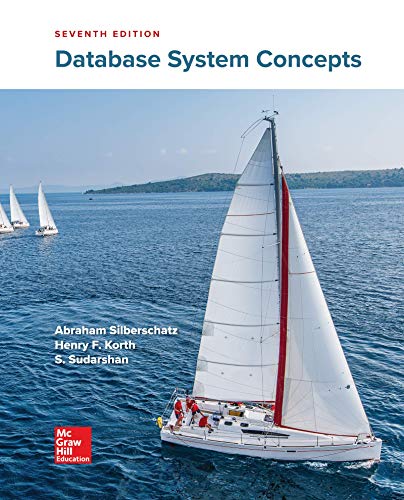
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
![1 import java.util.Random;
2
3
4
5
6-
7
8
9
10
11
12
▼
import java.util.StringJoiner;
public class preLabC {
public static MathVector myMethod(int[] testValues) {
// Create an object of type "MathVector".
// return the Math Vector Object back to the testing script.
return VectorObject;
13
14
15 }
}
▸ Comments
Description
The MathVector.java file contains a class (Math Vector). This class allows you to create objects in your program that
can contain vectors (a 1D array) and perform actions on those vectors. This is useful for doing math operations like
you would in your linear algebra class.
Your method should evaluate against two tests.
What do you need to do in this exercise? You need to create an "instance" of the MathVector object, VectorObject,
in your method. If you do this correctly, your method will accept a 1D array of integers, testValues, feed it into the
VectorObject and then return the VectorObject.
If you look in the file MathVector.java you'll see that this is one way in which MathVector objects can be created
(constructed):
24
25
26
27
28
29 ▼
30
31 -
32
/**
* Creates a MathVector instance backed by the given array.
*
* @param source the array to use
*/
public MathVector(int[] source) {
this.array = source;
}](https://content.bartleby.com/qna-images/question/cfd79b32-4de5-49e8-861a-3fd719d02c63/8885cd42-2469-4ad1-a2f5-7f6a28c3d998/z56n3nt_thumbnail.png)
Transcribed Image Text:1 import java.util.Random;
2
3
4
5
6-
7
8
9
10
11
12
▼
import java.util.StringJoiner;
public class preLabC {
public static MathVector myMethod(int[] testValues) {
// Create an object of type "MathVector".
// return the Math Vector Object back to the testing script.
return VectorObject;
13
14
15 }
}
▸ Comments
Description
The MathVector.java file contains a class (Math Vector). This class allows you to create objects in your program that
can contain vectors (a 1D array) and perform actions on those vectors. This is useful for doing math operations like
you would in your linear algebra class.
Your method should evaluate against two tests.
What do you need to do in this exercise? You need to create an "instance" of the MathVector object, VectorObject,
in your method. If you do this correctly, your method will accept a 1D array of integers, testValues, feed it into the
VectorObject and then return the VectorObject.
If you look in the file MathVector.java you'll see that this is one way in which MathVector objects can be created
(constructed):
24
25
26
27
28
29 ▼
30
31 -
32
/**
* Creates a MathVector instance backed by the given array.
*
* @param source the array to use
*/
public MathVector(int[] source) {
this.array = source;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I got another question. What would you do for this?
![preLabC.java
1 import java.util.Random;
2 import java.util.StringJoiner;
3
4- public class preLabC {
5
6-
7
8
9
10
11
12
13
14
15
16
17
18
19
20 }
public static String myMethod(MathVector inputVector) {
String vectorStringValue = new String(); // empty String object
System.out.println("here is the contents object, inputVector: + inputVector);
// get a String out of that MathVector object. Do it here. On the next line.
// return the double value here.
return vectorStringValue;
"1
▸ Compilation
Description
A MathVector object will be passed to your method. Return its contents as a String.
If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector
object as a String. This makes it useful for displaying to the user.
/**
* Returns a String representation of this vector. The String should be in the format "[1, 2, 3]"
*
* @return a String representation of this vector
* @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method.
*/
@Override
public String toString() {
var sj = new StringJoiner(",", "[", "]");
for (var e: array) {
sj.add(String.value0f(e));
}
return sj.toString();
You might have noticed that there's an @override term there. That's because many objects already
have a "toString()" method associated with them... because Java was designed to include them by
default. Here, the override tells Java "I know, I know. You already have a toString() that you'd assign
here. But it's not good enough. Here's a better one for this particular kind of object." It's a little bit
like saying "Most humans have two legs. So, by default, I'll give everyone two legs. But sometimes we
override that and give no legs, or just one leg to a person. And sometimes we give them four so that
they can be a centaur!"
