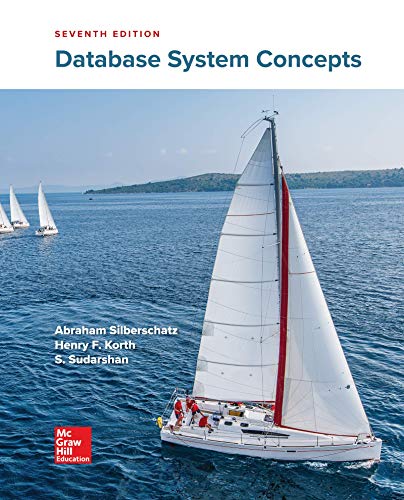
Must attach output screenshot, editable output
In java
Implement a generic min heap class and write a test code to test the program. Use hashmap for the update method. You can also use it for the getIndex method.
public int getIndex(AnyType x) {
//average case O(1)
//returns the index of the item in the heap
//or -1 if it isn't in the heap
//return -1;
}
public boolean update(AnyType x) {
//O(lg n) average case
//or O(lg n) worst case if getIndex() is guarenteed O(1)
We want an update() operation which increases (or decreases) the priority of the item. The item (or an equal item) will be provided and it should update the priority queue appropriately.
The only way to do this efficiently is to use a map to map heap items to indexes so that you know where to start the update (in average case O(1) time). Without this, update() would be O(n) and not O(lg n). Therefore before you implement updating, you need to integrate a map into the code. Whenever an item is placed, moved, or removed from the heap, the map should updated to reflect the item's new index (or remove it from the map in the case of removal from the heap).
return false; //dummy return

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Implement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 3. Define TestSLinkedList Classa. Declare an instance of the Single List class.b. Test all the methods of the Single List class.arrow_forwardWrite a recursive instance method isSorted that takes a Link parameter and determines whether a linked list is sorted in descending order or not (return a boolean value).arrow_forward
- Add the following method in the BST class that returns aniterator for traversing the elements in a BST in preorder./** Return an iterator for traversing the elements in preorder */java.util.Iterator<E> preorderIterator()arrow_forwardThis is Java programming! The current code for BstMaxHeap is printing names backwords. So please modify the code BstMaxHeap.java so it prints as the following: The heap is not empty; it contains 9 entries. The largest entry is Whitney Removing entries in descending order: Removing Whitney Removing Regis Removing Megan Removing Matt Removing Jim Removing Jared Removing Doug Removing Brittany Removing Brettarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
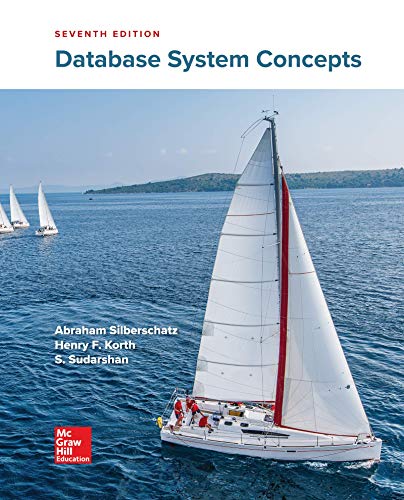
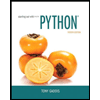
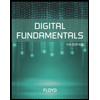
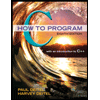
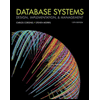
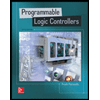