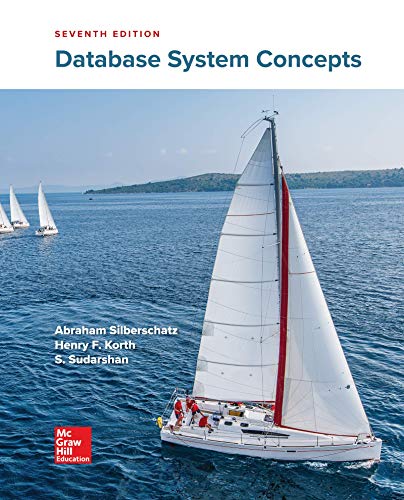
JAVA PROGRAM
MODIFY THIS PROGRAM SO IT READS THE TEXT FILES IN HYPERGRADE. I HAVE PROVIDED THE INPUTS AND THE FAILED TEST CASE AS A SCREENSHOT. HERE IS THE WORKING CODE TO MODIFY:
import java.io.*;
import java.util.*;
public class NameSearcher {
private static List<String> loadFileToList(String filename) throws FileNotFoundException {
List<String> namesList = new ArrayList<>();
File file = new File(filename);
if (!file.exists()) {
throw new FileNotFoundException(filename);
}
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine().trim();
String[] names = line.split("\\s+");
for (String name : names) {
namesList.add(name.toLowerCase());
}
}
}
return namesList;
}
private static Integer searchNameInList(String name, List<String> namesList) {
int index = namesList.indexOf(name.toLowerCase());
return index == -1 ? null : index + 1;
}
public static void main(String[] args) {
List<String> boyNames = new ArrayList<>(); // Initialize with an empty list
List<String> girlNames = new ArrayList<>(); // Initialize with an empty list
try {
boyNames = loadFileToList("Boynames.txt");
} catch (FileNotFoundException e) {}
try {
girlNames = loadFileToList("Girlnames.txt");
} catch (FileNotFoundException e) {}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Enter a name to search or type QUIT to exit: ");
String input = scanner.nextLine().trim();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
String capitalizedInput = input.substring(0, 1).toUpperCase() + input.substring(1).toLowerCase();
Integer boyIndex = searchNameInList(input, boyNames);
Integer girlIndex = searchNameInList(input, girlNames);
if (boyIndex == null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was not found in either list.");
} else if (boyIndex != null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular boy names list (line " + boyIndex + ").");
} else if (boyIndex == null && girlIndex != null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular girl names list (line " + girlIndex + ").");
} else {
System.out.println("The name '" + capitalizedInput + "' was found in both lists: boy names (line " + boyIndex + ") and girl names (line " + girlIndex + ").");
}
}
scanner.close();
}
}
TEST CASE:
Enter a name to search or type QUIT to exit:\n
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER
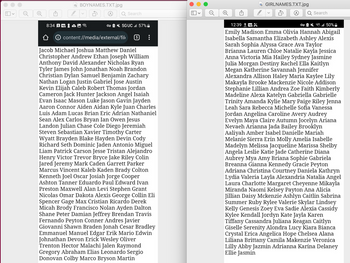
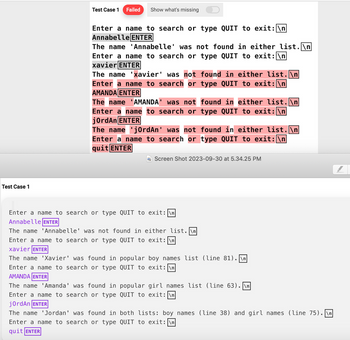

Step by stepSolved in 4 steps with 3 images

- Please answer the question in the screenshot. The language used here is in Java.arrow_forwardIn python two possible ways pleasearrow_forwardWrite a python hangman program Modify your program so that the following functionality is added:1. Instead of selecting the word from a hard coded list, incorporate problem2 so that thelist is read from a file, then a word is randomly selected from this list.2. when the game ends, the following information should be outputted to a new file:● the word● the state of the hangman● if the user won or lostExample outputs:quokkaO\||You won!kangarooO\|/|/ \You lostarrow_forward
- Java Program ASAP Please modify Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); in the program so it reads the two text files and passes the test cases. Down below is a working code. Also dont add any import libraries in the program just modify the rest of the code so it passes the test cases. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.HashMap;import java.util.Map;import java.util.Scanner;public class MorseCodeConverter { public static void main(String[] args) { Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); Scanner scanner = new Scanner(System.in); System.out.print("Please enter the file name or type QUIT to exit:\n"); do { String fileName = scanner.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { String text =…arrow_forwardGrey lines (start - 20 & 22-end of code) can NOT be edited. New JAVA code must be in between theses as show on line 21 in image.arrow_forwardMain.java, show output of the given cases.arrow_forward