To use this in a println() method, just name your object. The toString() method will
be called implicitly. Or you can just write System.out.println("look! " +
myObject.toString());](https://content.bartleby.com/qna-images/question/cfd79b32-4de5-49e8-861a-3fd719d02c63/a6e99a85-ecb1-4bcb-97cb-991ddd746f2d/0jv0ihe_thumbnail.png)
Transcribed Image Text:preLabC.java
1 import java.util.Random;
2 import java.util.StringJoiner;
3
4- public class preLabC {
5
6-
7
8
9
10
11
12
13
14
15
16
17
18
19
20 }
public static String myMethod(MathVector inputVector) {
String vectorStringValue = new String(); // empty String object
System.out.println("here is the contents object, inputVector: + inputVector);
// get a String out of that MathVector object. Do it here. On the next line.
// return the double value here.
return vectorStringValue;
"1
▸ Compilation
Description
A MathVector object will be passed to your method. Return its contents as a String.
If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector
object as a String. This makes it useful for displaying to the user.
/**
* Returns a String representation of this vector. The String should be in the format "[1, 2, 3]"
*
* @return a String representation of this vector
* @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method.
*/
@Override
public String toString() {
var sj = new StringJoiner(",", "[", "]");
for (var e: array) {
sj.add(String.value0f(e));
}
return sj.toString();
You might have noticed that there's an @override term there. That's because many objects already
have a "toString()" method associated with them... because Java was designed to include them by
default. Here, the override tells Java "I know, I know. You already have a toString() that you'd assign
here. But it's not good enough. Here's a better one for this particular kind of object." It's a little bit
like saying "Most humans have two legs. So, by default, I'll give everyone two legs. But sometimes we
override that and give no legs, or just one leg to a person. And sometimes we give them four so that
they can be a centaur!"
To use this in a println() method, just name your object. The toString() method will
be called implicitly. Or you can just write System.out.println("look! " +
myObject.toString());
Solution
by Bartleby Expert
Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I got another question. What would you do for this?
![preLabC.java
1 import java.util.Random;
2 import java.util.StringJoiner;
3
4- public class preLabC {
5
6-
7
8
9
10
11
12
13
14
15
16
17
18
19
20 }
public static String myMethod(MathVector inputVector) {
String vectorStringValue = new String(); // empty String object
System.out.println("here is the contents object, inputVector: + inputVector);
// get a String out of that MathVector object. Do it here. On the next line.
// return the double value here.
return vectorStringValue;
"1
▸ Compilation
Description
A MathVector object will be passed to your method. Return its contents as a String.
If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector
object as a String. This makes it useful for displaying to the user.
/**
* Returns a String representation of this vector. The String should be in the format "[1, 2, 3]"
*
* @return a String representation of this vector
* @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method.
*/
@Override
public String toString() {
var sj = new StringJoiner(",", "[", "]");
for (var e: array) {
sj.add(String.value0f(e));
}
return sj.toString();
You might have noticed that there's an @override term there. That's because many objects already
have a "toString()" method associated with them... because Java was designed to include them by
default. Here, the override tells Java "I know, I know. You already have a toString() that you'd assign
here. But it's not good enough. Here's a better one for this particular kind of object." It's a little bit
like saying "Most humans have two legs. So, by default, I'll give everyone two legs. But sometimes we
override that and give no legs, or just one leg to a person. And sometimes we give them four so that
they can be a centaur!"
To use this in a println() method, just name your object. The toString() method will
be called implicitly. Or you can just write System.out.println("look! " +
myObject.toString());](https://content.bartleby.com/qna-images/question/cfd79b32-4de5-49e8-861a-3fd719d02c63/a6e99a85-ecb1-4bcb-97cb-991ddd746f2d/0jv0ihe_thumbnail.png)
Transcribed Image Text:preLabC.java
1 import java.util.Random;
2 import java.util.StringJoiner;
3
4- public class preLabC {
5
6-
7
8
9
10
11
12
13
14
15
16
17
18
19
20 }
public static String myMethod(MathVector inputVector) {
String vectorStringValue = new String(); // empty String object
System.out.println("here is the contents object, inputVector: + inputVector);
// get a String out of that MathVector object. Do it here. On the next line.
// return the double value here.
return vectorStringValue;
"1
▸ Compilation
Description
A MathVector object will be passed to your method. Return its contents as a String.
If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector
object as a String. This makes it useful for displaying to the user.
/**
* Returns a String representation of this vector. The String should be in the format "[1, 2, 3]"
*
* @return a String representation of this vector
* @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method.
