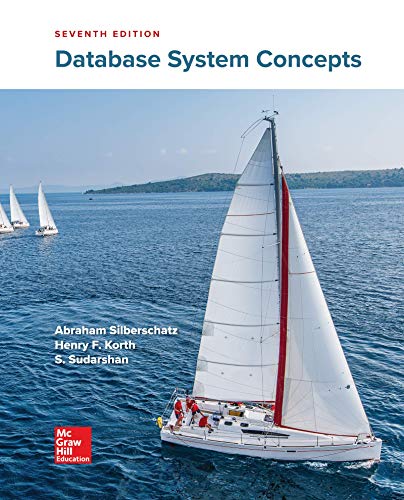
Concept explainers
USING JAVA CODE
Consider the interface below:
package prelim;
import java.util.NoSuchElementException;
public interface MyList<E> {
public int getSize();
public void insert(E data) throws ListOverflowException;
public E getElement(E data) throws NoSuchElementException;
public boolean delete(E data); // returns false if the data is not deleted in the list
public boolean search(E data); // returns index of data, else -1 is return
1. Create a simple menu-like program that will act like a virtual classroom. The menu will act as a means for the user to interact with the program.
2. The required menu options are the CRUD (create, read, update, and delete) and search operations.
3. The program should use at least one of the following:
- singly-linked node class
-doubly-linked node class
-singly-linked circular node class
-doubly-linked circular node class

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 5 images

- Add the method below to the parking office.java class. Method getParkingCharges(ParkingPermit) : Money Parkingoffice.java public class ParkingOffice {String name;String address;String phone; List<Customer> customers;List<Car> cars;List<ParkingLot> lots;List<ParkingCharge> charges; public ParkingOffice(){customers = new ArrayList<>();cars = new ArrayList<>();lots = new ArrayList<>();charges = new ArrayList<>();}public Customer register() {Customer cust = new Customer(name,address,phone);customers.add(cust);return cust;}public Car register(Customer c,String licence, CarType t) {Car car = new Car(c,licence,t);cars.add(car);return car;}public Customer getCustomer(String name) {for(Customer cust : customers)if(cust.getName().equals(name))return cust;return null;}public double addCharge(ParkingCharge p) {charges.add(p);return p.amount;} public String[] getCustomerIds(){String[] stringArray1 = new String[2];for(int…arrow_forwardAdd the method below to the parking office.java class. Method getParkingCharges(Customer) : Money Parkingoffice.java public class ParkingOffice {String name;String address;String phone; List<Customer> customers;List<Car> cars;List<ParkingLot> lots;List<ParkingCharge> charges; public ParkingOffice(){customers = new ArrayList<>();cars = new ArrayList<>();lots = new ArrayList<>();charges = new ArrayList<>();}public Customer register() {Customer cust = new Customer(name,address,phone);customers.add(cust);return cust;}public Car register(Customer c,String licence, CarType t) {Car car = new Car(c,licence,t);cars.add(car);return car;}public Customer getCustomer(String name) {for(Customer cust : customers)if(cust.getName().equals(name))return cust;return null;}public double addCharge(ParkingCharge p) {charges.add(p);return p.amount;} public String[] getCustomerIds(){String[] stringArray1 = new String[2];for(int i=0;i<2;i++){stringArray1[i]…arrow_forwardpackage edu.umsl.iterator;import java.util.ArrayList;import java.util.Arrays;import java.util.Collection;import java.util.Iterator;public class Main {public static void main(String[] args) {String[] cities = {"New York", "Atlanta", "Dallas", "Madison"};Collection<String> stringCollection = new ArrayList<>(Arrays.asList(cities));Iterator<String> iterator = stringCollection.iterator();while (iterator.hasNext()) {System.out.println(/* Fill in here */);}}} Rewrite the while loop to print out the collection using an iterator. Group of answer choices iterator.toString() iterator.getClass(java.lang.String) iterator.remove() iterator.next()arrow_forward
