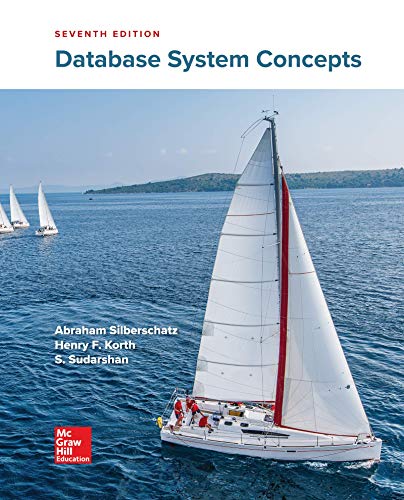
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
#include<bits/stdc++.h>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int binarySearch(int arr[], int l, int r, int x, int& comp) {
comp++;
if (r >= l) {
int mid = l + (r - l) / 2;
if (arr[mid] == x) {
return mid;
}
if (arr[mid] > x) {
return binarySearch(arr, l, mid - 1, x, comp);
}
return binarySearch(arr, mid + 1, r, x, comp);
}
return -1;
}
int main() {
int Num[8192];
srand(time(NULL));
for (int i = 0; i < 8192; i++) {
Num[i] = rand() % 10001;
}
clock_t starting_time = clock();
bubbleSort(Num, 8192);
clock_t ending_time = clock();
clock_t result = ending_time - starting_time;
print_Title();
cout << setprecision(2) << fixed;
cout << "Bubble sort takes " << (double)result/CLOCKS_PER_SEC << " seconds. \n";
cout << "Bubble sort takes " << (double)result << " milliseconds. \n\n";
int x = 71, comparisons = 0;
int index = binarySearch(Num, 0, 8191, x, comparisons);
if (index != -1) {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - found." << endl;
}
else {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - not found." << endl;
}
x = 9997; comparisons = 0;
index = binarySearch(Num, 0, 8191, x, comparisons);
if (index != -1) {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - found." << endl;
}
else {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - not found." << endl;
}
x = 5000; comparisons = 0;
index = binarySearch(Num, 0, 8191, x, comparisons);
if (index != -1) {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - found." << endl;
}
else {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - not found." << endl;
}
x = 2; comparisons = 0;
index = binarySearch(Num, 0, 8191, x, comparisons);
if (index != -1) {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - found." << endl;
}
else {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - not found." << endl;
}
x = 3015; comparisons = 0;
index = binarySearch(Num, 0, 8191, x, comparisons);
if (index != -1) {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - found." << endl;
}
else {
cout << "Binary search " << x << " takes " << comparisons << " comparisons - not found." << endl;
}
return 0;
}
implement a selection sort function that measures the time in seconds and milliseconds like how bubble sort was done.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- #include <iostream>using namespace std;class test{public:test(){cout<<"alpha\n";}~test(){cout<<"beta\n";}};int main(int argc, char const *argv[]){test *a = new test[5];delete[] a;return 0;} Give output.arrow_forward#include <iostream>using namespace std;class test{public:test(){cout<<"alpha\n";}~test(){cout<<"beta\n";}};int main(int argc, char const *argv[]){test *a = new test[5];delete[] a;return 0;} Give output.arrow_forward) Consider the following C code snippet. void swap(int *xp, int *yp) { int temp = *xp; *xp = *yp; *yp = temp; } int findMinimum(int arr[], int N) { // variable to store the index of minimum element int min_idx = 0; int min_E = arr[min_idx]; // Traverse the given array for (int i = 1; i < N; i++) { // If current element is smaller than min_idx then update it if (arr[i] < min_E) { min_idx = i; min_E = arr[min_idx]; } } return min_idx; } /* Function to sort an array using selection sort*/ void selectionSort(int arr[], int n) { int i, min_idx; // One by one move boundary of unsorted subarray for (i = 0; i < n-1; i++) { // Find the minimum element in unsorted array min_idx = findMinimum(&arr[i], n-i); // Swap the found minimum element with the first element if(min_idx != 0) swap(&arr[min_idx+i], &arr[i]); } }…arrow_forward