*/
@Override
public String toString() {
var sj = new StringJoiner(",", "[", "]");
for (var e: array) {
sj.add(String.value0f(e));
}
return sj.toString();
You might have noticed that there's an @override term there. That's because many objects already
have a "toString()" method associated with them... because Java was designed to include them by
default. Here, the override tells Java "I know, I know. You already have a toString() that you'd assign
here. But it's not good enough. Here's a better one for this particular kind of object." It's a little bit
like saying "Most humans have two legs. So, by default, I'll give everyone two legs. But sometimes we
override that and give no legs, or just one leg to a person. And sometimes we give them four so that
they can be a centaur!"
To use this in a println() method, just name your object. The toString() method will
be called implicitly. Or you can just write System.out.println("look! " +
myObject.toString());
Solution
by Bartleby Expert
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 1. Square Roots Create a class with a method that, given an integer, returns an array of double-precision floating point numbers (known in Java as double), each of which is a square root of a number between 2 and the parameter to the method. For example, if the parameter is 5, the method should return an array of 4 doubles, with approximate values 1.4142135623, 1.7320508075, 2.0, and 2.2360679774. Your method should check the validity of the parameter, and take appropriate action if the parameter is invalid. 2. Reading Files Create a class with a method that, given a string representing a file name, returns an integer with the number of characters in the file. For example, if the file has 10 characters, your method must return the number 10. 3. Main Class Create a class HW1.java with a main method that does both of the following: calls the method from part 1 with a random integer between 0 and 10, and prints each of the numbers in the result. The numbers should all be printed on the…arrow_forwardThe provided file has syntax and/or logical errors. Determine the problem and fix the program.arrow_forwardcan i get answer plzarrow_forward
- JavaDriver Program Write a driver program that contains a main method and two other methods. Create an Array of Objects In the main method, create an array of Food that holds at least two foods and at least two vegetables. You can decide what data to use. Method: Print Write a method that prints the text representation of the foods in the array in reverse order (meaning from the end of the array to the beginning). Invoke this method from main. Method: Highest Sugar Food Write a method that takes an array of Food objects and finds the Food that has the most sugar. Note that the method is finding the Food, not the grams of sugar. Hint: in looping to find the food, keep track of both the current highest index and value. Invoke this method from main.arrow_forwardprivate Vector location;private Vector velocity;private Vector acceleration;private int lifespan;private static final int MAX_LIFESPAN = 25;private static final int RADIUS = 7; public boolean isAlive() { } public void update() {//code here} Using java, if particle is alive then add velocity vector to location vector, add acceleration vector to velocity vector, and decrement lifespan by 1. Thank you.arrow_forwardAjva.arrow_forward
- JAVA PROGRAM Chapter 7. PC #1. Rainfall Class Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: • the total rainfall for the year • the average monthly rainfall • the month with the most rain • the month with the least rain Demonstrate the class in a complete program. Main class name: RainFall (no package name) est Case 1 Enter the rainfall amount for month 1:\n1.2ENTEREnter the rainfall amount for month 2:\n2.3ENTEREnter the rainfall amount for month 3:\n3.4ENTEREnter the rainfall amount for month 4:\n5.1ENTEREnter the rainfall amount for month 5:\n1.7ENTEREnter the rainfall amount for month 6:\n6.5ENTEREnter the rainfall amount for month 7:\n2.5ENTEREnter the rainfall amount for month 8:\n3.3ENTEREnter the rainfall amount for month 9:\n1.1ENTEREnter the rainfall amount for month 10:\n5.5ENTEREnter the rainfall amount for month 11:\n6.6ENTEREnter…arrow_forwardusing System; class main { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { int i; Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo[i]); } } } } how can this program be fixed to count from 1-88 and then choose 3 different random numbers?arrow_forwardDesign a class named CustomerRecord that holds a customer number, name, and address. Include separate methods to 'set' the value for each data field using the parameter being passed. Include separate methods to 'get' the value for each data field, i.e. "return" the field's value. Pseudocode:arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
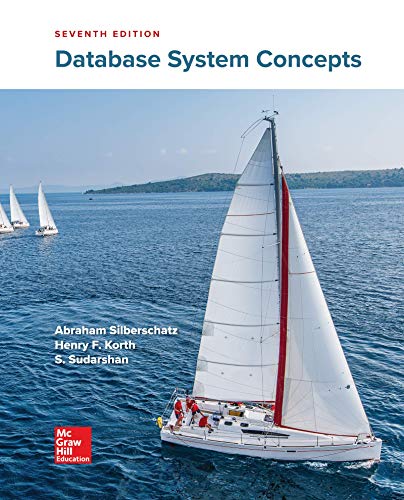
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
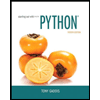
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
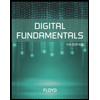
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
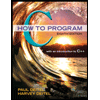
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
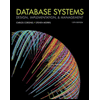
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
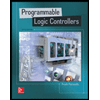
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education