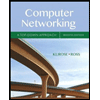
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Update it to create a class Student that includes four properties: an id (type Integer), a name (type String), a Major (type String) and a Grade (type Double). Use class Student to create objects (using buttonAdd) that will be read from the TextFields then save it into an ArrayList. Perform the following queries on the ArrayList of Student objects and show the results on the listViewStudents (Hint: add buttons as needed):
d) Use lambdas and streams to calculate the total average of all Students grades, then show the result.
1. | |
Ch3HW; | |
import javafx.application.Application; | |
import javafx.fxml.FXMLLoader; | |
import javafx.scene.Parent; | |
import javafx.scene.Scene; | |
import javafx.stage.Stage; | |
public class MainApp extends Application{ | |
@Override | |
publicvoidstart(StageprimaryStage) throwsException { | |
FXMLLoader loader =newFXMLLoader(getClass().getResource("StudentScreen.fxml")); | |
Parent parent = loader.load(); | |
Scene scene =newScene(parent); | |
primaryStage.setScene(scene); | |
primaryStage.setTitle("Student Processing Screen"); | |
primaryStage.show(); | |
} | |
publicstaticvoidmain(String[] args) { | |
launch(args); | |
} | |
} |
2. | |
<?xml version="1.0" encoding="UTF-8"?> | |
<?import javafx.geometry.*?> | |
<?import javafx.scene.effect.*?> | |
<?import java.lang.*?> | |
<?import java.util.*?> | |
<?import javafx.scene.*?> | |
<?import javafx.scene.control.*?> | |
<?import javafx.scene.layout.*?> | |
<FlowPane alignment="CENTER" maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" style="-fx-font-size: 14; -fx-font-weight: bold;" xmlns="http://javafx.com/javafx/8" xmlns:fx="http://javafx.com/fxml/1" fx:controller="Ch3HW.StudentScreenController"> | |
<children> | |
<VBox alignment="CENTER" prefHeight="395.0" prefWidth="602.0"> | |
<children> | |
<HBox alignment="CENTER" prefHeight="321.0" prefWidth="602.0" spacing="15.0"> | |
<children> | |
<GridPane hgap="10.0" maxHeight="-Infinity" maxWidth="-Infinity" prefHeight="141.0" prefWidth="239.0" vgap="10.0"> | |
<columnConstraints> | |
<ColumnConstraints halignment="RIGHT" hgrow="SOMETIMES" maxWidth="142.0" minWidth="10.0" prefWidth="52.0" /> | |
<ColumnConstraints hgrow="SOMETIMES" maxWidth="188.0" minWidth="10.0" prefWidth="177.0" /> | |
</columnConstraints> | |
<rowConstraints> | |
<RowConstraints minHeight="10.0" prefHeight="30.0" vgrow="SOMETIMES" /> | |
<RowConstraints minHeight="10.0" prefHeight="30.0" vgrow="SOMETIMES" /> | |
<RowConstraints minHeight="10.0" prefHeight="30.0" vgrow="SOMETIMES" /> | |
<RowConstraints minHeight="10.0" prefHeight="30.0" vgrow="SOMETIMES" /> | |
</rowConstraints> | |
<children> | |
<Label text="ID:" /> | |
<Label text="Name:" GridPane.rowIndex="1" /> | |
<Label text="Major:" GridPane.rowIndex="2" /> | |
<Label text="Grade:" GridPane.rowIndex="3" /> | |
<TextField fx:id="textFieldID" prefHeight="25.0" prefWidth="171.0" GridPane.columnIndex="1" /> | |
<TextField fx:id="textFieldName" prefHeight="25.0" prefWidth="145.0" GridPane.columnIndex="1" GridPane.rowIndex="1" /> | |
<TextField fx:id="textFieldMajor" GridPane.columnIndex="1" GridPane.rowIndex="2" /> | |
<TextField fx:id="textFieldGrade" GridPane.columnIndex="1" GridPane.rowIndex="3" /> | |
</children> | |
</GridPane> | |
<ListView fx:id="listViewStudents" prefHeight="321.0" prefWidth="302.0" style="-fx-font-family: monospaced;" /> | |
</children> | |
</HBox> | |
<HBox alignment="CENTER" prefHeight="74.0" prefWidth="602.0" spacing="15.0"> | |
<children> | |
<Button fx:id="buttonAdd" mnemonicParsing="false" onAction="#buttonAddHandle" text="Add" /> | |
<Button fx:id="buttonClear" mnemonicParsing="false" onAction="#buttonClearHandle" text="Clear" /> | |
</children></HBox> | |
</children> | |
<effect> | |
<InnerShadow choke="0.38" color="CORAL" height="85.5" radius="43.175" width="89.2" /> | |
</effect> | |
<padding> | |
<Insets bottom="10.0" left="10.0" right="10.0" top="10.0" /> | |
</padding> | |
</VBox> | |
</children> | |
</FlowPane> |
3. | |
package Ch3HW; | |
import java.net.URL; | |
import java.util.ResourceBundle; | |
import javafx.event.ActionEvent; | |
import javafx.fxml.FXML; | |
import javafx.fxml.Initializable; | |
import javafx.scene.control.Button; | |
import javafx.scene.control.ListView; | |
import javafx.scene.control.TextField; | |
/*** FXML Controller class*/ | |
public class StudentScreenController implements Initializable { | |
@FXML | |
privateTextField textFieldID; | |
@FXML | |
privateTextField textFieldName; | |
@FXML | |
privateTextField textFieldMajor; | |
@FXML | |
privateTextField textFieldGrade; | |
@FXML | |
