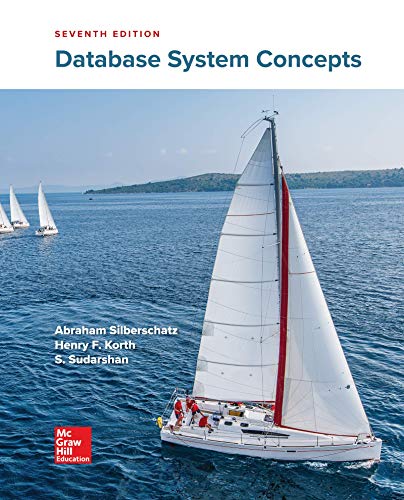
Java
I need help writing a program that has 3 methods: the main method, a method that swaps the digit pairs, and another method that swaps letter pairs. first you would ask the user for a number and call the method that swaps the digit pairs to swap the number shown in the picture. Then ask the user to input a string of letters and numbers and call the method that swaps lettter pairs to swap the letters as shown in the picture. in the method that swaps digit pairs, it accepts an integer for its parameter and then returns a new integer that has each pair of digits swapped in order. if it is an odd number of digits, leave the leftmost digit in its original place. the method that swaps digit pairs shouldnt use the string class.
but for the swap letter pairs, it is similar operation as the swap digit pairs method but accepts a string as a parameter and return the swapped string. it can use string class.
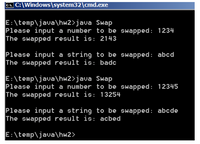

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Java Your program will read in a credit card number and expiration date from the user and validate the data. The program will continue to ask for user input until a valid number and date are entered. The rules for what is valid are specified below. readYear() and readMonth(int) methods Complete the readYear() and readMonth(int) methods. These methods ask the user to input the expiration year and month of their credit card. Each method should contain a loop inside the method so the code repeats until a valid month or year is entered by the user. If the user enters an invalid year or month, print an error message and ask them to try again. The readMonth(int) method reads in the month as a number. A valid month is any number between 1 and 12 (inclusive). A valid year is 2022 or later. readCreditCardNumber() method Complete the readCreditCardNumber() method. This method asks the user to input their credit card number. This method should contain a loop so the code repeats until a valid…arrow_forwardWrite in JAVA When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This adjustment can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain fewer than 20 floating-point values. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:System.out.printf("%.2f", yourValue);arrow_forwardJAVA Practice Question for Midterm Write a class with a constructor that accepts a String object as its argument. The class should have a method that returns the number of vowels in the string, and another method that returns the number of consonants in the string. Demonstrate the class in a program by invoking the methods that return the number of vowels and consonants. Print the counts returned. Imagine you are developing a software package for an online shopping site that requires users to enter their own passwords. Your software requires that users’ passwords meet the following criteria: The password should be at least six characters long. The password should contain at least one uppercase and at least one lowercase letter. The password should have at least one digit. a program that asks the user to enter a password, then displays a message indicating whether it is valid or not.arrow_forward
- Write this program in Java using a custom method. Implementation details You will implement this program in a specific way in order to gain some experience with loops, arrays and array lists. Use an array of strings to store the 4 strings listed in the description. Use a do-while loop for your 'game engine'. This means the game starts once the user enters money. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for this 'game engine' is shown below: determine the fruits to display (step 3 below) and print them determine if there are 3 or 4 of the same image display the results update the customer balance as necessary prompt to play or quit continue loop if customer wants to play and there's money for another game. Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an…arrow_forwardJava with out string formarrow_forwardbasic java please Write a void method named emptyBox()that accepts two parameters: the first parameter should be the height of a box to be drawn, and the second parameter will be the width. The method should then draw an empty box of the correct size. For example, the call emptyBox(8, 5) should produce the following output: ***** * * * * * * * * * * * * ***** The width of the box is 5. The middle lines have 3 spaces in the middle. The height of the box is 8. The middle columns have 6 spacesarrow_forward
