JAVA PROGRAM PLEASE PLEASE PLEASE MODIFY THIS PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES THE TEST BECAUSE IT DOES NOT RUN IN HYPERGRADE. THEN TAKE OUT THE FOLLOWING FROM THE PROGRAM System.err.println("Error: " + filename + " is missing."); System.err.println("Error: Boynames.txt is missing."); and System.err.println("Error: Girlnames.txt is missing."); ALSO MAKE SURE IT PRINTS OUT THE FOLLOWING TEST CASE: Enter a name to search or type QUIT to exit:\n AnnabelleENTER The name 'Annabelle' was not found in either list.\n Enter a name to search or type QUIT to exit:\n xavierENTER The name 'Xavier' was found in popular boy names list (line 81).\n Enter a name to search or type QUIT to exit:\n AMANDAENTER The name 'Amanda' was found in popular girl names list (line 63).\n Enter a name to search or type QUIT to exit:\n jOrdAnENTER The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n Enter a name to search or type QUIT to exit:\n quitENTER IT HAS TO SAY Enter a name to search or type QUIT to exit:\n THEH I PUT THE NAME ANNABELLE THEN IT PRINTS OUT: The name 'Annabelle' was not found in either list.\n THEN IT REPEATS Enter a name to search or type QUIT to exit:\n THEN I PUT THE NAME xavier THEN IT PRINTS OUT: The name 'Xavier' was found in popular boy names list (line 81).\n AND SO ON. UNTIL THE LAST IT HAS TO SAY Enter a name to search or type QUIT to exit:\n THEN I PUT QUIT IT ENDS THE TEST CASE. THE INPUTS ARE ALREADY IN HYPERGRADE SO MAKE SURE THIS PROGRAM PASSES THE TEST CASE WHEN I UPLOAD IT TO HYPERGRADE and take out the following from the program please: Boynames.txt is missing.\n Girlnames.txt is missing.\n Both data files are missing.\n THE TEST CASE DOES NOT ASK FOR IT. PLEASE REMOVE IT. I HAVE PROVIDED THE TEST CASE THAT FAILED AND THE INPUTS AS A SCREENSHOT. import java.io.*; import java.util.*; public class NameSearcher { private static Map loadFileToMap(String filename) throws FileNotFoundException { Map namesMap = new HashMap<>(); File file = new File(filename); try (Scanner scanner = new Scanner(file)) { int lineNumber = 1; while (scanner.hasNext()) { String line = scanner.nextLine().trim().toLowerCase(); namesMap.put(line, lineNumber); lineNumber++; } } catch (FileNotFoundException e) { // Handle the exception properly System.err.println("Error: " + filename + " is missing."); } return namesMap; } private static Integer searchNameInMap(String name, Map namesMap) { return namesMap.get(name.toLowerCase()); }
JAVA PROGRAM
PLEASE PLEASE PLEASE MODIFY THIS PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES THE TEST BECAUSE IT DOES NOT RUN IN HYPERGRADE. THEN TAKE OUT THE FOLLOWING FROM THE PROGRAM System.err.println("Error: " + filename + " is missing."); System.err.println("Error: Boynames.txt is missing."); and System.err.println("Error: Girlnames.txt is missing."); ALSO MAKE SURE IT PRINTS OUT THE FOLLOWING TEST CASE:
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER
THE INPUTS ARE ALREADY IN HYPERGRADE SO MAKE SURE THIS PROGRAM PASSES THE TEST CASE WHEN I UPLOAD IT TO HYPERGRADE and take out the following from the program please: Boynames.txt is missing.\n Girlnames.txt is missing.\n Both data files are missing.\n THE TEST CASE DOES NOT ASK FOR IT. PLEASE REMOVE IT. I HAVE PROVIDED THE TEST CASE THAT FAILED AND THE INPUTS AS A SCREENSHOT.
