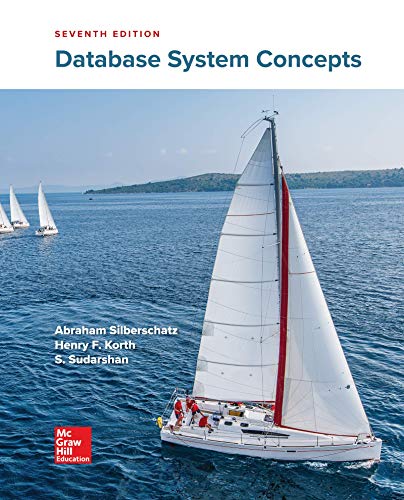
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
java
Implement a Stack class using a linked list,
i) Insert at least 10 elements into the stack.
ii) show push and pop operations on the stack.
iii) Print the order of elements in the stack.
iv) Print first and last elements of the stack.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

Knowledge Booster
Similar questions
- Given main() complete the Stack class by writing the methods push() and pop(). The stack uses an array of size 5 to store elements. The command Push followed by a positive number pushes the number onto the stack. The command Pop pops the top element from the stack. Entering -1 exits the program. Ex. If the input is Push 1 Push 2 Push 3 Push 4 Push 5 Pop -1 the output is Stack contents (top to bottom): 1 Stack contents (top to bottom): 2 1 Stack contents (top to bottom): 3 2 1 Stack contents (top to bottom): 4 3 2 1 Stack contents (top to bottom): 5 4 3 2 1 Stack contents (top to bottom): 4 3 2 1 import java.util.Scanner; public class PushPopStack { public static void main (String[] args) {Scanner scnr = new Scanner(System.in);Stack stack = new Stack(5);String action; // Push or Pop int numInput; // Integer value to push action = scnr.next(); while (!action.equals("-1")) {if (action.equals("Push")) {numInput = scnr.nextInt();stack.push(numInput);System.out.println("Stack contents…arrow_forwardC# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. This would occur in the NumberStack class, not the main class. These are the provided variables: private int [] stack;private int size; Create a method that will reverse the stack when put into the main class.arrow_forwardC# Display the stack with the given information: int topNum; NumberStack stack2 = new NumberStack(5); stack2.Push(5);stack2.Push(7);stack2.Push(12);stack2.Push(3); public void PrintStack() { //Loop through each element of the stack and display it for (int i = size - 1 ; i >= 0; i--) { Console.WriteLine(stack[i]); } }arrow_forward
- Stack: push(x) adds x to top of stack pop() removes top element of stack and returns it size() returns number of elements in stack Select all options that allow for an efficient implementation based on the discussions from class. For any array implementation, you can assume the array is large enough so that making a larger one is not needed when pushing an item to the stack. Using an array with the top at the front of the array. Using an array with the top at the back of the array. Using a singly linked list with the top at the head of the list. Using a singly linked list with the top at the tail of the list. None of these choices allows for an efficient implementation of all methods.arrow_forwardsuf: C nn.instru @ 2 S if the stack contains (from top) "5", "4", "3", "2", "1" and element = "new", the stack will contain "5", "4", "3", "new", "new" after interchange (). if the stack contains (from top) "5" and element "new", the stack will contain "new", "new" after interchange(). 30% Write public instanc method for MyStáck, called interchange (T element) to replac the bottom *two* items in the stack with element. wer than two items on the stack, upon return the stack should contain exactly two items that are element. Examples: F2 # ۲ 3 ۳ WE E ص X Assume class MyStack implements the following StackGen interface. For this question, make no assumptions about the implementation of MyStack except that the follo wing interface methods are implemented and work as documented. public interface StackGen { void push(T 0); // conventional stack behavior T pop(); // conventional stack behavior T top(); // returns, but does not remove, top element in stack boolean isEmpty(); // returns true…arrow_forward54. What is the difference between the top and pop operations of a stack? Group of answer choices The top operation removes the top item of the stack. The pop operation returns a reference to the top item on the stack and remove it. The top operation removes the top item of the stack. The pop operation returns a reference to the top item on the stack without removing it. The pop operation removes the top item of the stack. The top operation returns a reference to the top item on the stack without removing it. The pop and top operations are doing the same thing which removes the top item of the stack. The pop operation removes the top item of the stack. The top operation returns a reference to the top item on the stack and remove it.arrow_forward
- i want the solution for subpart 1arrow_forwardRecall the Stack ADT. It has the following operations: push(item): adds item to the top of the stack pop() : removes and returns the top item in the stack peek() : returns the top item in the stack (but does NOT remove it) size() : returns the number of items in the queue Write pseudocode for a function called remove(S, item) that takes a stack S and a value called item as input. The function removes all occurrences of item in S and returns the number of occurrences. The order of items in the modified stack must be the same as their original ordering (but with all occurrences of item absent). You can ONLY use basic control flow structures and Stack operations. You can use multiple stacks if you wish. Examples: S:= empty stack S.push('a'), S.push('t'), S.push('a'), S.push('u'), S.push('p') assert: the stack S now looks like ['a', 't', 'a', 'u', 'p'] (where 'p' is the top value) n = remove(S, 'a') assert : n is 2 assert : the stack S now looks like ['t', 'u', 'p'] (where 'p' is the top…arrow_forwardsolution for subpart 3arrow_forward
- Project Overview: This project is for testing the use and understanding of stacks. In this assignment, you will be writing a program that reads in a stream of text and tests for mismatched delimiters. First, you will create a stack class that stores, in each node, a character (char), a line number (int) and a character count (int). This can either be based on a dynamic stack or a static stack from the book, modified according to these requirements. I suggest using a stack of structs that have the char, line number and character count as members, but you can do this separately if you wish.Second, your program should start reading in lines of text from the user. This reading in of lines of text (using getline) should only end when it receives the line “DONE”.While the text is being read, you will use this stack to test for matching “blocks”. That is, the text coming in will have the delimiters to bracket information into blocks, the braces {}, parentheses (), and brackets [ ]. A string…arrow_forwardjava /* Practice Stacks and ourVector Write a java program that creates a stack of integers. Fill the stack with 30 random numbers between -300 and +300. A)- With the help of one ourVector Object and an additional stack, reorganize the numbers in the stack so that numbers smaller than -100 go to the bottom of the stack, numbers between -100 and +100 in the middle and numbers larger than +100 in the top (order does not matter) B)- (a little harder) Solve the same problem using only one ourVector object for help C)- (harder) Solve the same problem using only one additional stack as a helper */ public class HWStacks { public static void main(String[] args) { // TODO Auto-generated method stub } }arrow_forwardsolution for subpart 4arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
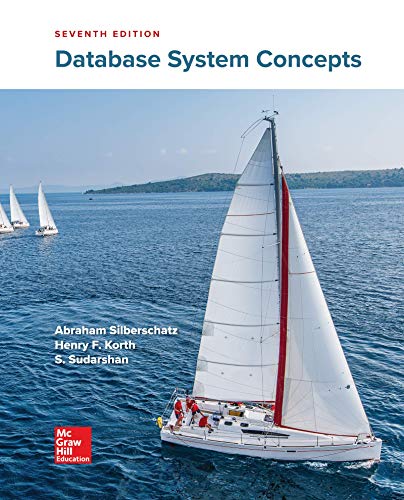
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
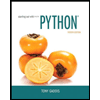
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
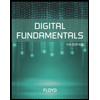
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
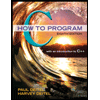
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
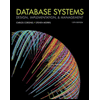
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
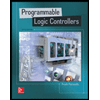
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education