- In C++ whats the answer from below ? int size = 10; for ( int i = -1; i < size ; i++) { arr[size - i] = i; } This array: has 12 positions is out of bounds has 10 positions has 11 positionsarrow_forward#include <iostream>using namespace std; char* duplicateWithoutBlanks(char *word){int len = sizeof(word); char *new_str = new char[len + 1]; int k = 0; for (int i = 0; i < len; ++i){if (word[i] != ' ')new_str[k++] = word[i];} new_str[k] = '\0'; return new_str;} int main(){// Testing code char word[] = "Hello, World!"; char* result = duplicateWithoutBlanks(word); cout<< "word: "<< word<< "\nResult: "<< result; ; return 0;} What to change to out put the same word without blank?arrow_forwardJava Programming language Please help me with this questionarrow_forward
- ii) In JAVA language input the elements of an integer array A of size 10 and find the count of all hills in the array. A[i] is a Hill if A[i-1]A[i+1]. A[i] >= 0 All elements of the array are unique.arrow_forwardJava Programming language Please help me with this questionarrow_forward#include<bits/stdc++.h>using namespace std;bool isPalindrome(string &s){ int start=0; int end=s.length()-1; while(start<=end) { if(s[start]!=s[end]) { return false; } start++; end--; } return true;}int main(){ string s; cout<<"ENTER STRING:"; getline(cin,s); int n=s.length(); bool flag=true; for(int i=1;i<n;i++) { string lowerHalf=s.substr(0,i); string upperHalf=s.substr(i,n-i); if(isPalindrome(lowerHalf) && isPalindrome(upperHalf)) { flag=false; cout<<"String A is:"<<lowerHalf<<"\n"; cout<<"String B is:"<<upperHalf<<"\n"; break; } } if(flag) { cout<<"NO\n"; } return 0;} change to stdio.h string.harrow_forward
- Find error #include<bits/stdc++.h> using namespace std; class Solution{ public: bool isPossible(vector<int>ank,int n,int mid) { int count=0; for(int i=0;i<rank.size()) { int val= (-1 + sqrt(1+(8*mid)/rank[i]))/2; count+=val; } return count>=n; } int findMinTime(int N, vector<int>&A, int L){ int low=*min_element(A.end()),high=1000000; int ans=high; while(low<=high) { int mid=low+(high)/2; if(isPossible(A,mid)) { ans=mid; high=mid; } else low=mid; } return ans; } }; int main() { int t; cin>>t; while(t--) { int l; cin >> l; vector<int>arr(l); for(int i = 0; i < l; i++){ cin >> arr[i]; } Solution ob; int ans = ob.findMin(n, *arr, l); cout…arrow_forwarddef ppv(tp, fp): # TODO 1 return def TEST_ppv(): ppv_score = ppv(tp=100, fp=3) todo_check([ (np.isclose(ppv_score,0.9708, rtol=.01),"ppv_score is incorrect") ]) TEST_ppv() garbage_collect(['TEST_ppv'])arrow_forwardJava (ArrayIndexOutOfBoundsException)Write a program that meets the following requirement Create an array with 100 randomly chosen integers Prompts the user to enter the index of then displays the corresponding element value. If the specified index is out bounds display the message Out of Bounds.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
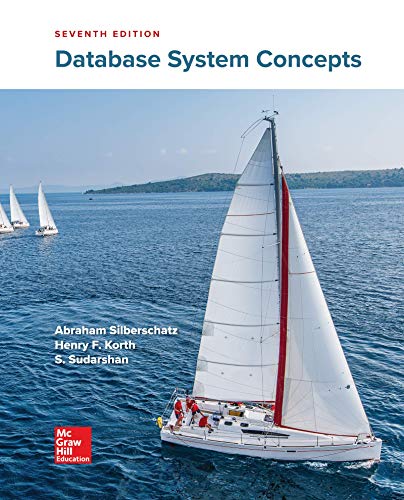
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
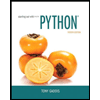
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
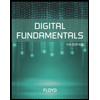
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
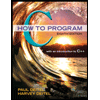
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
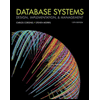
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
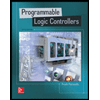
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education