import java.io.*;
import java.util.*;
public class NameSearcher {
private static Map<String, Integer> loadFileToMap(String filename) throws FileNotFoundException {
Map<String, Integer> namesMap = new HashMap<>();
File file = new File(filename);
try (Scanner scanner = new Scanner(file)) {
int lineNumber = 1;
while (scanner.hasNext()) {
String line = scanner.nextLine().trim().toLowerCase();
namesMap.put(line, lineNumber);
lineNumber++;
}
} catch (FileNotFoundException e) {
// Handle the exception properly
System.err.println("Error: " + filename + " is missing.");
}
return namesMap;
}
private static Integer searchNameInMap(String name, Map<String, Integer> namesMap) {
return namesMap.get(name.toLowerCase());
}
public static void main(String[] args) {
Map<String, Integer> boyNames = new HashMap<>();
Map<String, Integer> girlNames = new HashMap<>();
try {
boyNames = loadFileToMap("Boynames.txt");
} catch (FileNotFoundException e) {
// Handle the exception properly
System.err.println("Error: Boynames.txt is missing.");
}
try {
girlNames = loadFileToMap("Girlnames.txt");
} catch (FileNotFoundException e) {
// Handle the exception properly
System.err.println("Error: Girlnames.txt is missing.");
}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter a name to search or type QUIT to exit:\n");
