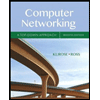
System.out.print("Enter a name (or 'QUIT' to exit): "); to: Enter a name to search or type QUIT to exit and take out this: System.out.println(filename + " is missing. Exiting...");
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> girlsNames = loadNames("GirlNames.txt");
List<String> boysNames = loadNames("BoyNames.txt");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter a name (or 'QUIT' to exit): ");
String input = scanner.nextLine();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
searchAndDisplay(input, girlsNames, boysNames);
}
scanner.close();
}
private static List<String> loadNames(String filename) {
List<String> names = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = reader.readLine()) != null) {
names.add(line.trim());
}
} catch (IOException e) {
System.out.println(filename + " is missing. Exiting...");
System.exit(1); // Exit the program if a file is missing
}
return names;
}
private static void searchAndDisplay(String name, List<String> girlsNames, List<String> boysNames) {
int girlIndex = searchName(name, girlsNames);
int boyIndex = searchName(name, boysNames);
String capitalizedName = name.substring(0, 1).toUpperCase() + name.substring(1).toLowerCase();
if (girlIndex == -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was not found in either list.");
} else if (girlIndex != -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular girl names list (line " + (girlIndex + 1) + ").");
} else if (girlIndex == -1 && boyIndex != -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular boy names list (line " + (boyIndex + 1) + ").");
} else {
System.out.println("The name '" + capitalizedName + "' was found in both lists: boy names (line " + (boyIndex + 1) + ") and girl names (line " + (girlIndex + 1) + ").");
}
}
private static int searchName(String name, List<String> namesList) {
for (int i = 0; i < namesList.size(); i++) {
if (namesList.get(i).equalsIgnoreCase(name)) {
return i;
}
}
return -1;
}
}
THE TEXT FILES ARE LOCATED IN HYPERGRADE I provided them and the failed test case as a screenshot. Thank you.
Test Case 1
Enter a name to search or type QUIT to exit:\n
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER
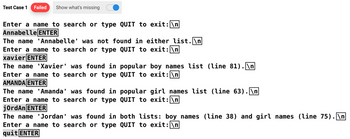
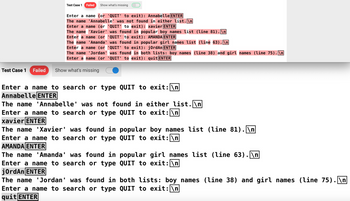

Step by stepSolved in 3 steps with 1 images

- Is the static block always run execute first in java. Explain it with java code. Considering a class with one variable and static block.explain both conditions when static execute first and other is when the static block does not executearrow_forwardWrite a Java program correctly that generates a report of proof of vaccination against COVID-19 for the peoplewho received their dose of vaccination. Write a program which:1. Display a welcome message.2. Then prompts the user to enter the following:• First name, with first letter uppercase, which is then stores in a variable of type String.• Last name, with first letter uppercase which is then stores in a variable of type String.• Day of birth, set as a number between 1 and 31, which is then stores in a variable of typeint.• Month of birth, set as a number between 1 and 12, which is then stores in a variable of typeint.• Year of birth, set as a number between 1920 and 2006, which is then stores in a variable oftype int.• Day of vaccination, set as a number between 1 and 31, which is then stores in a variable oftype int.• Month of vaccination. set as a number between 1 and 12, which is then stores in a variableof type int.• Year of vaccination, set as a number between 2020 and 2021,…arrow_forwardJava codearrow_forward
- Write a JAVA program to convert the temperature in Degree Centigrade to Fahrenheit. The main file should be named Fah.java. It should have import java.util.Scanner; with just one method and class called Fah. The program should take in a integer number with prompt: "Enter temp in Centigrade: “, and output a prompt "Temp in Fahrenheit is: “. The formula to convert Degree Centigrade to Fahrenheit is as follows: Conversion = ((9* Centigrade)/5)+32arrow_forwardJAVA PROGRAM PLEASE PLEASE PLEASE MODIFY THIS PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES THE TEST BECAUSE IT DOES NOT RUN IN HYPERGRADE. THEN TAKE OUT THE FOLLOWING FROM THE PROGRAM System.err.println("Error: " + filename + " is missing."); System.err.println("Error: Boynames.txt is missing."); and System.err.println("Error: Girlnames.txt is missing."); ALSO MAKE SURE IT PRINTS OUT THE FOLLOWING TEST CASE: Enter a name to search or type QUIT to exit:\nAnnabelleENTERThe name 'Annabelle' was not found in either list.\nEnter a name to search or type QUIT to exit:\nxavierENTERThe name 'Xavier' was found in popular boy names list (line 81).\nEnter a name to search or type QUIT to exit:\nAMANDAENTERThe name 'Amanda' was found in popular girl names list (line 63).\nEnter a name to search or type QUIT to exit:\njOrdAnENTERThe name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\nEnter a name to search or type QUIT to exit:\nquitENTER IT HAS TO SAY…arrow_forwardJAVA PPROGRAM Please Modify this program with further modifications as listed below: ALSO, take out and change the following in the program: System.out.println("Program terminated."); because the test case does not need it And change this in the program: Please enter the file name or type QUIT to exit:\n so it reperats once for every test case because it repeats twice in every case as shown in the screenshot. I only need it once. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.*;import java.util.ArrayList;import java.util.Scanner;public class SymmetricalNameMatcher { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { // Prompt the user to enter a file name or 'QUIT' to exit. System.out.print("Please enter the file name or type QUIT to exit:\n"); fileName =…arrow_forward
