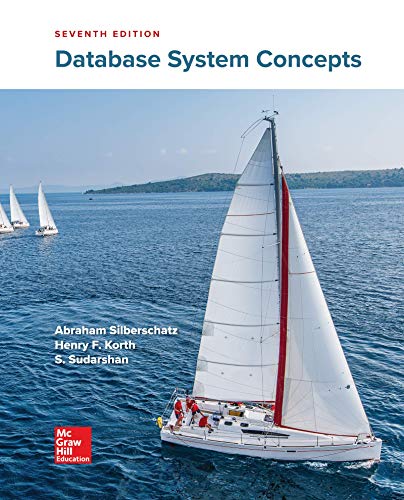
Java :
Write the Java code segment that uses a stack to determine if a string is a palindrome (assume all that blanks have been removed). A palindrome contains the same characters forwards as backwards, for example level is a palindrome. Your solution must use only a stack, you MUST process the string from left to right, and are allowed to go through the string only once. You must use an efficient
Use :
ArrayStack class given below:
/**
* Creates an empty stack using the default capacity.
*/
public ArrayStack()
{
this(DEFAULT_CAPACITY);
}
/**
* Creates an empty stack using the specified capacity.
* @param initialCapacity the initial size of the array
*/
public ArrayStack(int initialCapacity)
{
top = 0;
stack = (T[])(new Object[initialCapacity]);
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- If the elements “A”, “B”, “C” and “D” are placed in a stack and are removed one at a time, in what order will they be removed?arrow_forwardexamine a very simple task: reversing a word. When you run the program, it asks you to type in a word. When you press Enter, it displays the word with the letters in reverse order. A stack is used to reverse the letters. First the characters are extracted one by one from the input string and pushed onto the stack. Then they’re popped off the stack and displayed. Because of its last-in-first-out characteristic, the stack reverses the order of the characters.arrow_forwardCreate a programme that sorts a stack so that the smallest elements appear on top. You can use a second temporary stack, but you can't transfer the components into another data structure (such an array). The following operations are supported by the stack: push, pop, peek, and is Empty.arrow_forward
- Java - This project will allow you to compare & contrast different 4 sorting techniques, the last of which will be up to you to select. You will implement the following: Bubble Sort (pair-wise) Bubble Sort (list-wise) [This is the selection sort] Merge Sort Your choice (candidates are the heap, quick, shell, cocktail, bucket, or radix sorts) [These will require independent research) General rules: Structures can be static or dynamic You are not allowed to use built in methods that are direct or indirect requirements for this project – You cannot use ANY built in sorting functions - I/O (System.in/out *) are ok. All compare/swap/move methods must be your own. (You can use string compares) Your program will be sorting names – you need at least 100 unique names (you can use the 50 given in project #3) – read them into the program in a random fashion (i.e. not in any kind of alpha order). *The more names you have, the easier it is to see trends in speed. All sorts will be from…arrow_forwardJava - All objects in a sorted list must be objects that can be compared to each other. True or False?arrow_forwardJava - Elements in a Rangearrow_forward
- I have implemented a stack using arrays. The array is 5 elements long and is called examstack. The contents are: examstack[0] = 36 examstack[1] = 49 examstack[2] = 7 examstack[3] = 67 examstack[4] = 9 If I did a Pop what would be returned? 9 or 36 09 07 O 67 O 9 or 67 36 or 49 O 36 49arrow_forwardWhat is the operation that adds items to a stack? Get Set Pop Pusharrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
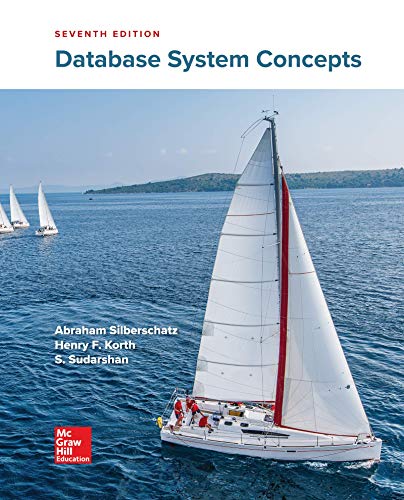
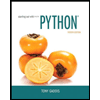
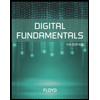
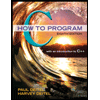
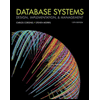
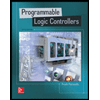