String input = scanner.nextLine().trim();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
Integer boyIndex = searchNameInMap(input, boyNames);
Integer girlIndex = searchNameInMap(input, girlNames);
String capitalizedInput = Character.toUpperCase(input.charAt(0)) + input.substring(1).toLowerCase();
if (boyIndex == null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was not found in either list.");
} else if (boyIndex != null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular boy names list (line " + boyIndex + ").");
} else if (boyIndex == null && girlIndex != null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular girl names list (line " + girlIndex + ").");
} else {
System.out.println("The name '" + capitalizedInput + "' was found in both lists: boy names (line " + boyIndex + ") and girl names (line " + girlIndex + ").");
}
}
scanner.close();
}
}
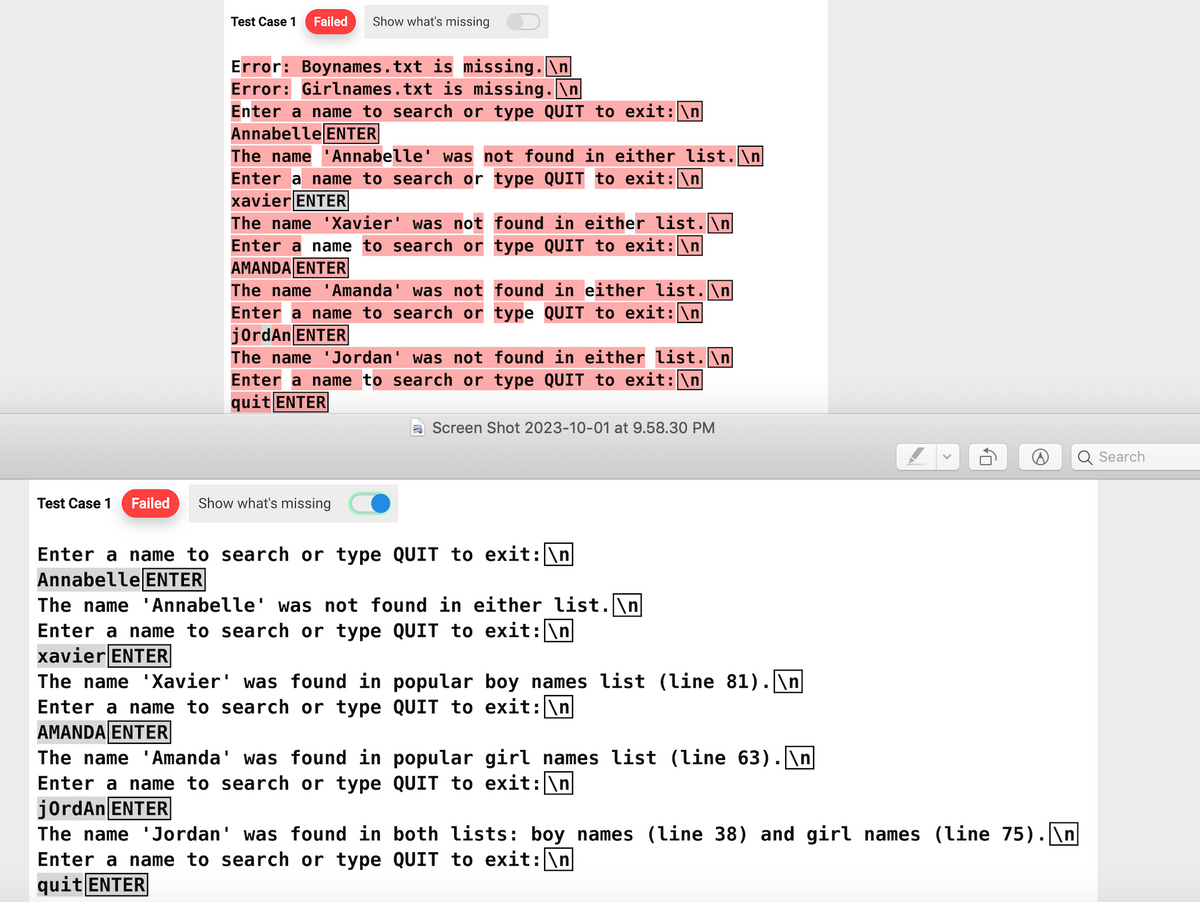
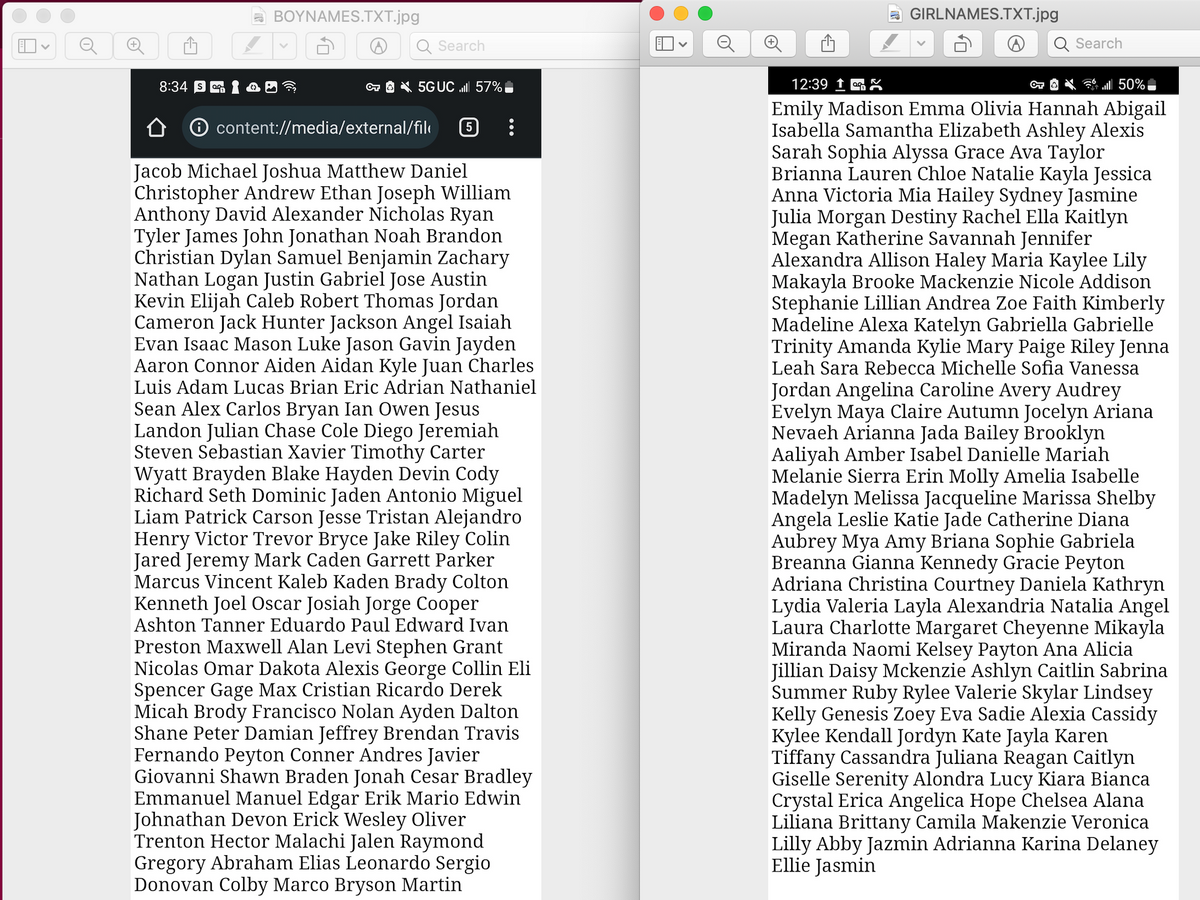

Step by step
Solved in 3 steps with 1 images

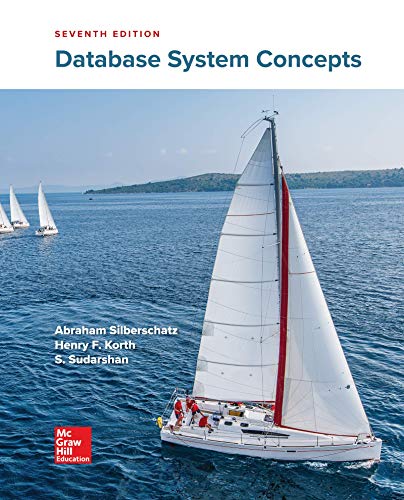
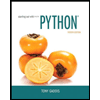
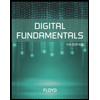
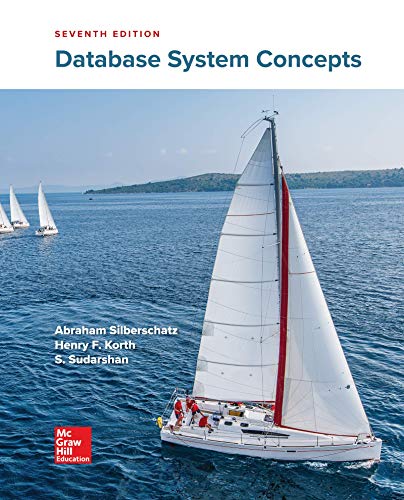
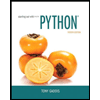
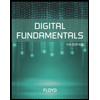
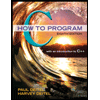
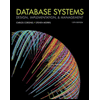
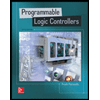