- 9 from breezypythongui import EasyFrame 10 11 class TemperatureConverter(EasyFrame): 12 ""A termperature conversion program.""" II II II 13 def -_init__(self): "I"Sets up the window and widgets.""" EasyFrame._init_-(self, title = "Temperature Converter") 14 15 II IIII 16 17 18 # Label and field for Celsius self.addLabel(text = "Celsius", 19 20 row = 0, column = 0) 21 self.celsiusField = self.addFloatField(value = 0.0, 22 row = 1, column = Ø, 23 24 precision = 2) 25 # Label and field for Fahrenheit self.addLabel(text = "Fahrenheit", 26 27 28 row = 0, column = 1) 29 self.fahrField = self.addFloatField(value = 32.0, 30 row = 1, 31 column = 1, 32 precision = 2) 33 # Celsius to Fahrenheit button self.addButton(text = ">>>>", 34 35 row = 2, column = 0, command = self.computeFahr) 36 37 38 39 # Fahrenheit to Celsius button 40 self.addButton(text = "<«««", 41 row = 2, column = 1, 42 command = self.computeCelsius) 43 44 # The controller methods def computeFahr(self): "I"Inputs the Celsius…arrow_forwardJava Program ASAP Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. And do the following; 1) For test cases 2 and 3 for the outputted numbers there needs to be commas and there needs to be nothing after that 2)for test case 1 outputted numbers there needs to be nothing after that, 3) for test 4 when file is not found there needs to be nothing after that 4) and for test case 5 and 7 afer a file is not found it needs to display Please re-enter the file name or type QUIT to exit: so you input the text file and displays the numbers. import java.io.*;import java.util.Scanner;public class FileSorting { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.equalsIgnoreCase("QUIT")) { break; // Exit the program…arrow_forwardA controlled transaction solution for Joe's Pizza, your friendly neighborhood pizza shop.Joe only make $200 worth of pizza everyday. Modify your program so that if the running total will exceed $200, print a warning and DO NOT add this value to running total. Instead, go to the beginning of loop statement block and wait for next transaction. can you please write it in import java.util.Scanner; and can you make it so i can copy and past it. thank you in advance the code from before /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */package personal;import java.util.Scanner;/** * * @author Mabda */public class Personal { /** * @param args the command line arguments */ public static void main(String[] args) { int num, sum = 0; char choice; Scanner key = new Scanner(System.in); while (true) {…arrow_forward
- Write a java program called Sales considering the following directions and the sample run. Practice here first the write answer on the answer sheet. Also, make sure to distinguish high and low caps as Java is case - sensitive. - import and declare a Scanner class - declare three floating – point variables named price, discount, and discountPrice - ask the user for the price (print statement) and get the price (Scanner) from the user - calculate the discount if price is less than 100, then the discount is 10% of price, else discount is 14% of price - print the discounted prices for the item Sample Run Enter the price 90.0 The discount price is 81.0 Enter the price 200.0 The discount price is 172.0arrow_forwardThe following code implements a Java Pasta timer that looks like the application below.• Once the start button is pressed the timer will count to two minutes.• The timer can be stopped by pressing stop.• When the timer is finished, it should pop-up an appropriate dialog box that states the timeis up.You are given a partial implementation of program. Fill out the missing code to complete theprogram import javax.swing.*;import java.awt.event.*;import javax.swing.border.*;public class PastaTimerApp extends implements { private JProgressBar progrBar; private JButton startButton, stopButton; private JLabel textLabel; private PastaTimer PastaTimer; public PastaTimerApp() { JPanel p = new JPanel(); p.setLayout(new BoxLayout(p, BoxLayout.Y_AXIS)); p.setBorder(new TitledBorder("Pasta Timer:")); progrBar = new JProgressBar(JProgressBar.HORIZONTAL, 0, 540); textLabel = new JLabel(" 0 Seconds "); p.add(progrBar); p.add(textLabel); // Initialize buttons and add action listeners JPanel controls =…arrow_forwardThis assignment will help you to get started with NetBeans and using Java compiler: To begin, put a block comment at the beginning of your Java file (and every Java source file in the future) with the assignment number, assignment title, program author [your name], and the date it's due. Next, similar to the programs presented in the first chapter of the textbook, use System.out object to display the following information: Your first name The name of the operating system you are using The name of your project (or simply the folder name if not using the IDE) The name of the class that contains the main() method in your source file. Note: Your program should print each of the above items on a separate output line. "The name of the class that contains the main() method in your source file.public class MyClass { public static void main(String[]) { System.out.println( "Hi" ); }}arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
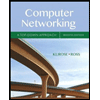
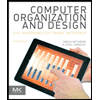
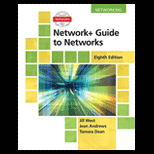
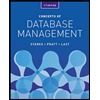
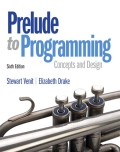
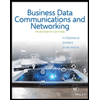