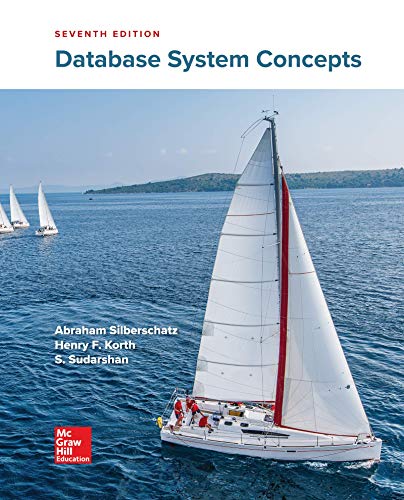
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
#include <iostream>
using namespace std;
class Player
{
private:
int id;
static int next_id;
public:
int getID() { return id; }
Player() { id = next_id++; }
};
int Player::next_id = 1;
int main()
{
Player p1;
Player p2;
Player p3;
cout << p1.getID() << " ";
cout << p2.getID() << " ";
cout << p3.getID();
return 0;
}
Run the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- Network Class The Network class represents a network of people that are connected to each other and are able to contact and send messages to each other through the network. Create a Network class with the following: Member Variables A Network has just one private member variable: phonebook_ - a std::map which maps from a std::string for a person's name, to the std::shared_ptr<Phone> object that belongs to that person. Constructor You do not need to explicitly define a constructor. The default constructor will implicitly be created for us by the compiler, initializing the phonebook_ to an empty map. AddPhone Create a function AddPhone that accepts a std::shared_ptr to a Phone and inserts that Phone to the phonebook_. The key is the name of that phone's owner, and the value is the shared pointer to the Phone. SendMessage Create a function SendMessage that accepts a std::shared_ptr to a Message and a const reference to a std::string representing the intended recipient of this…arrow_forwardDate class to use: #ifndef DATE_H_ #define DATE_H_ #include <iostream> #include <iomanip> using namespace std; class Date { friend ostream &operator<<( ostream &, const Date & ); private: int day; int month; int year; static const int days[]; // array of days per month void helpIncrement(); // utility function for incrementing date public: Date(int=1, int=1, int=0); void setDate(int,int,int); bool leapYear( int ) const; // is date in a leap year? bool endOfMonth( int ) const; // is date at the end of month? Date &operator++(); // prefix increment operator Date operator++( int ); // postfix increment operator const Date &operator+=( int ); // add days, modify object bool operator<(const Date&) const; void showdate(); }; const int Date::days[] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 }; Date::Date(int d, int m, int y) { day = d; month = m; year = y; // initialize static member at file scope; one classwide copy } // set month,…arrow_forwardclass Airplane – highlights -- planeID : String -planeModel: String //”Boeing 757” etc. --seatCapacity : int //would vary by outfitting ………… Assume getters and setters, toString(), constructor with parameters and constructor ……………… Write lambdas using a standard functional interface to: a) Given a plane object and a required number of seats as an input, determine if the given plane meets or exceeds the required capacity b) Output the plane description if capacity is less than 80% of a specified number (We would use his for planning aircraft assigned to various routes. ) c) Adjust the seats in a plane by a specified positive or negative number [after modifications have been made to the interior.] …and write an example for each showing the usage, using an Airplane instance plane1arrow_forward
- Take the following code: class Song { private: string name; string author; string genre; int year_released; public: Song() { /* initialize attributes */} string get_name() { return name; } void set_name(string new_name) { name = new_name; } string get_author() { return author; } void set_author(string new_author) { author = new_author; } int get_age(int curr_year) { return curr_year - year_released; } void set_year_released(int new_year) { year_released = new_year ; } }; indicate the setters of this class. Edit View Insert Format Tools Table 12pt v Paragraph v B IUAarrow_forwardC++arrow_forward#include <iostream> using namespace std; class Player { private: int id; static int next_id; public: int getID() { return id; } Player() { id = next_id++; } }; int Player::next_id = 1; int main() { Player p1; Player p2; Player p3; cout << p1.getID() << " "; cout << p2.getID() << " "; cout << p3.getID(); return 0; } Run the program and give its output.arrow_forward
- class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const; error: expected initializer before 'sequence' 125 | sequence<Item>value_type sequence ::current() const { | ^~~~~~~~arrow_forwardVigenere.h #include<string>using namespace std;//Create class Vigenereclass Vigenere {//Membersprivate:string key;//Functionspublic://ConstructorVigenere();//Setter and gettervoid setKey(string key);string getKey();//Convert into upper casestring toUpperCase(string k);//Encryptstring encrypt(string word);//Decryptstring decrypt(string word);}; Vigenere.cpp //Implementation#include "Vigenere.h"//ConstructorVigenere::Vigenere() { this->key = "";}//Setter and gettervoid Vigenere::setKey(string key) { this->key = key;}string Vigenere::getKey() { return key;}//Convert into upper casestring Vigenere::toUpperCase(string k) { for (int i = 0; i < k.length(); i++) { if (isalpha(k[i])) { k[i] = toupper(k[i]); } } return k;}//Encryptstring Vigenere::encrypt(string word) { return key; string output = ""; for (int i = 0, j = 0; i < word.length(); i++) { char c = word[i]; if (c >= 'a' && c <= 'z') { c += 'A'…arrow_forward#include <iostream> #include <iomanip> double double showMenu(); double takePurchase(double); double takePayment(); double takePayment(); void displayInfo(double, double); double showMenu() {} double takePayment() { double takePurchase() { } double takePurchase (double) { } double takePurchase(double price) { } double takePayment() {} displayInfo (double purchase, double payment) void displayInfo (double purchase, double payment){} int quantity; return (price * quantity);arrow_forward
- namespace CS3358_FA2022_A04_sequenceOfNum{template <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;bool is_item();};} error: invalid use of template-name 'CS3358_FA2022_A04_sequenceOfNum::sequence' without an argument list 120 | sequence::size_type sequence<Item>::size() const { | ^~~~~~~~arrow_forwardinterface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
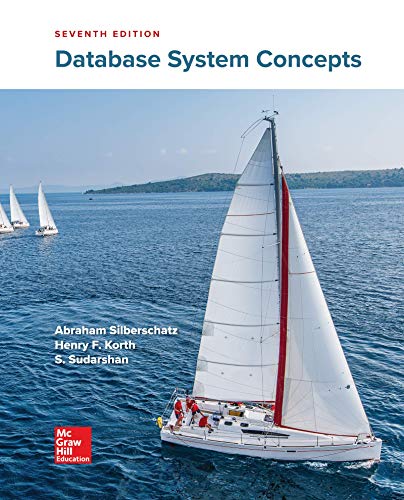
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
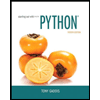
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
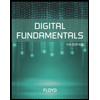
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
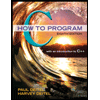
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
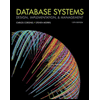
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
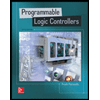
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education