- in Java Tasks Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don't display the computer's choice yet.) 2. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. (You can use a menu if you prefer.) 3. The computer's choice is displayed. Tasks 4. A winner is selected according to the following rules: a. If one player chooses rock and the other player chooses scissors, then rock wins. (The rock smashes the scissors.) b. If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.) C. If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps…arrow_forwardIn Java: When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by subtracting the smallest value from all the values. The input begins with an integer indicating the number of integers that follow. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 30 50 10 70 65 the output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program must define and call a method:public static int getMinimumInt(int[] listInts, int listSize) import java.util.Scanner; public class LabProgram {/* Define your method here */ public static void main(String[] args) {/* Type your code here. */}}arrow_forwardJAVA PROGRAMarrow_forward
- Java: Receives a phrase and returns the converted phrase to Pig Latin. A word is translated into Pig Latin according to the following rules: a. If there are no vowels in the word, then add “ay” to the end of it. (a, e, i, o, and u are all the vowels considered). b. If the word begins with a vowel, then add “yay” to the end of it. c. Otherwise, take all the letters up to the first vowel and move those letters to the end then add “ay”. Sample Input: System.out.println(toPigLatin("I am fluent in Pig Latin")); System.out.println(toPigLatin(“Hey”)); Sample Output: Iyay amyay uentflay inyay igPay atinLay Heyayarrow_forwardIn this assignment you will demonstrate your knowledge of debugging by fixing the errors you find in the program below. Fix the code, and paste it in this document, along with the list of the problems you fixed.This example allows the user to display the string for the day of the week. For example, if the user passed the integer 1, the method will return the string Sunday. If the user passed the integer 2, the method will return Monday. This code has both syntax errors and logic errors. Hint: There are two logic errors to find and fix (in addition to the syntax errors).Inport daysAndDates.DaysOfWeek;public class TestDaysOfWeek {public static void main(String[] args) {System.out.println("Days Of week: ");for (int i = 0;i < 8;i++) {System.out.println("Number: " + i + "\tDay Of Week: " + DaysOfWeek.DayOfWeekStr(i) )}}}package daysAndDatespublic class DaysOfWeek {public static String DayOfWeekStr(int NumberOfDay) {String dayStr = ""switch (NumberOfDay) {case 1:dayStr =…arrow_forwardFor the code in java below it shows a deck of 52 cards and asks the name of the two players and makes both players draw five cards from the deck. What I want to be added into the code is that both Players are people that can select what card they pick and Player A starts. Player A picks a card in his/her hand. Player B gets to choose the two cards which add to the value of Player A’s card, if player B lies and the two cards they choose do not add up to A's card, player B loses a point, if player B does not have two cards whose value adds to the value of Player A’s card, then no one gets a point. Player’s A card (if selected) and the two cards from Player B are discarded both players draw back to 5 cards from the deck. Main class code: import java.util.ArrayList; import java.util.Scanner; import java.util.List; import java.util.Random; class Main { publicstaticvoid main(String[] args) { // card game, two players, take turns. String[] suits = {"Hearts", "Clubs", "Spades",…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
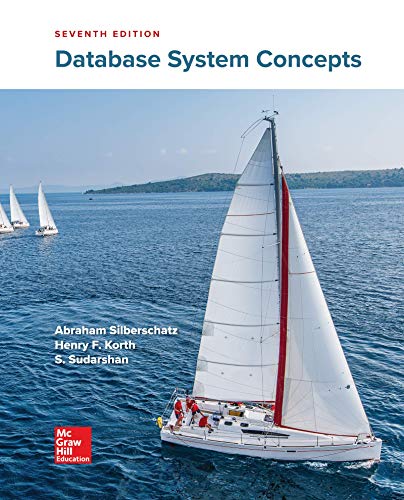
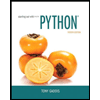
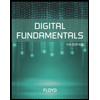
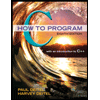
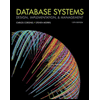
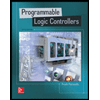