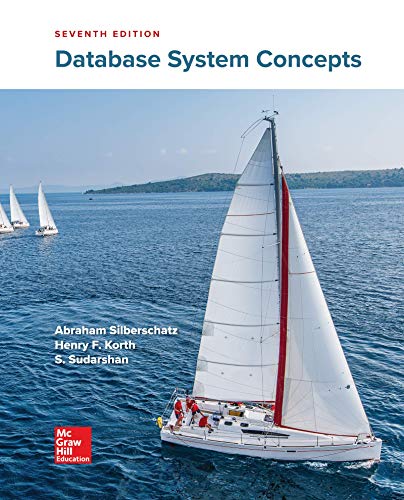
Concept explainers
C++
is my answer correct ?
- Write a function to convert a given string :
Answer:
#include <iostream>
#include <string>
using namespace std;
void toUpper(string &str)
{
for (int i = 0; i < str.length(); i++)
{
str[i] = toupper(str[i]);
}
}
void toLower(string &str)
{
for (int i = 0; i < str.length(); i++)
{
str[i] = tolower(str[i]);
}
}
int main()
{
string str = "khaled";
toUpper(str);
cout << str << endl;
str = "MOHAMMED";
toLower(str);
cout << str << endl;
return 0;
}
- Write a function to calculate the sum of the even number from 1 - 100.
Answer:
#include <iostream>
using namespace std;
int sumEven() {
int sum = 0;
for (int i = 1; i <= 100; i++) {
if (i % 2 == 0) {
sum += i;
}
}
return sum;
}
int main() {
cout << sumEven() << endl;
return 0;
}

Step by stepSolved in 2 steps with 2 images

- #include <iostream>#include <string.h>using namespace std;int index1(char *T,char *P){int t=0,p=0,i,j,r;t = strlen(T);p = strlen(P);i=0;int max = t - p + 1;while(i<max){for(j=0;j<p;j++){if(P[j]==T[i+j]){r = i;break;}else r = -1;}i++;}return r;} int main(){ int l;char c[100];char d[100];cout<<"Enter First String\n"; cin>>c;cout<<"Enter Second String\n";cin>>d;l = index1(c,d);cout<<l; return 0;} Note: Remove Funcationarrow_forwardC++languagearrow_forwardProgramming Language :- Carrow_forward
- C++ Programming 1arrow_forward//the language is c++ Implement function to tokenize a string. std::vector<std::string> tokenize(std::string& line) { } int main(){ std::string ts = "This is a string with no commas semicolons or fullstops"; std::vector<std::string> tok = tokenize(ts); for (auto x : tok) { std::cout<<x<<std::endl; } return 0; }arrow_forwardc++ coding language I need help with part B and C please. If you are unable to do both, then PLEASE prioritize part C. I am really stuck and really can use the help. This is the code for c that was provided in order to guide me: const int N =31; // N parking spaces bool parking[N]; // the garage void EmptyTheLot(bool parking[], int N) { for(int i=0; i<N; i++) p[i]=false; // empty space } // returns -1 if no space found, //otherwise it returns 0<=i<N for a valid space. int FindSpace(int PlateNumber, bool parking[], int N) { // ????? } main() { EmptyTheLot(parking, N); // start with an empty parking garage. // get plate numbers and fill lot. }arrow_forward
- in c++ i have this struct struct Student { string firstName, lastName; int pointTotal; }; void printStudent(const Student & s) { cout << s.pointTotal << "\t" << s.lastName << ", " << s.firstName << endl;}void printAll(Student students[], int numStudents) { for (int i = 0; i < numStudents; i++) printStudent(students[i]); cout << endl;}int main() { const int NUMSTUDENTS = 7; Student students[NUMSTUDENTS] = { {"Brian", "Jones", 45},{"Edith", "Piaf", 45},{"Jacques", "Brel", 64},{"Anna", "Brel", 64}, {"Carmen", "Jones", 45} , {"Carmen", "Brel", 64}, {"Antoine", "Piaf", 45}, {"Pascal", "Piaf", 64} }; printAll(students, NUMSTUDENTS); sortByPointTotal(students, NUMSTUDENTS); printAll(students, NUMSTUDENTS); return 0;} i need to make a function that uses bubble sort(coded from scratch), but modify it so that the comparison for out-of-order elements takes into account all three fields:…arrow_forwardC++ Given code is #pragma once#include <iostream>#include "ourvector.h"using namespace std; ourvector<int> intersect(ourvector<int> &v1, ourvector<int> &v2) { // TO DO: write this function return {};}arrow_forwardpalindromes Write a function palindromes that accepts a sentence as an argument. The function then returns a list of all words in the sentence that are palindromes, that is they are the same forwards and backwards. Guidelines: • punctuation characters .,;!? should be ignored • the palindrome check should not depend on case Sample usage: >>> palindromes ("Hey Anna, would you prefer to ride in a kayak or a racecar?") ['Anna', 'a', 'kayak', 'a', 'racecar'] >>> palindromes ("Able was I ere I saw Elba.") ['I', 'ere', 'I'] >>> palindromes ("Otto, go see Tacocat at the Civic Center, their guitar solos are Wow!") ['Otto', 'Tacocat', 'Civic', 'solos', 'wow'] >>> palindromes ("Otto, go see Tacocat at the Civic Center, their guitar solos are wow!")==['Otto', 'Tacocat', 'Civic', 'solos', 'wow'] Truearrow_forward
- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardC++ languagearrow_forwardC++languagearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
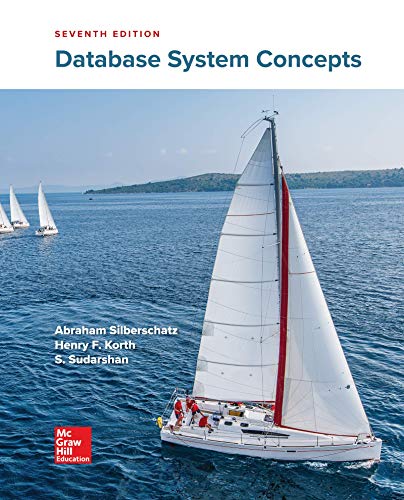
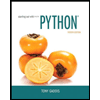
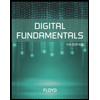
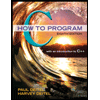
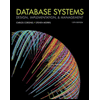
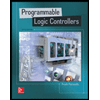