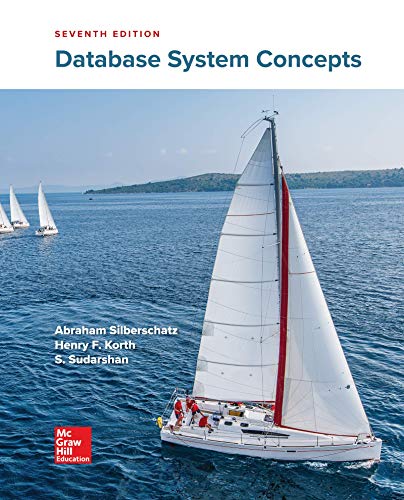
Concept explainers
Specify and implement an iterator method called sortedElements for IntSet. The iterator method sortedElements returns an iterator that will produce each element of the intset in ascending order. The intset cannot be mutated when the iterator is in use.
Be sure to specify the iterator method before implementation. Do not forget to give the rep invariant and abstraction function for your implementation.
Note: IntSet.java has been provided. You can directly add in the code the specification, the implementation of the iterator method sortedElements, and the inner iterator class.
There is a segment of simple testing code for sortedElements in main() close to the end in the provided IntSet.java. The program will not compile until you have properly implemented sortedElements.
find the provided code below:
https://drive.google.com/open?id=1hANO8kVigGXwFLLJDJQLE5joM1BBeCCn

Step by stepSolved in 3 steps with 1 images

- Please answer the question in the screenshot. The language used is Java.arrow_forwardJava Code: Below are the methods for parser.java. Make sure to use the existing Parser.java file to add in all the methods and show the output. OperationNode: Has enum, left and Optional right members, good constructors and ToString is good VariableReferenceNode: Has name and Optional index, good constructors and ToString is good Constant & Node Pattern: Have name, good constructor and ToString is good ParseLValue - variables: Accepts a variable name and creates an appropriate Variable Reference Node ParseLValue - arrays: Accepts a name, appropriately gets an index and creates an appropriate Variable Reference Node ParseLValue - dollar: Creates an operation node, gets the value of the $ operator appropriately ParseBottomLevel – constants & patterns: Detects strings, numbers and patterns and creates appropriate nodes ParseBottomLevel – parenthesis: Creates an operation node AND gets the contents of the parenthesis appropriately ParseBottomLevel – unary operators: All…arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forward
- The two classes you will create will implement the operations defined in the interface as shown in the UML class diagram above. In addition, BinarySearchArray will implement a static method testBinarySearchArray() that populates the lists, and lets the user interactively test the two classes by adding and removing elements. The parameter BinarySearch can represent either class and tells testBinarySearchArray which class to test. Steps to Implement: 1) To get started create a new project in IntelliJ called BinarySearch. Add a class to your project called BinarySearchArray. Then add another class BinarySearchArrayList and an interface called BinarySearch. The interface BinarySearch includes public method stubs as shown in the diagram. You are allowed to add BinarySearchArrayList to the same file as BinarySearch but don't add an access modifier to this class, or for easier reading, you can declare the classes in separate files with public access modifiers. Only the class…arrow_forwardwriting a general-purpose sort method in Java, the sort method needs to compareobjects. There are two ways to give the sort method the code to compare objects,implementing the interfaces Comparable and Comparator respectively. Briefly, describe thetwo ways. Write excerpts of use examplesarrow_forwardI had an error with grades[i] = stoi because it is saying that stoi is not declared. What should I do to fix this?arrow_forward
- Java Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardIn this project, you will implement a Set class that represents a general collection of values. For this assignment, a set is generally defined as a list of values that are sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. (in java)Requirements among all implementations there are some requirements that all implementations must maintain. • Your implementation should always reflect the definition of a set. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections. the sort that automatically sorts a list may not be used. Instead, when a successful addition of an element to the Set is done, you can ensure that the elements inside the ArrayList<Integer>…arrow_forwardHi, I am a bit lost on how to do this coding assignment. It would be great if someone could help me fill in the MyStack.java file with the given information. All the information is in the pictures. Thank you!arrow_forward
- For the Assignment 5 (Part 2), Routes v.2 at the end of this module you will create a Route class that has a member a "bag" of Leg objects. The bag is to use a vector of pointers, but since all the objects in the bag are to be Leg s, it's okay to use Leg* instead of void* in the bag's declaration. You wrote its first constructor in a previous exercise. Now write a second one. (You may refer to the Assignment page to refer to the completed class declarations of Leg and Route classes). Assume that class Leg has data member C strings const char* const beginCity; and const char* const endCity; that are available to Route by a friend relationship. Write a constructor function for a Route class that takes exactly two parameters: a constant Route object reference to an already-existing Route , and a constant Leg object reference to be appended to the end of that Route to form the new Route . Here's the algorithm: Copy the first parameter's bag into the host object's bag. If the endCity of…arrow_forwardVariableReferenceNode.java, OperationNode.java, ConstantNode.java, and PatternNode.java must have their own java classes with the correct methods implemented: OperationNode: Has enum, left and Optional right members, good constructors and ToString is good VariableReferenceNode: Has name and Optional index, good constructors and ToString is good Constant & Node Pattern: Have name, good constructor and ToString is good Make sure to include the screenshot of the output of Parser.java as well.arrow_forwardIn this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
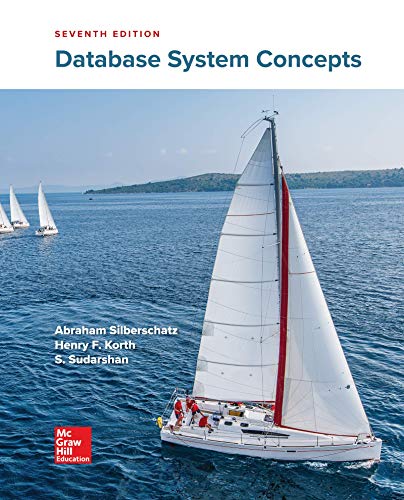
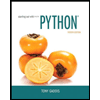
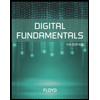
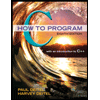
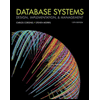
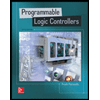