privateListView<String> listViewStudents; | |
@FXML | |
privateButton buttonAdd; | |
@FXML | |
privateButton buttonClear; | |
/*** Initializes the controller class.*/ | |
@Override | |
publicvoidinitialize(URLurl, ResourceBundlerb) { | |
// This is just a intitial data | |
listViewStudents.getItems() | |
.add(String.format("%-5s %-10s %-7s %8.2f", "111", "Ahmad", "CS", 93.2)); | |
listViewStudents.getItems() | |
.add(String.format("%-5s %-10s %-7s %8.2f","123", "Marwa", "EDUC", 87.9)); | |
} | |
@FXML | |
privatevoidbuttonAddHandle(ActionEventevent) { | |
} | |
@FXML | |
privatevoidbuttonClearHandle(ActionEventevent) { | |
} | |
} |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- //3. toggleCommentSection//a. Receives a postId as the parameter//b. Selects the section element with the data-post-id attribute equal to the postId//received as a parameter//c. Use code to verify the section exists before attempting to access the classList//property//d. At this point in your code, the section will not exist. You can create one to test if//desired.//e. Toggles the class 'hide' on the section element//f. Return the section element function code so far passes a.b,c fails rest function toggleCommentSection(postId) { // If Post Id Is Passed, Return Undefined if (!postId) { return undefined; } else { // Else, Get All Comment Sections const commentSections = document.querySelectorAll('[data-post-id]'); // Loop Through Each Comment Section for (let i = 0; i < commentSections.length; i++) { const commentSection = commentSections[i]; // If Post Id Attribut Of Comment Section Is Equal To Post Id Passed Arg…arrow_forwardQuestion N .Java Three Part I – Now build an AccountList Business Class. This class will have a list of Account Objects. You can use an Array or an ArrayList to store this list. I would also have a count property to keep track of how many Accounts are in the list. Methods should include a display() method, and an add(Account a1) method. Part II – Now add a new Property to the Customer class. AccountList aList; Make it so that when an User calls the selectDB(3001) method(and passes a custid), the Customer class will go look in the database for all accounts that belong to this customer and put them in the use the aList object. So the Customer class will contain a list of Accounts that the customer owns. Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forwardTests: In the test_sun.py module, create tests for the planet objects. You can (and should) do this before you have written any Sun code at all. Implementation: In the sun.py module (see template), implement a Sun class as described in Section 10.4. Your Sun class should include the following methods: __init__, get_mass, get_radius, get_temperature, and __str__. Your __str__ method should include the following information: Name, radius, mass, and temperature. You can assume that all numerical values are of type float and use type hints in all your method signatures accordingly.arrow_forward
- Update it to create a class Student that includes four properties: an id (type Integer), a name (type String), a Major (type String) and a Grade (type Double). Use class Student to create objects (using buttonAdd) that will be read from the TextFields then save it into an ArrayList. Perform the following queries on the ArrayList of Student objects and show the results on the listViewStudents (Hint: add buttons as needed): d) Use lambdas and streams to calculate the total average of all Students grades, then show the result. e) Use lambdas and streams to group Students by major, then show the results. 1. Ch3HW; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.stage.Stage; public class MainApp extends Application{ @Override publicvoidstart(StageprimaryStage) throwsException { FXMLLoader loader…arrow_forwardGiven these three ArrayLists: ArrayList collectionA = new ArrayList(); ArrayList collectionB = new ArrayList(15); ArrayList collectionC = new ArrayList(25) ; After adding 390 items to each collection, answer the following two questions: Blank 1: Which ArrayList has the largest capacity for the array data member being used internally for storing the items in the object? Blank 2: What is the capacity for the object in blank one? Blank # 1 A Blank # 2 Aarrow_forwardCreate a class called Student that models a student at Carleton University. A student's state consists of a name (String), id number (int) and a list of grades (array of double). Your class must have a constructor that has three input parameters (name, id and grade list) and sets the initial state for the object. Include a toString() method that returns a useful string representation of a student object. The string representation should contain the student's name, ID number, and the number of grades they have recorded (so NOT include all the grades). Include a gradesInRange(double lower, double upper) method that returns the number of grades that this student has that are in the range [lower,upper]. That is, the it returns the number of grades that are greater than or equal to lower and less than or equal to upper. Example Usage: double[] grades {81.2, 93.2, 76,2, 84.6, 63.11, 79.8}; Student s new Student("cat", 100123987, grades); s.gradesInRange (80,100); int num_grades_A // assert…arrow_forward