- USING THE FOLLOWING METHOD SWAP CODE: import java.util.*; class ListIndexOutOfBoundsException extends IndexOutOfBoundsException { public ListIndexOutOfBoundsException(String s) { super(s); } } class ListUtil { public static int maxValue(List<Integer> aList) { if(aList == null || aList.size() == 0) { throw new IllegalArgumentException("List cannot be null or empty"); } int max = aList.get(0); for(int i = 1; i < aList.size(); i++) { if(aList.get(i) > max) { max = aList.get(i); } } return max; } public static void swap(List<Integer> aList, int i, int j) throws ListIndexOutOfBoundsException { if(aList == null) { throw new IllegalArgumentException("List cannot be null"); } if(i < 0 || i >= aList.size() || j < 0 || j >= aList.size()) { throw new ListIndexOutOfBoundsException("Index out…arrow_forwardstarter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardYou have to use comment function to describe what each line does import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class PreferenceData { private final List<Student> students; private final List<Project> projects; private int[][] preferences; private static enum ReadState { STUDENT_MODE, PROJECT_MODE, PREFERENCE_MODE, UNKNOWN; }; public PreferenceData() { super(); this.students = new ArrayList<Student>(); this.projects = new ArrayList<Project>(); } public void addStudent(Student s) { this.students.add(s); } public void addStudent(String s) { this.addStudent(Student.createStudent(s)); } public void addProject(Project p) { this.projects.add(p); } public void addProject(String p) { this.addProject(Project.createProject(p)); } public void createPreferenceMatrix() { this.preferences = new…arrow_forward
- New JAVA code can only be added between lines 9 and 10, as seen in image.arrow_forwardIn the following program skeleton, replace the < insert code here> line with theappropriate code. The resulting program should display the initial list of songs,prompt the user for a string of text to be replaced and then prompt for new text toreplace it. After making the replacement, it should display the updated list of songs.import java.util.Scanner;public class UpdateSongs{public static void main ( String [] args){Scanner stdIn = new Scanner(System.in);String songs =“ 1. Welcome to Your Life – Grouplove\n” +“ 2. Sedona – Houndmouth\n” +“3. Imagine – John Lennon\n” +“4. Bohemian Rhapsody –Queen\n”;String oldText, newText;< insert code here>}}Sample Session:1. Welcome to Your Life – Grouplove”2. Sedona – Houndmouth3. Imagine – John Lennon4. Bohemian Rhapsody –QueenEnter text to replace: LennonEnter new text: Dean1. Welcome to Your Life – Grouplove”2. Sedona – Houndmouth3. Imagine – John Dean4. Bohemian Rhapsody –Queenarrow_forwardpackage Q2;import java.io.FileInputStream;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Question2 {public static void main(String[] args) throws FileNotFoundException {/*** Part a* Finish creating an ArrayList called NameList that stores the names in the file Names.txt.*/ArrayList<String> NameList;/*** Part b* Replace null on the right-hand-side of the declaration of the FileInputStream object named inputStream* so that it is initialized correctly to the Names.txt file located in the folder specified in the question description*/FileInputStream inputStream = null;Scanner scnr = new Scanner(inputStream); //Do not modify this line of code/*** Part c* Using a loop and the Scanner object provided, read the names from Names.txt* and store them in NameList created in Part a.*//*** Part d* Reorder the names in the ArrayList so that they appear in reverse alphabetical order.*/// System.out.println("NameList after correct ordering: "…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
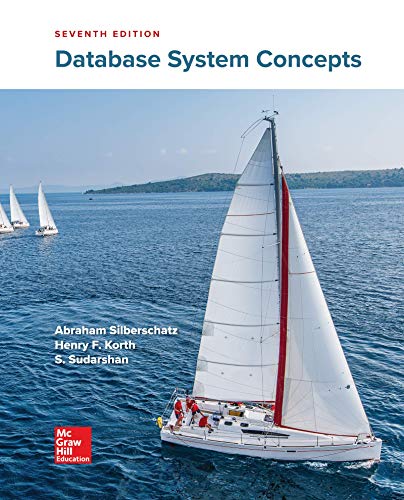
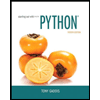
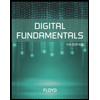
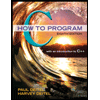
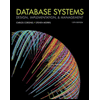
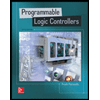