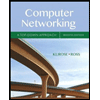
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
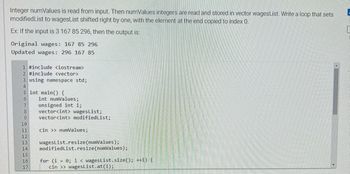
Transcribed Image Text:Integer numValues is read from input. Then numValues integers are read and stored in vector wagesList. Write a loop that sets
modified List to wagesList shifted right by one, with the element at the end copied to index 0.
Ex: If the input is 3 167 85 296, then the output is:
Original wages: 167 85 296
Updated wages: 296 167 85
1 #include <iostream>
2 #include <vector>
3 using namespace std;
4
5 int main() {
6
int numValues;
7
8
unsigned int i;
vector<int> wagesList;
9 vector<int> modifiedList;
10
11
12
13 wagesList.resize(numValues);
modifiedList.resize(numValues);
14
15
16
17
cin >> numValues;
for (i = 0; i < wagesList.size(); ++i) {
cin>> wagesList.at(i);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

Knowledge Booster
Similar questions
- C++arrow_forwardUsing C Sharp, Declare an array animals with 5 animals in it such as “dog”, “cat”, etc. After the declaration write the code to add another animal to the array. Write the code to add each animal to a list box called lstAnimals.arrow_forwardIntegers are read from input and stored into a vector until -1 is read. Output the negative elements in the vector in reverse order. End each number with a newline. Ex: If the input is -8 15 1 -12 -1, then the output is: -12 -8 Note: Negative integers are less than 0. 3 using namespace std; 4 5 int main() { 6 7 8 9 10 11 12 13 14 15 16 17 18 19 } vector dataVect; int value; int i; cin >> value; while (value != -1) { dataVect.push_back(value); cin >> value; /* Your code goes here */ return 0;arrow_forward
- static List<String> findWinners(String pattern, int minLength, boolean even, Stream<String> stream) This function will receive a stream of strings and it will return a sorted list of strings that meet all of the following criteria: They must contain the pattern The strings length must be equal to or greater than the min length number The strings length must be an even or odd number This Function must take no longer 10000 milliseconds to return. pattern - A pattern that all the strings in the list returned must containminLength - The length that all the strings in the list returned must be equal to or greater thaneven - True if even false if odd. Denotes a condition that all the strings in the list returned must have a length that is even or odd.stream - A stream of strings to be filteredreturn - A list of strings that meets the criteria outlined above sorted by their length from small to largearrow_forwardJS Write a function named count_in_range that takes a list/array of decimal numbers as a parameter. The function must return the number of entries in the parameter whose value is between 16.67 and 47.61. Your count should NOT include any entries whose value is equal to 16.67 or 47.61.arrow_forwardstruct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forward
- T/F: Binary Search can correctly determine whether an element is in an array if it is unsorted.arrow_forwardt. Given a string as a parameter to the function, return a square grid filled with all the characters of the string from left to right. Fill empty spaces in the grid with '_'. Sample Input: stringtogrid Output: {{s, t, r, i}, {n, g, t, o}, {g, r, i, d}, {_, _, _, _}} Complete the function: vector<vector<int> formGrid(string s){}arrow_forwardWrite a user defined function named as “length_list” function that finds the length of a list of list_node_t nodes. This code should fit with the following code.Partial Code: #include <stdio.h> #include <stdlib.h> /* gives access to malloc */ #define SENT -1 typedef struct list_node_s { int digit; struct list_node_s *restp; } list_node_t; list_node_t *get_list(void); int main(void) { list_node_t *x;int y; x=get_list(); printf("%d\n",x->digit); printf("%d\n",x->restp->digit); printf("%d\n",x->restp->restp->digit); // your length_list function should be called from here printf("Length is: %d",y); } /* * Forms a linked list of an input list of integers * terminated by SENT */ list_node_t * get_list(void) { int data; list_node_t *ansp; [20]scanf("%d", &data); if (data == SENT) { ansp = NULL; } else { ansp = (list_node_t *)malloc(sizeof (list_node_t)); ansp->digit = data; ansp->restp = get_list(); } return (ansp);arrow_forward
- Write a for loop to print all NUM_VALS elements of vector courseGrades, following each with a space (including the last). Print forwards, then backwards. End with newline. Ex: If courseGrades = {7, 9, 11, 10}, print:7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = courseGrades.size() - 1 (Notes)C++ without using std:: Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element vector (vector<int> courseGrades(4)). See "How to Use zyBooks".Also note: If the submitted code has errors, the test may generate strange results. Or the test may crash and report "Program end never reached", in which case the system doesn't print the test case that caused the reported message. #include <iostream>#include <vector>using namespace std; int main() { const int NUM_VALS = 4; vector<int> courseGrades(NUM_VALS); int i; for (i = 0; i < courseGrades.size(); ++i) { cin >>…arrow_forwardinitial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forwardExercise - Collection Functions Using the code below, use the map function to create an array of Int values, whose values are equal to the original integer value, plus 1. Use $0 as you iterate through the values of the array. Print the resulting collection. 5 let testScores = [65, 80, 88, 90, 47] Using the code below, use the filter function to create a new array of String values. The new array should only include Strings longer than four characters. Use $0 as you iterate through the values of the array. Print the resulting collection. Olet schoolSubjects = ["Math" , "Computer Science", "Gym", "English", "Biology"] Using the code below, use the reduce function to subtract all of the values within the array from the starting value 100. Print the resulting value. let damageTaken = [25, 10, 15, 30, 20]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
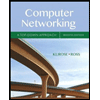
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
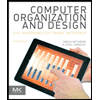
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
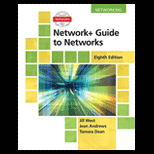
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
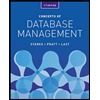
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
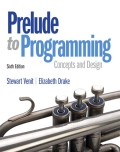
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
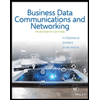
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY