JAVA Using the code provided below called intSet create an iterator called sortedElements and the inner class. This will return an interator that will produce each element of the inset in ascending order. Specify and implement an iterator method called sortedElements for IntSet. The iterator method sortedElements returns an iterator that will produce each element of the intset in ascending order. The intset cannot be mutated when the iterator is in use. Be sure to specify the iterator method before implementation. Do not forget to give the rep invariant and abstraction function for your implementation. Note: IntSet.java has been provided. You can directly add in the code the specification, the implementation of the iterator method sortedElements, and the inner iterator class. There is a segment of simple testing code for sortedElements in main() close to the end in the provided IntSet.java. The program will not compile until you have properly implemented sortedElements //provided code and tester class import java.util.ArrayList;import java.util.Iterator;import java.util.NoSuchElementException; /*** OVERVIEW: IntSets are unbounded, mutable sets of integers. A typical IntSet* is {x1,...,xn}.*/public class IntSet { /*** EFFECTS: Constructor. Initializes this to be empty.*/public IntSet() {els = new ArrayList<>();} /*** MODIFIES: this** EFFECTS: Adds x to the elements of this.*/public void insert(int x) {if (getIndex(x) < 0) {els.add(x);}} /*** MODIFIES: this** EFFECTS: Removes x from this.*/public void remove(int x) {int i = getIndex(x);if (i < 0) {return;}els.set(i, els.get(els.size() - 1));els.remove(els.size() - 1);} /*** EFFECTS: Returns true if x is in this; else returns false.*/public boolean isIn(int x) {return getIndex(x) >= 0;} /*** EFFECTS: Returns the cardinality of this.*/public int size() {return els.size();} /*** EFFECTS: If this is empty throws EmptyException; else returns an* arbitrary element of this.*/public int choose() throws EmptyException {if (els.isEmpty()) {throw new EmptyException("IntSet.choose");}return els.get(0);} /*** EFFECTS: Returns an iterator that produces all the elements of this (as* Integers), each exactly once, in arbitrary order.** REQUIRES: this must not be modified while the iterator is in use.*/public Iterator elements() {return els.iterator();} /*** EFFECTS: Returns an iterator that produces all the elements of this (as* Integers), each exactly once, in arbitrary order.** REQUIRES: this must not be modified while the iterator is in use.*/public Iterator elements2() {return new IntSetGen(this);} // inner class private static class IntSetGen implements Iterator { IntSetGen(IntSet set) {// REQUIRES: set != nulls = set;n = 0;} @Overridepublic boolean hasNext() {return n < s.els.size();} @Overridepublic Integer next() throws NoSuchElementException {if (hasNext()) {n++;return s.els.get(n - 1);} else {throw new NoSuchElementException("IntSet.elements");}} // unsupported@Overridepublic void remove() {throw new UnsupportedOperationException("IntSet.elements");} // The abstraction function for IntSet1Gen is:// AF(c) = [c.s.els[n], c.s.els[n + 1], ...]// The rep invariant for IntSet1Gen is // I(c) = c.s != null && (0 <= c.n <= c.s.size)private IntSet s; // the IntSet object being iteratedprivate int n; // the index of the next element in els to return} // end IntSetGen @Overridepublic IntSet clone() {return new IntSet(els);} public boolean equals(IntSet s) {if (s == null || els.size() != s.els.size()) {return false;} for (var val : els) {if (s.els.indexOf(val) == -1) {return false;}}return true;} @Overridepublic boolean equals(Object o) {if (!(o instanceof IntSet)) {return false;} return equals((IntSet) o);} @Overridepublic String toString() {if (els.isEmpty()) {return "IntSet: { }";}String s = "IntSet: {" + els.get(0);for (int i = 1; i < els.size(); i++) {s = s + ", " + els.get(i);}return s + "}";} public boolean repOk() {if (els == null) {return false;}for (int i = 0; i < els.size(); i++) {int x = els.get(i);for (int j = i + 1; j < els.size(); j++) {if (x == els.get(j)) {return false;}}}return true;} /*** REQUIRES: v is not null.** EFFECTS: Private Constructor. Initializes this to have the same elements* as those in v.*/private IntSet(ArrayList v) {els = new ArrayList<>();for (var val : v) {els.add(val);}} /*** EFFECTS: If x is in this returns the index where x appears; else returns* -1.*/private int getIndex(Integer x) {for (int i = 0; i < els.size(); i++) {if (x.equals(els.get(i))) {return i;}}return -1;} // A typical IntSet is {x1,...,xn}. // The abstraction function is// AF(c) = { c.els[i] | 0 <= i < c.els.size }// The rep invariant is // I(c) = c.els != null &&// for all int i, j, 0 < i, j < c.els.size &&// i != j => c.els[i] != c.els[j] private ArrayList els; // the rep
JAVA
Using the code provided below called intSet create an iterator called sortedElements and the inner class. This will return an interator that will produce each element of the inset in ascending order.
- Specify and implement an iterator method called sortedElements for IntSet. The iterator method sortedElements returns an iterator that will produce each element of the intset in ascending order. The intset cannot be mutated when the iterator is in use.
Be sure to specify the iterator method before implementation. Do not forget to give the rep invariant and abstraction function for your implementation.
Note: IntSet.java has been provided. You can directly add in the code the specification, the implementation of the iterator method sortedElements, and the inner iterator class.
There is a segment of simple testing code for sortedElements in main() close to the end in the provided IntSet.java. The program will not compile until you have properly implemented sortedElements
//provided code and tester class
import java.util.ArrayList;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* OVERVIEW: IntSets are unbounded, mutable sets of integers. A typical IntSet
* is {x1,...,xn}.
*/
public class IntSet {
/**
* EFFECTS: Constructor. Initializes this to be empty.
*/
public IntSet() {
els = new ArrayList<>();
}
/**
* MODIFIES: this
*
* EFFECTS: Adds x to the elements of this.
*/
public void insert(int x) {
if (getIndex(x) < 0) {
els.add(x);
}
}
/**
* MODIFIES: this
*
* EFFECTS: Removes x from this.
*/
public void remove(int x) {
int i = getIndex(x);
if (i < 0) {
return;
}
els.set(i, els.get(els.size() - 1));
els.remove(els.size() - 1);
}
/**
* EFFECTS: Returns true if x is in this; else returns false.
*/
public boolean isIn(int x) {
return getIndex(x) >= 0;
}
/**
* EFFECTS: Returns the cardinality of this.
*/
public int size() {
return els.size();
}
/**
* EFFECTS: If this is empty throws EmptyException; else returns an
* arbitrary element of this.
*/
public int choose() throws EmptyException {
if (els.isEmpty()) {
throw new EmptyException("IntSet.choose");
}
return els.get(0);
}
/**
* EFFECTS: Returns an iterator that produces all the elements of this (as
* Integers), each exactly once, in arbitrary order.
*
* REQUIRES: this must not be modified while the iterator is in use.
*/
public Iterator elements() {
return els.iterator();
}
/**
* EFFECTS: Returns an iterator that produces all the elements of this (as
* Integers), each exactly once, in arbitrary order.
*
* REQUIRES: this must not be modified while the iterator is in use.
*/
public Iterator elements2() {
return new IntSetGen(this);
}
// inner class
private static class IntSetGen implements Iterator {
IntSetGen(IntSet set) {
// REQUIRES: set != null
s = set;
n = 0;
}
@Override
public boolean hasNext() {
return n < s.els.size();
}
@Override
public Integer next() throws NoSuchElementException {
if (hasNext()) {
n++;
return s.els.get(n - 1);
} else {
throw new NoSuchElementException("IntSet.elements");
}
}
// unsupported
@Override
public void remove() {
throw new UnsupportedOperationException("IntSet.elements");
}
// The abstraction function for IntSet1Gen is:
// AF(c) = [c.s.els[n], c.s.els[n + 1], ...]
// The rep invariant for IntSet1Gen is
// I(c) = c.s != null && (0 <= c.n <= c.s.size)
private IntSet s; // the IntSet object being iterated
private int n; // the index of the next element in els to return
} // end IntSetGen
@Override
public IntSet clone() {
return new IntSet(els);
}
public boolean equals(IntSet s) {
if (s == null || els.size() != s.els.size()) {
return false;
}
for (var val : els) {
if (s.els.indexOf(val) == -1) {
return false;
}
}
return true;
}
@Override
public boolean equals(Object o) {
if (!(o instanceof IntSet)) {
return false;
}
return equals((IntSet) o);
}
@Override
public String toString() {
if (els.isEmpty()) {
return "IntSet: { }";
}
String s = "IntSet: {" + els.get(0);
for (int i = 1; i < els.size(); i++) {
s = s + ", " + els.get(i);
}
return s + "}";
}
public boolean repOk() {
if (els == null) {
return false;
}
for (int i = 0; i < els.size(); i++) {
int x = els.get(i);
for (int j = i + 1; j < els.size(); j++) {
if (x == els.get(j)) {
return false;
}
}
}
return true;
}
/**
* REQUIRES: v is not null.
*
* EFFECTS: Private Constructor. Initializes this to have the same elements
* as those in v.
*/
private IntSet(ArrayList v) {
els = new ArrayList<>();
for (var val : v) {
els.add(val);
}
}
/**
* EFFECTS: If x is in this returns the index where x appears; else returns
* -1.
*/
private int getIndex(Integer x) {
for (int i = 0; i < els.size(); i++) {
if (x.equals(els.get(i))) {
return i;
}
}
return -1;
}
// A typical IntSet is {x1,...,xn}.
// The abstraction function is
// AF(c) = { c.els[i] | 0 <= i < c.els.size }
// The rep invariant is
// I(c) = c.els != null &&
// for all int i, j, 0 < i, j < c.els.size &&
// i != j => c.els[i] != c.els[j]
private ArrayList els; // the rep

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

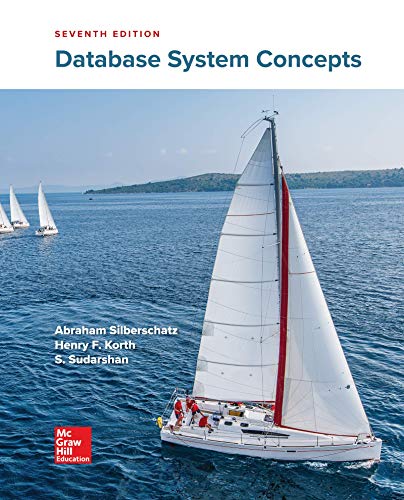
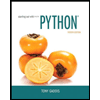
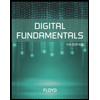
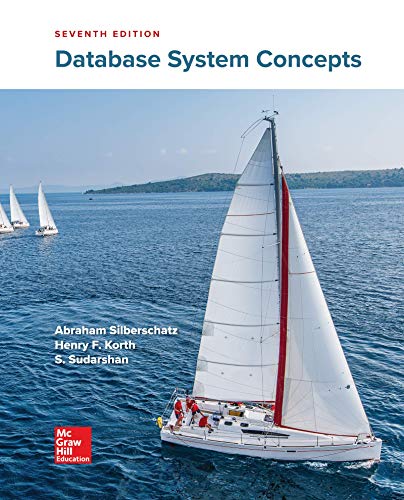
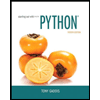
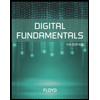
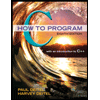
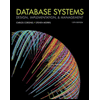
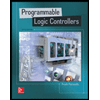