- create an array of "Opinion" objects called "poll" that will represent five students opinions of a class.arrow_forwardCreate a class called CustomerLister with a main method that instantiates an array of String objects called customerName. The array should have room for five String objects. Use an initializer list to put the following names into the array:CathyBenJorgeWandaFreddie Print the array of names.arrow_forwardCreate a Point class to hold x and y values for a point. Create methods show(), add() and subtract() to display the Point x and y values, and add and subtract point coordinates. Create another class Shape, which will form the basis of a set of shapes. The Shape class will contain default functions to calculate area and circumference of the shape, and provide the coordinates (Points) of a rectangle that encloses the shape (a bounding box). These will be overloaded by the derived classes; therefore, the default methods for Shape will only print a simple message to standard output. Create a display() function for Shape, which will display the name of the class and all stored information about the class (including area, circumference and bounding box). Build the hierarchy by creating the Shape classes Circle, Rectangle and Triangle. Search the Internet for the rules governing these shapes, if necessary. For these three Shape classes, create default constructors, as well as constructors…arrow_forward
- Define a class called Book. This class should store attributes such as the title, ISBN number, author, edition, publisher, and year of publication. Provide get/set methods in this class to access these attributes. Define a class called Bookshelf, which contains the main method. This class should create a few book objects with distinct names and store them in an ArrayList. This class should then list the names of all books in the ArrayList. Enhance the program by providing a sort function, which will sort the books in ascending order of their year of publication. Create a few more Bookobjects with the same names but with different edition numbers, ISBNs, and years of publication. Add these new Book objects to the ArrayList, and display the book list sorted by book name; for duplicate books of the same name, sort the list by year of publication. (Hint: You will need to define a comparator class that takes two Book objects as parameters of the compareTo This method should do a two-step…arrow_forwardCreate the VisualCounter class, which supports both increment and decrement actions. Take the function Object() { [native code] }'s two arguments N and max, where N specifies the maximum action number and max specifies the counter's maximum absolute value. Make a plot that displays the worth of the counter each time its tally changes as a byproduct.arrow_forwardModalProp detailsanimationType it's an enum of ('none', 'slide', 'fade') and it controls modal animation.visible its a bool that controls modal visiblity.onShow it allows passing a function that will be called once the modal has been shown.transparent bool to set transparency.onRequestClose (android) it always defining a method that will be called when user tabs back buttononOrientationChange (IOS) it always defining a method that will be called when orientation changessupportedOrientations (IOS) enum('portrait', 'portrait-upside-down', 'landscape', 'landscape-left', 'landscape-right')Modal component is a simple way to present content above an enclosing view.using given Modal make a Basic Example?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
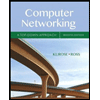
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
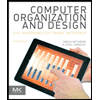
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
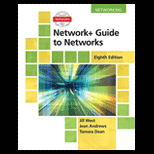
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
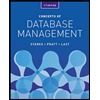
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
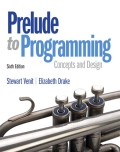
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
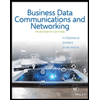
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY