24); System.out.println("\nThe stack has " + stack.size() + " elements."); System.out.println("\nThe contents of the stack are: " + getStackContents(stack)); System.out.println("\nThe stack has " + stack.size() + " elements."); stack.push(50); stack.push(80); stack.push(24); stack.push(95); stack.push(98); System.out.println("\nCalling stack.peek() returned: " + stack.peek()); System.out.println("\nThe stack has " + stack.size() + " elements."); stack.push(28); System.out.println("\nCalling stack.pop() returned: " + stack.pop()); System.out.println("\nThe contents of the stack are: " + getStackContents(stack) + "\n"); } private static String getStackContents(Stack stack) { var result = ""; while (stack.peek() != null) { result += stack.pop(); if (stack.peek() != null) { result += ", "; } } return result; } } Stack.java public class Stack> implements StackInterface { private int size = 0; private List list = new List(); // Implement your Stack here.. }
Implementing your own version of a stack. You will be using the List provided below (List.java) to create this stack.
Use the StackAssignment.zip (as shown below). Please look at javadoc comments in StackInterface.java to understand what each method should be doing; DO NOT modify StackInterface.java. You have been provided with the class header for the stack in Stack.java, you WILL be modifying Stack.java to implement your stack. I have also included StackTester.java to help test your stack; DO NOT modify StackTester.java.
You will need to create a new exception class "EmptyCollectionException" that extends "RuntimeException". Please read the javadoc comment for the pop() method in StackInterface.java for details.
Testing: Use the provided StackTester.java to test your stack. Do NOT modify StackTester.java. When running StackTester.java, your should see the following output:
List.java
public class List<T> {
private T[] items = (T[]) new Object[10];
private int size = 0;
public void add(T a) {
if (items.length == this.size) {
this.resize();
}
this.items[size] = a;
this.size++;
}
public void add(int index, T a) {
if (index < this.size && index >= 0) {
if (items.length == this.size) {
this.resize();
}
for (int i = this.size; i > index; i--) {
this.items[i] = this.items[i - 1];
}
this.items[index] = a;
this.size++;
}
}
public void remove(int index) {
if (index < size && index >= 0) {
for (int i = index; i < this.size - 1; i++) {
this.items[i] = this.items[i + 1];
}
size--;
}
}
public void remove(T a) {
for (int i = 0; i < this.size; i++) {
if (this.items[i] == a) {
this.remove(i);
break;
}
}
}
public void set(int index, T a) {
if (index < size && index >= 0) {
this.items[index] = a;
}
}
public T get(int index) {
if (index < this.size && index >= 0) {
return this.items[index];
}
return null;
}
private void resize() {
T[] newItems = (T[]) new Object[this.items.length * 2];
for (int i = 0; i < this.items.length; i++) {
newItems[i] = this.items[i];
}
this.items = newItems;
}
public int size() {
return this.size;
}
}
StackInterface.java (DO NOT MODIFY)
public interface StackInterface<T extends Comparable<T>> {
/**
* Adds an element to the top of the stack.
* @param element the element to add to the stack
*/
void push(T element);
/**
* Removes and returns an element from the top of the stack.
* If the stack is empty, throw EmptyCollectionException
* @return the element from top of the stack.
*/
T pop() throws RuntimeException;
/**
* Returns an element from the top of the stack or null if the stack is empty.
* @return the element from top of the stack.
*/
T peek();
/**
* Returns true if the stack is empty else false.
* @return true if the stack is empty.
*/
boolean isEmpty();
/**
* Returns the number of elements in the stack.
* @return size of the stack
*/
int size();
}
StackTester.java (DO NOT MODIFY)
public class StackTester {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
stack.push(10);
stack.push(24);
System.out.println("\nThe stack has " + stack.size() + " elements.");
System.out.println("\nThe contents of the stack are: " + getStackContents(stack));
System.out.println("\nThe stack has " + stack.size() + " elements.");
stack.push(50);
stack.push(80);
stack.push(24);
stack.push(95);
stack.push(98);
System.out.println("\nCalling stack.peek() returned: " + stack.peek());
System.out.println("\nThe stack has " + stack.size() + " elements.");
stack.push(28);
System.out.println("\nCalling stack.pop() returned: " + stack.pop());
System.out.println("\nThe contents of the stack are: " + getStackContents(stack) + "\n");
}
private static String getStackContents(Stack<Integer> stack) {
var result = "";
while (stack.peek() != null) {
result += stack.pop();
if (stack.peek() != null) {
result += ", ";
}
}
return result;
}
}
Stack.java
public class Stack<T extends Comparable<T>> implements StackInterface<T> {
private int size = 0;
private List<T> list = new List<T>();
// Implement your Stack here..
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

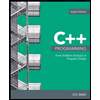
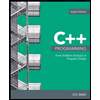