- Write a Java program to implement the following: Ask the user to enter the names of 3 Books and store them in an ArrayList called Book Remove the second book from the list Add the user to enter a new book name and store to be the first book of the list Ask the user to enter a book name, search for it in list, and print “exist” or “not exist” Print the book name at index 2 Print all list items using loop Print the size of the listarrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardUSING THE FOLLOWING METHOD SWAP CODE: import java.util.*; class ListIndexOutOfBoundsException extends IndexOutOfBoundsException { public ListIndexOutOfBoundsException(String s) { super(s); } } class ListUtil { public static int maxValue(List<Integer> aList) { if(aList == null || aList.size() == 0) { throw new IllegalArgumentException("List cannot be null or empty"); } int max = aList.get(0); for(int i = 1; i < aList.size(); i++) { if(aList.get(i) > max) { max = aList.get(i); } } return max; } public static void swap(List<Integer> aList, int i, int j) throws ListIndexOutOfBoundsException { if(aList == null) { throw new IllegalArgumentException("List cannot be null"); } if(i < 0 || i >= aList.size() || j < 0 || j >= aList.size()) { throw new ListIndexOutOfBoundsException("Index out…arrow_forward
- please fix code to match "enter patients name in lbs" thank you import java.util.LinkedList;import java.util.Queue;import java.util.Scanner; interface Patient { public String displayBMICategory(double bmi); public String displayInsuranceCategory(double bmi);} public class BMI implements Patient { public static void main(String[] args) { /* * local variable for saving the patient information */ double weight = 0; String birthDate = "", name = ""; int height = 0; Scanner scan = new Scanner(System.in); String cont = ""; Queue<String> patients = new LinkedList<>(); // do while loop for keep running program till user not entered q do { System.out.print("Press Y for continue (Press q for exit!) "); cont = scan.nextLine(); if (cont.equalsIgnoreCase("q")) { System.out.println("Thank you for using BMI calculator"); break;…arrow_forward*JAVA* complete method Delete the largest valueremoveMax(); Delete the smallest valueremoveMin(); class BinarHeap<T> { int root; static int[] arr; static int size; public BinarHeap() { arr = new int[50]; size = 0; } public void insert(int val) { arr[++size] = val; bubbleUP(size); } public void bubbleUP(int i) { int parent = (i) / 2; while (i > 1 && arr[parent] > arr[i]) { int temp = arr[parent]; arr[parent] = arr[i]; arr[i] = temp; i = parent; } } public int retMin() { return arr[1]; } public void removeMin() { } public void removeMax() { } public void print() { for (int i = 0; i <= size; i++) { System.out.print( arr[i] + " "); } }} public class BinarH { public static void main(String[] args) { BinarHeap Heap1 = new BinarHeap();…arrow_forwardIn Java please help with the following: Sees whether this list is empty.@return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint:Node class definition should look something like: public class Node<T> { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element;this.next = next;this.prev = prev; } }arrow_forward
- PROBLEM STATEMENT: In this problem you will need to insert 5 at the frontof the provided ArrayList and return the list. import java.util.ArrayList;public class InsertElementArrayList{public static ArrayList<Integer> solution(ArrayList<Integer> list){// ↓↓↓↓ your code goes here ↓↓↓↓return new ArrayList<>(); Can you help me with this question The Language is Javaarrow_forwardRedesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
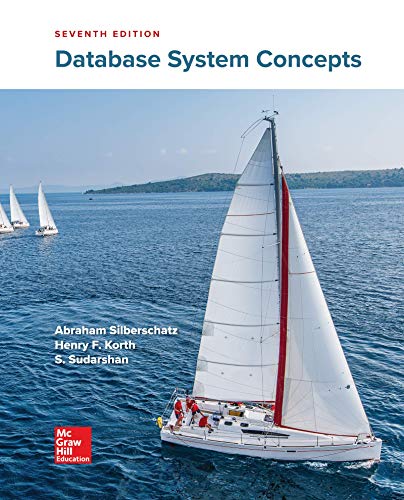
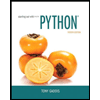
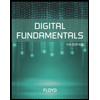
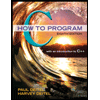
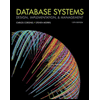
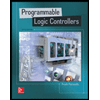